| // Copyright 2023 Google LLC |
| // |
| // Licensed under the Apache License, Version 2.0 (the "License"); |
| // you may not use this file except in compliance with the License. |
| // You may obtain a copy of the License at |
| // |
| // http://www.apache.org/licenses/LICENSE-2.0 |
| // |
| // Unless required by applicable law or agreed to in writing, software |
| // distributed under the License is distributed on an "AS IS" BASIS, |
| // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
| // See the License for the specific language governing permissions and |
| // limitations under the License. |
| |
| // Code generated by protoc-gen-go. DO NOT EDIT. |
| // versions: |
| // protoc-gen-go v1.26.0 |
| // protoc v4.24.4 |
| // source: google/apps/card/v1/card.proto |
| |
| package card |
| |
| import ( |
| reflect "reflect" |
| sync "sync" |
| |
| color "google.golang.org/genproto/googleapis/type/color" |
| protoreflect "google.golang.org/protobuf/reflect/protoreflect" |
| protoimpl "google.golang.org/protobuf/runtime/protoimpl" |
| ) |
| |
| const ( |
| // Verify that this generated code is sufficiently up-to-date. |
| _ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion) |
| // Verify that runtime/protoimpl is sufficiently up-to-date. |
| _ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20) |
| ) |
| |
| // The divider style of a card. Currently only used for dividers betweens card |
| // sections. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Card_DividerStyle int32 |
| |
| const ( |
| // Don't use. Unspecified. |
| Card_DIVIDER_STYLE_UNSPECIFIED Card_DividerStyle = 0 |
| // Default option. Render a solid divider between sections. |
| Card_SOLID_DIVIDER Card_DividerStyle = 1 |
| // If set, no divider is rendered between sections. |
| Card_NO_DIVIDER Card_DividerStyle = 2 |
| ) |
| |
| // Enum value maps for Card_DividerStyle. |
| var ( |
| Card_DividerStyle_name = map[int32]string{ |
| 0: "DIVIDER_STYLE_UNSPECIFIED", |
| 1: "SOLID_DIVIDER", |
| 2: "NO_DIVIDER", |
| } |
| Card_DividerStyle_value = map[string]int32{ |
| "DIVIDER_STYLE_UNSPECIFIED": 0, |
| "SOLID_DIVIDER": 1, |
| "NO_DIVIDER": 2, |
| } |
| ) |
| |
| func (x Card_DividerStyle) Enum() *Card_DividerStyle { |
| p := new(Card_DividerStyle) |
| *p = x |
| return p |
| } |
| |
| func (x Card_DividerStyle) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (Card_DividerStyle) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[0].Descriptor() |
| } |
| |
| func (Card_DividerStyle) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[0] |
| } |
| |
| func (x Card_DividerStyle) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use Card_DividerStyle.Descriptor instead. |
| func (Card_DividerStyle) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{0, 0} |
| } |
| |
| // In Google Workspace Add-ons, |
| // determines how a card is displayed. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| type Card_DisplayStyle int32 |
| |
| const ( |
| // Don't use. Unspecified. |
| Card_DISPLAY_STYLE_UNSPECIFIED Card_DisplayStyle = 0 |
| // The header of the card appears at the bottom of the |
| // sidebar, partially covering the current top card of the stack. Clicking |
| // the header pops the card into the card stack. If the card has no header, |
| // a generated header is used instead. |
| Card_PEEK Card_DisplayStyle = 1 |
| // Default value. The card is shown by replacing the view of the top card in |
| // the card stack. |
| Card_REPLACE Card_DisplayStyle = 2 |
| ) |
| |
| // Enum value maps for Card_DisplayStyle. |
| var ( |
| Card_DisplayStyle_name = map[int32]string{ |
| 0: "DISPLAY_STYLE_UNSPECIFIED", |
| 1: "PEEK", |
| 2: "REPLACE", |
| } |
| Card_DisplayStyle_value = map[string]int32{ |
| "DISPLAY_STYLE_UNSPECIFIED": 0, |
| "PEEK": 1, |
| "REPLACE": 2, |
| } |
| ) |
| |
| func (x Card_DisplayStyle) Enum() *Card_DisplayStyle { |
| p := new(Card_DisplayStyle) |
| *p = x |
| return p |
| } |
| |
| func (x Card_DisplayStyle) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (Card_DisplayStyle) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[1].Descriptor() |
| } |
| |
| func (Card_DisplayStyle) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[1] |
| } |
| |
| func (x Card_DisplayStyle) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use Card_DisplayStyle.Descriptor instead. |
| func (Card_DisplayStyle) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{0, 1} |
| } |
| |
| // The shape used to crop the image. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Widget_ImageType int32 |
| |
| const ( |
| // Default value. Applies a square mask to the image. For example, a 4x3 |
| // image becomes 3x3. |
| Widget_SQUARE Widget_ImageType = 0 |
| // Applies a circular mask to the image. For example, a 4x3 image becomes a |
| // circle with a diameter of 3. |
| Widget_CIRCLE Widget_ImageType = 1 |
| ) |
| |
| // Enum value maps for Widget_ImageType. |
| var ( |
| Widget_ImageType_name = map[int32]string{ |
| 0: "SQUARE", |
| 1: "CIRCLE", |
| } |
| Widget_ImageType_value = map[string]int32{ |
| "SQUARE": 0, |
| "CIRCLE": 1, |
| } |
| ) |
| |
| func (x Widget_ImageType) Enum() *Widget_ImageType { |
| p := new(Widget_ImageType) |
| *p = x |
| return p |
| } |
| |
| func (x Widget_ImageType) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (Widget_ImageType) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[2].Descriptor() |
| } |
| |
| func (Widget_ImageType) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[2] |
| } |
| |
| func (x Widget_ImageType) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use Widget_ImageType.Descriptor instead. |
| func (Widget_ImageType) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{1, 0} |
| } |
| |
| // Specifies whether widgets align to the left, right, or center of a column. |
| // |
| // [Google Chat apps](https://developers.google.com/workspace/chat): |
| type Widget_HorizontalAlignment int32 |
| |
| const ( |
| // Don't use. Unspecified. |
| Widget_HORIZONTAL_ALIGNMENT_UNSPECIFIED Widget_HorizontalAlignment = 0 |
| // Default value. Aligns widgets to the start position of the column. For |
| // left-to-right layouts, aligns to the left. For right-to-left layouts, |
| // aligns to the right. |
| Widget_START Widget_HorizontalAlignment = 1 |
| // Aligns widgets to the center of the column. |
| Widget_CENTER Widget_HorizontalAlignment = 2 |
| // Aligns widgets to the end position of the column. For left-to-right |
| // layouts, aligns widgets to the right. For right-to-left layouts, aligns |
| // widgets to the left. |
| Widget_END Widget_HorizontalAlignment = 3 |
| ) |
| |
| // Enum value maps for Widget_HorizontalAlignment. |
| var ( |
| Widget_HorizontalAlignment_name = map[int32]string{ |
| 0: "HORIZONTAL_ALIGNMENT_UNSPECIFIED", |
| 1: "START", |
| 2: "CENTER", |
| 3: "END", |
| } |
| Widget_HorizontalAlignment_value = map[string]int32{ |
| "HORIZONTAL_ALIGNMENT_UNSPECIFIED": 0, |
| "START": 1, |
| "CENTER": 2, |
| "END": 3, |
| } |
| ) |
| |
| func (x Widget_HorizontalAlignment) Enum() *Widget_HorizontalAlignment { |
| p := new(Widget_HorizontalAlignment) |
| *p = x |
| return p |
| } |
| |
| func (x Widget_HorizontalAlignment) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (Widget_HorizontalAlignment) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[3].Descriptor() |
| } |
| |
| func (Widget_HorizontalAlignment) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[3] |
| } |
| |
| func (x Widget_HorizontalAlignment) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use Widget_HorizontalAlignment.Descriptor instead. |
| func (Widget_HorizontalAlignment) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{1, 1} |
| } |
| |
| // How the switch appears in the user interface. |
| // |
| // [Google Workspace Add-ons |
| // and Chat apps](https://developers.google.com/workspace/extend): |
| type DecoratedText_SwitchControl_ControlType int32 |
| |
| const ( |
| // A toggle-style switch. |
| DecoratedText_SwitchControl_SWITCH DecoratedText_SwitchControl_ControlType = 0 |
| // Deprecated in favor of `CHECK_BOX`. |
| DecoratedText_SwitchControl_CHECKBOX DecoratedText_SwitchControl_ControlType = 1 |
| // A checkbox. |
| DecoratedText_SwitchControl_CHECK_BOX DecoratedText_SwitchControl_ControlType = 2 |
| ) |
| |
| // Enum value maps for DecoratedText_SwitchControl_ControlType. |
| var ( |
| DecoratedText_SwitchControl_ControlType_name = map[int32]string{ |
| 0: "SWITCH", |
| 1: "CHECKBOX", |
| 2: "CHECK_BOX", |
| } |
| DecoratedText_SwitchControl_ControlType_value = map[string]int32{ |
| "SWITCH": 0, |
| "CHECKBOX": 1, |
| "CHECK_BOX": 2, |
| } |
| ) |
| |
| func (x DecoratedText_SwitchControl_ControlType) Enum() *DecoratedText_SwitchControl_ControlType { |
| p := new(DecoratedText_SwitchControl_ControlType) |
| *p = x |
| return p |
| } |
| |
| func (x DecoratedText_SwitchControl_ControlType) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (DecoratedText_SwitchControl_ControlType) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[4].Descriptor() |
| } |
| |
| func (DecoratedText_SwitchControl_ControlType) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[4] |
| } |
| |
| func (x DecoratedText_SwitchControl_ControlType) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use DecoratedText_SwitchControl_ControlType.Descriptor instead. |
| func (DecoratedText_SwitchControl_ControlType) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{5, 0, 0} |
| } |
| |
| // How a text input field appears in the user interface. For example, |
| // whether it's a single line input field, or a multi-line input. If |
| // `initialSuggestions` is specified, `type` is always `SINGLE_LINE`, |
| // even if it's set to `MULTIPLE_LINE`. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type TextInput_Type int32 |
| |
| const ( |
| // The text input field has a fixed height of one line. |
| TextInput_SINGLE_LINE TextInput_Type = 0 |
| // The text input field has a fixed height of multiple lines. |
| TextInput_MULTIPLE_LINE TextInput_Type = 1 |
| ) |
| |
| // Enum value maps for TextInput_Type. |
| var ( |
| TextInput_Type_name = map[int32]string{ |
| 0: "SINGLE_LINE", |
| 1: "MULTIPLE_LINE", |
| } |
| TextInput_Type_value = map[string]int32{ |
| "SINGLE_LINE": 0, |
| "MULTIPLE_LINE": 1, |
| } |
| ) |
| |
| func (x TextInput_Type) Enum() *TextInput_Type { |
| p := new(TextInput_Type) |
| *p = x |
| return p |
| } |
| |
| func (x TextInput_Type) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (TextInput_Type) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[5].Descriptor() |
| } |
| |
| func (TextInput_Type) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[5] |
| } |
| |
| func (x TextInput_Type) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use TextInput_Type.Descriptor instead. |
| func (TextInput_Type) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{6, 0} |
| } |
| |
| // The format for the items that users can select. Different options support |
| // different types of interactions. For example, users can select multiple |
| // checkboxes, but can only select one item from a dropdown menu. |
| // |
| // Each selection input supports one type of selection. Mixing checkboxes |
| // and switches, for example, isn't supported. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type SelectionInput_SelectionType int32 |
| |
| const ( |
| // A set of checkboxes. Users can select one or more checkboxes. |
| SelectionInput_CHECK_BOX SelectionInput_SelectionType = 0 |
| // A set of radio buttons. Users can select one radio button. |
| SelectionInput_RADIO_BUTTON SelectionInput_SelectionType = 1 |
| // A set of switches. Users can turn on one or more switches. |
| SelectionInput_SWITCH SelectionInput_SelectionType = 2 |
| // A dropdown menu. Users can select one item from the menu. |
| SelectionInput_DROPDOWN SelectionInput_SelectionType = 3 |
| // A multiselect menu for static or dynamic data. From the menu bar, |
| // users select one or more items. Users can also input values to populate |
| // dynamic data. For example, users can start typing the name of a Google |
| // Chat space and the widget autosuggests the space. |
| // |
| // To populate items for a multiselect menu, you can use one of the |
| // following types of data sources: |
| // |
| // - Static data: Items are specified as `SelectionItem` objects in the |
| // widget. Up to 100 items. |
| // - Google Workspace data: Items are populated using data from Google |
| // Workspace, such as Google Workspace users or Google Chat spaces. |
| // - External data: Items are populated from an external data |
| // source outside of Google Workspace. |
| // |
| // For examples of how to implement multiselect menus, see |
| // [Add a multiselect |
| // menu](https://developers.google.com/workspace/chat/design-interactive-card-dialog#multiselect-menu). |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| // Multiselect for Google Workspace Add-ons are in |
| // Developer Preview. |
| SelectionInput_MULTI_SELECT SelectionInput_SelectionType = 4 |
| ) |
| |
| // Enum value maps for SelectionInput_SelectionType. |
| var ( |
| SelectionInput_SelectionType_name = map[int32]string{ |
| 0: "CHECK_BOX", |
| 1: "RADIO_BUTTON", |
| 2: "SWITCH", |
| 3: "DROPDOWN", |
| 4: "MULTI_SELECT", |
| } |
| SelectionInput_SelectionType_value = map[string]int32{ |
| "CHECK_BOX": 0, |
| "RADIO_BUTTON": 1, |
| "SWITCH": 2, |
| "DROPDOWN": 3, |
| "MULTI_SELECT": 4, |
| } |
| ) |
| |
| func (x SelectionInput_SelectionType) Enum() *SelectionInput_SelectionType { |
| p := new(SelectionInput_SelectionType) |
| *p = x |
| return p |
| } |
| |
| func (x SelectionInput_SelectionType) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (SelectionInput_SelectionType) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[6].Descriptor() |
| } |
| |
| func (SelectionInput_SelectionType) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[6] |
| } |
| |
| func (x SelectionInput_SelectionType) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use SelectionInput_SelectionType.Descriptor instead. |
| func (SelectionInput_SelectionType) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{9, 0} |
| } |
| |
| // A data source shared by all [Google Workspace |
| // applications] |
| // (https://developers.google.com/workspace/chat/api/reference/rest/v1/HostApp). |
| // |
| // [Google Chat apps](https://developers.google.com/workspace/chat): |
| type SelectionInput_PlatformDataSource_CommonDataSource int32 |
| |
| const ( |
| // Default value. Don't use. |
| SelectionInput_PlatformDataSource_UNKNOWN SelectionInput_PlatformDataSource_CommonDataSource = 0 |
| // Google Workspace users. The user can only view and select users from |
| // their Google Workspace organization. |
| SelectionInput_PlatformDataSource_USER SelectionInput_PlatformDataSource_CommonDataSource = 1 |
| ) |
| |
| // Enum value maps for SelectionInput_PlatformDataSource_CommonDataSource. |
| var ( |
| SelectionInput_PlatformDataSource_CommonDataSource_name = map[int32]string{ |
| 0: "UNKNOWN", |
| 1: "USER", |
| } |
| SelectionInput_PlatformDataSource_CommonDataSource_value = map[string]int32{ |
| "UNKNOWN": 0, |
| "USER": 1, |
| } |
| ) |
| |
| func (x SelectionInput_PlatformDataSource_CommonDataSource) Enum() *SelectionInput_PlatformDataSource_CommonDataSource { |
| p := new(SelectionInput_PlatformDataSource_CommonDataSource) |
| *p = x |
| return p |
| } |
| |
| func (x SelectionInput_PlatformDataSource_CommonDataSource) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (SelectionInput_PlatformDataSource_CommonDataSource) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[7].Descriptor() |
| } |
| |
| func (SelectionInput_PlatformDataSource_CommonDataSource) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[7] |
| } |
| |
| func (x SelectionInput_PlatformDataSource_CommonDataSource) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use SelectionInput_PlatformDataSource_CommonDataSource.Descriptor instead. |
| func (SelectionInput_PlatformDataSource_CommonDataSource) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{9, 1, 0} |
| } |
| |
| // The format for the date and time in the `DateTimePicker` widget. |
| // Determines whether users can input a date, a time, or both a date and time. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type DateTimePicker_DateTimePickerType int32 |
| |
| const ( |
| // Users input a date and time. |
| DateTimePicker_DATE_AND_TIME DateTimePicker_DateTimePickerType = 0 |
| // Users input a date. |
| DateTimePicker_DATE_ONLY DateTimePicker_DateTimePickerType = 1 |
| // Users input a time. |
| DateTimePicker_TIME_ONLY DateTimePicker_DateTimePickerType = 2 |
| ) |
| |
| // Enum value maps for DateTimePicker_DateTimePickerType. |
| var ( |
| DateTimePicker_DateTimePickerType_name = map[int32]string{ |
| 0: "DATE_AND_TIME", |
| 1: "DATE_ONLY", |
| 2: "TIME_ONLY", |
| } |
| DateTimePicker_DateTimePickerType_value = map[string]int32{ |
| "DATE_AND_TIME": 0, |
| "DATE_ONLY": 1, |
| "TIME_ONLY": 2, |
| } |
| ) |
| |
| func (x DateTimePicker_DateTimePickerType) Enum() *DateTimePicker_DateTimePickerType { |
| p := new(DateTimePicker_DateTimePickerType) |
| *p = x |
| return p |
| } |
| |
| func (x DateTimePicker_DateTimePickerType) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (DateTimePicker_DateTimePickerType) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[8].Descriptor() |
| } |
| |
| func (DateTimePicker_DateTimePickerType) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[8] |
| } |
| |
| func (x DateTimePicker_DateTimePickerType) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use DateTimePicker_DateTimePickerType.Descriptor instead. |
| func (DateTimePicker_DateTimePickerType) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{10, 0} |
| } |
| |
| // Represents the crop style applied to an image. |
| // |
| // [Google Workspace Add-ons |
| // and Chat apps](https://developers.google.com/workspace/extend): |
| type ImageCropStyle_ImageCropType int32 |
| |
| const ( |
| // Don't use. Unspecified. |
| ImageCropStyle_IMAGE_CROP_TYPE_UNSPECIFIED ImageCropStyle_ImageCropType = 0 |
| // Default value. Applies a square crop. |
| ImageCropStyle_SQUARE ImageCropStyle_ImageCropType = 1 |
| // Applies a circular crop. |
| ImageCropStyle_CIRCLE ImageCropStyle_ImageCropType = 2 |
| // Applies a rectangular crop with a custom aspect ratio. Set the custom |
| // aspect ratio with `aspectRatio`. |
| ImageCropStyle_RECTANGLE_CUSTOM ImageCropStyle_ImageCropType = 3 |
| // Applies a rectangular crop with a 4:3 aspect ratio. |
| ImageCropStyle_RECTANGLE_4_3 ImageCropStyle_ImageCropType = 4 |
| ) |
| |
| // Enum value maps for ImageCropStyle_ImageCropType. |
| var ( |
| ImageCropStyle_ImageCropType_name = map[int32]string{ |
| 0: "IMAGE_CROP_TYPE_UNSPECIFIED", |
| 1: "SQUARE", |
| 2: "CIRCLE", |
| 3: "RECTANGLE_CUSTOM", |
| 4: "RECTANGLE_4_3", |
| } |
| ImageCropStyle_ImageCropType_value = map[string]int32{ |
| "IMAGE_CROP_TYPE_UNSPECIFIED": 0, |
| "SQUARE": 1, |
| "CIRCLE": 2, |
| "RECTANGLE_CUSTOM": 3, |
| "RECTANGLE_4_3": 4, |
| } |
| ) |
| |
| func (x ImageCropStyle_ImageCropType) Enum() *ImageCropStyle_ImageCropType { |
| p := new(ImageCropStyle_ImageCropType) |
| *p = x |
| return p |
| } |
| |
| func (x ImageCropStyle_ImageCropType) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (ImageCropStyle_ImageCropType) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[9].Descriptor() |
| } |
| |
| func (ImageCropStyle_ImageCropType) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[9] |
| } |
| |
| func (x ImageCropStyle_ImageCropType) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use ImageCropStyle_ImageCropType.Descriptor instead. |
| func (ImageCropStyle_ImageCropType) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{14, 0} |
| } |
| |
| // Represents the border types applied to widgets. |
| // |
| // [Google Workspace Add-ons |
| // and Chat apps](https://developers.google.com/workspace/extend): |
| type BorderStyle_BorderType int32 |
| |
| const ( |
| // Don't use. Unspecified. |
| BorderStyle_BORDER_TYPE_UNSPECIFIED BorderStyle_BorderType = 0 |
| // Default value. No border. |
| BorderStyle_NO_BORDER BorderStyle_BorderType = 1 |
| // Outline. |
| BorderStyle_STROKE BorderStyle_BorderType = 2 |
| ) |
| |
| // Enum value maps for BorderStyle_BorderType. |
| var ( |
| BorderStyle_BorderType_name = map[int32]string{ |
| 0: "BORDER_TYPE_UNSPECIFIED", |
| 1: "NO_BORDER", |
| 2: "STROKE", |
| } |
| BorderStyle_BorderType_value = map[string]int32{ |
| "BORDER_TYPE_UNSPECIFIED": 0, |
| "NO_BORDER": 1, |
| "STROKE": 2, |
| } |
| ) |
| |
| func (x BorderStyle_BorderType) Enum() *BorderStyle_BorderType { |
| p := new(BorderStyle_BorderType) |
| *p = x |
| return p |
| } |
| |
| func (x BorderStyle_BorderType) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (BorderStyle_BorderType) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[10].Descriptor() |
| } |
| |
| func (BorderStyle_BorderType) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[10] |
| } |
| |
| func (x BorderStyle_BorderType) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use BorderStyle_BorderType.Descriptor instead. |
| func (BorderStyle_BorderType) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{15, 0} |
| } |
| |
| // Represents the various layout options available for a grid item. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Grid_GridItem_GridItemLayout int32 |
| |
| const ( |
| // Don't use. Unspecified. |
| Grid_GridItem_GRID_ITEM_LAYOUT_UNSPECIFIED Grid_GridItem_GridItemLayout = 0 |
| // The title and subtitle are shown below the grid item's image. |
| Grid_GridItem_TEXT_BELOW Grid_GridItem_GridItemLayout = 1 |
| // The title and subtitle are shown above the grid item's image. |
| Grid_GridItem_TEXT_ABOVE Grid_GridItem_GridItemLayout = 2 |
| ) |
| |
| // Enum value maps for Grid_GridItem_GridItemLayout. |
| var ( |
| Grid_GridItem_GridItemLayout_name = map[int32]string{ |
| 0: "GRID_ITEM_LAYOUT_UNSPECIFIED", |
| 1: "TEXT_BELOW", |
| 2: "TEXT_ABOVE", |
| } |
| Grid_GridItem_GridItemLayout_value = map[string]int32{ |
| "GRID_ITEM_LAYOUT_UNSPECIFIED": 0, |
| "TEXT_BELOW": 1, |
| "TEXT_ABOVE": 2, |
| } |
| ) |
| |
| func (x Grid_GridItem_GridItemLayout) Enum() *Grid_GridItem_GridItemLayout { |
| p := new(Grid_GridItem_GridItemLayout) |
| *p = x |
| return p |
| } |
| |
| func (x Grid_GridItem_GridItemLayout) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (Grid_GridItem_GridItemLayout) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[11].Descriptor() |
| } |
| |
| func (Grid_GridItem_GridItemLayout) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[11] |
| } |
| |
| func (x Grid_GridItem_GridItemLayout) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use Grid_GridItem_GridItemLayout.Descriptor instead. |
| func (Grid_GridItem_GridItemLayout) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{17, 0, 0} |
| } |
| |
| // Specifies how a column fills the width of the card. The width of each |
| // column depends on both the `HorizontalSizeStyle` and the width of the |
| // widgets within the column. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| // Columns for Google Workspace Add-ons are in |
| // Developer Preview. |
| type Columns_Column_HorizontalSizeStyle int32 |
| |
| const ( |
| // Don't use. Unspecified. |
| Columns_Column_HORIZONTAL_SIZE_STYLE_UNSPECIFIED Columns_Column_HorizontalSizeStyle = 0 |
| // Default value. Column fills the available space, up to 70% of the |
| // card's width. If both columns are set to `FILL_AVAILABLE_SPACE`, each |
| // column fills 50% of the space. |
| Columns_Column_FILL_AVAILABLE_SPACE Columns_Column_HorizontalSizeStyle = 1 |
| // Column fills the least amount of space possible and no more than 30% of |
| // the card's width. |
| Columns_Column_FILL_MINIMUM_SPACE Columns_Column_HorizontalSizeStyle = 2 |
| ) |
| |
| // Enum value maps for Columns_Column_HorizontalSizeStyle. |
| var ( |
| Columns_Column_HorizontalSizeStyle_name = map[int32]string{ |
| 0: "HORIZONTAL_SIZE_STYLE_UNSPECIFIED", |
| 1: "FILL_AVAILABLE_SPACE", |
| 2: "FILL_MINIMUM_SPACE", |
| } |
| Columns_Column_HorizontalSizeStyle_value = map[string]int32{ |
| "HORIZONTAL_SIZE_STYLE_UNSPECIFIED": 0, |
| "FILL_AVAILABLE_SPACE": 1, |
| "FILL_MINIMUM_SPACE": 2, |
| } |
| ) |
| |
| func (x Columns_Column_HorizontalSizeStyle) Enum() *Columns_Column_HorizontalSizeStyle { |
| p := new(Columns_Column_HorizontalSizeStyle) |
| *p = x |
| return p |
| } |
| |
| func (x Columns_Column_HorizontalSizeStyle) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (Columns_Column_HorizontalSizeStyle) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[12].Descriptor() |
| } |
| |
| func (Columns_Column_HorizontalSizeStyle) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[12] |
| } |
| |
| func (x Columns_Column_HorizontalSizeStyle) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use Columns_Column_HorizontalSizeStyle.Descriptor instead. |
| func (Columns_Column_HorizontalSizeStyle) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{18, 0, 0} |
| } |
| |
| // Specifies whether widgets align to the top, bottom, or center of a |
| // column. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| // Columns for Google Workspace Add-ons are in |
| // Developer Preview. |
| type Columns_Column_VerticalAlignment int32 |
| |
| const ( |
| // Don't use. Unspecified. |
| Columns_Column_VERTICAL_ALIGNMENT_UNSPECIFIED Columns_Column_VerticalAlignment = 0 |
| // Default value. Aligns widgets to the center of a column. |
| Columns_Column_CENTER Columns_Column_VerticalAlignment = 1 |
| // Aligns widgets to the top of a column. |
| Columns_Column_TOP Columns_Column_VerticalAlignment = 2 |
| // Aligns widgets to the bottom of a column. |
| Columns_Column_BOTTOM Columns_Column_VerticalAlignment = 3 |
| ) |
| |
| // Enum value maps for Columns_Column_VerticalAlignment. |
| var ( |
| Columns_Column_VerticalAlignment_name = map[int32]string{ |
| 0: "VERTICAL_ALIGNMENT_UNSPECIFIED", |
| 1: "CENTER", |
| 2: "TOP", |
| 3: "BOTTOM", |
| } |
| Columns_Column_VerticalAlignment_value = map[string]int32{ |
| "VERTICAL_ALIGNMENT_UNSPECIFIED": 0, |
| "CENTER": 1, |
| "TOP": 2, |
| "BOTTOM": 3, |
| } |
| ) |
| |
| func (x Columns_Column_VerticalAlignment) Enum() *Columns_Column_VerticalAlignment { |
| p := new(Columns_Column_VerticalAlignment) |
| *p = x |
| return p |
| } |
| |
| func (x Columns_Column_VerticalAlignment) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (Columns_Column_VerticalAlignment) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[13].Descriptor() |
| } |
| |
| func (Columns_Column_VerticalAlignment) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[13] |
| } |
| |
| func (x Columns_Column_VerticalAlignment) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use Columns_Column_VerticalAlignment.Descriptor instead. |
| func (Columns_Column_VerticalAlignment) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{18, 0, 1} |
| } |
| |
| // When an `OnClick` action opens a link, then the client can either open it |
| // as a full-size window (if that's the frame used by the client), or an |
| // overlay (such as a pop-up). The implementation depends on the client |
| // platform capabilities, and the value selected might be ignored if the |
| // client doesn't support it. `FULL_SIZE` is supported by all clients. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| type OpenLink_OpenAs int32 |
| |
| const ( |
| // The link opens as a full-size window (if that's the frame used by the |
| // client). |
| OpenLink_FULL_SIZE OpenLink_OpenAs = 0 |
| // The link opens as an overlay, such as a pop-up. |
| OpenLink_OVERLAY OpenLink_OpenAs = 1 |
| ) |
| |
| // Enum value maps for OpenLink_OpenAs. |
| var ( |
| OpenLink_OpenAs_name = map[int32]string{ |
| 0: "FULL_SIZE", |
| 1: "OVERLAY", |
| } |
| OpenLink_OpenAs_value = map[string]int32{ |
| "FULL_SIZE": 0, |
| "OVERLAY": 1, |
| } |
| ) |
| |
| func (x OpenLink_OpenAs) Enum() *OpenLink_OpenAs { |
| p := new(OpenLink_OpenAs) |
| *p = x |
| return p |
| } |
| |
| func (x OpenLink_OpenAs) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (OpenLink_OpenAs) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[14].Descriptor() |
| } |
| |
| func (OpenLink_OpenAs) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[14] |
| } |
| |
| func (x OpenLink_OpenAs) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use OpenLink_OpenAs.Descriptor instead. |
| func (OpenLink_OpenAs) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{20, 0} |
| } |
| |
| // What the client does when a link opened by an `OnClick` action is closed. |
| // |
| // Implementation depends on client platform capabilities. For example, a web |
