[dart] Update 3p packages
Change-Id: Ibae8445befa8fb76bfc1b373fc731f2eb276d81e
diff --git a/_discoveryapis_commons/BUILD.gn b/_discoveryapis_commons/BUILD.gn
index 4d4d6a3..8c50ada 100644
--- a/_discoveryapis_commons/BUILD.gn
+++ b/_discoveryapis_commons/BUILD.gn
@@ -1,4 +1,4 @@
-# This file is generated by importer.py for _discoveryapis_commons-0.1.6
+# This file is generated by importer.py for _discoveryapis_commons-0.1.6+1
import("//build/dart/dart_library.gni")
diff --git a/_discoveryapis_commons/CHANGELOG.md b/_discoveryapis_commons/CHANGELOG.md
index ce94f04..f745593 100644
--- a/_discoveryapis_commons/CHANGELOG.md
+++ b/_discoveryapis_commons/CHANGELOG.md
@@ -1,3 +1,7 @@
+## 0.1.6+1
+
+- Require Dart 2.0.0-dev.64
+
## 0.1.6
- Fix Dart 2 runtime issue.
diff --git a/_discoveryapis_commons/analysis_options.yaml b/_discoveryapis_commons/analysis_options.yaml
index ee64972..4ef8ee7 100644
--- a/_discoveryapis_commons/analysis_options.yaml
+++ b/_discoveryapis_commons/analysis_options.yaml
@@ -1,3 +1,80 @@
# https://www.dartlang.org/guides/language/analysis-options
analyzer:
- strong-mode: true
+ strong-mode:
+ implicit-casts: false
+ errors:
+ dead_code: error
+ override_on_non_overriding_method: error
+ unused_element: error
+ unused_import: error
+ unused_local_variable: error
+linter:
+ rules:
+ - always_declare_return_types
+ #- annotate_overrides
+ - avoid_empty_else
+ - avoid_function_literals_in_foreach_calls
+ - avoid_init_to_null
+ - avoid_null_checks_in_equality_operators
+ - avoid_renaming_method_parameters
+ - avoid_return_types_on_setters
+ - avoid_returning_null
+ - avoid_types_as_parameter_names
+ - avoid_unused_constructor_parameters
+ - await_only_futures
+ - camel_case_types
+ - cancel_subscriptions
+ #- cascade_invocations
+ #- comment_references
+ #- constant_identifier_names
+ - control_flow_in_finally
+ - directives_ordering
+ - empty_catches
+ - empty_constructor_bodies
+ - empty_statements
+ - hash_and_equals
+ - implementation_imports
+ - invariant_booleans
+ - iterable_contains_unrelated_type
+ #- library_names
+ - library_prefixes
+ - list_remove_unrelated_type
+ - literal_only_boolean_expressions
+ - no_adjacent_strings_in_list
+ - no_duplicate_case_values
+ #- non_constant_identifier_names
+ - omit_local_variable_types
+ - only_throw_errors
+ - overridden_fields
+ #- package_api_docs
+ - package_names
+ - package_prefixed_library_names
+ - prefer_adjacent_string_concatenation
+ - prefer_collection_literals
+ - prefer_conditional_assignment
+ - prefer_const_constructors
+ - prefer_contains
+ - prefer_equal_for_default_values
+ - prefer_final_fields
+ - prefer_initializing_formals
+ #- prefer_interpolation_to_compose_strings
+ - prefer_is_empty
+ - prefer_is_not_empty
+ - prefer_single_quotes
+ - prefer_typing_uninitialized_variables
+ - recursive_getters
+ #- slash_for_doc_comments
+ - super_goes_last
+ - test_types_in_equals
+ - throw_in_finally
+ - type_init_formals
+ - unawaited_futures
+ - unnecessary_brace_in_string_interps
+ - unnecessary_getters_setters
+ - unnecessary_lambdas
+ - unnecessary_null_aware_assignments
+ - unnecessary_statements
+ - unnecessary_this
+ - unrelated_type_equality_checks
+ - use_rethrow_when_possible
+ - valid_regexps
diff --git a/_discoveryapis_commons/lib/src/clients.dart b/_discoveryapis_commons/lib/src/clients.dart
index c28b252..be90347 100644
--- a/_discoveryapis_commons/lib/src/clients.dart
+++ b/_discoveryapis_commons/lib/src/clients.dart
@@ -4,11 +4,10 @@
library discoveryapis_commons.clients;
-import "dart:async";
-import "dart:collection" as collection;
-import "dart:convert";
+import 'dart:async';
+import 'dart:convert';
-import "package:http/http.dart" as http;
+import 'package:http/http.dart' as http;
import 'requests.dart' as client_requests;
@@ -47,14 +46,14 @@
*/
Future request(String requestUrl, String method,
{String body,
- Map queryParams,
+ Map<String, List<String>> queryParams,
client_requests.Media uploadMedia,
client_requests.UploadOptions uploadOptions,
- client_requests.DownloadOptions downloadOptions:
+ client_requests.DownloadOptions downloadOptions =
client_requests.DownloadOptions.Metadata}) {
if (uploadMedia != null &&
downloadOptions != client_requests.DownloadOptions.Metadata) {
- throw new ArgumentError('When uploading a [Media] you cannot download a '
+ throw ArgumentError('When uploading a [Media] you cannot download a '
'[Media] at the same time!');
}
client_requests.ByteRange downloadRange;
@@ -81,18 +80,18 @@
return json.decode(bodyString);
});
} else {
- throw new client_requests.ApiRequestError(
- "Unable to read response with content-type "
+ throw client_requests.ApiRequestError(
+ 'Unable to read response with content-type '
"${response.headers['content-type']}.");
}
} else {
// Downloading Media.
var contentType = response.headers['content-type'];
if (contentType == null) {
- throw new client_requests.ApiRequestError(
+ throw client_requests.ApiRequestError(
"No 'content-type' header in media response.");
}
- var contentLength;
+ int contentLength;
try {
contentLength = int.parse(response.headers['content-length']);
} catch (_) {
@@ -104,20 +103,20 @@
if (downloadRange != null) {
if (contentLength != downloadRange.length) {
- throw new client_requests.ApiRequestError(
- "Content length of response does not match requested range "
- "length.");
+ throw client_requests.ApiRequestError(
+ 'Content length of response does not match requested range '
+ 'length.');
}
var contentRange = response.headers['content-range'];
var expected = 'bytes ${downloadRange.start}-${downloadRange.end}/';
if (contentRange == null || !contentRange.startsWith(expected)) {
- throw new client_requests.ApiRequestError("Attempting partial "
+ throw client_requests.ApiRequestError('Attempting partial '
"download but got invalid 'Content-Range' header "
- "(was: $contentRange, expected: $expected).");
+ '(was: $contentRange, expected: $expected).');
}
}
- return new client_requests.Media(response.stream, contentLength,
+ return client_requests.Media(response.stream, contentLength,
contentType: contentType);
}
});
@@ -132,10 +131,10 @@
client_requests.UploadOptions uploadOptions,
client_requests.DownloadOptions downloadOptions,
client_requests.ByteRange downloadRange) {
- bool downloadAsMedia = downloadOptions != null &&
+ var downloadAsMedia = downloadOptions != null &&
downloadOptions != client_requests.DownloadOptions.Metadata;
- if (queryParams == null) queryParams = {};
+ queryParams ??= {};
if (uploadMedia != null) {
if (uploadOptions is client_requests.ResumableUploadOptions) {
@@ -153,15 +152,15 @@
queryParams['alt'] = const ['json'];
}
- var path;
+ String path;
if (requestUrl.startsWith('/')) {
- path = "$_rootUrl${requestUrl.substring(1)}";
+ path = '$_rootUrl${requestUrl.substring(1)}';
} else {
- path = "$_rootUrl${_basePath}$requestUrl";
+ path = '$_rootUrl$_basePath$requestUrl';
}
- bool containsQueryParameter = path.contains('?');
- addQueryParameter(String name, String value) {
+ var containsQueryParameter = path.contains('?');
+ void addQueryParameter(String name, String value) {
name = Escaper.escapeQueryComponent(name);
value = Escaper.escapeQueryComponent(value);
if (containsQueryParameter) {
@@ -180,9 +179,9 @@
var uri = Uri.parse(path);
- Future simpleUpload() {
+ Future<http.StreamedResponse> simpleUpload() {
var bodyStream = uploadMedia.stream;
- var request = new _RequestImpl(method, uri, bodyStream);
+ var request = _RequestImpl(method, uri, bodyStream);
request.headers.addAll({
'user-agent': _userAgent,
'content-type': uploadMedia.contentType,
@@ -191,9 +190,9 @@
return _httpClient.send(request);
}
- Future simpleRequest() {
+ Future<http.StreamedResponse> simpleRequest() {
var length = 0;
- var bodyController = new StreamController<List<int>>();
+ var bodyController = StreamController<List<int>>();
if (body != null) {
var bytes = utf8.encode(body);
bodyController.add(bytes);
@@ -201,7 +200,7 @@
}
bodyController.close();
- var headers;
+ Map<String, String> headers;
if (downloadRange != null) {
headers = {
'user-agent': _userAgent,
@@ -217,7 +216,7 @@
};
}
- var request = new _RequestImpl(method, uri, bodyController.stream);
+ var request = _RequestImpl(method, uri, bodyController.stream);
request.headers.addAll(headers);
return _httpClient.send(request);
}
@@ -229,13 +228,13 @@
// 3. Multipart: Upload of data + metadata.
if (uploadOptions is client_requests.ResumableUploadOptions) {
- var helper = new ResumableMediaUploader(_httpClient, uploadMedia, body,
- uri, method, uploadOptions, _userAgent);
+ var helper = ResumableMediaUploader(_httpClient, uploadMedia, body, uri,
+ method, uploadOptions, _userAgent);
return helper.upload();
}
if (uploadMedia.length == null) {
- throw new ArgumentError(
+ throw ArgumentError(
'For non-resumable uploads you need to specify the length of the '
'media to upload.');
}
@@ -243,7 +242,7 @@
if (body == null) {
return simpleUpload();
} else {
- var uploader = new MultipartMediaUploader(
+ var uploader = MultipartMediaUploader(
_httpClient, uploadMedia, body, uri, method, _userAgent);
return uploader.upload();
}
@@ -257,7 +256,7 @@
*/
class MultipartMediaUploader {
static final _boundary = '314159265358979323846';
- static final _base64Encoder = new Base64Encoder();
+ static final _base64Encoder = Base64Encoder();
final http.Client _httpClient;
final client_requests.Media _uploadMedia;
@@ -279,21 +278,21 @@
// This guarantees us that [_body] cannot contain a valid multipart
// boundary.
var bodyHead = '--$_boundary\r\n'
- "Content-Type: $CONTENT_TYPE_JSON_UTF8\r\n\r\n" +
+ 'Content-Type: $CONTENT_TYPE_JSON_UTF8\r\n\r\n' +
_body +
'\r\n--$_boundary\r\n'
- "Content-Type: ${_uploadMedia.contentType}\r\n"
- "Content-Transfer-Encoding: base64\r\n\r\n";
+ 'Content-Type: ${_uploadMedia.contentType}\r\n'
+ 'Content-Transfer-Encoding: base64\r\n\r\n';
var bodyTail = '\r\n--$_boundary--';
var totalLength =
bodyHead.length + base64MediaStreamLength + bodyTail.length;
- var bodyController = new StreamController<List<int>>();
+ var bodyController = StreamController<List<int>>();
bodyController.add(utf8.encode(bodyHead));
bodyController.addStream(base64MediaStream).then((_) {
bodyController.add(utf8.encode(bodyTail));
- }).catchError((error, stack) {
+ }).catchError((error, StackTrace stack) {
bodyController.addError(error, stack);
}).then((_) {
bodyController.close();
@@ -301,11 +300,11 @@
var headers = {
'user-agent': _userAgent,
- 'content-type': "multipart/related; boundary=\"$_boundary\"",
+ 'content-type': 'multipart/related; boundary=\"$_boundary\"',
'content-length': '$totalLength'
};
var bodyStream = bodyController.stream;
- var request = new _RequestImpl(_method, _uri, bodyStream);
+ var request = _RequestImpl(_method, _uri, bodyStream);
request.headers.addAll(headers);
return _httpClient.send(request);
}
@@ -323,7 +322,7 @@
StreamController<String> controller;
// Holds between 0 and 3 bytes and is used as a buffer.
- List<int> remainingBytes = [];
+ var remainingBytes = <int>[];
void onData(List<int> bytes) {
if ((remainingBytes.length + bytes.length) < 3) {
@@ -331,7 +330,7 @@
return;
}
int start;
- if (remainingBytes.length == 0) {
+ if (remainingBytes.isEmpty) {
start = 0;
} else if (remainingBytes.length == 1) {
remainingBytes.add(bytes[0]);
@@ -343,13 +342,13 @@
}
// Convert & Send bytes from buffer (if necessary).
- if (remainingBytes.length > 0) {
+ if (remainingBytes.isNotEmpty) {
controller.add(base64.encode(remainingBytes));
remainingBytes.clear();
}
- int chunksOf3 = (bytes.length - start) ~/ 3;
- int end = start + 3 * chunksOf3;
+ var chunksOf3 = (bytes.length - start) ~/ 3;
+ var end = start + 3 * chunksOf3;
// Convert & Send main bytes.
if (start == 0 && end == bytes.length) {
@@ -365,20 +364,20 @@
}
}
- void onError(error, stack) {
+ void onError(error, StackTrace stack) {
controller.addError(error, stack);
}
void onDone() {
- if (remainingBytes.length > 0) {
+ if (remainingBytes.isNotEmpty) {
controller.add(base64.encode(remainingBytes));
remainingBytes.clear();
}
controller.close();
}
- var subscription;
- controller = new StreamController<String>(onListen: () {
+ StreamSubscription subscription;
+ controller = StreamController<String>(onListen: () {
subscription = stream.listen(onData, onError: onError, onDone: onDone);
}, onPause: () {
subscription.pause();
@@ -417,40 +416,40 @@
return _startSession().then((Uri uploadUri) {
StreamSubscription subscription;
- var completer = new Completer<http.StreamedResponse>();
- bool completed = false;
+ var completer = Completer<http.StreamedResponse>();
+ var completed = false;
- var chunkStack = new ChunkStack(_options.chunkSize);
+ var chunkStack = ChunkStack(_options.chunkSize);
subscription = _uploadMedia.stream.listen((List<int> bytes) {
chunkStack.addBytes(bytes);
// Upload all but the last chunk.
// The final send will be done in the [onDone] handler.
- bool hasPartialChunk = chunkStack.hasPartialChunk;
+ var hasPartialChunk = chunkStack.hasPartialChunk;
if (chunkStack.length > 1 ||
(chunkStack.length == 1 && hasPartialChunk)) {
// Pause the input stream.
subscription.pause();
// Upload all chunks except the last one.
- var fullChunks;
+ Iterable<ResumableChunk> fullChunks;
if (hasPartialChunk) {
fullChunks = chunkStack.removeSublist(0, chunkStack.length);
} else {
fullChunks = chunkStack.removeSublist(0, chunkStack.length - 1);
}
- Future
- .forEach(fullChunks, (c) => _uploadChunkDrained(uploadUri, c))
+ Future.forEach(fullChunks,
+ (ResumableChunk c) => _uploadChunkDrained(uploadUri, c))
.then((_) {
// All chunks uploaded, we can continue consuming data.
subscription.resume();
- }).catchError((error, stack) {
+ }).catchError((error, StackTrace stack) {
subscription.cancel();
completed = true;
completer.completeError(error, stack);
});
}
- }, onError: (error, stack) {
+ }, onError: (error, StackTrace stack) {
subscription.cancel();
if (!completed) {
completed = true;
@@ -460,11 +459,11 @@
if (!completed) {
chunkStack.finalize();
- var lastChunk;
+ ResumableChunk lastChunk;
if (chunkStack.length == 1) {
lastChunk = chunkStack.removeSublist(0, chunkStack.length).first;
} else {
- completer.completeError(new StateError(
+ completer.completeError(StateError(
'Resumable uploads need to result in at least one non-empty '
'chunk at the end.'));
return;
@@ -475,11 +474,11 @@
// specified.
if (_uploadMedia.length != null) {
if (end < _uploadMedia.length) {
- completer.completeError(new client_requests.ApiRequestError(
+ completer.completeError(client_requests.ApiRequestError(
'Received less bytes than indicated by [Media.length].'));
return;
} else if (end > _uploadMedia.length) {
- completer.completeError(new client_requests.ApiRequestError(
+ completer.completeError(client_requests.ApiRequestError(
'Received more bytes than indicated by [Media.length].'));
return;
}
@@ -490,7 +489,7 @@
_uploadChunkResumable(uploadUri, lastChunk, lastChunk: true)
.then((response) {
completer.complete(response);
- }).catchError((error, stack) {
+ }).catchError((error, StackTrace stack) {
completer.completeError(error, stack);
});
}
@@ -507,14 +506,14 @@
*/
Future<Uri> _startSession() {
var length = 0;
- var bytes;
+ List<int> bytes;
if (_body != null) {
bytes = utf8.encode(_body);
length = bytes.length;
}
var bodyStream = _bytes2Stream(bytes);
- var request = new _RequestImpl(_method, _uri, bodyStream);
+ var request = _RequestImpl(_method, _uri, bodyStream);
request.headers.addAll({
'user-agent': _userAgent,
'content-type': CONTENT_TYPE_JSON_UTF8,
@@ -527,7 +526,7 @@
return response.stream.drain().then((_) {
var uploadUri = response.headers['location'];
if (response.statusCode != 200 || uploadUri == null) {
- throw new client_requests.ApiRequestError(
+ throw client_requests.ApiRequestError(
'Invalid response for resumable upload attempt '
'(status was: ${response.statusCode})');
}
@@ -549,9 +548,10 @@
/**
* Does repeated attempts to upload [chunk].