| // browser might open a link in a pop-up window with an `OnClose` handler. |
| // |
| // If both `OnOpen` and `OnClose` handlers are set, and the client platform |
| // can't support both values, `OnClose` takes precedence. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| type OpenLink_OnClose int32 |
| |
| const ( |
| // Default value. The card doesn't reload; nothing happens. |
| OpenLink_NOTHING OpenLink_OnClose = 0 |
| // Reloads the card after the child window closes. |
| // |
| // If used in conjunction with |
| // [`OpenAs.OVERLAY`](https://developers.google.com/workspace/add-ons/reference/rpc/google.apps.card.v1#openas), |
| // the child window acts as a modal dialog and the parent card is blocked |
| // until the child window closes. |
| OpenLink_RELOAD OpenLink_OnClose = 1 |
| ) |
| |
| // Enum value maps for OpenLink_OnClose. |
| var ( |
| OpenLink_OnClose_name = map[int32]string{ |
| 0: "NOTHING", |
| 1: "RELOAD", |
| } |
| OpenLink_OnClose_value = map[string]int32{ |
| "NOTHING": 0, |
| "RELOAD": 1, |
| } |
| ) |
| |
| func (x OpenLink_OnClose) Enum() *OpenLink_OnClose { |
| p := new(OpenLink_OnClose) |
| *p = x |
| return p |
| } |
| |
| func (x OpenLink_OnClose) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (OpenLink_OnClose) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[15].Descriptor() |
| } |
| |
| func (OpenLink_OnClose) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[15] |
| } |
| |
| func (x OpenLink_OnClose) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use OpenLink_OnClose.Descriptor instead. |
| func (OpenLink_OnClose) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{20, 1} |
| } |
| |
| // Specifies the loading indicator that the action displays while |
| // making the call to the action. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Action_LoadIndicator int32 |
| |
| const ( |
| // Displays a spinner to indicate that content is loading. |
| Action_SPINNER Action_LoadIndicator = 0 |
| // Nothing is displayed. |
| Action_NONE Action_LoadIndicator = 1 |
| ) |
| |
| // Enum value maps for Action_LoadIndicator. |
| var ( |
| Action_LoadIndicator_name = map[int32]string{ |
| 0: "SPINNER", |
| 1: "NONE", |
| } |
| Action_LoadIndicator_value = map[string]int32{ |
| "SPINNER": 0, |
| "NONE": 1, |
| } |
| ) |
| |
| func (x Action_LoadIndicator) Enum() *Action_LoadIndicator { |
| p := new(Action_LoadIndicator) |
| *p = x |
| return p |
| } |
| |
| func (x Action_LoadIndicator) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (Action_LoadIndicator) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[16].Descriptor() |
| } |
| |
| func (Action_LoadIndicator) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[16] |
| } |
| |
| func (x Action_LoadIndicator) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use Action_LoadIndicator.Descriptor instead. |
| func (Action_LoadIndicator) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{21, 0} |
| } |
| |
| // Optional. Required when opening a |
| // [dialog](https://developers.google.com/workspace/chat/dialogs). |
| // |
| // What to do in response to an interaction with a user, such as a user |
| // clicking a button in a card message. |
| // |
| // If unspecified, the app responds by executing an `action`—like opening a |
| // link or running a function—as normal. |
| // |
| // By specifying an `interaction`, the app can respond in special interactive |
| // ways. For example, by setting `interaction` to `OPEN_DIALOG`, the app can |
| // open a [dialog](https://developers.google.com/workspace/chat/dialogs). |
| // |
| // When specified, a loading indicator isn't shown. If specified for |
| // an add-on, the entire card is stripped and nothing is shown in the client. |
| // |
| // [Google Chat apps](https://developers.google.com/workspace/chat): |
| type Action_Interaction int32 |
| |
| const ( |
| // Default value. The `action` executes as normal. |
| Action_INTERACTION_UNSPECIFIED Action_Interaction = 0 |
| // Opens a [dialog](https://developers.google.com/workspace/chat/dialogs), a |
| // windowed, card-based interface that Chat apps use to interact with users. |
| // |
| // Only supported by Chat apps in response to button-clicks on card |
| // messages. If specified for |
| // an add-on, the entire card is stripped and nothing is shown in the |
| // client. |
| // |
| // [Google Chat apps](https://developers.google.com/workspace/chat): |
| Action_OPEN_DIALOG Action_Interaction = 1 |
| ) |
| |
| // Enum value maps for Action_Interaction. |
| var ( |
| Action_Interaction_name = map[int32]string{ |
| 0: "INTERACTION_UNSPECIFIED", |
| 1: "OPEN_DIALOG", |
| } |
| Action_Interaction_value = map[string]int32{ |
| "INTERACTION_UNSPECIFIED": 0, |
| "OPEN_DIALOG": 1, |
| } |
| ) |
| |
| func (x Action_Interaction) Enum() *Action_Interaction { |
| p := new(Action_Interaction) |
| *p = x |
| return p |
| } |
| |
| func (x Action_Interaction) String() string { |
| return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x)) |
| } |
| |
| func (Action_Interaction) Descriptor() protoreflect.EnumDescriptor { |
| return file_google_apps_card_v1_card_proto_enumTypes[17].Descriptor() |
| } |
| |
| func (Action_Interaction) Type() protoreflect.EnumType { |
| return &file_google_apps_card_v1_card_proto_enumTypes[17] |
| } |
| |
| func (x Action_Interaction) Number() protoreflect.EnumNumber { |
| return protoreflect.EnumNumber(x) |
| } |
| |
| // Deprecated: Use Action_Interaction.Descriptor instead. |
| func (Action_Interaction) EnumDescriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{21, 1} |
| } |
| |
| // A card interface displayed in a Google Chat message or Google Workspace |
| // Add-on. |
| // |
| // Cards support a defined layout, interactive UI elements like buttons, and |
| // rich media like images. Use cards to present detailed information, |
| // gather information from users, and guide users to take a next step. |
| // |
| // [Card builder](https://addons.gsuite.google.com/uikit/builder) |
| // |
| // To learn how |
| // to build cards, see the following documentation: |
| // |
| // - For Google Chat apps, see [Design the components of a card or |
| // dialog](https://developers.google.com/workspace/chat/design-components-card-dialog). |
| // - For Google Workspace Add-ons, see [Card-based |
| // |
| // interfaces](https://developers.google.com/apps-script/add-ons/concepts/cards). |
| // |
| // **Example: Card message for a Google Chat app** |
| // |
| // 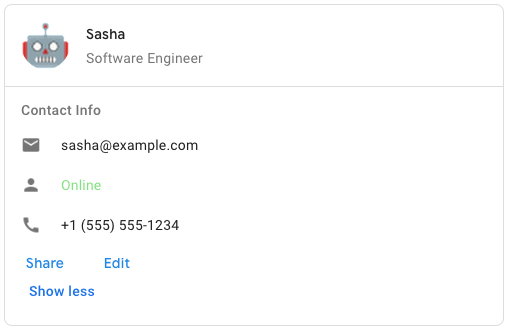 |
| // |
| // To create the sample card message in Google Chat, use the following JSON: |
| // |
| // ``` |
| // |
| // { |
| // "cardsV2": [ |
| // { |
| // "cardId": "unique-card-id", |
| // "card": { |
| // "header": { |
| // "title": "Sasha", |
| // "subtitle": "Software Engineer", |
| // "imageUrl": |
| // "https://developers.google.com/workspace/chat/images/quickstart-app-avatar.png", |
| // "imageType": "CIRCLE", |
| // "imageAltText": "Avatar for Sasha" |
| // }, |
| // "sections": [ |
| // { |
| // "header": "Contact Info", |
| // "collapsible": true, |
| // "uncollapsibleWidgetsCount": 1, |
| // "widgets": [ |
| // { |
| // "decoratedText": { |
| // "startIcon": { |
| // "knownIcon": "EMAIL" |
| // }, |
| // "text": "sasha@example.com" |
| // } |
| // }, |
| // { |
| // "decoratedText": { |
| // "startIcon": { |
| // "knownIcon": "PERSON" |
| // }, |
| // "text": "<font color=\"#80e27e\">Online</font>" |
| // } |
| // }, |
| // { |
| // "decoratedText": { |
| // "startIcon": { |
| // "knownIcon": "PHONE" |
| // }, |
| // "text": "+1 (555) 555-1234" |
| // } |
| // }, |
| // { |
| // "buttonList": { |
| // "buttons": [ |
| // { |
| // "text": "Share", |
| // "onClick": { |
| // "openLink": { |
| // "url": "https://example.com/share" |
| // } |
| // } |
| // }, |
| // { |
| // "text": "Edit", |
| // "onClick": { |
| // "action": { |
| // "function": "goToView", |
| // "parameters": [ |
| // { |
| // "key": "viewType", |
| // "value": "EDIT" |
| // } |
| // ] |
| // } |
| // } |
| // } |
| // ] |
| // } |
| // } |
| // ] |
| // } |
| // ] |
| // } |
| // } |
| // ] |
| // } |
| // |
| // ``` |
| type Card struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The header of the card. A header usually contains a leading image and a |
| // title. Headers always appear at the top of a card. |
| Header *Card_CardHeader `protobuf:"bytes,1,opt,name=header,proto3" json:"header,omitempty"` |
| // Contains a collection of widgets. Each section has its own, optional |
| // header. Sections are visually separated by a line divider. For an example |
| // in Google Chat apps, see [Define a section of a |
| // card](https://developers.google.com/workspace/chat/design-components-card-dialog#define_a_section_of_a_card). |
| Sections []*Card_Section `protobuf:"bytes,2,rep,name=sections,proto3" json:"sections,omitempty"` |
| // The divider style between sections. |
| SectionDividerStyle Card_DividerStyle `protobuf:"varint,9,opt,name=section_divider_style,json=sectionDividerStyle,proto3,enum=google.apps.card.v1.Card_DividerStyle" json:"section_divider_style,omitempty"` |
| // The card's actions. Actions are added to the card's toolbar menu. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| // |
| // For example, the following JSON constructs a card action menu with |
| // `Settings` and `Send Feedback` options: |
| // |
| // ``` |
| // "card_actions": [ |
| // |
| // { |
| // "actionLabel": "Settings", |
| // "onClick": { |
| // "action": { |
| // "functionName": "goToView", |
| // "parameters": [ |
| // { |
| // "key": "viewType", |
| // "value": "SETTING" |
| // } |
| // ], |
| // "loadIndicator": "LoadIndicator.SPINNER" |
| // } |
| // } |
| // }, |
| // { |
| // "actionLabel": "Send Feedback", |
| // "onClick": { |
| // "openLink": { |
| // "url": "https://example.com/feedback" |
| // } |
| // } |
| // } |
| // |
| // ] |
| // ``` |
| CardActions []*Card_CardAction `protobuf:"bytes,3,rep,name=card_actions,json=cardActions,proto3" json:"card_actions,omitempty"` |
| // Name of the card. Used as a card identifier in card navigation. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| Name string `protobuf:"bytes,4,opt,name=name,proto3" json:"name,omitempty"` |
| // The fixed footer shown at the bottom of this card. |
| // |
| // Setting `fixedFooter` without specifying a `primaryButton` or a |
| // `secondaryButton` causes an error. For Chat apps, you can use fixed footers |
| // in |
| // [dialogs](https://developers.google.com/workspace/chat/dialogs), but not |
| // [card |
| // messages](https://developers.google.com/workspace/chat/create-messages#create). |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| FixedFooter *Card_CardFixedFooter `protobuf:"bytes,5,opt,name=fixed_footer,json=fixedFooter,proto3" json:"fixed_footer,omitempty"` |
| // In Google Workspace Add-ons, sets the display properties of the |
| // `peekCardHeader`. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| DisplayStyle Card_DisplayStyle `protobuf:"varint,6,opt,name=display_style,json=displayStyle,proto3,enum=google.apps.card.v1.Card_DisplayStyle" json:"display_style,omitempty"` |
| // When displaying contextual content, the peek card header acts as a |
| // placeholder so that the user can navigate forward between the homepage |
| // cards and the contextual cards. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| PeekCardHeader *Card_CardHeader `protobuf:"bytes,7,opt,name=peek_card_header,json=peekCardHeader,proto3" json:"peek_card_header,omitempty"` |
| } |
| |
| func (x *Card) Reset() { |
| *x = Card{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[0] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Card) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Card) ProtoMessage() {} |
| |
| func (x *Card) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[0] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Card.ProtoReflect.Descriptor instead. |
| func (*Card) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{0} |
| } |
| |
| func (x *Card) GetHeader() *Card_CardHeader { |
| if x != nil { |
| return x.Header |
| } |
| return nil |
| } |
| |
| func (x *Card) GetSections() []*Card_Section { |
| if x != nil { |
| return x.Sections |
| } |
| return nil |
| } |
| |
| func (x *Card) GetSectionDividerStyle() Card_DividerStyle { |
| if x != nil { |
| return x.SectionDividerStyle |
| } |
| return Card_DIVIDER_STYLE_UNSPECIFIED |
| } |
| |
| func (x *Card) GetCardActions() []*Card_CardAction { |
| if x != nil { |
| return x.CardActions |
| } |
| return nil |
| } |
| |
| func (x *Card) GetName() string { |
| if x != nil { |
| return x.Name |
| } |
| return "" |
| } |
| |
| func (x *Card) GetFixedFooter() *Card_CardFixedFooter { |
| if x != nil { |
| return x.FixedFooter |
| } |
| return nil |
| } |
| |
| func (x *Card) GetDisplayStyle() Card_DisplayStyle { |
| if x != nil { |
| return x.DisplayStyle |
| } |
| return Card_DISPLAY_STYLE_UNSPECIFIED |
| } |
| |
| func (x *Card) GetPeekCardHeader() *Card_CardHeader { |
| if x != nil { |
| return x.PeekCardHeader |
| } |
| return nil |
| } |
| |
| // Each card is made up of widgets. |
| // |
| // A widget is a composite object that can represent one of text, images, |
| // buttons, and other object types. |
| type Widget struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // A widget can only have one of the following items. You can use multiple |
| // widget fields to display more items. |
| // |
| // Types that are assignable to Data: |
| // |
| // *Widget_TextParagraph |
| // *Widget_Image |
| // *Widget_DecoratedText |
| // *Widget_ButtonList |
| // *Widget_TextInput |
| // *Widget_SelectionInput |
| // *Widget_DateTimePicker |
| // *Widget_Divider |
| // *Widget_Grid |
| // *Widget_Columns |
| Data isWidget_Data `protobuf_oneof:"data"` |
| // Specifies whether widgets align to the left, right, or center of a column. |
| HorizontalAlignment Widget_HorizontalAlignment `protobuf:"varint,8,opt,name=horizontal_alignment,json=horizontalAlignment,proto3,enum=google.apps.card.v1.Widget_HorizontalAlignment" json:"horizontal_alignment,omitempty"` |
| } |
| |
| func (x *Widget) Reset() { |
| *x = Widget{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[1] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Widget) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Widget) ProtoMessage() {} |
| |
| func (x *Widget) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[1] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Widget.ProtoReflect.Descriptor instead. |
| func (*Widget) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{1} |
| } |
| |
| func (m *Widget) GetData() isWidget_Data { |
| if m != nil { |
| return m.Data |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetTextParagraph() *TextParagraph { |
| if x, ok := x.GetData().(*Widget_TextParagraph); ok { |
| return x.TextParagraph |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetImage() *Image { |
| if x, ok := x.GetData().(*Widget_Image); ok { |
| return x.Image |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetDecoratedText() *DecoratedText { |
| if x, ok := x.GetData().(*Widget_DecoratedText); ok { |
| return x.DecoratedText |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetButtonList() *ButtonList { |
| if x, ok := x.GetData().(*Widget_ButtonList); ok { |
| return x.ButtonList |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetTextInput() *TextInput { |
| if x, ok := x.GetData().(*Widget_TextInput); ok { |
| return x.TextInput |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetSelectionInput() *SelectionInput { |
| if x, ok := x.GetData().(*Widget_SelectionInput); ok { |
| return x.SelectionInput |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetDateTimePicker() *DateTimePicker { |
| if x, ok := x.GetData().(*Widget_DateTimePicker); ok { |
| return x.DateTimePicker |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetDivider() *Divider { |
| if x, ok := x.GetData().(*Widget_Divider); ok { |
| return x.Divider |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetGrid() *Grid { |
| if x, ok := x.GetData().(*Widget_Grid); ok { |
| return x.Grid |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetColumns() *Columns { |
| if x, ok := x.GetData().(*Widget_Columns); ok { |
| return x.Columns |
| } |
| return nil |
| } |
| |
| func (x *Widget) GetHorizontalAlignment() Widget_HorizontalAlignment { |
| if x != nil { |
| return x.HorizontalAlignment |
| } |
| return Widget_HORIZONTAL_ALIGNMENT_UNSPECIFIED |
| } |
| |
| type isWidget_Data interface { |
| isWidget_Data() |
| } |
| |
| type Widget_TextParagraph struct { |
| // Displays a text paragraph. Supports simple HTML formatted text. For more |
| // information about formatting text, see |
| // [Formatting text in Google Chat |
| // apps](https://developers.google.com/workspace/chat/format-messages#card-formatting) |
| // and |
| // [Formatting |
| // text in Google Workspace |
| // Add-ons](https://developers.google.com/apps-script/add-ons/concepts/widgets#text_formatting). |
| // |
| // For example, the following JSON creates a bolded text: |
| // ``` |
| // |
| // "textParagraph": { |
| // "text": " <b>bold text</b>" |
| // } |
| // |
| // ``` |
| TextParagraph *TextParagraph `protobuf:"bytes,1,opt,name=text_paragraph,json=textParagraph,proto3,oneof"` |
| } |
| |
| type Widget_Image struct { |
| // Displays an image. |
| // |
| // For example, the following JSON creates an image with alternative text: |
| // ``` |
| // |
| // "image": { |
| // "imageUrl": |
| // "https://developers.google.com/workspace/chat/images/quickstart-app-avatar.png", |
| // "altText": "Chat app avatar" |
| // } |
| // |
| // ``` |
| Image *Image `protobuf:"bytes,2,opt,name=image,proto3,oneof"` |
| } |
| |
| type Widget_DecoratedText struct { |
| // Displays a decorated text item. |
| // |
| // For example, the following JSON creates a decorated text widget showing |
| // email address: |
| // |
| // ``` |
| // |
| // "decoratedText": { |
| // "icon": { |
| // "knownIcon": "EMAIL" |
| // }, |
| // "topLabel": "Email Address", |
| // "text": "sasha@example.com", |
| // "bottomLabel": "This is a new Email address!", |
| // "switchControl": { |
| // "name": "has_send_welcome_email_to_sasha", |
| // "selected": false, |
| // "controlType": "CHECKBOX" |
| // } |
| // } |
| // |
| // ``` |
| DecoratedText *DecoratedText `protobuf:"bytes,3,opt,name=decorated_text,json=decoratedText,proto3,oneof"` |
| } |
| |
| type Widget_ButtonList struct { |
| // A list of buttons. |
| // |
| // For example, the following JSON creates two buttons. The first |
| // is a blue text button and the second is an image button that opens a |
| // link: |
| // ``` |
| // |
| // "buttonList": { |
| // "buttons": [ |
| // { |
| // "text": "Edit", |
| // "color": { |
| // "red": 0, |
| // "green": 0, |
| // "blue": 1, |
| // "alpha": 1 |
| // }, |
| // "disabled": true, |
| // }, |
| // { |
| // "icon": { |
| // "knownIcon": "INVITE", |
| // "altText": "check calendar" |
| // }, |
| // "onClick": { |
| // "openLink": { |
| // "url": "https://example.com/calendar" |
| // } |
| // } |
| // } |
| // ] |
| // } |
| // |
| // ``` |
| ButtonList *ButtonList `protobuf:"bytes,4,opt,name=button_list,json=buttonList,proto3,oneof"` |
| } |
| |
| type Widget_TextInput struct { |
| // Displays a text box that users can type into. |
| // |
| // For example, the following JSON creates a text input for an email |
| // address: |
| // |
| // ``` |
| // |
| // "textInput": { |
| // "name": "mailing_address", |
| // "label": "Mailing Address" |
| // } |
| // |
| // ``` |
| // |
| // As another example, the following JSON creates a text input for a |
| // programming language with static suggestions: |
| // ``` |
| // |
| // "textInput": { |
| // "name": "preferred_programing_language", |
| // "label": "Preferred Language", |
| // "initialSuggestions": { |
| // "items": [ |
| // { |
| // "text": "C++" |
| // }, |
| // { |
| // "text": "Java" |
| // }, |
| // { |
| // "text": "JavaScript" |
| // }, |
| // { |
| // "text": "Python" |
| // } |
| // ] |
| // } |
| // } |
| // |
| // ``` |
| TextInput *TextInput `protobuf:"bytes,5,opt,name=text_input,json=textInput,proto3,oneof"` |
| } |
| |
| type Widget_SelectionInput struct { |
| // Displays a selection control that lets users select items. Selection |
| // controls can be checkboxes, radio buttons, switches, or dropdown menus. |
| // |
| // For example, the following JSON creates a dropdown menu that lets users |
| // choose a size: |
| // |
| // ``` |
| // |
| // "selectionInput": { |
| // "name": "size", |
| // "label": "Size" |
| // "type": "DROPDOWN", |
| // "items": [ |
| // { |
| // "text": "S", |
| // "value": "small", |
| // "selected": false |
| // }, |
| // { |
| // "text": "M", |
| // "value": "medium", |
| // "selected": true |
| // }, |
| // { |
| // "text": "L", |
| // "value": "large", |
| // "selected": false |
| // }, |
| // { |
| // "text": "XL", |
| // "value": "extra_large", |
| // "selected": false |
| // } |
| // ] |
| // } |
| // |
| // ``` |
| SelectionInput *SelectionInput `protobuf:"bytes,6,opt,name=selection_input,json=selectionInput,proto3,oneof"` |
| } |
| |
| type Widget_DateTimePicker struct { |
| // Displays a widget that lets users input a date, time, or date and time. |
| // |
| // For example, the following JSON creates a date time picker to schedule an |
| // appointment: |
| // |
| // ``` |
| // |
| // "dateTimePicker": { |
| // "name": "appointment_time", |
| // "label": "Book your appointment at:", |
| // "type": "DATE_AND_TIME", |
| // "valueMsEpoch": "796435200000" |
| // } |
| // |
| // ``` |
| DateTimePicker *DateTimePicker `protobuf:"bytes,7,opt,name=date_time_picker,json=dateTimePicker,proto3,oneof"` |
| } |
| |
| type Widget_Divider struct { |
| // Displays a horizontal line divider between widgets. |
| // |
| // For example, the following JSON creates a divider: |
| // ``` |
| // "divider": { |
| // } |
| // ``` |
| Divider *Divider `protobuf:"bytes,9,opt,name=divider,proto3,oneof"` |
| } |
| |
| type Widget_Grid struct { |
| // Displays a grid with a collection of items. |
| // |
| // A grid supports any number of columns and items. The number of rows is |
| // determined by the upper bounds of the number items divided by the number |
| // of columns. A grid with 10 items and 2 columns has 5 rows. A grid with 11 |
| // items and 2 columns has 6 rows. |
| // |
| // [Google Workspace Add-ons and |
| // Chat apps](https://developers.google.com/workspace/extend): |
| // |
| // For example, the following JSON creates a 2 column grid with a single |
| // item: |
| // |
| // ``` |
| // |
| // "grid": { |
| // "title": "A fine collection of items", |
| // "columnCount": 2, |
| // "borderStyle": { |
| // "type": "STROKE", |
| // "cornerRadius": 4 |
| // }, |
| // "items": [ |
| // { |
| // "image": { |
| // "imageUri": "https://www.example.com/image.png", |
| // "cropStyle": { |
| // "type": "SQUARE" |
| // }, |
| // "borderStyle": { |
| // "type": "STROKE" |
| // } |
| // }, |
| // "title": "An item", |
| // "textAlignment": "CENTER" |
| // } |
| // ], |
| // "onClick": { |
| // "openLink": { |
| // "url": "https://www.example.com" |
| // } |
| // } |
| // } |
| // |
| // ``` |
| Grid *Grid `protobuf:"bytes,10,opt,name=grid,proto3,oneof"` |
| } |
| |
| type Widget_Columns struct { |
| // Displays up to 2 columns. |
| // |
| // To include more than 2 columns, or to use rows, use the `Grid` widget. |
| // |
| // For example, the following JSON creates 2 columns that each contain |
| // text paragraphs: |
| // |
| // ``` |
| // |
| // "columns": { |
| // "columnItems": [ |
| // { |
| // "horizontalSizeStyle": "FILL_AVAILABLE_SPACE", |
| // "horizontalAlignment": "CENTER", |
| // "verticalAlignment": "CENTER", |
| // "widgets": [ |
| // { |
| // "textParagraph": { |
| // "text": "First column text paragraph" |
| // } |
| // } |
| // ] |
| // }, |
| // { |
| // "horizontalSizeStyle": "FILL_AVAILABLE_SPACE", |
| // "horizontalAlignment": "CENTER", |
| // "verticalAlignment": "CENTER", |
| // "widgets": [ |
| // { |
| // "textParagraph": { |
| // "text": "Second column text paragraph" |
| // } |
| // } |
| // ] |
| // } |
| // ] |
| // } |
| // |
| // ``` |
| Columns *Columns `protobuf:"bytes,11,opt,name=columns,proto3,oneof"` |
| } |
| |
| func (*Widget_TextParagraph) isWidget_Data() {} |
| |
| func (*Widget_Image) isWidget_Data() {} |
| |
| func (*Widget_DecoratedText) isWidget_Data() {} |
| |
| func (*Widget_ButtonList) isWidget_Data() {} |
| |
| func (*Widget_TextInput) isWidget_Data() {} |
| |
| func (*Widget_SelectionInput) isWidget_Data() {} |
| |
| func (*Widget_DateTimePicker) isWidget_Data() {} |
| |
| func (*Widget_Divider) isWidget_Data() {} |
| |
| func (*Widget_Grid) isWidget_Data() {} |
| |
| func (*Widget_Columns) isWidget_Data() {} |
| |
| // A paragraph of text that supports formatting. For an example in |
| // Google Chat apps, see [Add a paragraph of formatted |
| // text](https://developers.google.com/workspace/chat/add-text-image-card-dialog#add_a_paragraph_of_formatted_text). |
| // For more information |
| // about formatting text, see |
| // [Formatting text in Google Chat |
| // apps](https://developers.google.com/workspace/chat/format-messages#card-formatting) |
| // and |
| // [Formatting |
| // text in Google Workspace |
| // Add-ons](https://developers.google.com/apps-script/add-ons/concepts/widgets#text_formatting). |
| // |
| // [Google Workspace Add-ons and |
| // Chat apps](https://developers.google.com/workspace/extend): |
| type TextParagraph struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The text that's shown in the widget. |
| Text string `protobuf:"bytes,1,opt,name=text,proto3" json:"text,omitempty"` |
| } |
| |
| func (x *TextParagraph) Reset() { |
| *x = TextParagraph{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[2] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *TextParagraph) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*TextParagraph) ProtoMessage() {} |
| |
| func (x *TextParagraph) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[2] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use TextParagraph.ProtoReflect.Descriptor instead. |
| func (*TextParagraph) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{2} |
| } |
| |
| func (x *TextParagraph) GetText() string { |
| if x != nil { |
| return x.Text |
| } |
| return "" |
| } |
| |
| // An image that is specified by a URL and can have an `onClick` action. For an |
| // example, see [Add an |
| // image](https://developers.google.com/workspace/chat/add-text-image-card-dialog#add_an_image). |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Image struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The HTTPS URL that hosts the image. |
| // |
| // For example: |
| // |
| // ``` |
| // https://developers.google.com/workspace/chat/images/quickstart-app-avatar.png |
| // ``` |
| ImageUrl string `protobuf:"bytes,1,opt,name=image_url,json=imageUrl,proto3" json:"image_url,omitempty"` |
| // When a user clicks the image, the click triggers this action. |
| OnClick *OnClick `protobuf:"bytes,2,opt,name=on_click,json=onClick,proto3" json:"on_click,omitempty"` |
| // The alternative text of this image that's used for accessibility. |
| AltText string `protobuf:"bytes,3,opt,name=alt_text,json=altText,proto3" json:"alt_text,omitempty"` |
| } |
| |
| func (x *Image) Reset() { |
| *x = Image{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[3] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Image) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Image) ProtoMessage() {} |
| |
| func (x *Image) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[3] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Image.ProtoReflect.Descriptor instead. |
| func (*Image) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{3} |
| } |
| |
| func (x *Image) GetImageUrl() string { |
| if x != nil { |
| return x.ImageUrl |
| } |
| return "" |
| } |
| |
| func (x *Image) GetOnClick() *OnClick { |
| if x != nil { |
| return x.OnClick |
| } |
| return nil |
| } |
| |
| func (x *Image) GetAltText() string { |
| if x != nil { |
| return x.AltText |
| } |
| return "" |
| } |
| |
| // Displays a divider between widgets as a horizontal line. For an example in |
| // Google Chat apps, see |
| // [Add a horizontal divider between |
| // widgets](https://developers.google.com/workspace/chat/format-structure-card-dialog#add_a_horizontal_divider_between_widgets). |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| // |
| // For example, the following JSON creates a divider: |
| // |
| // ``` |
| // "divider": {} |
| // ``` |
| type Divider struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| } |
| |