*/
- Future _uploadChunkResumable(Uri uri, ResumableChunk chunk,
- {bool lastChunk: false}) {
- tryUpload(int attemptsLeft) {
+ Future<http.StreamedResponse> _uploadChunkResumable(
+ Uri uri, ResumableChunk chunk,
+ {bool lastChunk = false}) {
+ Future<http.StreamedResponse> tryUpload(int attemptsLeft) {
return _uploadChunk(uri, chunk, lastChunk: lastChunk)
.then((http.StreamedResponse response) {
var status = response.statusCode;
@@ -559,29 +559,29 @@
(status == 500 || (502 <= status && status < 504))) {
return response.stream.drain().then((_) {
// Delay the next attempt. Default backoff function is exponential.
- int failedAttemts = _options.numberOfAttempts - attemptsLeft;
- var duration = _options.backoffFunction(failedAttemts);
+ var failedAttemts = _options.numberOfAttempts - attemptsLeft;
+ Duration duration = _options.backoffFunction(failedAttemts);
if (duration == null) {
- throw new client_requests.DetailedApiRequestError(
+ throw client_requests.DetailedApiRequestError(
status,
'Resumable upload: Uploading a chunk resulted in status '
'$status. Maximum number of retries reached.');
}
- return new Future.delayed(duration).then((_) {
+ return Future.delayed(duration).then((_) {
return tryUpload(attemptsLeft - 1);
});
});
} else if (!lastChunk && status != 308) {
return response.stream.drain().then((_) {
- throw new client_requests.DetailedApiRequestError(
+ throw client_requests.DetailedApiRequestError(
status,
'Resumable upload: Uploading a chunk resulted in status '
'$status instead of 308.');
});
} else if (lastChunk && status != 201 && status != 200) {
return response.stream.drain().then((_) {
- throw new client_requests.DetailedApiRequestError(
+ throw client_requests.DetailedApiRequestError(
status,
'Resumable upload: Uploading a chunk resulted in status '
'$status instead of 200 or 201.');
@@ -605,7 +605,7 @@
* the upload did not succeed. The response stream will not be listened to.
*/
Future<http.StreamedResponse> _uploadChunk(Uri uri, ResumableChunk chunk,
- {bool lastChunk: false}) {
+ {bool lastChunk = false}) {
// If [uploadMedia.length] is null, we do not know the length.
var mediaTotalLength =
_uploadMedia.length == null ? null : _uploadMedia.length.toString();
@@ -626,13 +626,13 @@
};
var stream = _listOfBytes2Stream(chunk.byteArrays);
- var request = new _RequestImpl('PUT', uri, stream);
+ var request = _RequestImpl('PUT', uri, stream);
request.headers.addAll(headers);
return _httpClient.send(request);
}
Stream<List<int>> _bytes2Stream(List<int> bytes) {
- var bodyController = new StreamController<List<int>>();
+ var bodyController = StreamController<List<int>>();
if (bytes != null) {
bodyController.add(bytes);
}
@@ -641,7 +641,7 @@
}
Stream<List<int>> _listOfBytes2Stream(List<List<int>> listOfBytes) {
- var controller = new StreamController<List<int>>();
+ var controller = StreamController<List<int>>();
for (var array in listOfBytes) {
controller.add(array);
}
@@ -683,7 +683,7 @@
*/
int get totalByteLength {
if (!_finalized) {
- throw new StateError('ChunkStack has not been finalized yet.');
+ throw StateError('ChunkStack has not been finalized yet.');
}
return _offset;
@@ -704,7 +704,7 @@
*/
void addBytes(List<int> bytes) {
if (_finalized) {
- throw new StateError('ChunkStack has already been finalized.');
+ throw StateError('ChunkStack has already been finalized.');
}
var remaining = _chunkSize - _length;
@@ -716,14 +716,14 @@
_byteArrays.add(left);
_length += left.length;
- _chunkStack.add(new ResumableChunk(_byteArrays, _offset, _length));
+ _chunkStack.add(ResumableChunk(_byteArrays, _offset, _length));
_byteArrays = [];
_offset += _length;
_length = 0;
addBytes(right);
- } else if (bytes.length > 0) {
+ } else if (bytes.isNotEmpty) {
_byteArrays.add(bytes);
_length += bytes.length;
}
@@ -735,12 +735,12 @@
*/
void finalize() {
if (_finalized) {
- throw new StateError('ChunkStack has already been finalized.');
+ throw StateError('ChunkStack has already been finalized.');
}
_finalized = true;
if (_length > 0) {
- _chunkStack.add(new ResumableChunk(_byteArrays, _offset, _length));
+ _chunkStack.add(ResumableChunk(_byteArrays, _offset, _length));
_offset += _length;
}
}
@@ -766,12 +766,12 @@
final Stream<List<int>> _stream;
_RequestImpl(String method, Uri url, [Stream<List<int>> stream])
- : _stream = stream == null ? new Stream.fromIterable([]) : stream,
+ : _stream = stream == null ? Stream.fromIterable([]) : stream,
super(method, url);
http.ByteStream finalize() {
super.finalize();
- return new http.ByteStream(_stream);
+ return http.ByteStream(_stream);
}
}
@@ -858,27 +858,27 @@
// TODO: We assume that status codes between [200..400[ are OK.
// Can we assume this?
if (statusCode < 200 || statusCode >= 400) {
- throwGeneralError() {
- throw new client_requests.DetailedApiRequestError(
- statusCode, 'No error details. HTTP status was: ${statusCode}.');
+ void throwGeneralError() {
+ throw client_requests.DetailedApiRequestError(
+ statusCode, 'No error details. HTTP status was: $statusCode.');
}
// Some error happened, try to decode the response and fetch the error.
- Stream<String> stringStream = _decodeStreamAsText(response);
+ var stringStream = _decodeStreamAsText(response);
if (stringStream != null) {
return stringStream.transform(json.decoder).first.then((jsonResponse) {
if (jsonResponse is Map && jsonResponse['error'] is Map) {
final Map error = jsonResponse['error'];
- final code = error['code'];
- final message = error['message'];
+ final code = error['code'] as int;
+ final message = error['message'] as String;
var errors = <client_requests.ApiRequestErrorDetail>[];
if (error.containsKey('errors') && error['errors'] is List) {
- errors = error['errors']
- .map<client_requests.ApiRequestErrorDetail>((e) =>
- new client_requests.ApiRequestErrorDetail.fromJson(e))
+ errors = (error['errors'] as List)
+ .map((e) =>
+ client_requests.ApiRequestErrorDetail.fromJson(e as Map))
.toList();
}
- throw new client_requests.DetailedApiRequestError(code, message,
+ throw client_requests.DetailedApiRequestError(code, message,
errors: errors);
} else {
throwGeneralError();
@@ -889,7 +889,7 @@
}
}
- return new Future.value(response);
+ return Future.value(response);
}
Stream<String> _decodeStreamAsText(http.StreamedResponse response) {
@@ -897,10 +897,10 @@
// decoder.
// Currently we assume that the api endpoint is responding with json
// encoded in UTF8.
- String contentType = response.headers['content-type'];
+ var contentType = response.headers['content-type'];
if (contentType != null &&
contentType.toLowerCase().startsWith('application/json')) {
- return response.stream.transform(new Utf8Decoder(allowMalformed: true));
+ return response.stream.transform(const Utf8Decoder(allowMalformed: true));
} else {
return null;
}
@@ -911,7 +911,7 @@
Map<String, T> mapMap<F, T>(Map<String, F> source, T convert(F source)) {
assert(source != null);
assert(convert != null);
- final Map<String, T> result = new collection.LinkedHashMap<String, T>();
+ var result = <String, T>{};
source.forEach((String key, F value) {
result[key] = convert(value);
});
diff --git a/_discoveryapis_commons/lib/src/requests.dart b/_discoveryapis_commons/lib/src/requests.dart
index 32b1de6..a47243a 100644
--- a/_discoveryapis_commons/lib/src/requests.dart
+++ b/_discoveryapis_commons/lib/src/requests.dart
@@ -24,13 +24,13 @@
* is used.
*/
Media(this.stream, this.length,
- {this.contentType: "application/octet-stream"}) {
+ {this.contentType = 'application/octet-stream'}) {
if (stream == null || contentType == null) {
- throw new core.ArgumentError(
+ throw core.ArgumentError(
'Arguments stream, contentType and length must not be null.');
}
if (length != null && length < 0) {
- throw new core.ArgumentError('A negative content length is not allowed');
+ throw core.ArgumentError('A negative content length is not allowed');
}
}
}
@@ -40,10 +40,10 @@
*/
class UploadOptions {
/** Use either simple uploads (only media) or multipart for media+metadata */
- static const UploadOptions Default = const UploadOptions();
+ static const UploadOptions Default = UploadOptions();
/** Make resumable uploads */
- static final ResumableUploadOptions Resumable = new ResumableUploadOptions();
+ static final ResumableUploadOptions Resumable = ResumableUploadOptions();
const UploadOptions();
}
@@ -58,7 +58,7 @@
// Wait for 2^(failedAttempts-1) seconds, before retrying.
// i.e. 1 second, 2 seconds, 4 seconds, ...
- return new core.Duration(seconds: 1 << (failedAttempts - 1));
+ return core.Duration(seconds: 1 << (failedAttempts - 1));
};
/**
@@ -81,8 +81,8 @@
final core.Function backoffFunction;
ResumableUploadOptions(
- {this.numberOfAttempts: 3,
- this.chunkSize: 1024 * 1024,
+ {this.numberOfAttempts = 3,
+ this.chunkSize = 1024 * 1024,
core.Function backoffFunction})
: backoffFunction =
backoffFunction == null ? ExponentialBackoff : backoffFunction {
@@ -99,7 +99,7 @@
// to keep the upload efficient.
//
if (numberOfAttempts < 1 || (chunkSize % (256 * 1024)) != 0) {
- throw new core.ArgumentError('Invalid arguments.');
+ throw core.ArgumentError('Invalid arguments.');
}
}
}
@@ -111,11 +111,11 @@
*/
class DownloadOptions {
/** Download only metadata. */
- static const DownloadOptions Metadata = const DownloadOptions();
+ static const DownloadOptions Metadata = DownloadOptions();
/** Download full media. */
static final PartialDownloadOptions FullMedia =
- new PartialDownloadOptions(new ByteRange(0, -1));
+ PartialDownloadOptions(ByteRange(0, -1));
const DownloadOptions();
@@ -156,7 +156,7 @@
ByteRange(this.start, this.end) {
if (!(start == 0 && end == -1 || start >= 0 && end >= start)) {
- throw new core.ArgumentError('Invalid media range [$start, $end]');
+ throw core.ArgumentError('Invalid media range [$start, $end]');
}
}
}
@@ -181,7 +181,7 @@
final core.List<ApiRequestErrorDetail> errors;
DetailedApiRequestError(this.status, core.String message,
- {this.errors: const []})
+ {this.errors = const []})
: super(message);
core.String toString() =>
@@ -237,15 +237,12 @@
this.sendReport})
: originalJson = null;
- ApiRequestErrorDetail.fromJson(core.Map json)
- : originalJson = json,
- domain = json.containsKey('domain') ? json['domain'] : null,
- reason = json.containsKey('reason') ? json['reason'] : null,
- message = json.containsKey('message') ? json['message'] : null,
- location = json.containsKey('location') ? json['location'] : null,
- locationType =
- json.containsKey('locationType') ? json['locationType'] : null,
- extendedHelp =
- json.containsKey('extendedHelp') ? json['extendedHelp'] : null,
- sendReport = json.containsKey('sendReport') ? json['sendReport'] : null;
+ ApiRequestErrorDetail.fromJson(this.originalJson)
+ : domain = originalJson['domain'] as core.String,
+ reason = originalJson['reason'] as core.String,
+ message = originalJson['message'] as core.String,
+ location = originalJson['location'] as core.String,
+ locationType = originalJson['locationType'] as core.String,
+ extendedHelp = originalJson['extendedHelp'] as core.String,
+ sendReport = originalJson['sendReport'] as core.String;
}
diff --git a/_discoveryapis_commons/pubspec.yaml b/_discoveryapis_commons/pubspec.yaml
index fbc9009..6cea540 100644
--- a/_discoveryapis_commons/pubspec.yaml
+++ b/_discoveryapis_commons/pubspec.yaml
@@ -1,11 +1,11 @@
name: _discoveryapis_commons
-version: 0.1.6
+version: 0.1.6+1
author: Dart Team <misc@dartlang.org>
description: Library for use by client APIs generated from Discovery Documents.
homepage: https://github.com/dart-lang/discoveryapis_commons
environment:
- sdk: '>=2.0.0-dev.22.0 <2.0.0'
+ sdk: '>=2.0.0-dev.64.0 <3.0.0'
dependencies:
http: '>=0.11.1 <0.12.0'
dev_dependencies:
- test: '^0.12.0'
+ test: ^1.2.0
diff --git a/ansicolor/.gitignore b/ansicolor/.gitignore
deleted file mode 100644
index 1a90bb8..0000000
--- a/ansicolor/.gitignore
+++ /dev/null
@@ -1,5 +0,0 @@
-packages
-pubspec.lock
-.project
-.children
-*~
\ No newline at end of file
diff --git a/ansicolor/AUTHORS b/ansicolor/AUTHORS
deleted file mode 100644
index c3c748b..0000000
--- a/ansicolor/AUTHORS
+++ /dev/null
@@ -1,4 +0,0 @@
-Authors:
-
-John Thomas McDole <codefu@google.com>
-
diff --git a/ansicolor/BUILD.gn b/ansicolor/BUILD.gn
deleted file mode 100644
index cc0e919..0000000
--- a/ansicolor/BUILD.gn
+++ /dev/null
@@ -1,16 +0,0 @@
-# This file is generated by importer.py for ansicolor-0.0.10
-
-import("//build/dart/dart_library.gni")
-
-dart_library("ansicolor") {
- package_name = "ansicolor"
-
- # This parameter is left empty as we don't care about analysis or exporting
- # these sources outside of the tree.
- sources = []
-
- disable_analysis = true
-
- deps = [
- ]
-}
diff --git a/ansicolor/LICENSE b/ansicolor/LICENSE
deleted file mode 100644
index d645695..0000000
--- a/ansicolor/LICENSE
+++ /dev/null
@@ -1,202 +0,0 @@
-
- Apache License
- Version 2.0, January 2004
- http://www.apache.org/licenses/
-
- TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
-
- 1. Definitions.
-
- "License" shall mean the terms and conditions for use, reproduction,
- and distribution as defined by Sections 1 through 9 of this document.
-
- "Licensor" shall mean the copyright owner or entity authorized by
- the copyright owner that is granting the License.
-
- "Legal Entity" shall mean the union of the acting entity and all
- other entities that control, are controlled by, or are under common
- control with that entity. For the purposes of this definition,
- "control" means (i) the power, direct or indirect, to cause the
- direction or management of such entity, whether by contract or
- otherwise, or (ii) ownership of fifty percent (50%) or more of the
- outstanding shares, or (iii) beneficial ownership of such entity.
-
- "You" (or "Your") shall mean an individual or Legal Entity
- exercising permissions granted by this License.
-
- "Source" form shall mean the preferred form for making modifications,
- including but not limited to software source code, documentation
- source, and configuration files.
-
- "Object" form shall mean any form resulting from mechanical
- transformation or translation of a Source form, including but
- not limited to compiled object code, generated documentation,
- and conversions to other media types.
-
- "Work" shall mean the work of authorship, whether in Source or
- Object form, made available under the License, as indicated by a
- copyright notice that is included in or attached to the work
- (an example is provided in the Appendix below).
-
- "Derivative Works" shall mean any work, whether in Source or Object
- form, that is based on (or derived from) the Work and for which the
- editorial revisions, annotations, elaborations, or other modifications
- represent, as a whole, an original work of authorship. For the purposes
- of this License, Derivative Works shall not include works that remain
- separable from, or merely link (or bind by name) to the interfaces of,
- the Work and Derivative Works thereof.
-
- "Contribution" shall mean any work of authorship, including
- the original version of the Work and any modifications or additions
- to that Work or Derivative Works thereof, that is intentionally
- submitted to Licensor for inclusion in the Work by the copyright owner
- or by an individual or Legal Entity authorized to submit on behalf of
- the copyright owner. For the purposes of this definition, "submitted"
- means any form of electronic, verbal, or written communication sent
- to the Licensor or its representatives, including but not limited to
- communication on electronic mailing lists, source code control systems,
- and issue tracking systems that are managed by, or on behalf of, the
- Licensor for the purpose of discussing and improving the Work, but
- excluding communication that is conspicuously marked or otherwise
- designated in writing by the copyright owner as "Not a Contribution."