| func (x *Divider) Reset() { |
| *x = Divider{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[4] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Divider) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Divider) ProtoMessage() {} |
| |
| func (x *Divider) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[4] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Divider.ProtoReflect.Descriptor instead. |
| func (*Divider) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{4} |
| } |
| |
| // A widget that displays text with optional decorations such as a label above |
| // or below the text, an icon in front of the text, a selection widget, or a |
| // button after the text. For an example in |
| // Google Chat apps, see [Display text with decorative |
| // text](https://developers.google.com/workspace/chat/add-text-image-card-dialog#display_text_with_decorative_elements). |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type DecoratedText struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // Deprecated in favor of `startIcon`. |
| // |
| // Deprecated: Do not use. |
| Icon *Icon `protobuf:"bytes,1,opt,name=icon,proto3" json:"icon,omitempty"` |
| // The icon displayed in front of the text. |
| StartIcon *Icon `protobuf:"bytes,12,opt,name=start_icon,json=startIcon,proto3" json:"start_icon,omitempty"` |
| // The text that appears above `text`. Always truncates. |
| TopLabel string `protobuf:"bytes,3,opt,name=top_label,json=topLabel,proto3" json:"top_label,omitempty"` |
| // Required. The primary text. |
| // |
| // Supports simple formatting. For more information |
| // about formatting text, see |
| // [Formatting text in Google Chat |
| // apps](https://developers.google.com/workspace/chat/format-messages#card-formatting) |
| // and |
| // [Formatting |
| // text in Google Workspace |
| // Add-ons](https://developers.google.com/apps-script/add-ons/concepts/widgets#text_formatting). |
| Text string `protobuf:"bytes,4,opt,name=text,proto3" json:"text,omitempty"` |
| // The wrap text setting. If `true`, the text wraps and displays on |
| // multiple lines. Otherwise, the text is truncated. |
| // |
| // Only applies to `text`, not `topLabel` and `bottomLabel`. |
| WrapText bool `protobuf:"varint,5,opt,name=wrap_text,json=wrapText,proto3" json:"wrap_text,omitempty"` |
| // The text that appears below `text`. Always wraps. |
| BottomLabel string `protobuf:"bytes,6,opt,name=bottom_label,json=bottomLabel,proto3" json:"bottom_label,omitempty"` |
| // This action is triggered when users click `topLabel` or `bottomLabel`. |
| OnClick *OnClick `protobuf:"bytes,7,opt,name=on_click,json=onClick,proto3" json:"on_click,omitempty"` |
| // A button, switch, checkbox, or image that appears to the right-hand side |
| // of text in the `decoratedText` widget. |
| // |
| // Types that are assignable to Control: |
| // |
| // *DecoratedText_Button |
| // *DecoratedText_SwitchControl_ |
| // *DecoratedText_EndIcon |
| Control isDecoratedText_Control `protobuf_oneof:"control"` |
| } |
| |
| func (x *DecoratedText) Reset() { |
| *x = DecoratedText{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[5] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *DecoratedText) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*DecoratedText) ProtoMessage() {} |
| |
| func (x *DecoratedText) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[5] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use DecoratedText.ProtoReflect.Descriptor instead. |
| func (*DecoratedText) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{5} |
| } |
| |
| // Deprecated: Do not use. |
| func (x *DecoratedText) GetIcon() *Icon { |
| if x != nil { |
| return x.Icon |
| } |
| return nil |
| } |
| |
| func (x *DecoratedText) GetStartIcon() *Icon { |
| if x != nil { |
| return x.StartIcon |
| } |
| return nil |
| } |
| |
| func (x *DecoratedText) GetTopLabel() string { |
| if x != nil { |
| return x.TopLabel |
| } |
| return "" |
| } |
| |
| func (x *DecoratedText) GetText() string { |
| if x != nil { |
| return x.Text |
| } |
| return "" |
| } |
| |
| func (x *DecoratedText) GetWrapText() bool { |
| if x != nil { |
| return x.WrapText |
| } |
| return false |
| } |
| |
| func (x *DecoratedText) GetBottomLabel() string { |
| if x != nil { |
| return x.BottomLabel |
| } |
| return "" |
| } |
| |
| func (x *DecoratedText) GetOnClick() *OnClick { |
| if x != nil { |
| return x.OnClick |
| } |
| return nil |
| } |
| |
| func (m *DecoratedText) GetControl() isDecoratedText_Control { |
| if m != nil { |
| return m.Control |
| } |
| return nil |
| } |
| |
| func (x *DecoratedText) GetButton() *Button { |
| if x, ok := x.GetControl().(*DecoratedText_Button); ok { |
| return x.Button |
| } |
| return nil |
| } |
| |
| func (x *DecoratedText) GetSwitchControl() *DecoratedText_SwitchControl { |
| if x, ok := x.GetControl().(*DecoratedText_SwitchControl_); ok { |
| return x.SwitchControl |
| } |
| return nil |
| } |
| |
| func (x *DecoratedText) GetEndIcon() *Icon { |
| if x, ok := x.GetControl().(*DecoratedText_EndIcon); ok { |
| return x.EndIcon |
| } |
| return nil |
| } |
| |
| type isDecoratedText_Control interface { |
| isDecoratedText_Control() |
| } |
| |
| type DecoratedText_Button struct { |
| // A button that a user can click to trigger an action. |
| Button *Button `protobuf:"bytes,8,opt,name=button,proto3,oneof"` |
| } |
| |
| type DecoratedText_SwitchControl_ struct { |
| // A switch widget that a user can click to change its state and trigger an |
| // action. |
| SwitchControl *DecoratedText_SwitchControl `protobuf:"bytes,9,opt,name=switch_control,json=switchControl,proto3,oneof"` |
| } |
| |
| type DecoratedText_EndIcon struct { |
| // An icon displayed after the text. |
| // |
| // Supports |
| // [built-in](https://developers.google.com/workspace/chat/format-messages#builtinicons) |
| // and |
| // [custom](https://developers.google.com/workspace/chat/format-messages#customicons) |
| // icons. |
| EndIcon *Icon `protobuf:"bytes,11,opt,name=end_icon,json=endIcon,proto3,oneof"` |
| } |
| |
| func (*DecoratedText_Button) isDecoratedText_Control() {} |
| |
| func (*DecoratedText_SwitchControl_) isDecoratedText_Control() {} |
| |
| func (*DecoratedText_EndIcon) isDecoratedText_Control() {} |
| |
| // A field in which users can enter text. Supports suggestions and on-change |
| // actions. For an example in Google Chat apps, see [Add a field in which a user |
| // can enter |
| // text](https://developers.google.com/workspace/chat/design-interactive-card-dialog#add_a_field_in_which_a_user_can_enter_text). |
| // |
| // Chat apps receive and can process the value of entered text during form input |
| // events. For details about working with form inputs, see [Receive form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| // |
| // When you need to collect undefined or abstract data from users, |
| // use a text input. To collect defined or enumerated data from users, use the |
| // [SelectionInput][google.apps.card.v1.SelectionInput] widget. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type TextInput struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The name by which the text input is identified in a form input event. |
| // |
| // For details about working with form inputs, see [Receive form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| Name string `protobuf:"bytes,1,opt,name=name,proto3" json:"name,omitempty"` |
| // The text that appears above the text input field in the user interface. |
| // |
| // Specify text that helps the user enter the information your app needs. |
| // For example, if you are asking someone's name, but specifically need their |
| // surname, write `surname` instead of `name`. |
| // |
| // Required if `hintText` is unspecified. Otherwise, optional. |
| Label string `protobuf:"bytes,2,opt,name=label,proto3" json:"label,omitempty"` |
| // Text that appears below the text input field meant to assist users by |
| // prompting them to enter a certain value. This text is always visible. |
| // |
| // Required if `label` is unspecified. Otherwise, optional. |
| HintText string `protobuf:"bytes,3,opt,name=hint_text,json=hintText,proto3" json:"hint_text,omitempty"` |
| // The value entered by a user, returned as part of a form input event. |
| // |
| // For details about working with form inputs, see [Receive form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| Value string `protobuf:"bytes,4,opt,name=value,proto3" json:"value,omitempty"` |
| // How a text input field appears in the user interface. |
| // For example, whether the field is single or multi-line. |
| Type TextInput_Type `protobuf:"varint,5,opt,name=type,proto3,enum=google.apps.card.v1.TextInput_Type" json:"type,omitempty"` |
| // What to do when a change occurs in the text input field. For example, a |
| // user adding to the field or deleting text. |
| // |
| // Examples of actions to take include running a custom function or opening |
| // a [dialog](https://developers.google.com/workspace/chat/dialogs) |
| // in Google Chat. |
| OnChangeAction *Action `protobuf:"bytes,6,opt,name=on_change_action,json=onChangeAction,proto3" json:"on_change_action,omitempty"` |
| // Suggested values that users can enter. These values appear when users click |
| // inside the text input field. As users type, the suggested values |
| // dynamically filter to match what the users have typed. |
| // |
| // For example, a text input field for programming language might suggest |
| // Java, JavaScript, Python, and C++. When users start typing `Jav`, the list |
| // of suggestions filters to show just `Java` and `JavaScript`. |
| // |
| // Suggested values help guide users to enter values that your app can make |
| // sense of. When referring to JavaScript, some users might enter `javascript` |
| // and others `java script`. Suggesting `JavaScript` can standardize how users |
| // interact with your app. |
| // |
| // When specified, `TextInput.type` is always `SINGLE_LINE`, even if it's set |
| // to `MULTIPLE_LINE`. |
| // |
| // [Google Workspace |
| // Add-ons and Chat apps](https://developers.google.com/workspace/extend): |
| InitialSuggestions *Suggestions `protobuf:"bytes,7,opt,name=initial_suggestions,json=initialSuggestions,proto3" json:"initial_suggestions,omitempty"` |
| // Optional. Specify what action to take when the text input field provides |
| // suggestions to users who interact with it. |
| // |
| // If unspecified, the suggestions are set by `initialSuggestions` and |
| // are processed by the client. |
| // |
| // If specified, the app takes the action specified here, such as running |
| // a custom function. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| AutoCompleteAction *Action `protobuf:"bytes,8,opt,name=auto_complete_action,json=autoCompleteAction,proto3" json:"auto_complete_action,omitempty"` |
| // Text that appears in the text input field when the field is empty. |
| // Use this text to prompt users to enter a value. For example, `Enter a |
| // number from 0 to 100`. |
| // |
| // [Google Chat apps](https://developers.google.com/workspace/chat): |
| PlaceholderText string `protobuf:"bytes,12,opt,name=placeholder_text,json=placeholderText,proto3" json:"placeholder_text,omitempty"` |
| } |
| |
| func (x *TextInput) Reset() { |
| *x = TextInput{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[6] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *TextInput) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*TextInput) ProtoMessage() {} |
| |
| func (x *TextInput) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[6] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use TextInput.ProtoReflect.Descriptor instead. |
| func (*TextInput) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{6} |
| } |
| |
| func (x *TextInput) GetName() string { |
| if x != nil { |
| return x.Name |
| } |
| return "" |
| } |
| |
| func (x *TextInput) GetLabel() string { |
| if x != nil { |
| return x.Label |
| } |
| return "" |
| } |
| |
| func (x *TextInput) GetHintText() string { |
| if x != nil { |
| return x.HintText |
| } |
| return "" |
| } |
| |
| func (x *TextInput) GetValue() string { |
| if x != nil { |
| return x.Value |
| } |
| return "" |
| } |
| |
| func (x *TextInput) GetType() TextInput_Type { |
| if x != nil { |
| return x.Type |
| } |
| return TextInput_SINGLE_LINE |
| } |
| |
| func (x *TextInput) GetOnChangeAction() *Action { |
| if x != nil { |
| return x.OnChangeAction |
| } |
| return nil |
| } |
| |
| func (x *TextInput) GetInitialSuggestions() *Suggestions { |
| if x != nil { |
| return x.InitialSuggestions |
| } |
| return nil |
| } |
| |
| func (x *TextInput) GetAutoCompleteAction() *Action { |
| if x != nil { |
| return x.AutoCompleteAction |
| } |
| return nil |
| } |
| |
| func (x *TextInput) GetPlaceholderText() string { |
| if x != nil { |
| return x.PlaceholderText |
| } |
| return "" |
| } |
| |
| // Suggested values that users can enter. These values appear when users click |
| // inside the text input field. As users type, the suggested values |
| // dynamically filter to match what the users have typed. |
| // |
| // For example, a text input field for programming language might suggest |
| // Java, JavaScript, Python, and C++. When users start typing `Jav`, the list |
| // of suggestions filters to show `Java` and `JavaScript`. |
| // |
| // Suggested values help guide users to enter values that your app can make |
| // sense of. When referring to JavaScript, some users might enter `javascript` |
| // and others `java script`. Suggesting `JavaScript` can standardize how users |
| // interact with your app. |
| // |
| // When specified, `TextInput.type` is always `SINGLE_LINE`, even if it's set |
| // to `MULTIPLE_LINE`. |
| // |
| // [Google Workspace |
| // Add-ons and Chat apps](https://developers.google.com/workspace/extend): |
| type Suggestions struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // A list of suggestions used for autocomplete recommendations in text input |
| // fields. |
| Items []*Suggestions_SuggestionItem `protobuf:"bytes,1,rep,name=items,proto3" json:"items,omitempty"` |
| } |
| |
| func (x *Suggestions) Reset() { |
| *x = Suggestions{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[7] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Suggestions) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Suggestions) ProtoMessage() {} |
| |
| func (x *Suggestions) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[7] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Suggestions.ProtoReflect.Descriptor instead. |
| func (*Suggestions) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{7} |
| } |
| |
| func (x *Suggestions) GetItems() []*Suggestions_SuggestionItem { |
| if x != nil { |
| return x.Items |
| } |
| return nil |
| } |
| |
| // A list of buttons layed out horizontally. For an example in |
| // Google Chat apps, see |
| // [Add a |
| // button](https://developers.google.com/workspace/chat/design-interactive-card-dialog#add_a_button). |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type ButtonList struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // An array of buttons. |
| Buttons []*Button `protobuf:"bytes,1,rep,name=buttons,proto3" json:"buttons,omitempty"` |
| } |
| |
| func (x *ButtonList) Reset() { |
| *x = ButtonList{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[8] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *ButtonList) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*ButtonList) ProtoMessage() {} |
| |
| func (x *ButtonList) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[8] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use ButtonList.ProtoReflect.Descriptor instead. |
| func (*ButtonList) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{8} |
| } |
| |
| func (x *ButtonList) GetButtons() []*Button { |
| if x != nil { |
| return x.Buttons |
| } |
| return nil |
| } |
| |
| // A widget that creates one or more UI items that users can select. |
| // For example, a dropdown menu or checkboxes. You can use this widget to |
| // collect data that can be predicted or enumerated. For an example in Google |
| // Chat apps, see [Add selectable UI |
| // elements](/workspace/chat/design-interactive-card-dialog#add_selectable_ui_elements). |
| // |
| // Chat apps can process the value of items that users select or input. For |
| // details about working with form inputs, see [Receive form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| // |
| // To collect undefined or abstract data from users, use |
| // the [TextInput][google.apps.card.v1.TextInput] widget. |
| // |
| // [Google Workspace Add-ons |
| // and Chat apps](https://developers.google.com/workspace/extend): |
| type SelectionInput struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The name that identifies the selection input in a form input event. |
| // |
| // For details about working with form inputs, see [Receive form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| Name string `protobuf:"bytes,1,opt,name=name,proto3" json:"name,omitempty"` |
| // The text that appears above the selection input field in the user |
| // interface. |
| // |
| // Specify text that helps the user enter the information your app needs. |
| // For example, if users are selecting the urgency of a work ticket from a |
| // drop-down menu, the label might be "Urgency" or "Select urgency". |
| Label string `protobuf:"bytes,2,opt,name=label,proto3" json:"label,omitempty"` |
| // The type of items that are displayed to users in a `SelectionInput` widget. |
| // Selection types support different types of interactions. For example, users |
| // can select one or more checkboxes, but they can only select one value from |
| // a dropdown menu. |
| Type SelectionInput_SelectionType `protobuf:"varint,3,opt,name=type,proto3,enum=google.apps.card.v1.SelectionInput_SelectionType" json:"type,omitempty"` |
| // An array of selectable items. For example, an array of radio buttons or |
| // checkboxes. Supports up to 100 items. |
| Items []*SelectionInput_SelectionItem `protobuf:"bytes,4,rep,name=items,proto3" json:"items,omitempty"` |
| // If specified, the form is submitted when the selection changes. If not |
| // specified, you must specify a separate button that submits the form. |
| // |
| // For details about working with form inputs, see [Receive form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| OnChangeAction *Action `protobuf:"bytes,5,opt,name=on_change_action,json=onChangeAction,proto3" json:"on_change_action,omitempty"` |
| // For multiselect menus, the maximum number of items that a user can select. |
| // Minimum value is 1 item. If unspecified, defaults to 3 items. |
| MultiSelectMaxSelectedItems int32 `protobuf:"varint,6,opt,name=multi_select_max_selected_items,json=multiSelectMaxSelectedItems,proto3" json:"multi_select_max_selected_items,omitempty"` |
| // For multiselect menus, the number of text characters that a user inputs |
| // before the app queries autocomplete and displays suggested items |
| // in the menu. |
| // |
| // If unspecified, defaults to 0 characters for static data sources and 3 |
| // characters for external data sources. |
| MultiSelectMinQueryLength int32 `protobuf:"varint,7,opt,name=multi_select_min_query_length,json=multiSelectMinQueryLength,proto3" json:"multi_select_min_query_length,omitempty"` |
| // For a multiselect menu, the data source that populates |
| // selection items. |
| // |
| // [Google Chat apps](https://developers.google.com/workspace/chat): |
| // |
| // Types that are assignable to MultiSelectDataSource: |
| // |
| // *SelectionInput_ExternalDataSource |
| // *SelectionInput_PlatformDataSource_ |
| MultiSelectDataSource isSelectionInput_MultiSelectDataSource `protobuf_oneof:"multi_select_data_source"` |
| } |
| |
| func (x *SelectionInput) Reset() { |
| *x = SelectionInput{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[9] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *SelectionInput) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*SelectionInput) ProtoMessage() {} |
| |
| func (x *SelectionInput) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[9] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use SelectionInput.ProtoReflect.Descriptor instead. |
| func (*SelectionInput) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{9} |
| } |
| |
| func (x *SelectionInput) GetName() string { |
| if x != nil { |
| return x.Name |
| } |
| return "" |
| } |
| |
| func (x *SelectionInput) GetLabel() string { |
| if x != nil { |
| return x.Label |
| } |
| return "" |
| } |
| |
| func (x *SelectionInput) GetType() SelectionInput_SelectionType { |
| if x != nil { |
| return x.Type |
| } |
| return SelectionInput_CHECK_BOX |
| } |
| |
| func (x *SelectionInput) GetItems() []*SelectionInput_SelectionItem { |
| if x != nil { |
| return x.Items |
| } |
| return nil |
| } |
| |
| func (x *SelectionInput) GetOnChangeAction() *Action { |
| if x != nil { |
| return x.OnChangeAction |
| } |
| return nil |
| } |
| |
| func (x *SelectionInput) GetMultiSelectMaxSelectedItems() int32 { |
| if x != nil { |
| return x.MultiSelectMaxSelectedItems |
| } |
| return 0 |
| } |
| |
| func (x *SelectionInput) GetMultiSelectMinQueryLength() int32 { |
| if x != nil { |
| return x.MultiSelectMinQueryLength |
| } |
| return 0 |
| } |
| |
| func (m *SelectionInput) GetMultiSelectDataSource() isSelectionInput_MultiSelectDataSource { |
| if m != nil { |
| return m.MultiSelectDataSource |
| } |
| return nil |
| } |
| |
| func (x *SelectionInput) GetExternalDataSource() *Action { |
| if x, ok := x.GetMultiSelectDataSource().(*SelectionInput_ExternalDataSource); ok { |
| return x.ExternalDataSource |
| } |
| return nil |
| } |
| |
| func (x *SelectionInput) GetPlatformDataSource() *SelectionInput_PlatformDataSource { |
| if x, ok := x.GetMultiSelectDataSource().(*SelectionInput_PlatformDataSource_); ok { |
| return x.PlatformDataSource |
| } |
| return nil |
| } |
| |
| type isSelectionInput_MultiSelectDataSource interface { |
| isSelectionInput_MultiSelectDataSource() |
| } |
| |
| type SelectionInput_ExternalDataSource struct { |
| // An external data source, such as a relational data base. |
| ExternalDataSource *Action `protobuf:"bytes,8,opt,name=external_data_source,json=externalDataSource,proto3,oneof"` |
| } |
| |
| type SelectionInput_PlatformDataSource_ struct { |
| // A data source from Google Workspace. |
| PlatformDataSource *SelectionInput_PlatformDataSource `protobuf:"bytes,9,opt,name=platform_data_source,json=platformDataSource,proto3,oneof"` |
| } |
| |
| func (*SelectionInput_ExternalDataSource) isSelectionInput_MultiSelectDataSource() {} |
| |
| func (*SelectionInput_PlatformDataSource_) isSelectionInput_MultiSelectDataSource() {} |
| |
| // Lets users input a date, a time, or both a date and a time. For an example in |
| // Google Chat apps, see [Let a user pick a date and |
| // time](https://developers.google.com/workspace/chat/design-interactive-card-dialog#let_a_user_pick_a_date_and_time). |
| // |
| // Users can input text or use the picker to select dates and times. If users |
| // input an invalid date or time, the picker shows an error that prompts users |
| // to input the information correctly. |
| // |
| // [Google Workspace |
| // Add-ons and Chat apps](https://developers.google.com/workspace/extend): |
| type DateTimePicker struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The name by which the `DateTimePicker` is identified in a form input event. |
| // |
| // For details about working with form inputs, see [Receive form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| Name string `protobuf:"bytes,1,opt,name=name,proto3" json:"name,omitempty"` |
| // The text that prompts users to input a date, a time, or a date and time. |
| // For example, if users are scheduling an appointment, use a label such as |
| // `Appointment date` or `Appointment date and time`. |
| Label string `protobuf:"bytes,2,opt,name=label,proto3" json:"label,omitempty"` |
| // Whether the widget supports inputting a date, a time, or the date and time. |
| Type DateTimePicker_DateTimePickerType `protobuf:"varint,3,opt,name=type,proto3,enum=google.apps.card.v1.DateTimePicker_DateTimePickerType" json:"type,omitempty"` |
| // The default value displayed in the widget, in milliseconds since [Unix |
| // epoch time](https://en.wikipedia.org/wiki/Unix_time). |
| // |
| // Specify the value based on the type of picker (`DateTimePickerType`): |
| // |
| // - `DATE_AND_TIME`: a calendar date and time in UTC. For example, to |
| // represent January 1, 2023 at 12:00 PM UTC, use `1672574400000`. |
| // - `DATE_ONLY`: a calendar date at 00:00:00 UTC. For example, to represent |
| // January 1, 2023, use `1672531200000`. |
| // - `TIME_ONLY`: a time in UTC. For example, to represent 12:00 PM, use |
| // `43200000` (or `12 * 60 * 60 * 1000`). |
| ValueMsEpoch int64 `protobuf:"varint,4,opt,name=value_ms_epoch,json=valueMsEpoch,proto3" json:"value_ms_epoch,omitempty"` |
| // The number representing the time zone offset from UTC, in minutes. |
| // If set, the `value_ms_epoch` is displayed in the specified time zone. |
| // If unset, the value defaults to the user's time zone setting. |
| TimezoneOffsetDate int32 `protobuf:"varint,5,opt,name=timezone_offset_date,json=timezoneOffsetDate,proto3" json:"timezone_offset_date,omitempty"` |
| // Triggered when the user clicks **Save** or **Clear** from the |
| // `DateTimePicker` interface. |
| OnChangeAction *Action `protobuf:"bytes,6,opt,name=on_change_action,json=onChangeAction,proto3" json:"on_change_action,omitempty"` |
| } |
| |
| func (x *DateTimePicker) Reset() { |
| *x = DateTimePicker{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[10] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *DateTimePicker) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*DateTimePicker) ProtoMessage() {} |
| |
| func (x *DateTimePicker) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[10] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use DateTimePicker.ProtoReflect.Descriptor instead. |
| func (*DateTimePicker) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{10} |
| } |
| |
| func (x *DateTimePicker) GetName() string { |
| if x != nil { |
| return x.Name |
| } |
| return "" |
| } |
| |
| func (x *DateTimePicker) GetLabel() string { |
| if x != nil { |
| return x.Label |
| } |
| return "" |
| } |
| |
| func (x *DateTimePicker) GetType() DateTimePicker_DateTimePickerType { |
| if x != nil { |
| return x.Type |
| } |
| return DateTimePicker_DATE_AND_TIME |
| } |
| |
| func (x *DateTimePicker) GetValueMsEpoch() int64 { |
| if x != nil { |
| return x.ValueMsEpoch |
| } |
| return 0 |
| } |
| |
| func (x *DateTimePicker) GetTimezoneOffsetDate() int32 { |
| if x != nil { |
| return x.TimezoneOffsetDate |
| } |
| return 0 |
| } |
| |
| func (x *DateTimePicker) GetOnChangeAction() *Action { |
| if x != nil { |
| return x.OnChangeAction |
| } |
| return nil |
| } |
| |
| // A text, icon, or text and icon button that users can click. For an example in |
| // Google Chat apps, see |
| // [Add a |
| // button](https://developers.google.com/workspace/chat/design-interactive-card-dialog#add_a_button). |
| // |
| // To make an image a clickable button, specify an |
| // [`Image`][google.apps.card.v1.Image] (not an |
| // [`ImageComponent`][google.apps.card.v1.ImageComponent]) and set an |
| // `onClick` action. |
| // |
| // [Google Workspace |
| // Add-ons and Chat apps](https://developers.google.com/workspace/extend): |
| type Button struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The text displayed inside the button. |
| Text string `protobuf:"bytes,1,opt,name=text,proto3" json:"text,omitempty"` |
| // The icon image. If both `icon` and `text` are set, then the icon appears |
| // before the text. |
| Icon *Icon `protobuf:"bytes,2,opt,name=icon,proto3" json:"icon,omitempty"` |
| // If set, the button is filled with a solid background color and the font |
| // color changes to maintain contrast with the background color. For example, |
| // setting a blue background likely results in white text. |
| // |
| // If unset, the image background is white and the font color is blue. |
| // |
| // For red, green, and blue, the value of each field is a `float` number that |
| // you can express in either of two ways: as a number between 0 and 255 |
| // divided by 255 (153/255), or as a value between 0 and 1 (0.6). 0 represents |
| // the absence of a color and 1 or 255/255 represent the full presence of that |
| // color on the RGB scale. |
| // |
| // Optionally set `alpha`, which sets a level of transparency using this |
| // equation: |
| // |
| // ``` |