-
- "Contributor" shall mean Licensor and any individual or Legal Entity
- on behalf of whom a Contribution has been received by Licensor and
- subsequently incorporated within the Work.
-
- 2. Grant of Copyright License. Subject to the terms and conditions of
- this License, each Contributor hereby grants to You a perpetual,
- worldwide, non-exclusive, no-charge, royalty-free, irrevocable
- copyright license to reproduce, prepare Derivative Works of,
- publicly display, publicly perform, sublicense, and distribute the
- Work and such Derivative Works in Source or Object form.
-
- 3. Grant of Patent License. Subject to the terms and conditions of
- this License, each Contributor hereby grants to You a perpetual,
- worldwide, non-exclusive, no-charge, royalty-free, irrevocable
- (except as stated in this section) patent license to make, have made,
- use, offer to sell, sell, import, and otherwise transfer the Work,
- where such license applies only to those patent claims licensable
- by such Contributor that are necessarily infringed by their
- Contribution(s) alone or by combination of their Contribution(s)
- with the Work to which such Contribution(s) was submitted. If You
- institute patent litigation against any entity (including a
- cross-claim or counterclaim in a lawsuit) alleging that the Work
- or a Contribution incorporated within the Work constitutes direct
- or contributory patent infringement, then any patent licenses
- granted to You under this License for that Work shall terminate
- as of the date such litigation is filed.
-
- 4. Redistribution. You may reproduce and distribute copies of the
- Work or Derivative Works thereof in any medium, with or without
- modifications, and in Source or Object form, provided that You
- meet the following conditions:
-
- (a) You must give any other recipients of the Work or
- Derivative Works a copy of this License; and
-
- (b) You must cause any modified files to carry prominent notices
- stating that You changed the files; and
-
- (c) You must retain, in the Source form of any Derivative Works
- that You distribute, all copyright, patent, trademark, and
- attribution notices from the Source form of the Work,
- excluding those notices that do not pertain to any part of
- the Derivative Works; and
-
- (d) If the Work includes a "NOTICE" text file as part of its
- distribution, then any Derivative Works that You distribute must
- include a readable copy of the attribution notices contained
- within such NOTICE file, excluding those notices that do not
- pertain to any part of the Derivative Works, in at least one
- of the following places: within a NOTICE text file distributed
- as part of the Derivative Works; within the Source form or
- documentation, if provided along with the Derivative Works; or,
- within a display generated by the Derivative Works, if and
- wherever such third-party notices normally appear. The contents
- of the NOTICE file are for informational purposes only and
- do not modify the License. You may add Your own attribution
- notices within Derivative Works that You distribute, alongside
- or as an addendum to the NOTICE text from the Work, provided
- that such additional attribution notices cannot be construed
- as modifying the License.
-
- You may add Your own copyright statement to Your modifications and
- may provide additional or different license terms and conditions
- for use, reproduction, or distribution of Your modifications, or
- for any such Derivative Works as a whole, provided Your use,
- reproduction, and distribution of the Work otherwise complies with
- the conditions stated in this License.
-
- 5. Submission of Contributions. Unless You explicitly state otherwise,
- any Contribution intentionally submitted for inclusion in the Work
- by You to the Licensor shall be under the terms and conditions of
- this License, without any additional terms or conditions.
- Notwithstanding the above, nothing herein shall supersede or modify
- the terms of any separate license agreement you may have executed
- with Licensor regarding such Contributions.
-
- 6. Trademarks. This License does not grant permission to use the trade
- names, trademarks, service marks, or product names of the Licensor,
- except as required for reasonable and customary use in describing the
- origin of the Work and reproducing the content of the NOTICE file.
-
- 7. Disclaimer of Warranty. Unless required by applicable law or
- agreed to in writing, Licensor provides the Work (and each
- Contributor provides its Contributions) on an "AS IS" BASIS,
- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
- implied, including, without limitation, any warranties or conditions
- of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
- PARTICULAR PURPOSE. You are solely responsible for determining the
- appropriateness of using or redistributing the Work and assume any
- risks associated with Your exercise of permissions under this License.
-
- 8. Limitation of Liability. In no event and under no legal theory,
- whether in tort (including negligence), contract, or otherwise,
- unless required by applicable law (such as deliberate and grossly
- negligent acts) or agreed to in writing, shall any Contributor be
- liable to You for damages, including any direct, indirect, special,
- incidental, or consequential damages of any character arising as a
- result of this License or out of the use or inability to use the
- Work (including but not limited to damages for loss of goodwill,
- work stoppage, computer failure or malfunction, or any and all
- other commercial damages or losses), even if such Contributor
- has been advised of the possibility of such damages.
-
- 9. Accepting Warranty or Additional Liability. While redistributing
- the Work or Derivative Works thereof, You may choose to offer,
- and charge a fee for, acceptance of support, warranty, indemnity,
- or other liability obligations and/or rights consistent with this
- License. However, in accepting such obligations, You may act only
- on Your own behalf and on Your sole responsibility, not on behalf
- of any other Contributor, and only if You agree to indemnify,
- defend, and hold each Contributor harmless for any liability
- incurred by, or claims asserted against, such Contributor by reason
- of your accepting any such warranty or additional liability.
-
- END OF TERMS AND CONDITIONS
-
- APPENDIX: How to apply the Apache License to your work.
-
- To apply the Apache License to your work, attach the following
- boilerplate notice, with the fields enclosed by brackets "[]"
- replaced with your own identifying information. (Don't include
- the brackets!) The text should be enclosed in the appropriate
- comment syntax for the file format. We also recommend that a
- file or class name and description of purpose be included on the
- same "printed page" as the copyright notice for easier
- identification within third-party archives.
-
- Copyright [yyyy] [name of copyright owner]
-
- Licensed under the Apache License, Version 2.0 (the "License");
- you may not use this file except in compliance with the License.
- You may obtain a copy of the License at
-
- http://www.apache.org/licenses/LICENSE-2.0
-
- Unless required by applicable law or agreed to in writing, software
- distributed under the License is distributed on an "AS IS" BASIS,
- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
- See the License for the specific language governing permissions and
- limitations under the License.
diff --git a/ansicolor/README.md b/ansicolor/README.md
deleted file mode 100644
index de45e99..0000000
--- a/ansicolor/README.md
+++ /dev/null
@@ -1,31 +0,0 @@
-ANSI / Xterm 256 color library for Dart
-------
-
-Feel like you're missing some color in your terminal programs? Use AnsiPen to add ANSI color codes to your log messages.
-
-Easy to disable for production, just set `color_disabled = true` and all codes will be empty - no re-writing debug messages.
-
-Note: `color_disabled` is a global variable for all pen colors.
-
-Example
-------
-Note: Be mindful of contrasting colors. If you set "bright white" foreground and don't adjust the background, you'll have a bad time with lighter terminals.
-
-Foreground to bright white with default background:
-```dart
-AnsiPen pen = new AnsiPen()..white(bold: true);
-print(pen("Bright white foreground") + " this text is default fg/bg");
-```
-
-Background as a peach, foreground as white:
-```dart
-AnsiPen pen = new AnsiPen()..white()..rgb(r: 1.0, g: 0.8, b: 0.2);
-print(pen("White foreground with a peach background"));
-```
-
-Rainbow Demo
-------
-
-If you want a specific color, you can call the `xterm()` with the index listed in the rainbow below. To show the rainbow on your own terminal, just call `print(ansi_demo());` or run src/demo.dart
-
-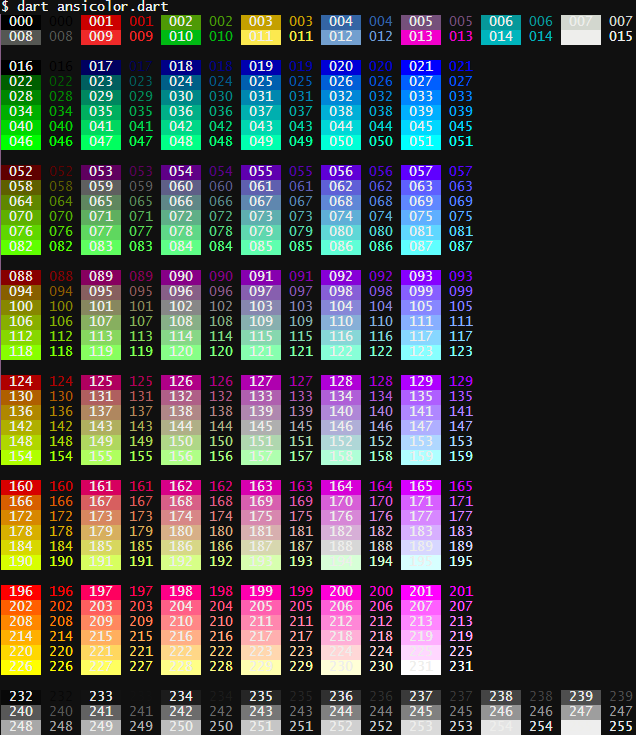
diff --git a/ansicolor/bin/demo.dart b/ansicolor/bin/demo.dart
deleted file mode 100644
index 52c347d..0000000
--- a/ansicolor/bin/demo.dart
+++ /dev/null
@@ -1,68 +0,0 @@
-import 'package:ansicolor/ansicolor.dart';
-
-main() {
- print(ansi_demo());
-}
-
-/// Due to missing sprintf(), this is my cheap "%03d".