| // pixel color = alpha * (this color) + (1.0 - alpha) * (background color) |
| // ``` |
| // |
| // For `alpha`, a value of `1` corresponds with a solid color, and a value of |
| // `0` corresponds with a completely transparent color. |
| // |
| // For example, the following color represents a half transparent red: |
| // |
| // ``` |
| // |
| // "color": { |
| // "red": 1, |
| // "green": 0, |
| // "blue": 0, |
| // "alpha": 0.5 |
| // } |
| // |
| // ``` |
| Color *color.Color `protobuf:"bytes,3,opt,name=color,proto3" json:"color,omitempty"` |
| // Required. The action to perform when a user clicks the button, such as |
| // opening a hyperlink or running a custom function. |
| OnClick *OnClick `protobuf:"bytes,4,opt,name=on_click,json=onClick,proto3" json:"on_click,omitempty"` |
| // If `true`, the button is displayed in an inactive state and doesn't respond |
| // to user actions. |
| Disabled bool `protobuf:"varint,5,opt,name=disabled,proto3" json:"disabled,omitempty"` |
| // The alternative text that's used for accessibility. |
| // |
| // Set descriptive text that lets users know what the button does. For |
| // example, if a button opens a hyperlink, you might write: "Opens a new |
| // browser tab and navigates to the Google Chat developer documentation at |
| // https://developers.google.com/workspace/chat". |
| AltText string `protobuf:"bytes,6,opt,name=alt_text,json=altText,proto3" json:"alt_text,omitempty"` |
| } |
| |
| func (x *Button) Reset() { |
| *x = Button{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[11] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Button) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Button) ProtoMessage() {} |
| |
| func (x *Button) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[11] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Button.ProtoReflect.Descriptor instead. |
| func (*Button) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{11} |
| } |
| |
| func (x *Button) GetText() string { |
| if x != nil { |
| return x.Text |
| } |
| return "" |
| } |
| |
| func (x *Button) GetIcon() *Icon { |
| if x != nil { |
| return x.Icon |
| } |
| return nil |
| } |
| |
| func (x *Button) GetColor() *color.Color { |
| if x != nil { |
| return x.Color |
| } |
| return nil |
| } |
| |
| func (x *Button) GetOnClick() *OnClick { |
| if x != nil { |
| return x.OnClick |
| } |
| return nil |
| } |
| |
| func (x *Button) GetDisabled() bool { |
| if x != nil { |
| return x.Disabled |
| } |
| return false |
| } |
| |
| func (x *Button) GetAltText() string { |
| if x != nil { |
| return x.AltText |
| } |
| return "" |
| } |
| |
| // An icon displayed in a widget on a card. For an example in Google Chat apps, |
| // see [Add an |
| // icon](https://developers.google.com/workspace/chat/add-text-image-card-dialog#add_an_icon). |
| // |
| // Supports |
| // [built-in](https://developers.google.com/workspace/chat/format-messages#builtinicons) |
| // and |
| // [custom](https://developers.google.com/workspace/chat/format-messages#customicons) |
| // icons. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Icon struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The icon displayed in the widget on the card. |
| // |
| // Types that are assignable to Icons: |
| // |
| // *Icon_KnownIcon |
| // *Icon_IconUrl |
| // *Icon_MaterialIcon |
| Icons isIcon_Icons `protobuf_oneof:"icons"` |
| // Optional. A description of the icon used for accessibility. |
| // If unspecified, the default value `Button` is provided. As a best practice, |
| // you should set a helpful description for what the icon displays, and if |
| // applicable, what it does. For example, `A user's account portrait`, or |
| // `Opens a new browser tab and navigates to the Google Chat developer |
| // documentation at https://developers.google.com/workspace/chat`. |
| // |
| // If the icon is set in a [`Button`][google.apps.card.v1.Button], the |
| // `altText` appears as helper text when the user hovers over the button. |
| // However, if the button also sets `text`, the icon's `altText` is ignored. |
| AltText string `protobuf:"bytes,3,opt,name=alt_text,json=altText,proto3" json:"alt_text,omitempty"` |
| // The crop style applied to the image. In some cases, applying a |
| // `CIRCLE` crop causes the image to be drawn larger than a built-in |
| // icon. |
| ImageType Widget_ImageType `protobuf:"varint,4,opt,name=image_type,json=imageType,proto3,enum=google.apps.card.v1.Widget_ImageType" json:"image_type,omitempty"` |
| } |
| |
| func (x *Icon) Reset() { |
| *x = Icon{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[12] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Icon) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Icon) ProtoMessage() {} |
| |
| func (x *Icon) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[12] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Icon.ProtoReflect.Descriptor instead. |
| func (*Icon) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{12} |
| } |
| |
| func (m *Icon) GetIcons() isIcon_Icons { |
| if m != nil { |
| return m.Icons |
| } |
| return nil |
| } |
| |
| func (x *Icon) GetKnownIcon() string { |
| if x, ok := x.GetIcons().(*Icon_KnownIcon); ok { |
| return x.KnownIcon |
| } |
| return "" |
| } |
| |
| func (x *Icon) GetIconUrl() string { |
| if x, ok := x.GetIcons().(*Icon_IconUrl); ok { |
| return x.IconUrl |
| } |
| return "" |
| } |
| |
| func (x *Icon) GetMaterialIcon() *MaterialIcon { |
| if x, ok := x.GetIcons().(*Icon_MaterialIcon); ok { |
| return x.MaterialIcon |
| } |
| return nil |
| } |
| |
| func (x *Icon) GetAltText() string { |
| if x != nil { |
| return x.AltText |
| } |
| return "" |
| } |
| |
| func (x *Icon) GetImageType() Widget_ImageType { |
| if x != nil { |
| return x.ImageType |
| } |
| return Widget_SQUARE |
| } |
| |
| type isIcon_Icons interface { |
| isIcon_Icons() |
| } |
| |
| type Icon_KnownIcon struct { |
| // Display one of the built-in icons provided by Google Workspace. |
| // |
| // For example, to display an airplane icon, specify `AIRPLANE`. |
| // For a bus, specify `BUS`. |
| // |
| // For a full list of supported icons, see [built-in |
| // icons](https://developers.google.com/workspace/chat/format-messages#builtinicons). |
| KnownIcon string `protobuf:"bytes,1,opt,name=known_icon,json=knownIcon,proto3,oneof"` |
| } |
| |
| type Icon_IconUrl struct { |
| // Display a custom icon hosted at an HTTPS URL. |
| // |
| // For example: |
| // |
| // ``` |
| // "iconUrl": |
| // "https://developers.google.com/workspace/chat/images/quickstart-app-avatar.png" |
| // ``` |
| // |
| // Supported file types include `.png` and `.jpg`. |
| IconUrl string `protobuf:"bytes,2,opt,name=icon_url,json=iconUrl,proto3,oneof"` |
| } |
| |
| type Icon_MaterialIcon struct { |
| // Display one of the [Google Material |
| // Icons](https://fonts.google.com/icons). |
| // |
| // For example, to display a [checkbox |
| // icon](https://fonts.google.com/icons?selected=Material%20Symbols%20Outlined%3Acheck_box%3AFILL%400%3Bwght%40400%3BGRAD%400%3Bopsz%4048), |
| // use |
| // ``` |
| // |
| // "material_icon": { |
| // "name": "check_box" |
| // } |
| // |
| // ``` |
| // |
| // [Google Chat apps](https://developers.google.com/workspace/chat): |
| MaterialIcon *MaterialIcon `protobuf:"bytes,5,opt,name=material_icon,json=materialIcon,proto3,oneof"` |
| } |
| |
| func (*Icon_KnownIcon) isIcon_Icons() {} |
| |
| func (*Icon_IconUrl) isIcon_Icons() {} |
| |
| func (*Icon_MaterialIcon) isIcon_Icons() {} |
| |
| // A [Google Material Icon](https://fonts.google.com/icons), which includes over |
| // 2500+ options. |
| // |
| // For example, to display a [checkbox |
| // icon](https://fonts.google.com/icons?selected=Material%20Symbols%20Outlined%3Acheck_box%3AFILL%400%3Bwght%40400%3BGRAD%400%3Bopsz%4048) |
| // with customized weight and grade, write the following: |
| // |
| // ``` |
| // |
| // { |
| // "name": "check_box", |
| // "fill": true, |
| // "weight": 300, |
| // "grade": -25 |
| // } |
| // |
| // ``` |
| // |
| // [Google Chat apps](https://developers.google.com/workspace/chat): |
| type MaterialIcon struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The icon name defined in the [Google Material |
| // Icon](https://fonts.google.com/icons), for example, `check_box`. Any |
| // invalid names are abandoned and replaced with empty string and |
| // results in the icon failing to render. |
| Name string `protobuf:"bytes,1,opt,name=name,proto3" json:"name,omitempty"` |
| // Whether the icon renders as filled. Default value is false. |
| // |
| // To preview different icon settings, go to |
| // [Google Font Icons](https://fonts.google.com/icons) and adjust the |
| // settings under **Customize**. |
| Fill bool `protobuf:"varint,2,opt,name=fill,proto3" json:"fill,omitempty"` |
| // The stroke weight of the icon. Choose from {100, 200, 300, 400, |
| // 500, 600, 700}. If absent, default value is 400. If any other value is |
| // specified, the default value is used. |
| // |
| // To preview different icon settings, go to |
| // [Google Font Icons](https://fonts.google.com/icons) and adjust the |
| // settings under **Customize**. |
| Weight int32 `protobuf:"varint,3,opt,name=weight,proto3" json:"weight,omitempty"` |
| // Weight and grade affect a symbol’s thickness. Adjustments to grade are more |
| // granular than adjustments to weight and have a small impact on the size of |
| // the symbol. Choose from {-25, 0, 200}. If absent, default value is 0. If |
| // any other value is specified, the default value is used. |
| // |
| // To preview different icon settings, go to |
| // [Google Font Icons](https://fonts.google.com/icons) and adjust the |
| // settings under **Customize**. |
| Grade int32 `protobuf:"varint,4,opt,name=grade,proto3" json:"grade,omitempty"` |
| } |
| |
| func (x *MaterialIcon) Reset() { |
| *x = MaterialIcon{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[13] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *MaterialIcon) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*MaterialIcon) ProtoMessage() {} |
| |
| func (x *MaterialIcon) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[13] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use MaterialIcon.ProtoReflect.Descriptor instead. |
| func (*MaterialIcon) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{13} |
| } |
| |
| func (x *MaterialIcon) GetName() string { |
| if x != nil { |
| return x.Name |
| } |
| return "" |
| } |
| |
| func (x *MaterialIcon) GetFill() bool { |
| if x != nil { |
| return x.Fill |
| } |
| return false |
| } |
| |
| func (x *MaterialIcon) GetWeight() int32 { |
| if x != nil { |
| return x.Weight |
| } |
| return 0 |
| } |
| |
| func (x *MaterialIcon) GetGrade() int32 { |
| if x != nil { |
| return x.Grade |
| } |
| return 0 |
| } |
| |
| // Represents the crop style applied to an image. |
| // |
| // [Google Workspace Add-ons and |
| // Chat apps](https://developers.google.com/workspace/extend): |
| // |
| // For example, here's how to apply a 16:9 aspect ratio: |
| // |
| // ``` |
| // |
| // cropStyle { |
| // "type": "RECTANGLE_CUSTOM", |
| // "aspectRatio": 16/9 |
| // } |
| // |
| // ``` |
| type ImageCropStyle struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The crop type. |
| Type ImageCropStyle_ImageCropType `protobuf:"varint,1,opt,name=type,proto3,enum=google.apps.card.v1.ImageCropStyle_ImageCropType" json:"type,omitempty"` |
| // The aspect ratio to use if the crop type is `RECTANGLE_CUSTOM`. |
| // |
| // For example, here's how to apply a 16:9 aspect ratio: |
| // |
| // ``` |
| // |
| // cropStyle { |
| // "type": "RECTANGLE_CUSTOM", |
| // "aspectRatio": 16/9 |
| // } |
| // |
| // ``` |
| AspectRatio float64 `protobuf:"fixed64,2,opt,name=aspect_ratio,json=aspectRatio,proto3" json:"aspect_ratio,omitempty"` |
| } |
| |
| func (x *ImageCropStyle) Reset() { |
| *x = ImageCropStyle{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[14] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *ImageCropStyle) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*ImageCropStyle) ProtoMessage() {} |
| |
| func (x *ImageCropStyle) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[14] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use ImageCropStyle.ProtoReflect.Descriptor instead. |
| func (*ImageCropStyle) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{14} |
| } |
| |
| func (x *ImageCropStyle) GetType() ImageCropStyle_ImageCropType { |
| if x != nil { |
| return x.Type |
| } |
| return ImageCropStyle_IMAGE_CROP_TYPE_UNSPECIFIED |
| } |
| |
| func (x *ImageCropStyle) GetAspectRatio() float64 { |
| if x != nil { |
| return x.AspectRatio |
| } |
| return 0 |
| } |
| |
| // The style options for the border of a card or widget, including the border |
| // type and color. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type BorderStyle struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The border type. |
| Type BorderStyle_BorderType `protobuf:"varint,1,opt,name=type,proto3,enum=google.apps.card.v1.BorderStyle_BorderType" json:"type,omitempty"` |
| // The colors to use when the type is `BORDER_TYPE_STROKE`. |
| StrokeColor *color.Color `protobuf:"bytes,2,opt,name=stroke_color,json=strokeColor,proto3" json:"stroke_color,omitempty"` |
| // The corner radius for the border. |
| CornerRadius int32 `protobuf:"varint,3,opt,name=corner_radius,json=cornerRadius,proto3" json:"corner_radius,omitempty"` |
| } |
| |
| func (x *BorderStyle) Reset() { |
| *x = BorderStyle{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[15] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *BorderStyle) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*BorderStyle) ProtoMessage() {} |
| |
| func (x *BorderStyle) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[15] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use BorderStyle.ProtoReflect.Descriptor instead. |
| func (*BorderStyle) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{15} |
| } |
| |
| func (x *BorderStyle) GetType() BorderStyle_BorderType { |
| if x != nil { |
| return x.Type |
| } |
| return BorderStyle_BORDER_TYPE_UNSPECIFIED |
| } |
| |
| func (x *BorderStyle) GetStrokeColor() *color.Color { |
| if x != nil { |
| return x.StrokeColor |
| } |
| return nil |
| } |
| |
| func (x *BorderStyle) GetCornerRadius() int32 { |
| if x != nil { |
| return x.CornerRadius |
| } |
| return 0 |
| } |
| |
| // Represents an image. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type ImageComponent struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The image URL. |
| ImageUri string `protobuf:"bytes,1,opt,name=image_uri,json=imageUri,proto3" json:"image_uri,omitempty"` |
| // The accessibility label for the image. |
| AltText string `protobuf:"bytes,2,opt,name=alt_text,json=altText,proto3" json:"alt_text,omitempty"` |
| // The crop style to apply to the image. |
| CropStyle *ImageCropStyle `protobuf:"bytes,3,opt,name=crop_style,json=cropStyle,proto3" json:"crop_style,omitempty"` |
| // The border style to apply to the image. |
| BorderStyle *BorderStyle `protobuf:"bytes,4,opt,name=border_style,json=borderStyle,proto3" json:"border_style,omitempty"` |
| } |
| |
| func (x *ImageComponent) Reset() { |
| *x = ImageComponent{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[16] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *ImageComponent) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*ImageComponent) ProtoMessage() {} |
| |
| func (x *ImageComponent) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[16] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use ImageComponent.ProtoReflect.Descriptor instead. |
| func (*ImageComponent) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{16} |
| } |
| |
| func (x *ImageComponent) GetImageUri() string { |
| if x != nil { |
| return x.ImageUri |
| } |
| return "" |
| } |
| |
| func (x *ImageComponent) GetAltText() string { |
| if x != nil { |
| return x.AltText |
| } |
| return "" |
| } |
| |
| func (x *ImageComponent) GetCropStyle() *ImageCropStyle { |
| if x != nil { |
| return x.CropStyle |
| } |
| return nil |
| } |
| |
| func (x *ImageComponent) GetBorderStyle() *BorderStyle { |
| if x != nil { |
| return x.BorderStyle |
| } |
| return nil |
| } |
| |
| // Displays a grid with a collection of items. Items can only include text or |
| // images. For responsive columns, or to include more than text or images, use |
| // [`Columns`][google.apps.card.v1.Columns]. For an example in Google Chat apps, |
| // see [Display a Grid with a collection of |
| // items](https://developers.google.com/workspace/chat/format-structure-card-dialog#display_a_grid_with_a_collection_of_items). |
| // |
| // A grid supports any number of columns and items. The number of rows is |
| // determined by items divided by columns. A grid with |
| // 10 items and 2 columns has 5 rows. A grid with 11 items and 2 columns |
| // has 6 rows. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| // |
| // For example, the following JSON creates a 2 column grid with a single |
| // item: |
| // |
| // ``` |
| // |
| // "grid": { |
| // "title": "A fine collection of items", |
| // "columnCount": 2, |
| // "borderStyle": { |
| // "type": "STROKE", |
| // "cornerRadius": 4 |
| // }, |
| // "items": [ |
| // { |
| // "image": { |
| // "imageUri": "https://www.example.com/image.png", |
| // "cropStyle": { |
| // "type": "SQUARE" |
| // }, |
| // "borderStyle": { |
| // "type": "STROKE" |
| // } |
| // }, |
| // "title": "An item", |
| // "textAlignment": "CENTER" |
| // } |
| // ], |
| // "onClick": { |
| // "openLink": { |
| // "url": "https://www.example.com" |
| // } |
| // } |
| // } |
| // |
| // ``` |
| type Grid struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The text that displays in the grid header. |
| Title string `protobuf:"bytes,1,opt,name=title,proto3" json:"title,omitempty"` |
| // The items to display in the grid. |
| Items []*Grid_GridItem `protobuf:"bytes,2,rep,name=items,proto3" json:"items,omitempty"` |
| // The border style to apply to each grid item. |
| BorderStyle *BorderStyle `protobuf:"bytes,3,opt,name=border_style,json=borderStyle,proto3" json:"border_style,omitempty"` |
| // The number of columns to display in the grid. A default value |
| // is used if this field isn't specified, and that default value is |
| // different depending on where the grid is shown (dialog versus companion). |
| ColumnCount int32 `protobuf:"varint,4,opt,name=column_count,json=columnCount,proto3" json:"column_count,omitempty"` |
| // This callback is reused by each individual grid item, but with the |
| // item's identifier and index in the items list added to the callback's |
| // parameters. |
| OnClick *OnClick `protobuf:"bytes,5,opt,name=on_click,json=onClick,proto3" json:"on_click,omitempty"` |
| } |
| |
| func (x *Grid) Reset() { |
| *x = Grid{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[17] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Grid) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Grid) ProtoMessage() {} |
| |
| func (x *Grid) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[17] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Grid.ProtoReflect.Descriptor instead. |
| func (*Grid) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{17} |
| } |
| |
| func (x *Grid) GetTitle() string { |
| if x != nil { |
| return x.Title |
| } |
| return "" |
| } |
| |
| func (x *Grid) GetItems() []*Grid_GridItem { |
| if x != nil { |
| return x.Items |
| } |
| return nil |
| } |
| |
| func (x *Grid) GetBorderStyle() *BorderStyle { |
| if x != nil { |
| return x.BorderStyle |
| } |
| return nil |
| } |
| |
| func (x *Grid) GetColumnCount() int32 { |
| if x != nil { |
| return x.ColumnCount |
| } |
| return 0 |
| } |
| |
| func (x *Grid) GetOnClick() *OnClick { |
| if x != nil { |
| return x.OnClick |
| } |
| return nil |
| } |
| |
| // The `Columns` widget displays up to 2 columns in a card or dialog. You can |
| // add widgets to each column; the widgets appear in the order that they are |
| // specified. For an example in Google Chat apps, see |
| // [Display cards and dialogs in |
| // columns](https://developers.google.com/workspace/chat/format-structure-card-dialog#display_cards_and_dialogs_in_columns). |
| // |
| // The height of each column is determined by the taller column. For example, if |
| // the first column is taller than the second column, both columns have the |
| // height of the first column. Because each column can contain a different |
| // number of widgets, you can't define rows or align widgets between the |
| // columns. |
| // |
| // Columns are displayed side-by-side. You can customize the width of each |
| // column using the `HorizontalSizeStyle` field. If the user's |
| // screen width is too narrow, the second column wraps below the first: |
| // |
| // - On web, the second column wraps if the screen width is less than or equal |
| // to 480 pixels. |
| // - On iOS devices, the second column wraps if the screen width is |
| // less than or equal to 300 pt. |
| // - On Android devices, the second column wraps if the screen width is |
| // less than or equal to 320 dp. |
| // |
| // To include more than 2 columns, or to use rows, use the |
| // [`Grid`][google.apps.card.v1.Grid] widget. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| // Columns for Google Workspace Add-ons are in |
| // Developer Preview. |
| type Columns struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // An array of columns. You can include up to 2 columns in a card or dialog. |
| ColumnItems []*Columns_Column `protobuf:"bytes,2,rep,name=column_items,json=columnItems,proto3" json:"column_items,omitempty"` |
| } |
| |
| func (x *Columns) Reset() { |
| *x = Columns{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[18] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Columns) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Columns) ProtoMessage() {} |
| |
| func (x *Columns) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[18] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Columns.ProtoReflect.Descriptor instead. |
| func (*Columns) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{18} |
| } |
| |
| func (x *Columns) GetColumnItems() []*Columns_Column { |
| if x != nil { |
| return x.ColumnItems |
| } |
| return nil |
| } |
| |
| // Represents how to respond when users click an interactive element on |
| // a card, such as a button. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type OnClick struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // Types that are assignable to Data: |
| // |
| // *OnClick_Action |
| // *OnClick_OpenLink |
| // *OnClick_OpenDynamicLinkAction |
| // *OnClick_Card |
| Data isOnClick_Data `protobuf_oneof:"data"` |
| } |
| |
| func (x *OnClick) Reset() { |
| *x = OnClick{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[19] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *OnClick) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*OnClick) ProtoMessage() {} |
| |
| func (x *OnClick) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[19] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use OnClick.ProtoReflect.Descriptor instead. |
| func (*OnClick) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{19} |
| } |
| |
| func (m *OnClick) GetData() isOnClick_Data { |
| if m != nil { |
| return m.Data |
| } |
| return nil |
| } |
| |
| func (x *OnClick) GetAction() *Action { |
| if x, ok := x.GetData().(*OnClick_Action); ok { |
| return x.Action |
| } |
| return nil |
| } |
| |
| func (x *OnClick) GetOpenLink() *OpenLink { |
| if x, ok := x.GetData().(*OnClick_OpenLink); ok { |
| return x.OpenLink |
| } |
| return nil |
| } |
| |
| func (x *OnClick) GetOpenDynamicLinkAction() *Action { |
| if x, ok := x.GetData().(*OnClick_OpenDynamicLinkAction); ok { |
| return x.OpenDynamicLinkAction |
| } |
| return nil |
| } |
| |
| func (x *OnClick) GetCard() *Card { |
| if x, ok := x.GetData().(*OnClick_Card); ok { |
| return x.Card |
| } |
| return nil |
| } |
| |
| type isOnClick_Data interface { |
| isOnClick_Data() |
| } |
| |
| type OnClick_Action struct { |
| // If specified, an action is triggered by this `onClick`. |
| Action *Action `protobuf:"bytes,1,opt,name=action,proto3,oneof"` |
| } |
| |
| type OnClick_OpenLink struct { |
| // If specified, this `onClick` triggers an open link action. |
| OpenLink *OpenLink `protobuf:"bytes,2,opt,name=open_link,json=openLink,proto3,oneof"` |
| } |
| |
| type OnClick_OpenDynamicLinkAction struct { |
| // An add-on triggers this action when the action needs to open a |
| // link. This differs from the `open_link` above in that this needs to talk |
| // to server to get the link. Thus some preparation work is required for |
| // web client to do before the open link action response comes back. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| OpenDynamicLinkAction *Action `protobuf:"bytes,3,opt,name=open_dynamic_link_action,json=openDynamicLinkAction,proto3,oneof"` |
| } |
| |
| type OnClick_Card struct { |
| // A new card is pushed to the card stack after clicking if specified. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| Card *Card `protobuf:"bytes,4,opt,name=card,proto3,oneof"` |
| } |
| |
| func (*OnClick_Action) isOnClick_Data() {} |
| |
| func (*OnClick_OpenLink) isOnClick_Data() {} |
| |
| func (*OnClick_OpenDynamicLinkAction) isOnClick_Data() {} |
| |
| func (*OnClick_Card) isOnClick_Data() {} |
| |
| // Represents an `onClick` event that opens a hyperlink. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type OpenLink struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The URL to open. |
| Url string `protobuf:"bytes,1,opt,name=url,proto3" json:"url,omitempty"` |
| // How to open a link. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| OpenAs OpenLink_OpenAs `protobuf:"varint,2,opt,name=open_as,json=openAs,proto3,enum=google.apps.card.v1.OpenLink_OpenAs" json:"open_as,omitempty"` |
| // Whether the client forgets about a link after opening it, or observes it |
| // until the window closes. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| OnClose OpenLink_OnClose `protobuf:"varint,3,opt,name=on_close,json=onClose,proto3,enum=google.apps.card.v1.OpenLink_OnClose" json:"on_close,omitempty"` |
| } |
| |
| func (x *OpenLink) Reset() { |
| *x = OpenLink{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[20] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *OpenLink) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*OpenLink) ProtoMessage() {} |
| |
| func (x *OpenLink) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[20] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use OpenLink.ProtoReflect.Descriptor instead. |
| func (*OpenLink) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{20} |
| } |
| |
| func (x *OpenLink) GetUrl() string { |
| if x != nil { |
| return x.Url |
| } |
| return "" |
| } |
| |
| func (x *OpenLink) GetOpenAs() OpenLink_OpenAs { |
| if x != nil { |
| return x.OpenAs |
| } |
| return OpenLink_FULL_SIZE |
| } |
| |
| func (x *OpenLink) GetOnClose() OpenLink_OnClose { |
| if x != nil { |
| return x.OnClose |
| } |
| return OpenLink_NOTHING |
| } |
| |
| // An action that describes the behavior when the form is submitted. |
| // For example, you can invoke an Apps Script script to handle the form. |
| // If the action is triggered, the form values are sent to the server. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Action struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // A custom function to invoke when the containing element is |
| // clicked or othrwise activated. |
| // |
| // For example usage, see [Read form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| Function string `protobuf:"bytes,1,opt,name=function,proto3" json:"function,omitempty"` |
| // List of action parameters. |
| Parameters []*Action_ActionParameter `protobuf:"bytes,2,rep,name=parameters,proto3" json:"parameters,omitempty"` |
| // Specifies the loading indicator that the action displays while |
| // making the call to the action. |
| LoadIndicator Action_LoadIndicator `protobuf:"varint,3,opt,name=load_indicator,json=loadIndicator,proto3,enum=google.apps.card.v1.Action_LoadIndicator" json:"load_indicator,omitempty"` |
| // Indicates whether form values persist after the action. The default value |
| // is `false`. |
| // |
| // If `true`, form values remain after the action is triggered. To let the |
| // user make changes while the action is being processed, set |
| // [`LoadIndicator`](https://developers.google.com/workspace/add-ons/reference/rpc/google.apps.card.v1#loadindicator) |
| // to `NONE`. For [card |
| // messages](https://developers.google.com/workspace/chat/api/guides/v1/messages/create#create) |