-String _toSpace(int i, [int width = 3]) {
- if (width <= 0 && i == 0) return "";
- return "${_toSpace(i ~/ 10, --width)}${i % 10}";
-}
-
-/// Return a reference table for foreground and background colors.
-String ansi_demo() {
- StringBuffer sb = new StringBuffer();
-
- AnsiPen pen = new AnsiPen();
-
- for (int c = 0; c < 16; c++) {
- pen
- ..reset()
- ..white(bold: true)
- ..xterm(c, bg: true);
- sb.write(pen("${_toSpace(c)} "));
- pen
- ..reset()
- ..xterm(c);
- sb.write(pen(" ${_toSpace(c)} "));
- if (c == 7 || c == 15) {
- sb.write("\n");
- }
- }
-
- for (int r = 0; r < 6; r++) {
- sb.write("\n");
- for (int g = 0; g < 6; g++) {
- for (int b = 0; b < 6; b++) {
- var c = r * 36 + g * 6 + b + 16;
- pen
- ..reset()
- ..rgb(r: r / 5, g: g / 5, b: b / 5, bg: true)
- ..white(bold: true);
- sb.write(pen(" ${_toSpace(c)} "));
- pen
- ..reset()
- ..rgb(r: r / 5, g: g / 5, b: b / 5);
- sb.write(pen(" ${_toSpace(c)} "));
- }
- sb.write("\n");
- }
- }
-
- for (int c = 0; c < 24; c++) {
- if (0 == c % 8) {
- sb.write("\n");
- }
- pen
- ..reset()
- ..gray(level: c / 23, bg: true)
- ..white(bold: true);
- sb.write(pen(" ${_toSpace(c + 232)} "));
- pen
- ..reset()
- ..gray(level: c / 23);
- sb.write(pen(" ${_toSpace(c + 232)} "));
- }
- return sb.toString();
-}
diff --git a/ansicolor/lib/ansicolor.dart b/ansicolor/lib/ansicolor.dart
deleted file mode 100644
index 481db0f..0000000
--- a/ansicolor/lib/ansicolor.dart
+++ /dev/null
@@ -1,114 +0,0 @@
-///
-/// Copyright 2013 Google Inc. All Rights Reserved.
-///
-/// ANSI/XTERM SGR (Select Graphics Rendering) support for 256 colors.
-/// Note: if you're using the dart editor, these won't look right in the
-/// terminal; disable via [color_disabled] or use Eclipse with the Dart and
-/// AnsiConsol plugins!
-///
-library ansicolor;
-
-/// Globally enable or disable [AnsiPen] settings
-///
-/// Handy for turning on and off embedded colors without commenting out code.
-bool color_disabled = false;
-
-/// Pen attributes for foreground and background colors.
-///
-/// Use the pen in string interpolation to output ansi codes.
-/// Use [up] in string interpolation to globally reset colors.
-class AnsiPen {
- /// Treat a pen instance as a function such that pen("msg") is the same as
- /// pen.write("msg").
- call(String msg) => write(msg);
-
- /// Allow pen colors to be used in a string.
- ///
- /// Note: Once the pen is down, its attributes remain in effect till they are
- /// changed by another pen or [up].
- String toString() {
- if (color_disabled) return "";
- if (_pen != null) return _pen;
-
- StringBuffer sb = new StringBuffer();
- if (_fcolor != null) {
- sb.write("${ANSI_ESC}38;5;${_fcolor}m");
- }
-
- if (_bcolor != null) {
- sb.write("${ANSI_ESC}48;5;${_bcolor}m");
- }
-
- _pen = sb.toString();
- return _pen;
- }
-
- /// Returns control codes to change the terminal colors.
- String get down => this.toString();
-
- /// Resets all pen attributes in the terminal.
- String get up => color_disabled ? "" : ANSI_DEFAULT;
-
- /// Write the [msg] with the pen's current settings and then reset all
- /// attributes.
- String write(String msg) => "${this}$msg$up";
-
- void black({bool bg: false, bool bold: false}) => _std(0, bold, bg);
- void red({bool bg: false, bool bold: false}) => _std(1, bold, bg);
- void green({bool bg: false, bool bold: false}) => _std(2, bold, bg);
- void yellow({bool bg: false, bool bold: false}) => _std(3, bold, bg);
- void blue({bool bg: false, bool bold: false}) => _std(4, bold, bg);
- void magenta({bool bg: false, bool bold: false}) => _std(5, bold, bg);
- void cyan({bool bg: false, bool bold: false}) => _std(6, bold, bg);
- void white({bool bg: false, bool bold: false}) => _std(7, bold, bg);
-
- /// Sets the pen color to the rgb value between 0.0..1.0.
- void rgb({r: 1.0, g: 1.0, b: 1.0, bool bg: false}) => xterm(
- (r.clamp(0.0, 1.0) * 5).toInt() * 36 +
- (g.clamp(0.0, 1.0) * 5).toInt() * 6 +
- (b.clamp(0.0, 1.0) * 5).toInt() +
- 16,
- bg: bg);
-
- /// Sets the pen color to a grey scale value between 0.0 and 1.0.
- void gray({level: 1.0, bool bg: false}) =>
- xterm(232 + (level.clamp(0.0, 1.0) * 23).round(), bg: bg);
-
- void _std(int color, bool bold, bool bg) =>
- xterm(color + (bold ? 8 : 0), bg: bg);
-
- /// Directly index the xterm 256 color palette.
- void xterm(int color, {bool bg: false}) {
- _pen = null;
- var c = color.toInt().clamp(0, 256);
- if (bg) {
- _bcolor = c;
- } else {
- _fcolor = c;
- }
- }
-
- ///Resets the pen's attributes.
- void reset() {
- _pen = null;
- _bcolor = _fcolor = null;
- }
-
- int _fcolor;
- int _bcolor;
- String _pen;
-}
-
-/// ANSI Control Sequence Introducer, signals the terminal for new settings.
-String get ANSI_ESC => color_disabled ? "" : '\x1B[';
-
-/// Reset all colors and options for current SGRs to terminal defaults.
-String get ANSI_DEFAULT => color_disabled ? "" : "${ANSI_ESC}0m";
-
-/// Defaults the terminal's foreground color without altering the background.
-/// Does not modify [AnsiPen]!
-String resetForeground() => "${ANSI_ESC}39m";
-
-/// Defaults the terminal's background color without altering the foreground.
-/// Does not modify [AnsiPen]!
-String resetBackground() => "${ANSI_ESC}49m";
diff --git a/ansicolor/pubspec.yaml b/ansicolor/pubspec.yaml
deleted file mode 100644
index 2ee4408..0000000
--- a/ansicolor/pubspec.yaml
+++ /dev/null
@@ -1,9 +0,0 @@
-name: ansicolor
-version: 0.0.10
-author: John Thomas McDole <codefu@google.com>
-description: An xterm 256 color support library
-homepage: https://github.com/google/ansicolor-dart
-environment:
- sdk: '<2.0.0'
-dev_dependencies:
- test: ">=0.12.18"
diff --git a/ansicolor/src/demo.dart b/ansicolor/src/demo.dart
deleted file mode 100644
index 7a7c45d..0000000
--- a/ansicolor/src/demo.dart
+++ /dev/null
@@ -1,5 +0,0 @@
-import "../lib/ansicolor.dart";
-
-void main() {
- print(ansi_demo());
-}
diff --git a/coverage/.gitignore b/coverage/.gitignore
index dc04f09..e540fed 100644
--- a/coverage/.gitignore
+++ b/coverage/.gitignore
@@ -2,6 +2,7 @@
packages
pubspec.lock
build
+.dart_tool/
.pub
.packages
diff --git a/coverage/BUILD.gn b/coverage/BUILD.gn
index 57843b4..45c26ef 100644
--- a/coverage/BUILD.gn
+++ b/coverage/BUILD.gn
@@ -1,4 +1,4 @@
-# This file is generated by importer.py for coverage-0.11.0
+# This file is generated by importer.py for coverage-0.12.1
import("//build/dart/dart_library.gni")
diff --git a/coverage/CHANGELOG.md b/coverage/CHANGELOG.md
index e064eed..84f4982 100644
--- a/coverage/CHANGELOG.md
+++ b/coverage/CHANGELOG.md
@@ -1,3 +1,13 @@
+## 0.12.1 - 2018-06-26
+
+ * Minor type, dartfmt fixes.
+ * Require package:args >= 1.4.0.
+
+## 0.12.0 - 2018-06-26
+
+ * BREAKING CHANGE: This version requires Dart SDK 2.0.0-dev.64.1 or later.
+ * Strong mode fixes as of Dart SDK 2.0.0-dev.64.1.
+
## 0.11.0 - 2018-04-12
* BREAKING CHANGE: This version requires Dart SDK 2.0.0-dev.30 or later.
diff --git a/coverage/bin/format_coverage.dart b/coverage/bin/format_coverage.dart
index 327ed83..ef1045d 100644
--- a/coverage/bin/format_coverage.dart
+++ b/coverage/bin/format_coverage.dart
@@ -99,8 +99,7 @@
parser.addOption('in', abbr: 'i', help: 'input(s): may be file or directory');
parser.addOption('out',
abbr: 'o', defaultsTo: 'stdout', help: 'output: may be file or stdout');
- parser.addOption('report-on',
- allowMultiple: true,
+ parser.addMultiOption('report-on',
help: 'which directories or files to report coverage on');
parser.addOption('workers',
abbr: 'j', defaultsTo: '1', help: 'number of workers');
diff --git a/coverage/coverage.json b/coverage/coverage.json
new file mode 100644
index 0000000..28fdc1b
--- /dev/null
+++ b/coverage/coverage.json
@@ -0,0 +1 @@
+{"type":"CodeCoverage","coverage":[{"source":"dart:core/runtime/libtype_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibtype_patch.dart","uri":"dart:core/runtime/libtype_patch.dart","_kind":"library"},"hits":[11,0,16,0]},{"source":"dart:core/errors.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Ferrors.dart","uri":"dart:core/errors.dart","_kind":"library"},"hits":[514,0,515,0,205,0,217,0,237,0,264,0,266,0,267,0,282,0,284,0,286,0,288,0,308,1,312,2,317,2,314,0,319,0,331,0,332,0,335,0,336,0,339,0,340,0,341,0,344,0,345,0,346,0,347,0,348,0,352,0,380,0,383,0,388,0,389,0,391,0,392,0,394,0,397,0,400,0,572,0,424,0,529,0,531,0,532,0,536,0,580,0,581,0,584,0,585,0,588,0,589,0,592,0,593,0,602,0,603,0,604,0,607,0,608,0,609,0,617,0,618,0,622,0,623,0,627,0,628,0,638,0,639,0,640,0,643,0,644,0,645,0,648,0,649,0,650,0,653,0,654,0,655,0,658,0,659,0,660,0,663,0,664,0,665,0,673,0,674,0,675,0,684,0,685,0,686,0,689,0,690,0,691,0,694,0,695,0,696,0,699,0,700,0,701,0,704,0,705,0,706,0,709,0,710,0,711,0,714,0,715,0,716,0,719,0,720,0,721,0,724,0,725,0,726,0,729,0,730,0,731,0,739,0,740,0,748,0,749,0,758,0,759,0,760,0,484,0,485,0,548,0,549,0,551,0,142,0,160,0,167,0,173,0,174,0,176,0,178,0,179,0,181,0,182,0,183,0,185,0,186,0,187,0,500,0,501,0,502,0,503,0,69,0,77,0,78,0,79,0,81,0,82,0,84,0,413,0,101,0,102,0,563,0,564,0,566,0,119,0,541,0,542,0,544,0]},{"source":"dart:core/runtime/libimmutable_map.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibimmutable_map.dart","uri":"dart:core/runtime/libimmutable_map.dart","_kind":"library"},"hits":[182,0,184,0,185,0,186,0,187,0,188,0,191,0,195,0,124,0,126,0,127,0,130,0,135,0,137,0,138,0,141,0,13,0,16,0,17,0,21,0,22,0,23,0,29,0,30,0,33,0,35,0,36,0,39,0,40,0,41,0,45,0,46,0,49,0,50,0,53,0,54,0,55,0,62,0,63,0,64,0,71,0,72,0,75,0,76,0,79,0,80,0,83,0,84,0,87,0,88,0,91,0,92,0,94,0,95,0,96,0,97,0,98,0,103,0,104,0,107,0,108,0,111,0,112,0,115,0,116,0,119,0,203,0,205,0,206,0,207,0,208,0,209,0,210,0,213,0,217,0,161,0,163,0,164,0,165,0,166,0,167,0,170,0,174,0,147,0,149,0,150,0,153,0]},{"source":"dart:core/runtime/libregexp_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibregexp_patch.dart","uri":"dart:core/runtime/libregexp_patch.dart","_kind":"library"},"hits":[46,0,63,0,64,0,65,0,67,0,10,0,12,0,13,0,16,0,17,0,18,0,19,0,22,0,23,0,26,0,28,0,33,0,37,0,71,0,72,0,74,0,76,0,81,0,83,0,85,0,86,0,88,0,89,0,137,0,139,0,140,0,142,0,143,0,146,0,147,0,150,0,151,0,152,0,154,0,155,0,156,0,160,0,163,0,164,0,167,0,168,0,169,0,170,0,175,0,177,0,133,0,186,0,190,0,191,0,192,0,196,0,199,0,200,0,201,0,202,0,203,0,205,0,208,0,209,0,210,0,211,0,212,0,214,0,216,0,219,0,220,0,221,0,225,0,226,0,227,0,231,0,234,0,236,0,238,0,240,0,287,0,289,0,309,0,311,0,313,0,314,0,315,0,316,0,318,0,319,0,320,0,322,0,327,0,328,0,298,0,300,0,116,0,118,0,119,0,120,0,121,0,122,0,123,0]},{"source":"dart:core/runtime/libbool_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibbool_patch.dart","uri":"dart:core/runtime/libbool_patch.dart","_kind":"library"},"hits":[16,0,10,0,14,0]},{"source":"dart:core/bool.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fbool.dart","uri":"dart:core/bool.dart","_kind":"library"},"hits":[56,0]},{"source":"dart:core/runtime/libbigint_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibbigint_patch.dart","uri":"dart:core/runtime/libbigint_patch.dart","_kind":"library"},"hits":[2526,0,2527,0,2528,0,2530,0,2531,0,2532,0,2533,0,2534,0,2537,0,2538,0,2539,0,2554,0,2555,0,2556,0,2557,0,2558,0,2559,0,2560,0,2562,0,2563,0,2571,0,2572,0,2573,0,2574,0,2575,0,2576,0,2577,0,2578,0,2579,0,2581,0,2582,0,2583,0,2585,0,2586,0,2587,0,2588,0,2599,0,2600,0,2601,0,2602,0,2603,0,2604,0,2605,0,2606,0,2613,0,2617,0,2618,0,2619,0,2621,0,2622,0,2623,0,2624,0,2625,0,2630,0,2632,0,2633,0,2634,0,2635,0,2637,0,2638,0,2643,0,2644,0,2646,0,2649,0,2650,0,2652,0,2653,0,2655,0,2658,0,2659,0,2661,0,2662,0,2663,0,2665,0,2666,0,2669,0,2670,0,2675,0,2676,0,2677,0,2680,0,2683,0,2684,0,325,0,326,0,328,0,329,0,157,0,158,0,160,0,168,0,172,0,176,0,177,0,178,0,179,0,180,0,181,0,186,0,198,0,206,0,207,0,208,0,218,0,219,0,220,0,221,0,222,0,224,0,225,0,228,0,229,0,230,0,231,0,233,0,235,0,237,0,238,0,239,0,240,0,242,0,244,0,245,0,252,0,253,0,254,0,255,0,256,0,257,0,258,0,260,0,269,0,270,0,272,0,274,0,281