| // in Chat apps, you must also set the action's |
| // [`ResponseType`](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces.messages#responsetype) |
| // to `UPDATE_MESSAGE` and use the same |
| // [`card_id`](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces.messages#CardWithId) |
| // from the card that contained the action. |
| // |
| // If `false`, the form values are cleared when the action is triggered. |
| // To prevent the user from making changes while the action is being |
| // processed, set |
| // [`LoadIndicator`](https://developers.google.com/workspace/add-ons/reference/rpc/google.apps.card.v1#loadindicator) |
| // to `SPINNER`. |
| PersistValues bool `protobuf:"varint,4,opt,name=persist_values,json=persistValues,proto3" json:"persist_values,omitempty"` |
| // Optional. Required when opening a |
| // [dialog](https://developers.google.com/workspace/chat/dialogs). |
| // |
| // What to do in response to an interaction with a user, such as a user |
| // clicking a button in a card message. |
| // |
| // If unspecified, the app responds by executing an `action`—like opening a |
| // link or running a function—as normal. |
| // |
| // By specifying an `interaction`, the app can respond in special interactive |
| // ways. For example, by setting `interaction` to `OPEN_DIALOG`, the app can |
| // open a [dialog](https://developers.google.com/workspace/chat/dialogs). When |
| // specified, a loading indicator isn't shown. If specified for |
| // an add-on, the entire card is stripped and nothing is shown in the client. |
| // |
| // [Google Chat apps](https://developers.google.com/workspace/chat): |
| Interaction Action_Interaction `protobuf:"varint,5,opt,name=interaction,proto3,enum=google.apps.card.v1.Action_Interaction" json:"interaction,omitempty"` |
| } |
| |
| func (x *Action) Reset() { |
| *x = Action{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[21] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Action) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Action) ProtoMessage() {} |
| |
| func (x *Action) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[21] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Action.ProtoReflect.Descriptor instead. |
| func (*Action) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{21} |
| } |
| |
| func (x *Action) GetFunction() string { |
| if x != nil { |
| return x.Function |
| } |
| return "" |
| } |
| |
| func (x *Action) GetParameters() []*Action_ActionParameter { |
| if x != nil { |
| return x.Parameters |
| } |
| return nil |
| } |
| |
| func (x *Action) GetLoadIndicator() Action_LoadIndicator { |
| if x != nil { |
| return x.LoadIndicator |
| } |
| return Action_SPINNER |
| } |
| |
| func (x *Action) GetPersistValues() bool { |
| if x != nil { |
| return x.PersistValues |
| } |
| return false |
| } |
| |
| func (x *Action) GetInteraction() Action_Interaction { |
| if x != nil { |
| return x.Interaction |
| } |
| return Action_INTERACTION_UNSPECIFIED |
| } |
| |
| // Represents a card header. For an example in Google Chat apps, see [Add a |
| // header](https://developers.google.com/workspace/chat/design-components-card-dialog#add_a_header). |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Card_CardHeader struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // Required. The title of the card header. |
| // The header has a fixed height: if both a |
| // title and subtitle are specified, each takes up one line. If only the |
| // title is specified, it takes up both lines. |
| Title string `protobuf:"bytes,1,opt,name=title,proto3" json:"title,omitempty"` |
| // The subtitle of the card header. If specified, appears on its own line |
| // below the `title`. |
| Subtitle string `protobuf:"bytes,2,opt,name=subtitle,proto3" json:"subtitle,omitempty"` |
| // The shape used to crop the image. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| ImageType Widget_ImageType `protobuf:"varint,3,opt,name=image_type,json=imageType,proto3,enum=google.apps.card.v1.Widget_ImageType" json:"image_type,omitempty"` |
| // The HTTPS URL of the image in the card header. |
| ImageUrl string `protobuf:"bytes,4,opt,name=image_url,json=imageUrl,proto3" json:"image_url,omitempty"` |
| // The alternative text of this image that's used for accessibility. |
| ImageAltText string `protobuf:"bytes,5,opt,name=image_alt_text,json=imageAltText,proto3" json:"image_alt_text,omitempty"` |
| } |
| |
| func (x *Card_CardHeader) Reset() { |
| *x = Card_CardHeader{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[22] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Card_CardHeader) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Card_CardHeader) ProtoMessage() {} |
| |
| func (x *Card_CardHeader) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[22] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Card_CardHeader.ProtoReflect.Descriptor instead. |
| func (*Card_CardHeader) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{0, 0} |
| } |
| |
| func (x *Card_CardHeader) GetTitle() string { |
| if x != nil { |
| return x.Title |
| } |
| return "" |
| } |
| |
| func (x *Card_CardHeader) GetSubtitle() string { |
| if x != nil { |
| return x.Subtitle |
| } |
| return "" |
| } |
| |
| func (x *Card_CardHeader) GetImageType() Widget_ImageType { |
| if x != nil { |
| return x.ImageType |
| } |
| return Widget_SQUARE |
| } |
| |
| func (x *Card_CardHeader) GetImageUrl() string { |
| if x != nil { |
| return x.ImageUrl |
| } |
| return "" |
| } |
| |
| func (x *Card_CardHeader) GetImageAltText() string { |
| if x != nil { |
| return x.ImageAltText |
| } |
| return "" |
| } |
| |
| // A section contains a collection of widgets that are rendered |
| // vertically in the order that they're specified. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Card_Section struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // Text that appears at the top of a section. |
| // Supports simple HTML formatted text. For more information |
| // about formatting text, see |
| // [Formatting text in Google Chat |
| // apps](https://developers.google.com/workspace/chat/format-messages#card-formatting) |
| // and |
| // [Formatting |
| // text in Google Workspace |
| // Add-ons](https://developers.google.com/apps-script/add-ons/concepts/widgets#text_formatting). |
| Header string `protobuf:"bytes,1,opt,name=header,proto3" json:"header,omitempty"` |
| // All the widgets in the section. |
| // Must contain at least one widget. |
| Widgets []*Widget `protobuf:"bytes,2,rep,name=widgets,proto3" json:"widgets,omitempty"` |
| // Indicates whether this section is collapsible. |
| // |
| // Collapsible sections hide some or all widgets, but users can expand the |
| // section to reveal the hidden widgets by clicking **Show more**. Users |
| // can hide the widgets again by clicking **Show less**. |
| // |
| // To determine which widgets are hidden, specify |
| // `uncollapsibleWidgetsCount`. |
| Collapsible bool `protobuf:"varint,5,opt,name=collapsible,proto3" json:"collapsible,omitempty"` |
| // The number of uncollapsible widgets which remain visible even when a |
| // section is collapsed. |
| // |
| // For example, when a section |
| // contains five widgets and the `uncollapsibleWidgetsCount` is set to `2`, |
| // the first two widgets are always shown and the last three are collapsed |
| // by default. The `uncollapsibleWidgetsCount` is taken into account only |
| // when `collapsible` is `true`. |
| UncollapsibleWidgetsCount int32 `protobuf:"varint,6,opt,name=uncollapsible_widgets_count,json=uncollapsibleWidgetsCount,proto3" json:"uncollapsible_widgets_count,omitempty"` |
| } |
| |
| func (x *Card_Section) Reset() { |
| *x = Card_Section{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[23] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Card_Section) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Card_Section) ProtoMessage() {} |
| |
| func (x *Card_Section) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[23] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Card_Section.ProtoReflect.Descriptor instead. |
| func (*Card_Section) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{0, 1} |
| } |
| |
| func (x *Card_Section) GetHeader() string { |
| if x != nil { |
| return x.Header |
| } |
| return "" |
| } |
| |
| func (x *Card_Section) GetWidgets() []*Widget { |
| if x != nil { |
| return x.Widgets |
| } |
| return nil |
| } |
| |
| func (x *Card_Section) GetCollapsible() bool { |
| if x != nil { |
| return x.Collapsible |
| } |
| return false |
| } |
| |
| func (x *Card_Section) GetUncollapsibleWidgetsCount() int32 { |
| if x != nil { |
| return x.UncollapsibleWidgetsCount |
| } |
| return 0 |
| } |
| |
| // A card action is the action associated with the card. For example, |
| // an invoice card might include actions such as delete invoice, email |
| // invoice, or open the invoice in a browser. |
| // |
| // [Google Workspace |
| // Add-ons](https://developers.google.com/workspace/add-ons): |
| type Card_CardAction struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The label that displays as the action menu item. |
| ActionLabel string `protobuf:"bytes,1,opt,name=action_label,json=actionLabel,proto3" json:"action_label,omitempty"` |
| // The `onClick` action for this action item. |
| OnClick *OnClick `protobuf:"bytes,2,opt,name=on_click,json=onClick,proto3" json:"on_click,omitempty"` |
| } |
| |
| func (x *Card_CardAction) Reset() { |
| *x = Card_CardAction{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[24] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Card_CardAction) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Card_CardAction) ProtoMessage() {} |
| |
| func (x *Card_CardAction) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[24] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Card_CardAction.ProtoReflect.Descriptor instead. |
| func (*Card_CardAction) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{0, 2} |
| } |
| |
| func (x *Card_CardAction) GetActionLabel() string { |
| if x != nil { |
| return x.ActionLabel |
| } |
| return "" |
| } |
| |
| func (x *Card_CardAction) GetOnClick() *OnClick { |
| if x != nil { |
| return x.OnClick |
| } |
| return nil |
| } |
| |
| // A persistent (sticky) footer that that appears at the bottom of the card. |
| // |
| // Setting `fixedFooter` without specifying a `primaryButton` or a |
| // `secondaryButton` causes an error. |
| // |
| // For Chat apps, you can use fixed footers in |
| // [dialogs](https://developers.google.com/workspace/chat/dialogs), but not |
| // [card |
| // messages](https://developers.google.com/workspace/chat/create-messages#create). |
| // For an example in Google Chat apps, see [Add a persistent |
| // footer](https://developers.google.com/workspace/chat/design-components-card-dialog#add_a_persistent_footer). |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Card_CardFixedFooter struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The primary button of the fixed footer. The button must be a text button |
| // with text and color set. |
| PrimaryButton *Button `protobuf:"bytes,1,opt,name=primary_button,json=primaryButton,proto3" json:"primary_button,omitempty"` |
| // The secondary button of the fixed footer. The button must be a text |
| // button with text and color set. |
| // If `secondaryButton` is set, you must also set `primaryButton`. |
| SecondaryButton *Button `protobuf:"bytes,2,opt,name=secondary_button,json=secondaryButton,proto3" json:"secondary_button,omitempty"` |
| } |
| |
| func (x *Card_CardFixedFooter) Reset() { |
| *x = Card_CardFixedFooter{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[25] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Card_CardFixedFooter) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Card_CardFixedFooter) ProtoMessage() {} |
| |
| func (x *Card_CardFixedFooter) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[25] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Card_CardFixedFooter.ProtoReflect.Descriptor instead. |
| func (*Card_CardFixedFooter) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{0, 3} |
| } |
| |
| func (x *Card_CardFixedFooter) GetPrimaryButton() *Button { |
| if x != nil { |
| return x.PrimaryButton |
| } |
| return nil |
| } |
| |
| func (x *Card_CardFixedFooter) GetSecondaryButton() *Button { |
| if x != nil { |
| return x.SecondaryButton |
| } |
| return nil |
| } |
| |
| // Either a toggle-style switch or a checkbox inside a `decoratedText` widget. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| // |
| // Only supported in the `decoratedText` widget. |
| type DecoratedText_SwitchControl struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The name by which the switch widget is identified in a form input event. |
| // |
| // For details about working with form inputs, see [Receive form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| Name string `protobuf:"bytes,1,opt,name=name,proto3" json:"name,omitempty"` |
| // The value entered by a user, returned as part of a form input event. |
| // |
| // For details about working with form inputs, see [Receive form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| Value string `protobuf:"bytes,2,opt,name=value,proto3" json:"value,omitempty"` |
| // When `true`, the switch is selected. |
| Selected bool `protobuf:"varint,3,opt,name=selected,proto3" json:"selected,omitempty"` |
| // The action to perform when the switch state is changed, such as what |
| // |
| // function to run. |
| OnChangeAction *Action `protobuf:"bytes,4,opt,name=on_change_action,json=onChangeAction,proto3" json:"on_change_action,omitempty"` |
| // How the switch appears in the user interface. |
| // |
| // [Google Workspace Add-ons |
| // and Chat apps](https://developers.google.com/workspace/extend): |
| ControlType DecoratedText_SwitchControl_ControlType `protobuf:"varint,5,opt,name=control_type,json=controlType,proto3,enum=google.apps.card.v1.DecoratedText_SwitchControl_ControlType" json:"control_type,omitempty"` |
| } |
| |
| func (x *DecoratedText_SwitchControl) Reset() { |
| *x = DecoratedText_SwitchControl{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[26] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *DecoratedText_SwitchControl) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*DecoratedText_SwitchControl) ProtoMessage() {} |
| |
| func (x *DecoratedText_SwitchControl) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[26] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use DecoratedText_SwitchControl.ProtoReflect.Descriptor instead. |
| func (*DecoratedText_SwitchControl) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{5, 0} |
| } |
| |
| func (x *DecoratedText_SwitchControl) GetName() string { |
| if x != nil { |
| return x.Name |
| } |
| return "" |
| } |
| |
| func (x *DecoratedText_SwitchControl) GetValue() string { |
| if x != nil { |
| return x.Value |
| } |
| return "" |
| } |
| |
| func (x *DecoratedText_SwitchControl) GetSelected() bool { |
| if x != nil { |
| return x.Selected |
| } |
| return false |
| } |
| |
| func (x *DecoratedText_SwitchControl) GetOnChangeAction() *Action { |
| if x != nil { |
| return x.OnChangeAction |
| } |
| return nil |
| } |
| |
| func (x *DecoratedText_SwitchControl) GetControlType() DecoratedText_SwitchControl_ControlType { |
| if x != nil { |
| return x.ControlType |
| } |
| return DecoratedText_SwitchControl_SWITCH |
| } |
| |
| // One suggested value that users can enter in a text input field. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Suggestions_SuggestionItem struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // Types that are assignable to Content: |
| // |
| // *Suggestions_SuggestionItem_Text |
| Content isSuggestions_SuggestionItem_Content `protobuf_oneof:"content"` |
| } |
| |
| func (x *Suggestions_SuggestionItem) Reset() { |
| *x = Suggestions_SuggestionItem{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[27] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Suggestions_SuggestionItem) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Suggestions_SuggestionItem) ProtoMessage() {} |
| |
| func (x *Suggestions_SuggestionItem) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[27] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Suggestions_SuggestionItem.ProtoReflect.Descriptor instead. |
| func (*Suggestions_SuggestionItem) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{7, 0} |
| } |
| |
| func (m *Suggestions_SuggestionItem) GetContent() isSuggestions_SuggestionItem_Content { |
| if m != nil { |
| return m.Content |
| } |
| return nil |
| } |
| |
| func (x *Suggestions_SuggestionItem) GetText() string { |
| if x, ok := x.GetContent().(*Suggestions_SuggestionItem_Text); ok { |
| return x.Text |
| } |
| return "" |
| } |
| |
| type isSuggestions_SuggestionItem_Content interface { |
| isSuggestions_SuggestionItem_Content() |
| } |
| |
| type Suggestions_SuggestionItem_Text struct { |
| // The value of a suggested input to a text input field. This is |
| // equivalent to what users enter themselves. |
| Text string `protobuf:"bytes,1,opt,name=text,proto3,oneof"` |
| } |
| |
| func (*Suggestions_SuggestionItem_Text) isSuggestions_SuggestionItem_Content() {} |
| |
| // An item that users can select in a selection input, such as a checkbox |
| // or switch. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type SelectionInput_SelectionItem struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The text that identifies or describes the item to users. |
| Text string `protobuf:"bytes,1,opt,name=text,proto3" json:"text,omitempty"` |
| // The value associated with this item. The client should use this as a form |
| // input value. |
| // |
| // For details about working with form inputs, see [Receive form |
| // data](https://developers.google.com/workspace/chat/read-form-data). |
| Value string `protobuf:"bytes,2,opt,name=value,proto3" json:"value,omitempty"` |
| // Whether the item is selected by default. If the selection input only |
| // accepts one value (such as for radio buttons or a dropdown menu), only |
| // set this field for one item. |
| Selected bool `protobuf:"varint,3,opt,name=selected,proto3" json:"selected,omitempty"` |
| // For multiselect menus, the URL for the icon displayed next to |
| // the item's `text` field. Supports PNG and JPEG files. Must be an `HTTPS` |
| // URL. For example, |
| // `https://developers.google.com/workspace/chat/images/quickstart-app-avatar.png`. |
| StartIconUri string `protobuf:"bytes,4,opt,name=start_icon_uri,json=startIconUri,proto3" json:"start_icon_uri,omitempty"` |
| // For multiselect menus, a text description or label that's |
| // displayed below the item's `text` field. |
| BottomText string `protobuf:"bytes,5,opt,name=bottom_text,json=bottomText,proto3" json:"bottom_text,omitempty"` |
| } |
| |
| func (x *SelectionInput_SelectionItem) Reset() { |
| *x = SelectionInput_SelectionItem{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[28] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *SelectionInput_SelectionItem) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*SelectionInput_SelectionItem) ProtoMessage() {} |
| |
| func (x *SelectionInput_SelectionItem) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[28] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use SelectionInput_SelectionItem.ProtoReflect.Descriptor instead. |
| func (*SelectionInput_SelectionItem) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{9, 0} |
| } |
| |
| func (x *SelectionInput_SelectionItem) GetText() string { |
| if x != nil { |
| return x.Text |
| } |
| return "" |
| } |
| |
| func (x *SelectionInput_SelectionItem) GetValue() string { |
| if x != nil { |
| return x.Value |
| } |
| return "" |
| } |
| |
| func (x *SelectionInput_SelectionItem) GetSelected() bool { |
| if x != nil { |
| return x.Selected |
| } |
| return false |
| } |
| |
| func (x *SelectionInput_SelectionItem) GetStartIconUri() string { |
| if x != nil { |
| return x.StartIconUri |
| } |
| return "" |
| } |
| |
| func (x *SelectionInput_SelectionItem) GetBottomText() string { |
| if x != nil { |
| return x.BottomText |
| } |
| return "" |
| } |
| |
| // For a |
| // [`SelectionInput`][google.apps.card.v1.SelectionInput] widget that uses a |
| // multiselect menu, a data source from Google Workspace. Used to populate |
| // items in a multiselect menu. |
| // |
| // [Google Chat apps](https://developers.google.com/workspace/chat): |
| type SelectionInput_PlatformDataSource struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The data source. |
| // |
| // Types that are assignable to DataSource: |
| // |
| // *SelectionInput_PlatformDataSource_CommonDataSource_ |
| DataSource isSelectionInput_PlatformDataSource_DataSource `protobuf_oneof:"data_source"` |
| } |
| |
| func (x *SelectionInput_PlatformDataSource) Reset() { |
| *x = SelectionInput_PlatformDataSource{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[29] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *SelectionInput_PlatformDataSource) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*SelectionInput_PlatformDataSource) ProtoMessage() {} |
| |
| func (x *SelectionInput_PlatformDataSource) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[29] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use SelectionInput_PlatformDataSource.ProtoReflect.Descriptor instead. |
| func (*SelectionInput_PlatformDataSource) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{9, 1} |
| } |
| |
| func (m *SelectionInput_PlatformDataSource) GetDataSource() isSelectionInput_PlatformDataSource_DataSource { |
| if m != nil { |
| return m.DataSource |
| } |
| return nil |
| } |
| |
| func (x *SelectionInput_PlatformDataSource) GetCommonDataSource() SelectionInput_PlatformDataSource_CommonDataSource { |
| if x, ok := x.GetDataSource().(*SelectionInput_PlatformDataSource_CommonDataSource_); ok { |
| return x.CommonDataSource |
| } |
| return SelectionInput_PlatformDataSource_UNKNOWN |
| } |
| |
| type isSelectionInput_PlatformDataSource_DataSource interface { |
| isSelectionInput_PlatformDataSource_DataSource() |
| } |
| |
| type SelectionInput_PlatformDataSource_CommonDataSource_ struct { |
| // A data source shared by all Google Workspace applications, such as |
| // users in a Google Workspace organization. |
| CommonDataSource SelectionInput_PlatformDataSource_CommonDataSource `protobuf:"varint,1,opt,name=common_data_source,json=commonDataSource,proto3,enum=google.apps.card.v1.SelectionInput_PlatformDataSource_CommonDataSource,oneof"` |
| } |
| |
| func (*SelectionInput_PlatformDataSource_CommonDataSource_) isSelectionInput_PlatformDataSource_DataSource() { |
| } |
| |
| // Represents an item in a grid layout. Items can contain text, an image, or |
| // both text and an image. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Grid_GridItem struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // A user-specified identifier for this grid item. This identifier is |
| // returned in the parent grid's `onClick` callback parameters. |
| Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"` |
| // The image that displays in the grid item. |
| Image *ImageComponent `protobuf:"bytes,2,opt,name=image,proto3" json:"image,omitempty"` |
| // The grid item's title. |
| Title string `protobuf:"bytes,3,opt,name=title,proto3" json:"title,omitempty"` |
| // The grid item's subtitle. |
| Subtitle string `protobuf:"bytes,4,opt,name=subtitle,proto3" json:"subtitle,omitempty"` |
| // The layout to use for the grid item. |
| Layout Grid_GridItem_GridItemLayout `protobuf:"varint,9,opt,name=layout,proto3,enum=google.apps.card.v1.Grid_GridItem_GridItemLayout" json:"layout,omitempty"` |
| } |
| |
| func (x *Grid_GridItem) Reset() { |
| *x = Grid_GridItem{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[30] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Grid_GridItem) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Grid_GridItem) ProtoMessage() {} |
| |
| func (x *Grid_GridItem) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[30] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Grid_GridItem.ProtoReflect.Descriptor instead. |
| func (*Grid_GridItem) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{17, 0} |
| } |
| |
| func (x *Grid_GridItem) GetId() string { |
| if x != nil { |
| return x.Id |
| } |
| return "" |
| } |
| |
| func (x *Grid_GridItem) GetImage() *ImageComponent { |
| if x != nil { |
| return x.Image |
| } |
| return nil |
| } |
| |
| func (x *Grid_GridItem) GetTitle() string { |
| if x != nil { |
| return x.Title |
| } |
| return "" |
| } |
| |
| func (x *Grid_GridItem) GetSubtitle() string { |
| if x != nil { |
| return x.Subtitle |
| } |
| return "" |
| } |
| |
| func (x *Grid_GridItem) GetLayout() Grid_GridItem_GridItemLayout { |
| if x != nil { |
| return x.Layout |
| } |
| return Grid_GridItem_GRID_ITEM_LAYOUT_UNSPECIFIED |
| } |
| |
| // A column. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| // Columns for Google Workspace Add-ons are in |
| // Developer Preview. |
| type Columns_Column struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // Specifies how a column fills the width of the card. |
| HorizontalSizeStyle Columns_Column_HorizontalSizeStyle `protobuf:"varint,1,opt,name=horizontal_size_style,json=horizontalSizeStyle,proto3,enum=google.apps.card.v1.Columns_Column_HorizontalSizeStyle" json:"horizontal_size_style,omitempty"` |
| // Specifies whether widgets align to the left, right, or center of a |
| // column. |
| HorizontalAlignment Widget_HorizontalAlignment `protobuf:"varint,2,opt,name=horizontal_alignment,json=horizontalAlignment,proto3,enum=google.apps.card.v1.Widget_HorizontalAlignment" json:"horizontal_alignment,omitempty"` |
| // Specifies whether widgets align to the top, bottom, or center of a |
| // column. |
| VerticalAlignment Columns_Column_VerticalAlignment `protobuf:"varint,3,opt,name=vertical_alignment,json=verticalAlignment,proto3,enum=google.apps.card.v1.Columns_Column_VerticalAlignment" json:"vertical_alignment,omitempty"` |
| // An array of widgets included in a column. Widgets appear in the order |
| // that they are specified. |
| Widgets []*Columns_Column_Widgets `protobuf:"bytes,4,rep,name=widgets,proto3" json:"widgets,omitempty"` |
| } |
| |
| func (x *Columns_Column) Reset() { |
| *x = Columns_Column{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[31] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Columns_Column) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Columns_Column) ProtoMessage() {} |
| |
| func (x *Columns_Column) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[31] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Columns_Column.ProtoReflect.Descriptor instead. |
| func (*Columns_Column) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{18, 0} |
| } |
| |
| func (x *Columns_Column) GetHorizontalSizeStyle() Columns_Column_HorizontalSizeStyle { |
| if x != nil { |
| return x.HorizontalSizeStyle |
| } |
| return Columns_Column_HORIZONTAL_SIZE_STYLE_UNSPECIFIED |
| } |
| |
| func (x *Columns_Column) GetHorizontalAlignment() Widget_HorizontalAlignment { |
| if x != nil { |
| return x.HorizontalAlignment |
| } |
| return Widget_HORIZONTAL_ALIGNMENT_UNSPECIFIED |
| } |
| |
| func (x *Columns_Column) GetVerticalAlignment() Columns_Column_VerticalAlignment { |
| if x != nil { |
| return x.VerticalAlignment |
| } |
| return Columns_Column_VERTICAL_ALIGNMENT_UNSPECIFIED |
| } |
| |
| func (x *Columns_Column) GetWidgets() []*Columns_Column_Widgets { |
| if x != nil { |
| return x.Widgets |
| } |
| return nil |
| } |
| |
| // The supported widgets that you can include in a column. |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| // Columns for Google Workspace Add-ons are in |
| // Developer Preview. |
| type Columns_Column_Widgets struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // Types that are assignable to Data: |
| // |
| // *Columns_Column_Widgets_TextParagraph |
| // *Columns_Column_Widgets_Image |
| // *Columns_Column_Widgets_DecoratedText |
| // *Columns_Column_Widgets_ButtonList |
| // *Columns_Column_Widgets_TextInput |
| // *Columns_Column_Widgets_SelectionInput |
| // *Columns_Column_Widgets_DateTimePicker |
| Data isColumns_Column_Widgets_Data `protobuf_oneof:"data"` |
| } |
| |
| func (x *Columns_Column_Widgets) Reset() { |
| *x = Columns_Column_Widgets{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[32] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Columns_Column_Widgets) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Columns_Column_Widgets) ProtoMessage() {} |
| |
| func (x *Columns_Column_Widgets) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[32] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Columns_Column_Widgets.ProtoReflect.Descriptor instead. |
| func (*Columns_Column_Widgets) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{18, 0, 0} |
| } |
| |
| func (m *Columns_Column_Widgets) GetData() isColumns_Column_Widgets_Data { |
| if m != nil { |
| return m.Data |
| } |
| return nil |
| } |
| |
| func (x *Columns_Column_Widgets) GetTextParagraph() *TextParagraph { |
| if x, ok := x.GetData().(*Columns_Column_Widgets_TextParagraph); ok { |
| return x.TextParagraph |
| } |
| return nil |
| } |
| |
| func (x *Columns_Column_Widgets) GetImage() *Image { |
| if x, ok := x.GetData().(*Columns_Column_Widgets_Image); ok { |
| return x.Image |
| } |
| return nil |
| } |
| |
| func (x *Columns_Column_Widgets) GetDecoratedText() *DecoratedText { |
| if x, ok := x.GetData().(*Columns_Column_Widgets_DecoratedText); ok { |
| return x.DecoratedText |
| } |
| return nil |
| } |
| |
| func (x *Columns_Column_Widgets) GetButtonList() *ButtonList { |
| if x, ok := x.GetData().(*Columns_Column_Widgets_ButtonList); ok { |
| return x.ButtonList |
| } |
| return nil |
| } |
| |
| func (x *Columns_Column_Widgets) GetTextInput() *TextInput { |
| if x, ok := x.GetData().(*Columns_Column_Widgets_TextInput); ok { |
| return x.TextInput |
| } |
| return nil |
| } |
| |
| func (x *Columns_Column_Widgets) GetSelectionInput() *SelectionInput { |
| if x, ok := x.GetData().(*Columns_Column_Widgets_SelectionInput); ok { |
| return x.SelectionInput |
| } |
| return nil |
| } |
| |
| func (x *Columns_Column_Widgets) GetDateTimePicker() *DateTimePicker { |
| if x, ok := x.GetData().(*Columns_Column_Widgets_DateTimePicker); ok { |
| return x.DateTimePicker |
| } |
| return nil |
| } |
| |
| type isColumns_Column_Widgets_Data interface { |
| isColumns_Column_Widgets_Data() |
| } |
| |
| type Columns_Column_Widgets_TextParagraph struct { |
| // [TextParagraph][google.apps.card.v1.TextParagraph] widget. |
| TextParagraph *TextParagraph `protobuf:"bytes,1,opt,name=text_paragraph,json=textParagraph,proto3,oneof"` |
| } |
| |
| type Columns_Column_Widgets_Image struct { |
| // [Image][google.apps.card.v1.Image] widget. |
| Image *Image `protobuf:"bytes,2,opt,name=image,proto3,oneof"` |
| } |
| |
| type Columns_Column_Widgets_DecoratedText struct { |
| // [DecoratedText][google.apps.card.v1.DecoratedText] widget. |
| DecoratedText *DecoratedText `protobuf:"bytes,3,opt,name=decorated_text,json=decoratedText,proto3,oneof"` |
| } |
| |
| type Columns_Column_Widgets_ButtonList struct { |
| // [ButtonList][google.apps.card.v1.ButtonList] widget. |
| ButtonList *ButtonList `protobuf:"bytes,4,opt,name=button_list,json=buttonList,proto3,oneof"` |
| } |
| |
| type Columns_Column_Widgets_TextInput struct { |
| // [TextInput][google.apps.card.v1.TextInput] widget. |
| TextInput *TextInput `protobuf:"bytes,5,opt,name=text_input,json=textInput,proto3,oneof"` |
| } |
| |
| type Columns_Column_Widgets_SelectionInput struct { |
| // [SelectionInput][google.apps.card.v1.SelectionInput] widget. |
| SelectionInput *SelectionInput `protobuf:"bytes,6,opt,name=selection_input,json=selectionInput,proto3,oneof"` |
| } |
| |
| type Columns_Column_Widgets_DateTimePicker struct { |
| // [DateTimePicker][google.apps.card.v1.DateTimePicker] widget. |
| DateTimePicker *DateTimePicker `protobuf:"bytes,7,opt,name=date_time_picker,json=dateTimePicker,proto3,oneof"` |
| } |
| |
| func (*Columns_Column_Widgets_TextParagraph) isColumns_Column_Widgets_Data() {} |
| |
| func (*Columns_Column_Widgets_Image) isColumns_Column_Widgets_Data() {} |
| |
| func (*Columns_Column_Widgets_DecoratedText) isColumns_Column_Widgets_Data() {} |
| |
| func (*Columns_Column_Widgets_ButtonList) isColumns_Column_Widgets_Data() {} |
| |
| func (*Columns_Column_Widgets_TextInput) isColumns_Column_Widgets_Data() {} |
| |
| func (*Columns_Column_Widgets_SelectionInput) isColumns_Column_Widgets_Data() {} |
| |
| func (*Columns_Column_Widgets_DateTimePicker) isColumns_Column_Widgets_Data() {} |
| |
| // List of string parameters to supply when the action method is invoked. |
| // For example, consider three snooze buttons: snooze now, snooze one day, |
| // or snooze next week. You might use `action method = snooze()`, passing the |
| // snooze type and snooze time in the list of string parameters. |
| // |
| // To learn more, see |
| // [`CommonEventObject`](https://developers.google.com/workspace/chat/api/reference/rest/v1/Event#commoneventobject). |
| // |
| // [Google Workspace Add-ons and Chat |
| // apps](https://developers.google.com/workspace/extend): |
| type Action_ActionParameter struct { |
| state protoimpl.MessageState |
| sizeCache protoimpl.SizeCache |
| unknownFields protoimpl.UnknownFields |
| |
| // The name of the parameter for the action script. |
| Key string `protobuf:"bytes,1,opt,name=key,proto3" json:"key,omitempty"` |
| // The value of the parameter. |
| Value string `protobuf:"bytes,2,opt,name=value,proto3" json:"value,omitempty"` |
| } |
| |
| func (x *Action_ActionParameter) Reset() { |
| *x = Action_ActionParameter{} |
| if protoimpl.UnsafeEnabled { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[33] |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| ms.StoreMessageInfo(mi) |
| } |
| } |
| |
| func (x *Action_ActionParameter) String() string { |
| return protoimpl.X.MessageStringOf(x) |
| } |
| |
| func (*Action_ActionParameter) ProtoMessage() {} |
| |
| func (x *Action_ActionParameter) ProtoReflect() protoreflect.Message { |
| mi := &file_google_apps_card_v1_card_proto_msgTypes[33] |
| if protoimpl.UnsafeEnabled && x != nil { |
| ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x)) |
| if ms.LoadMessageInfo() == nil { |
| ms.StoreMessageInfo(mi) |
| } |
| return ms |
| } |
| return mi.MessageOf(x) |
| } |
| |
| // Deprecated: Use Action_ActionParameter.ProtoReflect.Descriptor instead. |
| func (*Action_ActionParameter) Descriptor() ([]byte, []int) { |
| return file_google_apps_card_v1_card_proto_rawDescGZIP(), []int{21, 0} |
| } |
| |
| func (x *Action_ActionParameter) GetKey() string { |
| if x != nil { |
| return x.Key |
| } |
| return "" |
| } |
| |
| func (x *Action_ActionParameter) GetValue() string { |
| if x != nil { |
| return x.Value |
| } |
| return "" |
| } |
| |
| var File_google_apps_card_v1_card_proto protoreflect.FileDescriptor |
| |
| var file_google_apps_card_v1_card_proto_rawDesc = []byte{ |
| 0x0a, 0x1e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f, 0x61, 0x70, 0x70, 0x73, 0x2f, 0x63, 0x61, |
| 0x72, 0x64, 0x2f, 0x76, 0x31, 0x2f, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, |
| 0x12, 0x13, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, |
| 0x72, 0x64, 0x2e, 0x76, 0x31, 0x1a, 0x17, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f, 0x74, 0x79, |
| 0x70, 0x65, 0x2f, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x22, 0xd0, |
| 0x0a, 0x0a, 0x04, 0x43, 0x61, 0x72, 0x64, 0x12, 0x3c, 0x0a, 0x06, 0x68, 0x65, 0x61, 0x64, 0x65, |
| 0x72, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x24, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, |
| 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, |
| 0x72, 0x64, 0x2e, 0x43, 0x61, 0x72, 0x64, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x52, 0x06, 0x68, |
| 0x65, 0x61, 0x64, 0x65, 0x72, 0x12, 0x3d, 0x0a, 0x08, 0x73, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, |
| 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, |
| 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, |
| 0x72, 0x64, 0x2e, 0x53, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x08, 0x73, 0x65, 0x63, 0x74, |
| 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x5a, 0x0a, 0x15, 0x73, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x5f, |
| 0x64, 0x69, 0x76, 0x69, 0x64, 0x65, 0x72, 0x5f, 0x73, 0x74, 0x79, 0x6c, 0x65, 0x18, 0x09, 0x20, |
| 0x01, 0x28, 0x0e, 0x32, 0x26, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, |
| 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, 0x72, 0x64, 0x2e, 0x44, |
| 0x69, 0x76, 0x69, 0x64, 0x65, 0x72, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x52, 0x13, 0x73, 0x65, 0x63, |
| 0x74, 0x69, 0x6f, 0x6e, 0x44, 0x69, 0x76, 0x69, 0x64, 0x65, 0x72, 0x53, 0x74, 0x79, 0x6c, 0x65, |
| 0x12, 0x47, 0x0a, 0x0c, 0x63, 0x61, 0x72, 0x64, 0x5f, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, |
| 0x18, 0x03, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x24, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, |
| 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, 0x72, |
| 0x64, 0x2e, 0x43, 0x61, 0x72, 0x64, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x0b, 0x63, 0x61, |
| 0x72, 0x64, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x12, 0x0a, 0x04, 0x6e, 0x61, 0x6d, |
| 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x12, 0x4c, 0x0a, |
| 0x0c, 0x66, 0x69, 0x78, 0x65, 0x64, 0x5f, 0x66, 0x6f, 0x6f, 0x74, 0x65, 0x72, 0x18, 0x05, 0x20, |
| 0x01, 0x28, 0x0b, 0x32, 0x29, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, |
| 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, 0x72, 0x64, 0x2e, 0x43, |
| 0x61, 0x72, 0x64, 0x46, 0x69, 0x78, 0x65, 0x64, 0x46, 0x6f, 0x6f, 0x74, 0x65, 0x72, 0x52, 0x0b, |
| 0x66, 0x69, 0x78, 0x65, 0x64, 0x46, 0x6f, 0x6f, 0x74, 0x65, 0x72, 0x12, 0x4b, 0x0a, 0x0d, 0x64, |
| 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79, 0x5f, 0x73, 0x74, 0x79, 0x6c, 0x65, 0x18, 0x06, 0x20, 0x01, |
| 0x28, 0x0e, 0x32, 0x26, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, |
| 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, 0x72, 0x64, 0x2e, 0x44, 0x69, |
| 0x73, 0x70, 0x6c, 0x61, 0x79, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x52, 0x0c, 0x64, 0x69, 0x73, 0x70, |
| 0x6c, 0x61, 0x79, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x12, 0x4e, 0x0a, 0x10, 0x70, 0x65, 0x65, 0x6b, |
| 0x5f, 0x63, 0x61, 0x72, 0x64, 0x5f, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x18, 0x07, 0x20, 0x01, |
| 0x28, 0x0b, 0x32, 0x24, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, |
| 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, 0x72, 0x64, 0x2e, 0x43, 0x61, |
| 0x72, 0x64, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x52, 0x0e, 0x70, 0x65, 0x65, 0x6b, 0x43, 0x61, |
| 0x72, 0x64, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x1a, 0xc7, 0x01, 0x0a, 0x0a, 0x43, 0x61, 0x72, |
| 0x64, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x12, 0x14, 0x0a, 0x05, 0x74, 0x69, 0x74, 0x6c, 0x65, |
| 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x74, 0x69, 0x74, 0x6c, 0x65, 0x12, 0x1a, 0x0a, |
| 0x08, 0x73, 0x75, 0x62, 0x74, 0x69, 0x74, 0x6c, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, |
| 0x08, 0x73, 0x75, 0x62, 0x74, 0x69, 0x74, 0x6c, 0x65, 0x12, 0x44, 0x0a, 0x0a, 0x69, 0x6d, 0x61, |
| 0x67, 0x65, 0x5f, 0x74, 0x79, 0x70, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x25, 0x2e, |
| 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, |
| 0x2e, 0x76, 0x31, 0x2e, 0x57, 0x69, 0x64, 0x67, 0x65, 0x74, 0x2e, 0x49, 0x6d, 0x61, 0x67, 0x65, |
| 0x54, 0x79, 0x70, 0x65, 0x52, 0x09, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x54, 0x79, 0x70, 0x65, 0x12, |
| 0x1b, 0x0a, 0x09, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x5f, 0x75, 0x72, 0x6c, 0x18, 0x04, 0x20, 0x01, |
| 0x28, 0x09, 0x52, 0x08, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x55, 0x72, 0x6c, 0x12, 0x24, 0x0a, 0x0e, |
| 0x69, 0x6d, 0x61, 0x67, 0x65, 0x5f, 0x61, 0x6c, 0x74, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x18, 0x05, |
| 0x20, 0x01, 0x28, 0x09, 0x52, 0x0c, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x41, 0x6c, 0x74, 0x54, 0x65, |
| 0x78, 0x74, 0x1a, 0xba, 0x01, 0x0a, 0x07, 0x53, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x16, |
| 0x0a, 0x06, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, |
| 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x12, 0x35, 0x0a, 0x07, 0x77, 0x69, 0x64, 0x67, 0x65, 0x74, |
| 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, |
| 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x57, 0x69, |
| 0x64, 0x67, 0x65, 0x74, 0x52, 0x07, 0x77, 0x69, 0x64, 0x67, 0x65, 0x74, 0x73, 0x12, 0x20, 0x0a, |
| 0x0b, 0x63, 0x6f, 0x6c, 0x6c, 0x61, 0x70, 0x73, 0x69, 0x62, 0x6c, 0x65, 0x18, 0x05, 0x20, 0x01, |
| 0x28, 0x08, 0x52, 0x0b, 0x63, 0x6f, 0x6c, 0x6c, 0x61, 0x70, 0x73, 0x69, 0x62, 0x6c, 0x65, 0x12, |
| 0x3e, 0x0a, 0x1b, 0x75, 0x6e, 0x63, 0x6f, 0x6c, 0x6c, 0x61, 0x70, 0x73, 0x69, 0x62, 0x6c, 0x65, |
| 0x5f, 0x77, 0x69, 0x64, 0x67, 0x65, 0x74, 0x73, 0x5f, 0x63, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x06, |
| 0x20, 0x01, 0x28, 0x05, 0x52, 0x19, 0x75, 0x6e, 0x63, 0x6f, 0x6c, 0x6c, 0x61, 0x70, 0x73, 0x69, |
| 0x62, 0x6c, 0x65, 0x57, 0x69, 0x64, 0x67, 0x65, 0x74, 0x73, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x1a, |
| 0x68, 0x0a, 0x0a, 0x43, 0x61, 0x72, 0x64, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x21, 0x0a, |
| 0x0c, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x6c, 0x61, 0x62, 0x65, 0x6c, 0x18, 0x01, 0x20, |
| 0x01, 0x28, 0x09, 0x52, 0x0b, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4c, 0x61, 0x62, 0x65, 0x6c, |
| 0x12, 0x37, 0x0a, 0x08, 0x6f, 0x6e, 0x5f, 0x63, 0x6c, 0x69, 0x63, 0x6b, 0x18, 0x02, 0x20, 0x01, |
| 0x28, 0x0b, 0x32, 0x1c, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, |
| 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x4f, 0x6e, 0x43, 0x6c, 0x69, 0x63, 0x6b, |
| 0x52, 0x07, 0x6f, 0x6e, 0x43, 0x6c, 0x69, 0x63, 0x6b, 0x1a, 0x9d, 0x01, 0x0a, 0x0f, 0x43, 0x61, |
| 0x72, 0x64, 0x46, 0x69, 0x78, 0x65, 0x64, 0x46, 0x6f, 0x6f, 0x74, 0x65, 0x72, 0x12, 0x42, 0x0a, |
| 0x0e, 0x70, 0x72, 0x69, 0x6d, 0x61, 0x72, 0x79, 0x5f, 0x62, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x18, |
| 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, |
| 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x74, 0x74, |
| 0x6f, 0x6e, 0x52, 0x0d, 0x70, 0x72, 0x69, 0x6d, 0x61, 0x72, 0x79, 0x42, 0x75, 0x74, 0x74, 0x6f, |
| 0x6e, 0x12, 0x46, 0x0a, 0x10, 0x73, 0x65, 0x63, 0x6f, 0x6e, 0x64, 0x61, 0x72, 0x79, 0x5f, 0x62, |
| 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x67, 0x6f, |
| 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, |
| 0x31, 0x2e, 0x42, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x52, 0x0f, 0x73, 0x65, 0x63, 0x6f, 0x6e, 0x64, |
| 0x61, 0x72, 0x79, 0x42, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x22, 0x50, 0x0a, 0x0c, 0x44, 0x69, 0x76, |
| 0x69, 0x64, 0x65, 0x72, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x12, 0x1d, 0x0a, 0x19, 0x44, 0x49, 0x56, |
| 0x49, 0x44, 0x45, 0x52, 0x5f, 0x53, 0x54, 0x59, 0x4c, 0x45, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45, |
| 0x43, 0x49, 0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12, 0x11, 0x0a, 0x0d, 0x53, 0x4f, 0x4c, 0x49, |
| 0x44, 0x5f, 0x44, 0x49, 0x56, 0x49, 0x44, 0x45, 0x52, 0x10, 0x01, 0x12, 0x0e, 0x0a, 0x0a, 0x4e, |
| 0x4f, 0x5f, 0x44, 0x49, 0x56, 0x49, 0x44, 0x45, 0x52, 0x10, 0x02, 0x22, 0x44, 0x0a, 0x0c, 0x44, |
| 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x12, 0x1d, 0x0a, 0x19, 0x44, |
| 0x49, 0x53, 0x50, 0x4c, 0x41, 0x59, 0x5f, 0x53, 0x54, 0x59, 0x4c, 0x45, 0x5f, 0x55, 0x4e, 0x53, |
| 0x50, 0x45, 0x43, 0x49, 0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12, 0x08, 0x0a, 0x04, 0x50, 0x45, |
| 0x45, 0x4b, 0x10, 0x01, 0x12, 0x0b, 0x0a, 0x07, 0x52, 0x45, 0x50, 0x4c, 0x41, 0x43, 0x45, 0x10, |
| 0x02, 0x22, 0x8f, 0x07, 0x0a, 0x06, 0x57, 0x69, 0x64, 0x67, 0x65, 0x74, 0x12, 0x4b, 0x0a, 0x0e, |
| 0x74, 0x65, 0x78, 0x74, 0x5f, 0x70, 0x61, 0x72, 0x61, 0x67, 0x72, 0x61, 0x70, 0x68, 0x18, 0x01, |
| 0x20, 0x01, 0x28, 0x0b, 0x32, 0x22, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, |
| 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x54, 0x65, 0x78, 0x74, 0x50, |
| 0x61, 0x72, 0x61, 0x67, 0x72, 0x61, 0x70, 0x68, 0x48, 0x00, 0x52, 0x0d, 0x74, 0x65, 0x78, 0x74, |
| 0x50, 0x61, 0x72, 0x61, 0x67, 0x72, 0x61, 0x70, 0x68, 0x12, 0x32, 0x0a, 0x05, 0x69, 0x6d, 0x61, |
| 0x67, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, |
| 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x49, |
| 0x6d, 0x61, 0x67, 0x65, 0x48, 0x00, 0x52, 0x05, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x12, 0x4b, 0x0a, |
| 0x0e, 0x64, 0x65, 0x63, 0x6f, 0x72, 0x61, 0x74, 0x65, 0x64, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x18, |
| 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x22, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, |
| 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x65, 0x63, 0x6f, |
| 0x72, 0x61, 0x74, 0x65, 0x64, 0x54, 0x65, 0x78, 0x74, 0x48, 0x00, 0x52, 0x0d, 0x64, 0x65, 0x63, |
| 0x6f, 0x72, 0x61, 0x74, 0x65, 0x64, 0x54, 0x65, 0x78, 0x74, 0x12, 0x42, 0x0a, 0x0b, 0x62, 0x75, |
| 0x74, 0x74, 0x6f, 0x6e, 0x5f, 0x6c, 0x69, 0x73, 0x74, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, |
| 0x1f, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, |
| 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x4c, 0x69, 0x73, 0x74, |
| 0x48, 0x00, 0x52, 0x0a, 0x62, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x4c, 0x69, 0x73, 0x74, 0x12, 0x3f, |
| 0x0a, 0x0a, 0x74, 0x65, 0x78, 0x74, 0x5f, 0x69, 0x6e, 0x70, 0x75, 0x74, 0x18, 0x05, 0x20, 0x01, |
| 0x28, 0x0b, 0x32, 0x1e, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, |
| 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x54, 0x65, 0x78, 0x74, 0x49, 0x6e, 0x70, |
| 0x75, 0x74, 0x48, 0x00, 0x52, 0x09, 0x74, 0x65, 0x78, 0x74, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x12, |
| 0x4e, 0x0a, 0x0f, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x69, 0x6e, 0x70, |
| 0x75, 0x74, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, |
| 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x53, |
| 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x48, 0x00, 0x52, |
| 0x0e, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x12, |
| 0x4f, 0x0a, 0x10, 0x64, 0x61, 0x74, 0x65, 0x5f, 0x74, 0x69, 0x6d, 0x65, 0x5f, 0x70, 0x69, 0x63, |
| 0x6b, 0x65, 0x72, 0x18, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x67, 0x6f, 0x6f, 0x67, |
| 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, |
| 0x44, 0x61, 0x74, 0x65, 0x54, 0x69, 0x6d, 0x65, 0x50, 0x69, 0x63, 0x6b, 0x65, 0x72, 0x48, 0x00, |
| 0x52, 0x0e, 0x64, 0x61, 0x74, 0x65, 0x54, 0x69, 0x6d, 0x65, 0x50, 0x69, 0x63, 0x6b, 0x65, 0x72, |
| 0x12, 0x38, 0x0a, 0x07, 0x64, 0x69, 0x76, 0x69, 0x64, 0x65, 0x72, 0x18, 0x09, 0x20, 0x01, 0x28, |
| 0x0b, 0x32, 0x1c, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, |
| 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x69, 0x76, 0x69, 0x64, 0x65, 0x72, 0x48, |
| 0x00, 0x52, 0x07, 0x64, 0x69, 0x76, 0x69, 0x64, 0x65, 0x72, 0x12, 0x2f, 0x0a, 0x04, 0x67, 0x72, |
| 0x69, 0x64, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, |
| 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x47, |
| 0x72, 0x69, 0x64, 0x48, 0x00, 0x52, 0x04, 0x67, 0x72, 0x69, 0x64, 0x12, 0x38, 0x0a, 0x07, 0x63, |
| 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x73, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1c, 0x2e, 0x67, |
| 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, |
| 0x76, 0x31, 0x2e, 0x43, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x73, 0x48, 0x00, 0x52, 0x07, 0x63, 0x6f, |
| 0x6c, 0x75, 0x6d, 0x6e, 0x73, 0x12, 0x62, 0x0a, 0x14, 0x68, 0x6f, 0x72, 0x69, 0x7a, 0x6f, 0x6e, |
| 0x74, 0x61, 0x6c, 0x5f, 0x61, 0x6c, 0x69, 0x67, 0x6e, 0x6d, 0x65, 0x6e, 0x74, 0x18, 0x08, 0x20, |
| 0x01, 0x28, 0x0e, 0x32, 0x2f, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, |
| 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x57, 0x69, 0x64, 0x67, 0x65, 0x74, |
| 0x2e, 0x48, 0x6f, 0x72, 0x69, 0x7a, 0x6f, 0x6e, 0x74, 0x61, 0x6c, 0x41, 0x6c, 0x69, 0x67, 0x6e, |
| 0x6d, 0x65, 0x6e, 0x74, 0x52, 0x13, 0x68, 0x6f, 0x72, 0x69, 0x7a, 0x6f, 0x6e, 0x74, 0x61, 0x6c, |
| 0x41, 0x6c, 0x69, 0x67, 0x6e, 0x6d, 0x65, 0x6e, 0x74, 0x22, 0x23, 0x0a, 0x09, 0x49, 0x6d, 0x61, |
| 0x67, 0x65, 0x54, 0x79, 0x70, 0x65, 0x12, 0x0a, 0x0a, 0x06, 0x53, 0x51, 0x55, 0x41, 0x52, 0x45, |
| 0x10, 0x00, 0x12, 0x0a, 0x0a, 0x06, 0x43, 0x49, 0x52, 0x43, 0x4c, 0x45, 0x10, 0x01, 0x22, 0x5b, |
| 0x0a, 0x13, 0x48, 0x6f, 0x72, 0x69, 0x7a, 0x6f, 0x6e, 0x74, 0x61, 0x6c, 0x41, 0x6c, 0x69, 0x67, |
| 0x6e, 0x6d, 0x65, 0x6e, 0x74, 0x12, 0x24, 0x0a, 0x20, 0x48, 0x4f, 0x52, 0x49, 0x5a, 0x4f, 0x4e, |
| 0x54, 0x41, 0x4c, 0x5f, 0x41, 0x4c, 0x49, 0x47, 0x4e, 0x4d, 0x45, 0x4e, 0x54, 0x5f, 0x55, 0x4e, |
| 0x53, 0x50, 0x45, 0x43, 0x49, 0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12, 0x09, 0x0a, 0x05, 0x53, |
| 0x54, 0x41, 0x52, 0x54, 0x10, 0x01, 0x12, 0x0a, 0x0a, 0x06, 0x43, 0x45, 0x4e, 0x54, 0x45, 0x52, |
| 0x10, 0x02, 0x12, 0x07, 0x0a, 0x03, 0x45, 0x4e, 0x44, 0x10, 0x03, 0x42, 0x06, 0x0a, 0x04, 0x64, |
| 0x61, 0x74, 0x61, 0x22, 0x23, 0x0a, 0x0d, 0x54, 0x65, 0x78, 0x74, 0x50, 0x61, 0x72, 0x61, 0x67, |
| 0x72, 0x61, 0x70, 0x68, 0x12, 0x12, 0x0a, 0x04, 0x74, 0x65, 0x78, 0x74, 0x18, 0x01, 0x20, 0x01, |
| 0x28, 0x09, 0x52, 0x04, 0x74, 0x65, 0x78, 0x74, 0x22, 0x78, 0x0a, 0x05, 0x49, 0x6d, 0x61, 0x67, |
| 0x65, 0x12, 0x1b, 0x0a, 0x09, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x5f, 0x75, 0x72, 0x6c, 0x18, 0x01, |
| 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x55, 0x72, 0x6c, 0x12, 0x37, |
| 0x0a, 0x08, 0x6f, 0x6e, 0x5f, 0x63, 0x6c, 0x69, 0x63, 0x6b, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, |
| 0x32, 0x1c, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, |
| 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x4f, 0x6e, 0x43, 0x6c, 0x69, 0x63, 0x6b, 0x52, 0x07, |
| 0x6f, 0x6e, 0x43, 0x6c, 0x69, 0x63, 0x6b, 0x12, 0x19, 0x0a, 0x08, 0x61, 0x6c, 0x74, 0x5f, 0x74, |
| 0x65, 0x78, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x61, 0x6c, 0x74, 0x54, 0x65, |
| 0x78, 0x74, 0x22, 0x09, 0x0a, 0x07, 0x44, 0x69, 0x76, 0x69, 0x64, 0x65, 0x72, 0x22, 0xb3, 0x06, |
| 0x0a, 0x0d, 0x44, 0x65, 0x63, 0x6f, 0x72, 0x61, 0x74, 0x65, 0x64, 0x54, 0x65, 0x78, 0x74, 0x12, |
| 0x31, 0x0a, 0x04, 0x69, 0x63, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, |
| 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, |
| 0x2e, 0x76, 0x31, 0x2e, 0x49, 0x63, 0x6f, 0x6e, 0x42, 0x02, 0x18, 0x01, 0x52, 0x04, 0x69, 0x63, |
| 0x6f, 0x6e, 0x12, 0x38, 0x0a, 0x0a, 0x73, 0x74, 0x61, 0x72, 0x74, 0x5f, 0x69, 0x63, 0x6f, 0x6e, |
| 0x18, 0x0c, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, |
| 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x49, 0x63, 0x6f, |
| 0x6e, 0x52, 0x09, 0x73, 0x74, 0x61, 0x72, 0x74, 0x49, 0x63, 0x6f, 0x6e, 0x12, 0x1b, 0x0a, 0x09, |
| 0x74, 0x6f, 0x70, 0x5f, 0x6c, 0x61, 0x62, 0x65, 0x6c, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, |
| 0x08, 0x74, 0x6f, 0x70, 0x4c, 0x61, 0x62, 0x65, 0x6c, 0x12, 0x12, 0x0a, 0x04, 0x74, 0x65, 0x78, |
| 0x74, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x74, 0x65, 0x78, 0x74, 0x12, 0x1b, 0x0a, |
| 0x09, 0x77, 0x72, 0x61, 0x70, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x18, 0x05, 0x20, 0x01, 0x28, 0x08, |
| 0x52, 0x08, 0x77, 0x72, 0x61, 0x70, 0x54, 0x65, 0x78, 0x74, 0x12, 0x21, 0x0a, 0x0c, 0x62, 0x6f, |
| 0x74, 0x74, 0x6f, 0x6d, 0x5f, 0x6c, 0x61, 0x62, 0x65, 0x6c, 0x18, 0x06, 0x20, 0x01, 0x28, 0x09, |
| 0x52, 0x0b, 0x62, 0x6f, 0x74, 0x74, 0x6f, 0x6d, 0x4c, 0x61, 0x62, 0x65, 0x6c, 0x12, 0x37, 0x0a, |
| 0x08, 0x6f, 0x6e, 0x5f, 0x63, 0x6c, 0x69, 0x63, 0x6b, 0x18, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, |
| 0x1c, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, |
| 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x4f, 0x6e, 0x43, 0x6c, 0x69, 0x63, 0x6b, 0x52, 0x07, 0x6f, |
| 0x6e, 0x43, 0x6c, 0x69, 0x63, 0x6b, 0x12, 0x35, 0x0a, 0x06, 0x62, 0x75, 0x74, 0x74, 0x6f, 0x6e, |
| 0x18, 0x08, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, |
| 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x74, |
| 0x74, 0x6f, 0x6e, 0x48, 0x00, 0x52, 0x06, 0x62, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x12, 0x59, 0x0a, |
| 0x0e, 0x73, 0x77, 0x69, 0x74, 0x63, 0x68, 0x5f, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x18, |
| 0x09, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x30, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, |
| 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x65, 0x63, 0x6f, |
| 0x72, 0x61, 0x74, 0x65, 0x64, 0x54, 0x65, 0x78, 0x74, 0x2e, 0x53, 0x77, 0x69, 0x74, 0x63, 0x68, |
| 0x43, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x48, 0x00, 0x52, 0x0d, 0x73, 0x77, 0x69, 0x74, 0x63, |
| 0x68, 0x43, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x12, 0x36, 0x0a, 0x08, 0x65, 0x6e, 0x64, 0x5f, |
| 0x69, 0x63, 0x6f, 0x6e, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x67, 0x6f, 0x6f, |
| 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, |
| 0x2e, 0x49, 0x63, 0x6f, 0x6e, 0x48, 0x00, 0x52, 0x07, 0x65, 0x6e, 0x64, 0x49, 0x63, 0x6f, 0x6e, |
| 0x1a, 0xb5, 0x02, 0x0a, 0x0d, 0x53, 0x77, 0x69, 0x74, 0x63, 0x68, 0x43, 0x6f, 0x6e, 0x74, 0x72, |
| 0x6f, 0x6c, 0x12, 0x12, 0x0a, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, |
| 0x52, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, |
| 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x12, 0x1a, 0x0a, 0x08, |
| 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x08, |
| 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x12, 0x45, 0x0a, 0x10, 0x6f, 0x6e, 0x5f, 0x63, |
| 0x68, 0x61, 0x6e, 0x67, 0x65, 0x5f, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x04, 0x20, 0x01, |
| 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, |
| 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, |
| 0x0e, 0x6f, 0x6e, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, |
| 0x5f, 0x0a, 0x0c, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x5f, 0x74, 0x79, 0x70, 0x65, 0x18, |
| 0x05, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x3c, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, |
| 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x65, 0x63, 0x6f, |
| 0x72, 0x61, 0x74, 0x65, 0x64, 0x54, 0x65, 0x78, 0x74, 0x2e, 0x53, 0x77, 0x69, 0x74, 0x63, 0x68, |
| 0x43, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x2e, 0x43, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x54, |
| 0x79, 0x70, 0x65, 0x52, 0x0b, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x54, 0x79, 0x70, 0x65, |
| 0x22, 0x36, 0x0a, 0x0b, 0x43, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x54, 0x79, 0x70, 0x65, 0x12, |
| 0x0a, 0x0a, 0x06, 0x53, 0x57, 0x49, 0x54, 0x43, 0x48, 0x10, 0x00, 0x12, 0x0c, 0x0a, 0x08, 0x43, |
| 0x48, 0x45, 0x43, 0x4b, 0x42, 0x4f, 0x58, 0x10, 0x01, 0x12, 0x0d, 0x0a, 0x09, 0x43, 0x48, 0x45, |
| 0x43, 0x4b, 0x5f, 0x42, 0x4f, 0x58, 0x10, 0x02, 0x42, 0x09, 0x0a, 0x07, 0x63, 0x6f, 0x6e, 0x74, |
| 0x72, 0x6f, 0x6c, 0x22, 0xe1, 0x03, 0x0a, 0x09, 0x54, 0x65, 0x78, 0x74, 0x49, 0x6e, 0x70, 0x75, |
| 0x74, 0x12, 0x12, 0x0a, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, |
| 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x12, 0x14, 0x0a, 0x05, 0x6c, 0x61, 0x62, 0x65, 0x6c, 0x18, 0x02, |
| 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x6c, 0x61, 0x62, 0x65, 0x6c, 0x12, 0x1b, 0x0a, 0x09, 0x68, |
| 0x69, 0x6e, 0x74, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, |
| 0x68, 0x69, 0x6e, 0x74, 0x54, 0x65, 0x78, 0x74, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, |
| 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x12, 0x37, |
| 0x0a, 0x04, 0x74, 0x79, 0x70, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x23, 0x2e, 0x67, |
| 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, |
| 0x76, 0x31, 0x2e, 0x54, 0x65, 0x78, 0x74, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x2e, 0x54, 0x79, 0x70, |
| 0x65, 0x52, 0x04, 0x74, 0x79, 0x70, 0x65, 0x12, 0x45, 0x0a, 0x10, 0x6f, 0x6e, 0x5f, 0x63, 0x68, |
| 0x61, 0x6e, 0x67, 0x65, 0x5f, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x06, 0x20, 0x01, 0x28, |
| 0x0b, 0x32, 0x1b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, |
| 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x0e, |
| 0x6f, 0x6e, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x51, |
| 0x0a, 0x13, 0x69, 0x6e, 0x69, 0x74, 0x69, 0x61, 0x6c, 0x5f, 0x73, 0x75, 0x67, 0x67, 0x65, 0x73, |
| 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x20, 0x2e, 0x67, 0x6f, |
| 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, |
| 0x31, 0x2e, 0x53, 0x75, 0x67, 0x67, 0x65, 0x73, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x52, 0x12, 0x69, |
| 0x6e, 0x69, 0x74, 0x69, 0x61, 0x6c, 0x53, 0x75, 0x67, 0x67, 0x65, 0x73, 0x74, 0x69, 0x6f, 0x6e, |
| 0x73, 0x12, 0x4d, 0x0a, 0x14, 0x61, 0x75, 0x74, 0x6f, 0x5f, 0x63, 0x6f, 0x6d, 0x70, 0x6c, 0x65, |
| 0x74, 0x65, 0x5f, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x08, 0x20, 0x01, 0x28, 0x0b, 0x32, |
| 0x1b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, |
| 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x12, 0x61, 0x75, |
| 0x74, 0x6f, 0x43, 0x6f, 0x6d, 0x70, 0x6c, 0x65, 0x74, 0x65, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, |
| 0x12, 0x29, 0x0a, 0x10, 0x70, 0x6c, 0x61, 0x63, 0x65, 0x68, 0x6f, 0x6c, 0x64, 0x65, 0x72, 0x5f, |
| 0x74, 0x65, 0x78, 0x74, 0x18, 0x0c, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0f, 0x70, 0x6c, 0x61, 0x63, |
| 0x65, 0x68, 0x6f, 0x6c, 0x64, 0x65, 0x72, 0x54, 0x65, 0x78, 0x74, 0x22, 0x2a, 0x0a, 0x04, 0x54, |
| 0x79, 0x70, 0x65, 0x12, 0x0f, 0x0a, 0x0b, 0x53, 0x49, 0x4e, 0x47, 0x4c, 0x45, 0x5f, 0x4c, 0x49, |
| 0x4e, 0x45, 0x10, 0x00, 0x12, 0x11, 0x0a, 0x0d, 0x4d, 0x55, 0x4c, 0x54, 0x49, 0x50, 0x4c, 0x45, |
| 0x5f, 0x4c, 0x49, 0x4e, 0x45, 0x10, 0x01, 0x22, 0x87, 0x01, 0x0a, 0x0b, 0x53, 0x75, 0x67, 0x67, |
| 0x65, 0x73, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x45, 0x0a, 0x05, 0x69, 0x74, 0x65, 0x6d, 0x73, |
| 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x2f, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, |
| 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x75, 0x67, |
| 0x67, 0x65, 0x73, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x2e, 0x53, 0x75, 0x67, 0x67, 0x65, 0x73, 0x74, |
| 0x69, 0x6f, 0x6e, 0x49, 0x74, 0x65, 0x6d, 0x52, 0x05, 0x69, 0x74, 0x65, 0x6d, 0x73, 0x1a, 0x31, |
| 0x0a, 0x0e, 0x53, 0x75, 0x67, 0x67, 0x65, 0x73, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x74, 0x65, 0x6d, |
| 0x12, 0x14, 0x0a, 0x04, 0x74, 0x65, 0x78, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x48, 0x00, |
| 0x52, 0x04, 0x74, 0x65, 0x78, 0x74, 0x42, 0x09, 0x0a, 0x07, 0x63, 0x6f, 0x6e, 0x74, 0x65, 0x6e, |
| 0x74, 0x22, 0x43, 0x0a, 0x0a, 0x42, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x4c, 0x69, 0x73, 0x74, 0x12, |
| 0x35, 0x0a, 0x07, 0x62, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, |
| 0x32, 0x1b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, |
| 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x52, 0x07, 0x62, |
| 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x73, 0x22, 0xb9, 0x08, 0x0a, 0x0e, 0x53, 0x65, 0x6c, 0x65, 0x63, |
| 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x12, 0x12, 0x0a, 0x04, 0x6e, 0x61, 0x6d, |
| 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x12, 0x14, 0x0a, |
| 0x05, 0x6c, 0x61, 0x62, 0x65, 0x6c, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x6c, 0x61, |
| 0x62, 0x65, 0x6c, 0x12, 0x45, 0x0a, 0x04, 0x74, 0x79, 0x70, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, |
| 0x0e, 0x32, 0x31, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, |
| 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, |
| 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x2e, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, |
| 0x54, 0x79, 0x70, 0x65, 0x52, 0x04, 0x74, 0x79, 0x70, 0x65, 0x12, 0x47, 0x0a, 0x05, 0x69, 0x74, |
| 0x65, 0x6d, 0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x31, 0x2e, 0x67, 0x6f, 0x6f, 0x67, |
| 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, |
| 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x2e, 0x53, |
| 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x74, 0x65, 0x6d, 0x52, 0x05, 0x69, 0x74, |
| 0x65, 0x6d, 0x73, 0x12, 0x45, 0x0a, 0x10, 0x6f, 0x6e, 0x5f, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, |
| 0x5f, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1b, 0x2e, |
| 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, |
| 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x0e, 0x6f, 0x6e, 0x43, 0x68, |
| 0x61, 0x6e, 0x67, 0x65, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x44, 0x0a, 0x1f, 0x6d, 0x75, |
| 0x6c, 0x74, 0x69, 0x5f, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x5f, 0x6d, 0x61, 0x78, 0x5f, 0x73, |
| 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x5f, 0x69, 0x74, 0x65, 0x6d, 0x73, 0x18, 0x06, 0x20, |
| 0x01, 0x28, 0x05, 0x52, 0x1b, 0x6d, 0x75, 0x6c, 0x74, 0x69, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, |
| 0x4d, 0x61, 0x78, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x49, 0x74, 0x65, 0x6d, 0x73, |
| 0x12, 0x40, 0x0a, 0x1d, 0x6d, 0x75, 0x6c, 0x74, 0x69, 0x5f, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, |
| 0x5f, 0x6d, 0x69, 0x6e, 0x5f, 0x71, 0x75, 0x65, 0x72, 0x79, 0x5f, 0x6c, 0x65, 0x6e, 0x67, 0x74, |
| 0x68, 0x18, 0x07, 0x20, 0x01, 0x28, 0x05, 0x52, 0x19, 0x6d, 0x75, 0x6c, 0x74, 0x69, 0x53, 0x65, |
| 0x6c, 0x65, 0x63, 0x74, 0x4d, 0x69, 0x6e, 0x51, 0x75, 0x65, 0x72, 0x79, 0x4c, 0x65, 0x6e, 0x67, |
| 0x74, 0x68, 0x12, 0x4f, 0x0a, 0x14, 0x65, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x5f, 0x64, |
| 0x61, 0x74, 0x61, 0x5f, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x18, 0x08, 0x20, 0x01, 0x28, 0x0b, |
| 0x32, 0x1b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, |
| 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x48, 0x00, 0x52, |
| 0x12, 0x65, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x44, 0x61, 0x74, 0x61, 0x53, 0x6f, 0x75, |
| 0x72, 0x63, 0x65, 0x12, 0x6a, 0x0a, 0x14, 0x70, 0x6c, 0x61, 0x74, 0x66, 0x6f, 0x72, 0x6d, 0x5f, |
| 0x64, 0x61, 0x74, 0x61, 0x5f, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x18, 0x09, 0x20, 0x01, 0x28, |
| 0x0b, 0x32, 0x36, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, |
| 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, |
| 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x2e, 0x50, 0x6c, 0x61, 0x74, 0x66, 0x6f, 0x72, 0x6d, 0x44, |
| 0x61, 0x74, 0x61, 0x53, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x48, 0x00, 0x52, 0x12, 0x70, 0x6c, 0x61, |
| 0x74, 0x66, 0x6f, 0x72, 0x6d, 0x44, 0x61, 0x74, 0x61, 0x53, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x1a, |
| 0x9c, 0x01, 0x0a, 0x0d, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x74, 0x65, |
| 0x6d, 0x12, 0x12, 0x0a, 0x04, 0x74, 0x65, 0x78, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, |
| 0x04, 0x74, 0x65, 0x78, 0x74, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, |
| 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x12, 0x1a, 0x0a, 0x08, 0x73, |
| 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x08, 0x73, |
| 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x12, 0x24, 0x0a, 0x0e, 0x73, 0x74, 0x61, 0x72, 0x74, |
| 0x5f, 0x69, 0x63, 0x6f, 0x6e, 0x5f, 0x75, 0x72, 0x69, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, |
| 0x0c, 0x73, 0x74, 0x61, 0x72, 0x74, 0x49, 0x63, 0x6f, 0x6e, 0x55, 0x72, 0x69, 0x12, 0x1f, 0x0a, |
| 0x0b, 0x62, 0x6f, 0x74, 0x74, 0x6f, 0x6d, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x18, 0x05, 0x20, 0x01, |
| 0x28, 0x09, 0x52, 0x0a, 0x62, 0x6f, 0x74, 0x74, 0x6f, 0x6d, 0x54, 0x65, 0x78, 0x74, 0x1a, 0xc7, |
| 0x01, 0x0a, 0x12, 0x50, 0x6c, 0x61, 0x74, 0x66, 0x6f, 0x72, 0x6d, 0x44, 0x61, 0x74, 0x61, 0x53, |
| 0x6f, 0x75, 0x72, 0x63, 0x65, 0x12, 0x77, 0x0a, 0x12, 0x63, 0x6f, 0x6d, 0x6d, 0x6f, 0x6e, 0x5f, |
| 0x64, 0x61, 0x74, 0x61, 0x5f, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, |
| 0x0e, 0x32, 0x47, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, |
| 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, |
| 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x2e, 0x50, 0x6c, 0x61, 0x74, 0x66, 0x6f, 0x72, 0x6d, 0x44, |
| 0x61, 0x74, 0x61, 0x53, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x2e, 0x43, 0x6f, 0x6d, 0x6d, 0x6f, 0x6e, |
| 0x44, 0x61, 0x74, 0x61, 0x53, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x48, 0x00, 0x52, 0x10, 0x63, 0x6f, |
| 0x6d, 0x6d, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x61, 0x53, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x22, 0x29, |
| 0x0a, 0x10, 0x43, 0x6f, 0x6d, 0x6d, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x61, 0x53, 0x6f, 0x75, 0x72, |
| 0x63, 0x65, 0x12, 0x0b, 0x0a, 0x07, 0x55, 0x4e, 0x4b, 0x4e, 0x4f, 0x57, 0x4e, 0x10, 0x00, 0x12, |
| 0x08, 0x0a, 0x04, 0x55, 0x53, 0x45, 0x52, 0x10, 0x01, 0x42, 0x0d, 0x0a, 0x0b, 0x64, 0x61, 0x74, |
| 0x61, 0x5f, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x22, 0x5c, 0x0a, 0x0d, 0x53, 0x65, 0x6c, 0x65, |
| 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x54, 0x79, 0x70, 0x65, 0x12, 0x0d, 0x0a, 0x09, 0x43, 0x48, 0x45, |
| 0x43, 0x4b, 0x5f, 0x42, 0x4f, 0x58, 0x10, 0x00, 0x12, 0x10, 0x0a, 0x0c, 0x52, 0x41, 0x44, 0x49, |
| 0x4f, 0x5f, 0x42, 0x55, 0x54, 0x54, 0x4f, 0x4e, 0x10, 0x01, 0x12, 0x0a, 0x0a, 0x06, 0x53, 0x57, |
| 0x49, 0x54, 0x43, 0x48, 0x10, 0x02, 0x12, 0x0c, 0x0a, 0x08, 0x44, 0x52, 0x4f, 0x50, 0x44, 0x4f, |
| 0x57, 0x4e, 0x10, 0x03, 0x12, 0x10, 0x0a, 0x0c, 0x4d, 0x55, 0x4c, 0x54, 0x49, 0x5f, 0x53, 0x45, |
| 0x4c, 0x45, 0x43, 0x54, 0x10, 0x04, 0x42, 0x1a, 0x0a, 0x18, 0x6d, 0x75, 0x6c, 0x74, 0x69, 0x5f, |
| 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x5f, 0x64, 0x61, 0x74, 0x61, 0x5f, 0x73, 0x6f, 0x75, 0x72, |
| 0x63, 0x65, 0x22, 0xec, 0x02, 0x0a, 0x0e, 0x44, 0x61, 0x74, 0x65, 0x54, 0x69, 0x6d, 0x65, 0x50, |
| 0x69, 0x63, 0x6b, 0x65, 0x72, 0x12, 0x12, 0x0a, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, |
| 0x01, 0x28, 0x09, 0x52, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x12, 0x14, 0x0a, 0x05, 0x6c, 0x61, 0x62, |
| 0x65, 0x6c, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x6c, 0x61, 0x62, 0x65, 0x6c, 0x12, |
| 0x4a, 0x0a, 0x04, 0x74, 0x79, 0x70, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x36, 0x2e, |
| 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, |
| 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x61, 0x74, 0x65, 0x54, 0x69, 0x6d, 0x65, 0x50, 0x69, 0x63, 0x6b, |
| 0x65, 0x72, 0x2e, 0x44, 0x61, 0x74, 0x65, 0x54, 0x69, 0x6d, 0x65, 0x50, 0x69, 0x63, 0x6b, 0x65, |
| 0x72, 0x54, 0x79, 0x70, 0x65, 0x52, 0x04, 0x74, 0x79, 0x70, 0x65, 0x12, 0x24, 0x0a, 0x0e, 0x76, |
| 0x61, 0x6c, 0x75, 0x65, 0x5f, 0x6d, 0x73, 0x5f, 0x65, 0x70, 0x6f, 0x63, 0x68, 0x18, 0x04, 0x20, |
| 0x01, 0x28, 0x03, 0x52, 0x0c, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x4d, 0x73, 0x45, 0x70, 0x6f, 0x63, |
| 0x68, 0x12, 0x30, 0x0a, 0x14, 0x74, 0x69, 0x6d, 0x65, 0x7a, 0x6f, 0x6e, 0x65, 0x5f, 0x6f, 0x66, |
| 0x66, 0x73, 0x65, 0x74, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x05, 0x52, |
| 0x12, 0x74, 0x69, 0x6d, 0x65, 0x7a, 0x6f, 0x6e, 0x65, 0x4f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x44, |
| 0x61, 0x74, 0x65, 0x12, 0x45, 0x0a, 0x10, 0x6f, 0x6e, 0x5f, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, |
| 0x5f, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1b, 0x2e, |
| 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, |
| 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x0e, 0x6f, 0x6e, 0x43, 0x68, |
| 0x61, 0x6e, 0x67, 0x65, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x22, 0x45, 0x0a, 0x12, 0x44, 0x61, |
| 0x74, 0x65, 0x54, 0x69, 0x6d, 0x65, 0x50, 0x69, 0x63, 0x6b, 0x65, 0x72, 0x54, 0x79, 0x70, 0x65, |
| 0x12, 0x11, 0x0a, 0x0d, 0x44, 0x41, 0x54, 0x45, 0x5f, 0x41, 0x4e, 0x44, 0x5f, 0x54, 0x49, 0x4d, |
| 0x45, 0x10, 0x00, 0x12, 0x0d, 0x0a, 0x09, 0x44, 0x41, 0x54, 0x45, 0x5f, 0x4f, 0x4e, 0x4c, 0x59, |
| 0x10, 0x01, 0x12, 0x0d, 0x0a, 0x09, 0x54, 0x49, 0x4d, 0x45, 0x5f, 0x4f, 0x4e, 0x4c, 0x59, 0x10, |
| 0x02, 0x22, 0xe5, 0x01, 0x0a, 0x06, 0x42, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x12, 0x12, 0x0a, 0x04, |
| 0x74, 0x65, 0x78, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x74, 0x65, 0x78, 0x74, |
| 0x12, 0x2d, 0x0a, 0x04, 0x69, 0x63, 0x6f, 0x6e, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, |
| 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, |
| 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x49, 0x63, 0x6f, 0x6e, 0x52, 0x04, 0x69, 0x63, 0x6f, 0x6e, 0x12, |
| 0x28, 0x0a, 0x05, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x12, |
| 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x2e, 0x43, 0x6f, 0x6c, |
| 0x6f, 0x72, 0x52, 0x05, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x12, 0x37, 0x0a, 0x08, 0x6f, 0x6e, 0x5f, |
| 0x63, 0x6c, 0x69, 0x63, 0x6b, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1c, 0x2e, 0x67, 0x6f, |
| 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, |
| 0x31, 0x2e, 0x4f, 0x6e, 0x43, 0x6c, 0x69, 0x63, 0x6b, 0x52, 0x07, 0x6f, 0x6e, 0x43, 0x6c, 0x69, |
| 0x63, 0x6b, 0x12, 0x1a, 0x0a, 0x08, 0x64, 0x69, 0x73, 0x61, 0x62, 0x6c, 0x65, 0x64, 0x18, 0x05, |
| 0x20, 0x01, 0x28, 0x08, 0x52, 0x08, 0x64, 0x69, 0x73, 0x61, 0x62, 0x6c, 0x65, 0x64, 0x12, 0x19, |
| 0x0a, 0x08, 0x61, 0x6c, 0x74, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x18, 0x06, 0x20, 0x01, 0x28, 0x09, |
| 0x52, 0x07, 0x61, 0x6c, 0x74, 0x54, 0x65, 0x78, 0x74, 0x22, 0xf8, 0x01, 0x0a, 0x04, 0x49, 0x63, |
| 0x6f, 0x6e, 0x12, 0x1f, 0x0a, 0x0a, 0x6b, 0x6e, 0x6f, 0x77, 0x6e, 0x5f, 0x69, 0x63, 0x6f, 0x6e, |
| 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x48, 0x00, 0x52, 0x09, 0x6b, 0x6e, 0x6f, 0x77, 0x6e, 0x49, |
| 0x63, 0x6f, 0x6e, 0x12, 0x1b, 0x0a, 0x08, 0x69, 0x63, 0x6f, 0x6e, 0x5f, 0x75, 0x72, 0x6c, 0x18, |
| 0x02, 0x20, 0x01, 0x28, 0x09, 0x48, 0x00, 0x52, 0x07, 0x69, 0x63, 0x6f, 0x6e, 0x55, 0x72, 0x6c, |
| 0x12, 0x48, 0x0a, 0x0d, 0x6d, 0x61, 0x74, 0x65, 0x72, 0x69, 0x61, 0x6c, 0x5f, 0x69, 0x63, 0x6f, |
| 0x6e, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, |
| 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x61, |
| 0x74, 0x65, 0x72, 0x69, 0x61, 0x6c, 0x49, 0x63, 0x6f, 0x6e, 0x48, 0x00, 0x52, 0x0c, 0x6d, 0x61, |
| 0x74, 0x65, 0x72, 0x69, 0x61, 0x6c, 0x49, 0x63, 0x6f, 0x6e, 0x12, 0x19, 0x0a, 0x08, 0x61, 0x6c, |
| 0x74, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x61, 0x6c, |
| 0x74, 0x54, 0x65, 0x78, 0x74, 0x12, 0x44, 0x0a, 0x0a, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x5f, 0x74, |
| 0x79, 0x70, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x25, 0x2e, 0x67, 0x6f, 0x6f, 0x67, |
| 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, |
| 0x57, 0x69, 0x64, 0x67, 0x65, 0x74, 0x2e, 0x49, 0x6d, 0x61, 0x67, 0x65, 0x54, 0x79, 0x70, 0x65, |
| 0x52, 0x09, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x54, 0x79, 0x70, 0x65, 0x42, 0x07, 0x0a, 0x05, 0x69, |
| 0x63, 0x6f, 0x6e, 0x73, 0x22, 0x64, 0x0a, 0x0c, 0x4d, 0x61, 0x74, 0x65, 0x72, 0x69, 0x61, 0x6c, |
| 0x49, 0x63, 0x6f, 0x6e, 0x12, 0x12, 0x0a, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, |
| 0x28, 0x09, 0x52, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x66, 0x69, 0x6c, 0x6c, |
| 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x04, 0x66, 0x69, 0x6c, 0x6c, 0x12, 0x16, 0x0a, 0x06, |
| 0x77, 0x65, 0x69, 0x67, 0x68, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x05, 0x52, 0x06, 0x77, 0x65, |
| 0x69, 0x67, 0x68, 0x74, 0x12, 0x14, 0x0a, 0x05, 0x67, 0x72, 0x61, 0x64, 0x65, 0x18, 0x04, 0x20, |
| 0x01, 0x28, 0x05, 0x52, 0x05, 0x67, 0x72, 0x61, 0x64, 0x65, 0x22, 0xed, 0x01, 0x0a, 0x0e, 0x49, |
| 0x6d, 0x61, 0x67, 0x65, 0x43, 0x72, 0x6f, 0x70, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x12, 0x45, 0x0a, |
| 0x04, 0x74, 0x79, 0x70, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x31, 0x2e, 0x67, 0x6f, |
| 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, |
| 0x31, 0x2e, 0x49, 0x6d, 0x61, 0x67, 0x65, 0x43, 0x72, 0x6f, 0x70, 0x53, 0x74, 0x79, 0x6c, 0x65, |
| 0x2e, 0x49, 0x6d, 0x61, 0x67, 0x65, 0x43, 0x72, 0x6f, 0x70, 0x54, 0x79, 0x70, 0x65, 0x52, 0x04, |
| 0x74, 0x79, 0x70, 0x65, 0x12, 0x21, 0x0a, 0x0c, 0x61, 0x73, 0x70, 0x65, 0x63, 0x74, 0x5f, 0x72, |
| 0x61, 0x74, 0x69, 0x6f, 0x18, 0x02, 0x20, 0x01, 0x28, 0x01, 0x52, 0x0b, 0x61, 0x73, 0x70, 0x65, |
| 0x63, 0x74, 0x52, 0x61, 0x74, 0x69, 0x6f, 0x22, 0x71, 0x0a, 0x0d, 0x49, 0x6d, 0x61, 0x67, 0x65, |
| 0x43, 0x72, 0x6f, 0x70, 0x54, 0x79, 0x70, 0x65, 0x12, 0x1f, 0x0a, 0x1b, 0x49, 0x4d, 0x41, 0x47, |
| 0x45, 0x5f, 0x43, 0x52, 0x4f, 0x50, 0x5f, 0x54, 0x59, 0x50, 0x45, 0x5f, 0x55, 0x4e, 0x53, 0x50, |
| 0x45, 0x43, 0x49, 0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12, 0x0a, 0x0a, 0x06, 0x53, 0x51, 0x55, |
| 0x41, 0x52, 0x45, 0x10, 0x01, 0x12, 0x0a, 0x0a, 0x06, 0x43, 0x49, 0x52, 0x43, 0x4c, 0x45, 0x10, |
| 0x02, 0x12, 0x14, 0x0a, 0x10, 0x52, 0x45, 0x43, 0x54, 0x41, 0x4e, 0x47, 0x4c, 0x45, 0x5f, 0x43, |
| 0x55, 0x53, 0x54, 0x4f, 0x4d, 0x10, 0x03, 0x12, 0x11, 0x0a, 0x0d, 0x52, 0x45, 0x43, 0x54, 0x41, |
| 0x4e, 0x47, 0x4c, 0x45, 0x5f, 0x34, 0x5f, 0x33, 0x10, 0x04, 0x22, 0xf0, 0x01, 0x0a, 0x0b, 0x42, |
| 0x6f, 0x72, 0x64, 0x65, 0x72, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x12, 0x3f, 0x0a, 0x04, 0x74, 0x79, |
| 0x70, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x2b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, |
| 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x42, |
| 0x6f, 0x72, 0x64, 0x65, 0x72, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x2e, 0x42, 0x6f, 0x72, 0x64, 0x65, |
| 0x72, 0x54, 0x79, 0x70, 0x65, 0x52, 0x04, 0x74, 0x79, 0x70, 0x65, 0x12, 0x35, 0x0a, 0x0c, 0x73, |
| 0x74, 0x72, 0x6f, 0x6b, 0x65, 0x5f, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x18, 0x02, 0x20, 0x01, 0x28, |
| 0x0b, 0x32, 0x12, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x2e, |
| 0x43, 0x6f, 0x6c, 0x6f, 0x72, 0x52, 0x0b, 0x73, 0x74, 0x72, 0x6f, 0x6b, 0x65, 0x43, 0x6f, 0x6c, |
| 0x6f, 0x72, 0x12, 0x23, 0x0a, 0x0d, 0x63, 0x6f, 0x72, 0x6e, 0x65, 0x72, 0x5f, 0x72, 0x61, 0x64, |
| 0x69, 0x75, 0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x05, 0x52, 0x0c, 0x63, 0x6f, 0x72, 0x6e, 0x65, |
| 0x72, 0x52, 0x61, 0x64, 0x69, 0x75, 0x73, 0x22, 0x44, 0x0a, 0x0a, 0x42, 0x6f, 0x72, 0x64, 0x65, |
| 0x72, 0x54, 0x79, 0x70, 0x65, 0x12, 0x1b, 0x0a, 0x17, 0x42, 0x4f, 0x52, 0x44, 0x45, 0x52, 0x5f, |
| 0x54, 0x59, 0x50, 0x45, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45, 0x43, 0x49, 0x46, 0x49, 0x45, 0x44, |
| 0x10, 0x00, 0x12, 0x0d, 0x0a, 0x09, 0x4e, 0x4f, 0x5f, 0x42, 0x4f, 0x52, 0x44, 0x45, 0x52, 0x10, |
| 0x01, 0x12, 0x0a, 0x0a, 0x06, 0x53, 0x54, 0x52, 0x4f, 0x4b, 0x45, 0x10, 0x02, 0x22, 0xd1, 0x01, |
| 0x0a, 0x0e, 0x49, 0x6d, 0x61, 0x67, 0x65, 0x43, 0x6f, 0x6d, 0x70, 0x6f, 0x6e, 0x65, 0x6e, 0x74, |
| 0x12, 0x1b, 0x0a, 0x09, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x5f, 0x75, 0x72, 0x69, 0x18, 0x01, 0x20, |
| 0x01, 0x28, 0x09, 0x52, 0x08, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x55, 0x72, 0x69, 0x12, 0x19, 0x0a, |
| 0x08, 0x61, 0x6c, 0x74, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, |
| 0x07, 0x61, 0x6c, 0x74, 0x54, 0x65, 0x78, 0x74, 0x12, 0x42, 0x0a, 0x0a, 0x63, 0x72, 0x6f, 0x70, |
| 0x5f, 0x73, 0x74, 0x79, 0x6c, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x67, |
| 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, |
| 0x76, 0x31, 0x2e, 0x49, 0x6d, 0x61, 0x67, 0x65, 0x43, 0x72, 0x6f, 0x70, 0x53, 0x74, 0x79, 0x6c, |
| 0x65, 0x52, 0x09, 0x63, 0x72, 0x6f, 0x70, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x12, 0x43, 0x0a, 0x0c, |
| 0x62, 0x6f, 0x72, 0x64, 0x65, 0x72, 0x5f, 0x73, 0x74, 0x79, 0x6c, 0x65, 0x18, 0x04, 0x20, 0x01, |
| 0x28, 0x0b, 0x32, 0x20, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, |
| 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x6f, 0x72, 0x64, 0x65, 0x72, 0x53, |
| 0x74, 0x79, 0x6c, 0x65, 0x52, 0x0b, 0x62, 0x6f, 0x72, 0x64, 0x65, 0x72, 0x53, 0x74, 0x79, 0x6c, |
| 0x65, 0x22, 0xa0, 0x04, 0x0a, 0x04, 0x47, 0x72, 0x69, 0x64, 0x12, 0x14, 0x0a, 0x05, 0x74, 0x69, |
| 0x74, 0x6c, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x74, 0x69, 0x74, 0x6c, 0x65, |
| 0x12, 0x38, 0x0a, 0x05, 0x69, 0x74, 0x65, 0x6d, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, |
| 0x22, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, |
| 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x47, 0x72, 0x69, 0x64, 0x2e, 0x47, 0x72, 0x69, 0x64, 0x49, |
| 0x74, 0x65, 0x6d, 0x52, 0x05, 0x69, 0x74, 0x65, 0x6d, 0x73, 0x12, 0x43, 0x0a, 0x0c, 0x62, 0x6f, |
| 0x72, 0x64, 0x65, 0x72, 0x5f, 0x73, 0x74, 0x79, 0x6c, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, |
| 0x32, 0x20, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, |
| 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x6f, 0x72, 0x64, 0x65, 0x72, 0x53, 0x74, 0x79, |
| 0x6c, 0x65, 0x52, 0x0b, 0x62, 0x6f, 0x72, 0x64, 0x65, 0x72, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x12, |
| 0x21, 0x0a, 0x0c, 0x63, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x5f, 0x63, 0x6f, 0x75, 0x6e, 0x74, 0x18, |
| 0x04, 0x20, 0x01, 0x28, 0x05, 0x52, 0x0b, 0x63, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x43, 0x6f, 0x75, |
| 0x6e, 0x74, 0x12, 0x37, 0x0a, 0x08, 0x6f, 0x6e, 0x5f, 0x63, 0x6c, 0x69, 0x63, 0x6b, 0x18, 0x05, |
| 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1c, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, |
| 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x4f, 0x6e, 0x43, 0x6c, 0x69, |
| 0x63, 0x6b, 0x52, 0x07, 0x6f, 0x6e, 0x43, 0x6c, 0x69, 0x63, 0x6b, 0x1a, 0xa6, 0x02, 0x0a, 0x08, |
| 0x47, 0x72, 0x69, 0x64, 0x49, 0x74, 0x65, 0x6d, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01, |
| 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x39, 0x0a, 0x05, 0x69, 0x6d, 0x61, 0x67, |
| 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, |
| 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x49, 0x6d, |
| 0x61, 0x67, 0x65, 0x43, 0x6f, 0x6d, 0x70, 0x6f, 0x6e, 0x65, 0x6e, 0x74, 0x52, 0x05, 0x69, 0x6d, |
| 0x61, 0x67, 0x65, 0x12, 0x14, 0x0a, 0x05, 0x74, 0x69, 0x74, 0x6c, 0x65, 0x18, 0x03, 0x20, 0x01, |
| 0x28, 0x09, 0x52, 0x05, 0x74, 0x69, 0x74, 0x6c, 0x65, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x75, 0x62, |
| 0x74, 0x69, 0x74, 0x6c, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x73, 0x75, 0x62, |
| 0x74, 0x69, 0x74, 0x6c, 0x65, 0x12, 0x49, 0x0a, 0x06, 0x6c, 0x61, 0x79, 0x6f, 0x75, 0x74, 0x18, |
| 0x09, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x31, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, |
| 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x47, 0x72, 0x69, 0x64, |
| 0x2e, 0x47, 0x72, 0x69, 0x64, 0x49, 0x74, 0x65, 0x6d, 0x2e, 0x47, 0x72, 0x69, 0x64, 0x49, 0x74, |
| 0x65, 0x6d, 0x4c, 0x61, 0x79, 0x6f, 0x75, 0x74, 0x52, 0x06, 0x6c, 0x61, 0x79, 0x6f, 0x75, 0x74, |
| 0x22, 0x52, 0x0a, 0x0e, 0x47, 0x72, 0x69, 0x64, 0x49, 0x74, 0x65, 0x6d, 0x4c, 0x61, 0x79, 0x6f, |
| 0x75, 0x74, 0x12, 0x20, 0x0a, 0x1c, 0x47, 0x52, 0x49, 0x44, 0x5f, 0x49, 0x54, 0x45, 0x4d, 0x5f, |
| 0x4c, 0x41, 0x59, 0x4f, 0x55, 0x54, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45, 0x43, 0x49, 0x46, 0x49, |
| 0x45, 0x44, 0x10, 0x00, 0x12, 0x0e, 0x0a, 0x0a, 0x54, 0x45, 0x58, 0x54, 0x5f, 0x42, 0x45, 0x4c, |
| 0x4f, 0x57, 0x10, 0x01, 0x12, 0x0e, 0x0a, 0x0a, 0x54, 0x45, 0x58, 0x54, 0x5f, 0x41, 0x42, 0x4f, |
| 0x56, 0x45, 0x10, 0x02, 0x22, 0xac, 0x09, 0x0a, 0x07, 0x43, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x73, |
| 0x12, 0x46, 0x0a, 0x0c, 0x63, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x5f, 0x69, 0x74, 0x65, 0x6d, 0x73, |
| 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, |
| 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x6f, 0x6c, |
| 0x75, 0x6d, 0x6e, 0x73, 0x2e, 0x43, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x52, 0x0b, 0x63, 0x6f, 0x6c, |
| 0x75, 0x6d, 0x6e, 0x49, 0x74, 0x65, 0x6d, 0x73, 0x1a, 0xd8, 0x08, 0x0a, 0x06, 0x43, 0x6f, 0x6c, |
| 0x75, 0x6d, 0x6e, 0x12, 0x6b, 0x0a, 0x15, 0x68, 0x6f, 0x72, 0x69, 0x7a, 0x6f, 0x6e, 0x74, 0x61, |
| 0x6c, 0x5f, 0x73, 0x69, 0x7a, 0x65, 0x5f, 0x73, 0x74, 0x79, 0x6c, 0x65, 0x18, 0x01, 0x20, 0x01, |
| 0x28, 0x0e, 0x32, 0x37, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, |
| 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x73, |
| 0x2e, 0x43, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x2e, 0x48, 0x6f, 0x72, 0x69, 0x7a, 0x6f, 0x6e, 0x74, |
| 0x61, 0x6c, 0x53, 0x69, 0x7a, 0x65, 0x53, 0x74, 0x79, 0x6c, 0x65, 0x52, 0x13, 0x68, 0x6f, 0x72, |
| 0x69, 0x7a, 0x6f, 0x6e, 0x74, 0x61, 0x6c, 0x53, 0x69, 0x7a, 0x65, 0x53, 0x74, 0x79, 0x6c, 0x65, |
| 0x12, 0x62, 0x0a, 0x14, 0x68, 0x6f, 0x72, 0x69, 0x7a, 0x6f, 0x6e, 0x74, 0x61, 0x6c, 0x5f, 0x61, |
| 0x6c, 0x69, 0x67, 0x6e, 0x6d, 0x65, 0x6e, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x2f, |
| 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, |
| 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x57, 0x69, 0x64, 0x67, 0x65, 0x74, 0x2e, 0x48, 0x6f, 0x72, 0x69, |
| 0x7a, 0x6f, 0x6e, 0x74, 0x61, 0x6c, 0x41, 0x6c, 0x69, 0x67, 0x6e, 0x6d, 0x65, 0x6e, 0x74, 0x52, |
| 0x13, 0x68, 0x6f, 0x72, 0x69, 0x7a, 0x6f, 0x6e, 0x74, 0x61, 0x6c, 0x41, 0x6c, 0x69, 0x67, 0x6e, |
| 0x6d, 0x65, 0x6e, 0x74, 0x12, 0x64, 0x0a, 0x12, 0x76, 0x65, 0x72, 0x74, 0x69, 0x63, 0x61, 0x6c, |
| 0x5f, 0x61, 0x6c, 0x69, 0x67, 0x6e, 0x6d, 0x65, 0x6e, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0e, |
| 0x32, 0x35, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, |
| 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x73, 0x2e, 0x43, |
| 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x2e, 0x56, 0x65, 0x72, 0x74, 0x69, 0x63, 0x61, 0x6c, 0x41, 0x6c, |
| 0x69, 0x67, 0x6e, 0x6d, 0x65, 0x6e, 0x74, 0x52, 0x11, 0x76, 0x65, 0x72, 0x74, 0x69, 0x63, 0x61, |
| 0x6c, 0x41, 0x6c, 0x69, 0x67, 0x6e, 0x6d, 0x65, 0x6e, 0x74, 0x12, 0x45, 0x0a, 0x07, 0x77, 0x69, |
| 0x64, 0x67, 0x65, 0x74, 0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x2b, 0x2e, 0x67, 0x6f, |
| 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, |
| 0x31, 0x2e, 0x43, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x73, 0x2e, 0x43, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, |
| 0x2e, 0x57, 0x69, 0x64, 0x67, 0x65, 0x74, 0x73, 0x52, 0x07, 0x77, 0x69, 0x64, 0x67, 0x65, 0x74, |
| 0x73, 0x1a, 0x85, 0x04, 0x0a, 0x07, 0x57, 0x69, 0x64, 0x67, 0x65, 0x74, 0x73, 0x12, 0x4b, 0x0a, |
| 0x0e, 0x74, 0x65, 0x78, 0x74, 0x5f, 0x70, 0x61, 0x72, 0x61, 0x67, 0x72, 0x61, 0x70, 0x68, 0x18, |
| 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x22, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, |
| 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x54, 0x65, 0x78, 0x74, |
| 0x50, 0x61, 0x72, 0x61, 0x67, 0x72, 0x61, 0x70, 0x68, 0x48, 0x00, 0x52, 0x0d, 0x74, 0x65, 0x78, |
| 0x74, 0x50, 0x61, 0x72, 0x61, 0x67, 0x72, 0x61, 0x70, 0x68, 0x12, 0x32, 0x0a, 0x05, 0x69, 0x6d, |
| 0x61, 0x67, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, |
| 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, |
| 0x49, 0x6d, 0x61, 0x67, 0x65, 0x48, 0x00, 0x52, 0x05, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x12, 0x4b, |
| 0x0a, 0x0e, 0x64, 0x65, 0x63, 0x6f, 0x72, 0x61, 0x74, 0x65, 0x64, 0x5f, 0x74, 0x65, 0x78, 0x74, |
| 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x22, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, |
| 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x65, 0x63, |
| 0x6f, 0x72, 0x61, 0x74, 0x65, 0x64, 0x54, 0x65, 0x78, 0x74, 0x48, 0x00, 0x52, 0x0d, 0x64, 0x65, |
| 0x63, 0x6f, 0x72, 0x61, 0x74, 0x65, 0x64, 0x54, 0x65, 0x78, 0x74, 0x12, 0x42, 0x0a, 0x0b, 0x62, |
| 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x5f, 0x6c, 0x69, 0x73, 0x74, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0b, |
| 0x32, 0x1f, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, |
| 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x4c, 0x69, 0x73, |
| 0x74, 0x48, 0x00, 0x52, 0x0a, 0x62, 0x75, 0x74, 0x74, 0x6f, 0x6e, 0x4c, 0x69, 0x73, 0x74, 0x12, |
| 0x3f, 0x0a, 0x0a, 0x74, 0x65, 0x78, 0x74, 0x5f, 0x69, 0x6e, 0x70, 0x75, 0x74, 0x18, 0x05, 0x20, |
| 0x01, 0x28, 0x0b, 0x32, 0x1e, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, |
| 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x54, 0x65, 0x78, 0x74, 0x49, 0x6e, |
| 0x70, 0x75, 0x74, 0x48, 0x00, 0x52, 0x09, 0x74, 0x65, 0x78, 0x74, 0x49, 0x6e, 0x70, 0x75, 0x74, |
| 0x12, 0x4e, 0x0a, 0x0f, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x69, 0x6e, |
| 0x70, 0x75, 0x74, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x67, 0x6f, 0x6f, 0x67, |
| 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, |
| 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x48, 0x00, |
| 0x52, 0x0e, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, |
| 0x12, 0x4f, 0x0a, 0x10, 0x64, 0x61, 0x74, 0x65, 0x5f, 0x74, 0x69, 0x6d, 0x65, 0x5f, 0x70, 0x69, |
| 0x63, 0x6b, 0x65, 0x72, 0x18, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x67, 0x6f, 0x6f, |
| 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, |
| 0x2e, 0x44, 0x61, 0x74, 0x65, 0x54, 0x69, 0x6d, 0x65, 0x50, 0x69, 0x63, 0x6b, 0x65, 0x72, 0x48, |
| 0x00, 0x52, 0x0e, 0x64, 0x61, 0x74, 0x65, 0x54, 0x69, 0x6d, 0x65, 0x50, 0x69, 0x63, 0x6b, 0x65, |
| 0x72, 0x42, 0x06, 0x0a, 0x04, 0x64, 0x61, 0x74, 0x61, 0x22, 0x6e, 0x0a, 0x13, 0x48, 0x6f, 0x72, |
| 0x69, 0x7a, 0x6f, 0x6e, 0x74, 0x61, 0x6c, 0x53, 0x69, 0x7a, 0x65, 0x53, 0x74, 0x79, 0x6c, 0x65, |
| 0x12, 0x25, 0x0a, 0x21, 0x48, 0x4f, 0x52, 0x49, 0x5a, 0x4f, 0x4e, 0x54, 0x41, 0x4c, 0x5f, 0x53, |
| 0x49, 0x5a, 0x45, 0x5f, 0x53, 0x54, 0x59, 0x4c, 0x45, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45, 0x43, |
| 0x49, 0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12, 0x18, 0x0a, 0x14, 0x46, 0x49, 0x4c, 0x4c, 0x5f, |
| 0x41, 0x56, 0x41, 0x49, 0x4c, 0x41, 0x42, 0x4c, 0x45, 0x5f, 0x53, 0x50, 0x41, 0x43, 0x45, 0x10, |
| 0x01, 0x12, 0x16, 0x0a, 0x12, 0x46, 0x49, 0x4c, 0x4c, 0x5f, 0x4d, 0x49, 0x4e, 0x49, 0x4d, 0x55, |
| 0x4d, 0x5f, 0x53, 0x50, 0x41, 0x43, 0x45, 0x10, 0x02, 0x22, 0x58, 0x0a, 0x11, 0x56, 0x65, 0x72, |
| 0x74, 0x69, 0x63, 0x61, 0x6c, 0x41, 0x6c, 0x69, 0x67, 0x6e, 0x6d, 0x65, 0x6e, 0x74, 0x12, 0x22, |
| 0x0a, 0x1e, 0x56, 0x45, 0x52, 0x54, 0x49, 0x43, 0x41, 0x4c, 0x5f, 0x41, 0x4c, 0x49, 0x47, 0x4e, |
| 0x4d, 0x45, 0x4e, 0x54, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45, 0x43, 0x49, 0x46, 0x49, 0x45, 0x44, |
| 0x10, 0x00, 0x12, 0x0a, 0x0a, 0x06, 0x43, 0x45, 0x4e, 0x54, 0x45, 0x52, 0x10, 0x01, 0x12, 0x07, |
| 0x0a, 0x03, 0x54, 0x4f, 0x50, 0x10, 0x02, 0x12, 0x0a, 0x0a, 0x06, 0x42, 0x4f, 0x54, 0x54, 0x4f, |
| 0x4d, 0x10, 0x03, 0x22, 0x8f, 0x02, 0x0a, 0x07, 0x4f, 0x6e, 0x43, 0x6c, 0x69, 0x63, 0x6b, 0x12, |
| 0x35, 0x0a, 0x06, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, |
| 0x1b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, |
| 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x48, 0x00, 0x52, 0x06, |
| 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x3c, 0x0a, 0x09, 0x6f, 0x70, 0x65, 0x6e, 0x5f, 0x6c, |
| 0x69, 0x6e, 0x6b, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1d, 0x2e, 0x67, 0x6f, 0x6f, 0x67, |
| 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, |
| 0x4f, 0x70, 0x65, 0x6e, 0x4c, 0x69, 0x6e, 0x6b, 0x48, 0x00, 0x52, 0x08, 0x6f, 0x70, 0x65, 0x6e, |
| 0x4c, 0x69, 0x6e, 0x6b, 0x12, 0x56, 0x0a, 0x18, 0x6f, 0x70, 0x65, 0x6e, 0x5f, 0x64, 0x79, 0x6e, |
| 0x61, 0x6d, 0x69, 0x63, 0x5f, 0x6c, 0x69, 0x6e, 0x6b, 0x5f, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, |
| 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, |
| 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x63, 0x74, |
| 0x69, 0x6f, 0x6e, 0x48, 0x00, 0x52, 0x15, 0x6f, 0x70, 0x65, 0x6e, 0x44, 0x79, 0x6e, 0x61, 0x6d, |
| 0x69, 0x63, 0x4c, 0x69, 0x6e, 0x6b, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x2f, 0x0a, 0x04, |
| 0x63, 0x61, 0x72, 0x64, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x67, 0x6f, 0x6f, |
| 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, |
| 0x2e, 0x43, 0x61, 0x72, 0x64, 0x48, 0x00, 0x52, 0x04, 0x63, 0x61, 0x72, 0x64, 0x42, 0x06, 0x0a, |
| 0x04, 0x64, 0x61, 0x74, 0x61, 0x22, 0xe7, 0x01, 0x0a, 0x08, 0x4f, 0x70, 0x65, 0x6e, 0x4c, 0x69, |
| 0x6e, 0x6b, 0x12, 0x10, 0x0a, 0x03, 0x75, 0x72, 0x6c, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, |
| 0x03, 0x75, 0x72, 0x6c, 0x12, 0x3d, 0x0a, 0x07, 0x6f, 0x70, 0x65, 0x6e, 0x5f, 0x61, 0x73, 0x18, |
| 0x02, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x24, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, |
| 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x4f, 0x70, 0x65, 0x6e, |
| 0x4c, 0x69, 0x6e, 0x6b, 0x2e, 0x4f, 0x70, 0x65, 0x6e, 0x41, 0x73, 0x52, 0x06, 0x6f, 0x70, 0x65, |
| 0x6e, 0x41, 0x73, 0x12, 0x40, 0x0a, 0x08, 0x6f, 0x6e, 0x5f, 0x63, 0x6c, 0x6f, 0x73, 0x65, 0x18, |
| 0x03, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x25, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, |
| 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x4f, 0x70, 0x65, 0x6e, |
| 0x4c, 0x69, 0x6e, 0x6b, 0x2e, 0x4f, 0x6e, 0x43, 0x6c, 0x6f, 0x73, 0x65, 0x52, 0x07, 0x6f, 0x6e, |
| 0x43, 0x6c, 0x6f, 0x73, 0x65, 0x22, 0x24, 0x0a, 0x06, 0x4f, 0x70, 0x65, 0x6e, 0x41, 0x73, 0x12, |
| 0x0d, 0x0a, 0x09, 0x46, 0x55, 0x4c, 0x4c, 0x5f, 0x53, 0x49, 0x5a, 0x45, 0x10, 0x00, 0x12, 0x0b, |
| 0x0a, 0x07, 0x4f, 0x56, 0x45, 0x52, 0x4c, 0x41, 0x59, 0x10, 0x01, 0x22, 0x22, 0x0a, 0x07, 0x4f, |
| 0x6e, 0x43, 0x6c, 0x6f, 0x73, 0x65, 0x12, 0x0b, 0x0a, 0x07, 0x4e, 0x4f, 0x54, 0x48, 0x49, 0x4e, |
| 0x47, 0x10, 0x00, 0x12, 0x0a, 0x0a, 0x06, 0x52, 0x45, 0x4c, 0x4f, 0x41, 0x44, 0x10, 0x01, 0x22, |
| 0xd5, 0x03, 0x0a, 0x06, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x1a, 0x0a, 0x08, 0x66, 0x75, |
| 0x6e, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x66, 0x75, |
| 0x6e, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x4b, 0x0a, 0x0a, 0x70, 0x61, 0x72, 0x61, 0x6d, 0x65, |
| 0x74, 0x65, 0x72, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x2b, 0x2e, 0x67, 0x6f, 0x6f, |
| 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, |
| 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x50, 0x61, |
| 0x72, 0x61, 0x6d, 0x65, 0x74, 0x65, 0x72, 0x52, 0x0a, 0x70, 0x61, 0x72, 0x61, 0x6d, 0x65, 0x74, |
| 0x65, 0x72, 0x73, 0x12, 0x50, 0x0a, 0x0e, 0x6c, 0x6f, 0x61, 0x64, 0x5f, 0x69, 0x6e, 0x64, 0x69, |
| 0x63, 0x61, 0x74, 0x6f, 0x72, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x29, 0x2e, 0x67, 0x6f, |
| 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, |
| 0x31, 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x2e, 0x4c, 0x6f, 0x61, 0x64, 0x49, 0x6e, 0x64, |
| 0x69, 0x63, 0x61, 0x74, 0x6f, 0x72, 0x52, 0x0d, 0x6c, 0x6f, 0x61, 0x64, 0x49, 0x6e, 0x64, 0x69, |
| 0x63, 0x61, 0x74, 0x6f, 0x72, 0x12, 0x25, 0x0a, 0x0e, 0x70, 0x65, 0x72, 0x73, 0x69, 0x73, 0x74, |
| 0x5f, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x73, 0x18, 0x04, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0d, 0x70, |
| 0x65, 0x72, 0x73, 0x69, 0x73, 0x74, 0x56, 0x61, 0x6c, 0x75, 0x65, 0x73, 0x12, 0x49, 0x0a, 0x0b, |
| 0x69, 0x6e, 0x74, 0x65, 0x72, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x05, 0x20, 0x01, 0x28, |
| 0x0e, 0x32, 0x27, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, |
| 0x63, 0x61, 0x72, 0x64, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x2e, 0x49, |
| 0x6e, 0x74, 0x65, 0x72, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x0b, 0x69, 0x6e, 0x74, 0x65, |
| 0x72, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x1a, 0x39, 0x0a, 0x0f, 0x41, 0x63, 0x74, 0x69, 0x6f, |
| 0x6e, 0x50, 0x61, 0x72, 0x61, 0x6d, 0x65, 0x74, 0x65, 0x72, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, |
| 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a, 0x05, |
| 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, |
| 0x75, 0x65, 0x22, 0x26, 0x0a, 0x0d, 0x4c, 0x6f, 0x61, 0x64, 0x49, 0x6e, 0x64, 0x69, 0x63, 0x61, |
| 0x74, 0x6f, 0x72, 0x12, 0x0b, 0x0a, 0x07, 0x53, 0x50, 0x49, 0x4e, 0x4e, 0x45, 0x52, 0x10, 0x00, |
| 0x12, 0x08, 0x0a, 0x04, 0x4e, 0x4f, 0x4e, 0x45, 0x10, 0x01, 0x22, 0x3b, 0x0a, 0x0b, 0x49, 0x6e, |
| 0x74, 0x65, 0x72, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x1b, 0x0a, 0x17, 0x49, 0x4e, 0x54, |
| 0x45, 0x52, 0x41, 0x43, 0x54, 0x49, 0x4f, 0x4e, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45, 0x43, 0x49, |
| 0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12, 0x0f, 0x0a, 0x0b, 0x4f, 0x50, 0x45, 0x4e, 0x5f, 0x44, |
| 0x49, 0x41, 0x4c, 0x4f, 0x47, 0x10, 0x01, 0x42, 0xa4, 0x01, 0x0a, 0x17, 0x63, 0x6f, 0x6d, 0x2e, |
| 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x61, 0x70, 0x70, 0x73, 0x2e, 0x63, 0x61, 0x72, 0x64, |
| 0x2e, 0x76, 0x31, 0x42, 0x09, 0x43, 0x61, 0x72, 0x64, 0x50, 0x72, 0x6f, 0x74, 0x6f, 0x50, 0x01, |
| 0x5a, 0x37, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x67, 0x6f, 0x6c, 0x61, 0x6e, 0x67, 0x2e, |
| 0x6f, 0x72, 0x67, 0x2f, 0x67, 0x65, 0x6e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x2f, 0x67, 0x6f, 0x6f, |
| 0x67, 0x6c, 0x65, 0x61, 0x70, 0x69, 0x73, 0x2f, 0x61, 0x70, 0x70, 0x73, 0x2f, 0x63, 0x61, 0x72, |
| 0x64, 0x2f, 0x76, 0x31, 0x3b, 0x63, 0x61, 0x72, 0x64, 0xaa, 0x02, 0x13, 0x47, 0x6f, 0x6f, 0x67, |
| 0x6c, 0x65, 0x2e, 0x41, 0x70, 0x70, 0x73, 0x2e, 0x43, 0x61, 0x72, 0x64, 0x2e, 0x56, 0x31, 0xca, |
| 0x02, 0x13, 0x47, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x5c, 0x41, 0x70, 0x70, 0x73, 0x5c, 0x43, 0x61, |
| 0x72, 0x64, 0x5c, 0x56, 0x31, 0xea, 0x02, 0x16, 0x47, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x3a, 0x3a, |
| 0x41, 0x70, 0x70, 0x73, 0x3a, 0x3a, 0x43, 0x61, 0x72, 0x64, 0x3a, 0x3a, 0x56, 0x31, 0x62, 0x06, |
| 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33, |
| } |
| |
| var ( |
| file_google_apps_card_v1_card_proto_rawDescOnce sync.Once |
| file_google_apps_card_v1_card_proto_rawDescData = file_google_apps_card_v1_card_proto_rawDesc |
| ) |
| |
| func file_google_apps_card_v1_card_proto_rawDescGZIP() []byte { |
| file_google_apps_card_v1_card_proto_rawDescOnce.Do(func() { |
| file_google_apps_card_v1_card_proto_rawDescData = protoimpl.X.CompressGZIP(file_google_apps_card_v1_card_proto_rawDescData) |
| }) |
| return file_google_apps_card_v1_card_proto_rawDescData |
| } |
| |
| var file_google_apps_card_v1_card_proto_enumTypes = make([]protoimpl.EnumInfo, 18) |
| var file_google_apps_card_v1_card_proto_msgTypes = make([]protoimpl.MessageInfo, 34) |
| var file_google_apps_card_v1_card_proto_goTypes = []interface{}{ |
| (Card_DividerStyle)(0), // 0: google.apps.card.v1.Card.DividerStyle |
| (Card_DisplayStyle)(0), // 1: google.apps.card.v1.Card.DisplayStyle |
| (Widget_ImageType)(0), // 2: google.apps.card.v1.Widget.ImageType |
| (Widget_HorizontalAlignment)(0), // 3: google.apps.card.v1.Widget.HorizontalAlignment |
| (DecoratedText_SwitchControl_ControlType)(0), // 4: google.apps.card.v1.DecoratedText.SwitchControl.ControlType |
| (TextInput_Type)(0), // 5: google.apps.card.v1.TextInput.Type |
| (SelectionInput_SelectionType)(0), // 6: google.apps.card.v1.SelectionInput.SelectionType |
| (SelectionInput_PlatformDataSource_CommonDataSource)(0), // 7: google.apps.card.v1.SelectionInput.PlatformDataSource.CommonDataSource |
| (DateTimePicker_DateTimePickerType)(0), // 8: google.apps.card.v1.DateTimePicker.DateTimePickerType |
| (ImageCropStyle_ImageCropType)(0), // 9: google.apps.card.v1.ImageCropStyle.ImageCropType |
| (BorderStyle_BorderType)(0), // 10: google.apps.card.v1.BorderStyle.BorderType |
| (Grid_GridItem_GridItemLayout)(0), // 11: google.apps.card.v1.Grid.GridItem.GridItemLayout |
| (Columns_Column_HorizontalSizeStyle)(0), // 12: google.apps.card.v1.Columns.Column.HorizontalSizeStyle |
| (Columns_Column_VerticalAlignment)(0), // 13: google.apps.card.v1.Columns.Column.VerticalAlignment |
| (OpenLink_OpenAs)(0), // 14: google.apps.card.v1.OpenLink.OpenAs |
| (OpenLink_OnClose)(0), // 15: google.apps.card.v1.OpenLink.OnClose |
| (Action_LoadIndicator)(0), // 16: google.apps.card.v1.Action.LoadIndicator |
| (Action_Interaction)(0), // 17: google.apps.card.v1.Action.Interaction |
| (*Card)(nil), // 18: google.apps.card.v1.Card |
| (*Widget)(nil), // 19: google.apps.card.v1.Widget |
| (*TextParagraph)(nil), // 20: google.apps.card.v1.TextParagraph |
| (*Image)(nil), // 21: google.apps.card.v1.Image |
| (*Divider)(nil), // 22: google.apps.card.v1.Divider |
| (*DecoratedText)(nil), // 23: google.apps.card.v1.DecoratedText |
| (*TextInput)(nil), // 24: google.apps.card.v1.TextInput |
| (*Suggestions)(nil), // 25: google.apps.card.v1.Suggestions |
| (*ButtonList)(nil), // 26: google.apps.card.v1.ButtonList |
| (*SelectionInput)(nil), // 27: google.apps.card.v1.SelectionInput |
| (*DateTimePicker)(nil), // 28: google.apps.card.v1.DateTimePicker |
| (*Button)(nil), // 29: google.apps.card.v1.Button |
| (*Icon)(nil), // 30: google.apps.card.v1.Icon |
| (*MaterialIcon)(nil), // 31: google.apps.card.v1.MaterialIcon |
| (*ImageCropStyle)(nil), // 32: google.apps.card.v1.ImageCropStyle |
| (*BorderStyle)(nil), // 33: google.apps.card.v1.BorderStyle |
| (*ImageComponent)(nil), // 34: google.apps.card.v1.ImageComponent |
| (*Grid)(nil), // 35: google.apps.card.v1.Grid |
| (*Columns)(nil), // 36: google.apps.card.v1.Columns |
| (*OnClick)(nil), // 37: google.apps.card.v1.OnClick |
| (*OpenLink)(nil), // 38: google.apps.card.v1.OpenLink |
| (*Action)(nil), // 39: google.apps.card.v1.Action |
| (*Card_CardHeader)(nil), // 40: google.apps.card.v1.Card.CardHeader |
| (*Card_Section)(nil), // 41: google.apps.card.v1.Card.Section |
| (*Card_CardAction)(nil), // 42: google.apps.card.v1.Card.CardAction |
| (*Card_CardFixedFooter)(nil), // 43: google.apps.card.v1.Card.CardFixedFooter |
| (*DecoratedText_SwitchControl)(nil), // 44: google.apps.card.v1.DecoratedText.SwitchControl |
| (*Suggestions_SuggestionItem)(nil), // 45: google.apps.card.v1.Suggestions.SuggestionItem |
| (*SelectionInput_SelectionItem)(nil), // 46: google.apps.card.v1.SelectionInput.SelectionItem |
| (*SelectionInput_PlatformDataSource)(nil), // 47: google.apps.card.v1.SelectionInput.PlatformDataSource |
| (*Grid_GridItem)(nil), // 48: google.apps.card.v1.Grid.GridItem |
| (*Columns_Column)(nil), // 49: google.apps.card.v1.Columns.Column |
| (*Columns_Column_Widgets)(nil), // 50: google.apps.card.v1.Columns.Column.Widgets |
| (*Action_ActionParameter)(nil), // 51: google.apps.card.v1.Action.ActionParameter |
| (*color.Color)(nil), // 52: google.type.Color |
| } |
| var file_google_apps_card_v1_card_proto_depIdxs = []int32{ |
| 40, // 0: google.apps.card.v1.Card.header:type_name -> google.apps.card.v1.Card.CardHeader |
| 41, // 1: google.apps.card.v1.Card.sections:type_name -> google.apps.card.v1.Card.Section |
| 0, // 2: google.apps.card.v1.Card.section_divider_style:type_name -> google.apps.card.v1.Card.DividerStyle |
| 42, // 3: google.apps.card.v1.Card.card_actions:type_name -> google.apps.card.v1.Card.CardAction |
| 43, // 4: google.apps.card.v1.Card.fixed_footer:type_name -> google.apps.card.v1.Card.CardFixedFooter |
| 1, // 5: google.apps.card.v1.Card.display_style:type_name -> google.apps.card.v1.Card.DisplayStyle |
| 40, // 6: google.apps.card.v1.Card.peek_card_header:type_name -> google.apps.card.v1.Card.CardHeader |
| 20, // 7: google.apps.card.v1.Widget.text_paragraph:type_name -> google.apps.card.v1.TextParagraph |
| 21, // 8: google.apps.card.v1.Widget.image:type_name -> google.apps.card.v1.Image |
| 23, // 9: google.apps.card.v1.Widget.decorated_text:type_name -> google.apps.card.v1.DecoratedText |
| 26, // 10: google.apps.card.v1.Widget.button_list:type_name -> google.apps.card.v1.ButtonList |
| 24, // 11: google.apps.card.v1.Widget.text_input:type_name -> google.apps.card.v1.TextInput |
| 27, // 12: google.apps.card.v1.Widget.selection_input:type_name -> google.apps.card.v1.SelectionInput |
| 28, // 13: google.apps.card.v1.Widget.date_time_picker:type_name -> google.apps.card.v1.DateTimePicker |
| 22, // 14: google.apps.card.v1.Widget.divider:type_name -> google.apps.card.v1.Divider |
| 35, // 15: google.apps.card.v1.Widget.grid:type_name -> google.apps.card.v1.Grid |
| 36, // 16: google.apps.card.v1.Widget.columns:type_name -> google.apps.card.v1.Columns |
| 3, // 17: google.apps.card.v1.Widget.horizontal_alignment:type_name -> google.apps.card.v1.Widget.HorizontalAlignment |
| 37, // 18: google.apps.card.v1.Image.on_click:type_name -> google.apps.card.v1.OnClick |
| 30, // 19: google.apps.card.v1.DecoratedText.icon:type_name -> google.apps.card.v1.Icon |
| 30, // 20: google.apps.card.v1.DecoratedText.start_icon:type_name -> google.apps.card.v1.Icon |
| 37, // 21: google.apps.card.v1.DecoratedText.on_click:type_name -> google.apps.card.v1.OnClick |
| 29, // 22: google.apps.card.v1.DecoratedText.button:type_name -> google.apps.card.v1.Button |
| 44, // 23: google.apps.card.v1.DecoratedText.switch_control:type_name -> google.apps.card.v1.DecoratedText.SwitchControl |
| 30, // 24: google.apps.card.v1.DecoratedText.end_icon:type_name -> google.apps.card.v1.Icon |
| 5, // 25: google.apps.card.v1.TextInput.type:type_name -> google.apps.card.v1.TextInput.Type |
| 39, // 26: google.apps.card.v1.TextInput.on_change_action:type_name -> google.apps.card.v1.Action |
| 25, // 27: google.apps.card.v1.TextInput.initial_suggestions:type_name -> google.apps.card.v1.Suggestions |
| 39, // 28: google.apps.card.v1.TextInput.auto_complete_action:type_name -> google.apps.card.v1.Action |
| 45, // 29: google.apps.card.v1.Suggestions.items:type_name -> google.apps.card.v1.Suggestions.SuggestionItem |
| 29, // 30: google.apps.card.v1.ButtonList.buttons:type_name -> google.apps.card.v1.Button |
| 6, // 31: google.apps.card.v1.SelectionInput.type:type_name -> google.apps.card.v1.SelectionInput.SelectionType |
| 46, // 32: google.apps.card.v1.SelectionInput.items:type_name -> google.apps.card.v1.SelectionInput.SelectionItem |
| 39, // 33: google.apps.card.v1.SelectionInput.on_change_action:type_name -> google.apps.card.v1.Action |
| 39, // 34: google.apps.card.v1.SelectionInput.external_data_source:type_name -> google.apps.card.v1.Action |
| 47, // 35: google.apps.card.v1.SelectionInput.platform_data_source:type_name -> google.apps.card.v1.SelectionInput.PlatformDataSource |
| 8, // 36: google.apps.card.v1.DateTimePicker.type:type_name -> google.apps.card.v1.DateTimePicker.DateTimePickerType |
| 39, // 37: google.apps.card.v1.DateTimePicker.on_change_action:type_name -> google.apps.card.v1.Action |
| 30, // 38: google.apps.card.v1.Button.icon:type_name -> google.apps.card.v1.Icon |
| 52, // 39: google.apps.card.v1.Button.color:type_name -> google.type.Color |
| 37, // 40: google.apps.card.v1.Button.on_click:type_name -> google.apps.card.v1.OnClick |
| 31, // 41: google.apps.card.v1.Icon.material_icon:type_name -> google.apps.card.v1.MaterialIcon |
| 2, // 42: google.apps.card.v1.Icon.image_type:type_name -> google.apps.card.v1.Widget.ImageType |
| 9, // 43: google.apps.card.v1.ImageCropStyle.type:type_name -> google.apps.card.v1.ImageCropStyle.ImageCropType |
| 10, // 44: google.apps.card.v1.BorderStyle.type:type_name -> google.apps.card.v1.BorderStyle.BorderType |
| 52, // 45: google.apps.card.v1.BorderStyle.stroke_color:type_name -> google.type.Color |
| 32, // 46: google.apps.card.v1.ImageComponent.crop_style:type_name -> google.apps.card.v1.ImageCropStyle |
| 33, // 47: google.apps.card.v1.ImageComponent.border_style:type_name -> google.apps.card.v1.BorderStyle |
| 48, // 48: google.apps.card.v1.Grid.items:type_name -> google.apps.card.v1.Grid.GridItem |
| 33, // 49: google.apps.card.v1.Grid.border_style:type_name -> google.apps.card.v1.BorderStyle |
| 37, // 50: google.apps.card.v1.Grid.on_click:type_name -> google.apps.card.v1.OnClick |
| 49, // 51: google.apps.card.v1.Columns.column_items:type_name -> google.apps.card.v1.Columns.Column |
| 39, // 52: google.apps.card.v1.OnClick.action:type_name -> google.apps.card.v1.Action |
| 38, // 53: google.apps.card.v1.OnClick.open_link:type_name -> google.apps.card.v1.OpenLink |
| 39, // 54: google.apps.card.v1.OnClick.open_dynamic_link_action:type_name -> google.apps.card.v1.Action |
| 18, // 55: google.apps.card.v1.OnClick.card:type_name -> google.apps.card.v1.Card |
| 14, // 56: google.apps.card.v1.OpenLink.open_as:type_name -> google.apps.card.v1.OpenLink.OpenAs |
| 15, // 57: google.apps.card.v1.OpenLink.on_close:type_name -> google.apps.card.v1.OpenLink.OnClose |
| 51, // 58: google.apps.card.v1.Action.parameters:type_name -> google.apps.card.v1.Action.ActionParameter |
| 16, // 59: google.apps.card.v1.Action.load_indicator:type_name -> google.apps.card.v1.Action.LoadIndicator |
| 17, // 60: google.apps.card.v1.Action.interaction:type_name -> google.apps.card.v1.Action.Interaction |
| 2, // 61: google.apps.card.v1.Card.CardHeader.image_type:type_name -> google.apps.card.v1.Widget.ImageType |
| 19, // 62: google.apps.card.v1.Card.Section.widgets:type_name -> google.apps.card.v1.Widget |
| 37, // 63: google.apps.card.v1.Card.CardAction.on_click:type_name -> google.apps.card.v1.OnClick |
| 29, // 64: google.apps.card.v1.Card.CardFixedFooter.primary_button:type_name -> google.apps.card.v1.Button |
| 29, // 65: google.apps.card.v1.Card.CardFixedFooter.secondary_button:type_name -> google.apps.card.v1.Button |
| 39, // 66: google.apps.card.v1.DecoratedText.SwitchControl.on_change_action:type_name -> google.apps.card.v1.Action |
| 4, // 67: google.apps.card.v1.DecoratedText.SwitchControl.control_type:type_name -> google.apps.card.v1.DecoratedText.SwitchControl.ControlType |
| 7, // 68: google.apps.card.v1.SelectionInput.PlatformDataSource.common_data_source:type_name -> google.apps.card.v1.SelectionInput.PlatformDataSource.CommonDataSource |
| 34, // 69: google.apps.card.v1.Grid.GridItem.image:type_name -> google.apps.card.v1.ImageComponent |
| 11, // 70: google.apps.card.v1.Grid.GridItem.layout:type_name -> google.apps.card.v1.Grid.GridItem.GridItemLayout |
| 12, // 71: google.apps.card.v1.Columns.Column.horizontal_size_style:type_name -> google.apps.card.v1.Columns.Column.HorizontalSizeStyle |
| 3, // 72: google.apps.card.v1.Columns.Column.horizontal_alignment:type_name -> google.apps.card.v1.Widget.HorizontalAlignment |
| 13, // 73: google.apps.card.v1.Columns.Column.vertical_alignment:type_name -> google.apps.card.v1.Columns.Column.VerticalAlignment |
| 50, // 74: google.apps.card.v1.Columns.Column.widgets:type_name -> google.apps.card.v1.Columns.Column.Widgets |
| 20, // 75: google.apps.card.v1.Columns.Column.Widgets.text_paragraph:type_name -> google.apps.card.v1.TextParagraph |
| 21, // 76: google.apps.card.v1.Columns.Column.Widgets.image:type_name -> google.apps.card.v1.Image |
| 23, // 77: google.apps.card.v1.Columns.Column.Widgets.decorated_text:type_name -> google.apps.card.v1.DecoratedText |
| 26, // 78: google.apps.card.v1.Columns.Column.Widgets.button_list:type_name -> google.apps.card.v1.ButtonList |
| 24, // 79: google.apps.card.v1.Columns.Column.Widgets.text_input:type_name -> google.apps.card.v1.TextInput |
| 27, // 80: google.apps.card.v1.Columns.Column.Widgets.selection_input:type_name -> google.apps.card.v1.SelectionInput |
| 28, // 81: google.apps.card.v1.Columns.Column.Widgets.date_time_picker:type_name -> google.apps.card.v1.DateTimePicker |
| 82, // [82:82] is the sub-list for method output_type |
| 82, // [82:82] is the sub-list for method input_type |
| 82, // [82:82] is the sub-list for extension type_name |
| 82, // [82:82] is the sub-list for extension extendee |
| 0, // [0:82] is the sub-list for field type_name |
| } |
| |
| func init() { file_google_apps_card_v1_card_proto_init() } |
| func file_google_apps_card_v1_card_proto_init() { |
| if File_google_apps_card_v1_card_proto != nil { |
| return |
| } |
| if !protoimpl.UnsafeEnabled { |
| file_google_apps_card_v1_card_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Card); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Widget); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[2].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*TextParagraph); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[3].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Image); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[4].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Divider); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[5].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*DecoratedText); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[6].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*TextInput); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[7].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Suggestions); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[8].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*ButtonList); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[9].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*SelectionInput); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[10].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*DateTimePicker); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[11].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Button); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[12].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Icon); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[13].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*MaterialIcon); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[14].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*ImageCropStyle); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[15].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*BorderStyle); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[16].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*ImageComponent); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[17].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Grid); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[18].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Columns); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[19].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*OnClick); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[20].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*OpenLink); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[21].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Action); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[22].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Card_CardHeader); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[23].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Card_Section); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[24].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Card_CardAction); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[25].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Card_CardFixedFooter); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[26].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*DecoratedText_SwitchControl); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[27].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Suggestions_SuggestionItem); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[28].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*SelectionInput_SelectionItem); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[29].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*SelectionInput_PlatformDataSource); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[30].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Grid_GridItem); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[31].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Columns_Column); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[32].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Columns_Column_Widgets); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[33].Exporter = func(v interface{}, i int) interface{} { |
| switch v := v.(*Action_ActionParameter); i { |
| case 0: |
| return &v.state |
| case 1: |
| return &v.sizeCache |
| case 2: |
| return &v.unknownFields |
| default: |
| return nil |
| } |
| } |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[1].OneofWrappers = []interface{}{ |
| (*Widget_TextParagraph)(nil), |
| (*Widget_Image)(nil), |
| (*Widget_DecoratedText)(nil), |
| (*Widget_ButtonList)(nil), |
| (*Widget_TextInput)(nil), |
| (*Widget_SelectionInput)(nil), |
| (*Widget_DateTimePicker)(nil), |
| (*Widget_Divider)(nil), |
| (*Widget_Grid)(nil), |
| (*Widget_Columns)(nil), |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[5].OneofWrappers = []interface{}{ |
| (*DecoratedText_Button)(nil), |
| (*DecoratedText_SwitchControl_)(nil), |
| (*DecoratedText_EndIcon)(nil), |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[9].OneofWrappers = []interface{}{ |
| (*SelectionInput_ExternalDataSource)(nil), |
| (*SelectionInput_PlatformDataSource_)(nil), |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[12].OneofWrappers = []interface{}{ |
| (*Icon_KnownIcon)(nil), |
| (*Icon_IconUrl)(nil), |
| (*Icon_MaterialIcon)(nil), |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[19].OneofWrappers = []interface{}{ |
| (*OnClick_Action)(nil), |
| (*OnClick_OpenLink)(nil), |
| (*OnClick_OpenDynamicLinkAction)(nil), |
| (*OnClick_Card)(nil), |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[27].OneofWrappers = []interface{}{ |
| (*Suggestions_SuggestionItem_Text)(nil), |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[29].OneofWrappers = []interface{}{ |
| (*SelectionInput_PlatformDataSource_CommonDataSource_)(nil), |
| } |
| file_google_apps_card_v1_card_proto_msgTypes[32].OneofWrappers = []interface{}{ |
| (*Columns_Column_Widgets_TextParagraph)(nil), |
| (*Columns_Column_Widgets_Image)(nil), |
| (*Columns_Column_Widgets_DecoratedText)(nil), |
| (*Columns_Column_Widgets_ButtonList)(nil), |
| (*Columns_Column_Widgets_TextInput)(nil), |
| (*Columns_Column_Widgets_SelectionInput)(nil), |
| (*Columns_Column_Widgets_DateTimePicker)(nil), |
| } |
| type x struct{} |
| out := protoimpl.TypeBuilder{ |
| File: protoimpl.DescBuilder{ |
| GoPackagePath: reflect.TypeOf(x{}).PkgPath(), |
| RawDescriptor: file_google_apps_card_v1_card_proto_rawDesc, |
| NumEnums: 18, |
| NumMessages: 34, |
| NumExtensions: 0, |
| NumServices: 0, |
| }, |
| GoTypes: file_google_apps_card_v1_card_proto_goTypes, |
| DependencyIndexes: file_google_apps_card_v1_card_proto_depIdxs, |
| EnumInfos: file_google_apps_card_v1_card_proto_enumTypes, |
| MessageInfos: file_google_apps_card_v1_card_proto_msgTypes, |
| }.Build() |
| File_google_apps_card_v1_card_proto = out.File |
| file_google_apps_card_v1_card_proto_rawDesc = nil |
| file_google_apps_card_v1_card_proto_goTypes = nil |
| file_google_apps_card_v1_card_proto_depIdxs = nil |
| } |