,0,283,0,284,0,285,0,290,0,294,0,299,0,300,0,302,0,303,0,305,0,306,0,308,0,309,0,312,0,317,0,318,0,335,0,340,0,342,0,343,0,344,0,345,0,351,0,352,0,353,0,354,0,356,0,357,0,358,0,359,0,362,0,363,0,365,0,369,0,370,0,371,0,373,0,375,0,376,0,377,0,379,0,380,0,381,0,388,0,391,0,392,0,394,0,395,0,397,0,398,0,400,0,401,0,402,0,404,0,406,0,407,0,411,0,412,0,413,0,415,0,416,0,418,0,420,0,421,0,422,0,423,0,425,0,435,0,436,0,437,0,445,0,448,0,449,0,450,0,451,0,453,0,454,0,455,0,456,0,457,0,459,0,467,0,469,0,472,0,475,0,477,0,478,0,480,0,481,0,483,0,484,0,490,0,491,0,492,0,493,0,495,0,496,0,497,0,499,0,500,0,501,0,502,0,504,0,505,0,507,0,508,0,509,0,521,0,523,0,524,0,528,0,529,0,531,0,532,0,545,0,548,0,549,0,550,0,551,0,553,0,554,0,555,0,556,0,558,0,573,0,574,0,575,0,577,0,578,0,579,0,580,0,581,0,584,0,585,0,586,0,587,0,592,0,594,0,595,0,596,0,597,0,600,0,602,0,604,0,605,0,607,0,608,0,609,0,610,0,618,0,621,0,622,0,623,0,624,0,625,0,626,0,627,0,628,0,629,0,630,0,632,0,644,0,645,0,646,0,648,0,649,0,650,0,651,0,652,0,654,0,655,0,656,0,657,0,659,0,660,0,661,0,662,0,663,0,665,0,666,0,668,0,669,0,670,0,679,0,681,0,682,0,683,0,684,0,686,0,687,0,691,0,692,0,693,0,694,0,695,0,704,0,706,0,715,0,717,0,718,0,720,0,722,0,729,0,731,0,732,0,733,0,734,0,735,0,745,0,749,0,750,0,751,0,752,0,754,0,755,0,756,0,757,0,759,0,766,0,770,0,771,0,772,0,773,0,775,0,776,0,777,0,778,0,783,0,784,0,785,0,786,0,787,0,789,0,791,0,793,0,794,0,796,0,797,0,798,0,799,0,805,0,807,0,808,0,810,0,812,0,813,0,814,0,816,0,817,0,818,0,822,0,823,0,824,0,825,0,826,0,827,0,828,0,830,0,834,0,835,0,836,0,837,0,838,0,839,0,840,0,841,0,843,0,844,0,846,0,850,0,851,0,852,0,853,0,854,0,855,0,856,0,858,0,865,0,866,0,868,0,869,0,870,0,872,0,876,0,877,0,878,0,879,0,880,0,881,0,882,0,884,0,891,0,892,0,894,0,895,0,896,0,898,0,911,0,913,0,914,0,915,0,919,0,920,0,922,0,924,0,928,0,937,0,938,0,951,0,953,0,954,0,955,0,956,0,960,0,961,0,963,0,965,0,969,0,978,0,980,0,993,0,995,0,996,0,997,0,998,0,1000,0,1001,0,1002,0,1004,0,1008,0,1017,0,1019,0,1030,0,1031,0,1032,0,1034,0,1038,0,1042,0,1044,0,1045,0,1046,0,1047,0,1050,0,1054,0,1055,0,1057,0,1061,0,1063,0,1064,0,1065,0,1066,0,1069,0,1073,0,1074,0,1076,0,1094,0,1102,0,1103,0,1108,0,1109,0,1110,0,1111,0,1112,0,1113,0,1114,0,1115,0,1116,0,1118,0,1119,0,1121,0,1122,0,1123,0,1124,0,1144,0,1146,0,1147,0,1148,0,1150,0,1151,0,1152,0,1153,0,1154,0,1155,0,1156,0,1157,0,1158,0,1159,0,1160,0,1161,0,1162,0,1163,0,1164,0,1165,0,1166,0,1167,0,1168,0,1169,0,1170,0,1172,0,1173,0,1175,0,1176,0,1177,0,1178,0,1180,0,1186,0,1188,0,1189,0,1190,0,1191,0,1193,0,1194,0,1195,0,1196,0,1198,0,1199,0,1201,0,1202,0,1208,0,1210,0,1211,0,1213,0,1214,0,1217,0,1218,0,1225,0,1227,0,1232,0,1233,0,1236,0,1237,0,1240,0,1241,0,1264,0,1267,0,1268,0,1272,0,1273,0,1274,0,1275,0,1277,0,1284,0,1287,0,1288,0,1290,0,1293,0,1294,0,1296,0,1297,0,1298,0,1304,0,1307,0,1310,0,1314,0,1315,0,1316,0,1317,0,1319,0,1320,0,1333,0,1335,0,1336,0,1337,0,1338,0,1343,0,1345,0,1346,0,1358,0,1360,0,1361,0,1362,0,1363,0,1364,0,1366,0,1367,0,1369,0,1370,0,1372,0,1373,0,1374,0,1377,0,1378,0,1380,0,1381,0,1384,0,1386,0,1388,0,1391,0,1393,0,1394,0,1397,0,1398,0,1399,0,1405,0,1406,0,1407,0,1408,0,1410,0,1414,0,1415,0,1417,0,1418,0,1419,0,1420,0,1426,0,1427,0,1429,0,1430,0,1431,0,1432,0,1434,0,1436,0,1437,0,1438,0,1439,0,1440,0,1442,0,1447,0,1450,0,1451,0,1452,0,1453,0,1473,0,1484,0,1487,0,1488,0,1489,0,1492,0,1494,0,1496,0,1499,0,1501,0,1502,0,1511,0,1512,0,1513,0,1514,0,1516,0,1520,0,1521,0,1522,0,1523,0,1524,0,1525,0,1531,0,1532,0,1533,0,1534,0,1535,0,1536,0,1538,0,1540,0,1541,0,1542,0,1543,0,1544,0,1546,0,1551,0,1555,0,1557,0,1562,0,1580,0,1581,0,1582,0,1583,0,1585,0,1596,0,1597,0,1622,0,1623,0,1624,0,1625,0,1647,0,1649,0,1652,0,1662,0,1664,0,1667,0,1671,0,1674,0,1677,0,1680,0,1683,0,1696,0,1698,0,1701,0,1702,0,1703,0,1704,0,1706,0,1718,0,1719,0,1720,0,1724,0,1727,0,1730,0,1732,0,1733,0,1734,0,1736,0,1739,0,1741,0,1742,0,1743,0,1745,0,1747,0,1748,0,1760,0,1763,0,1764,0,1766,0,1767,0,1769,0,1771,0,1772,0,1773,0,1774,0,1775,0,1776,0,1777,0,1778,0,1779,0,1780,0,1781,0,1784,0,1785,0,1789,0,1790,0,1791,0,1793,0,1804,0,1807,0,1809,0,1811,0,1813,0,1817,0,1819,0,1820,0,1821,0,1822,0,1823,0,1824,0,1825,0,1826,0,1827,0,1828,0,1829,0,1830,0,1831,0,1832,0,1837,0,1838,0,1839,0,1841,0,1842,0,1843,0,1844,0,1845,0,1846,0,1848,0,1849,0,1850,0,1854,0,1855,0,1856,0,1858,0,1859,0,1860,0,1864,0,1865,0,1866,0,1867,0,1868,0,1869,0,1873,0,1874,0,1875,0,1876,0,1878,0,1879,0,1888,0,1889,0,1891,0,1892,0,1899,0,1900,0,1901,0,1906,0,1912,0,1913,0,1914,0,1915,0,1916,0,1917,0,1918,0,1919,0,1920,0,1923,0,1924,0,1925,0,1928,0,1929,0,1931,0,1932,0,1934,0,1935,0,1936,0,1937,0,1938,0,1939,0,1941,0,1942,0,1943,0,1944,0,1945,0,1947,0,1957,0,1958,0,1959,0,1962,0,1963,0,1967,0,1969,0,1970,0,1973,0,1975,0,1977,0,1980,0,1981,0,1983,0,1985,0,1986,0,1987,0,1989,0,1993,0,1996,0,1997,0,1998,0,1999,0,2001,0,2005,0,2006,0,2008,0,2009,0,2010,0,2011,0,2013,0,2017,0,2019,0,2020,0,2022,0,2024,0,2025,0,2026,0,2028,0,2032,0,2035,0,2036,0,2037,0,2038,0,2040,0,2044,0,2045,0,2047,0,2048,0,2049,0,2050,0,2052,0,2056,0,2058,0,2059,0,2061,0,2062,0,2063,0,2064,0,2066,0,2067,0,2070,0,2073,0,2074,0,2075,0,2076,0,2078,0,2079,0,2082,0,2085,0,2087,0,2088,0,2089,0,2090,0,2092,0,2093,0,2096,0,2099,0,2100,0,2101,0,2102,0,2104,0,2105,0,2108,0,2113,0,2114,0,2117,0,2118,0,2120,0,2123,0,2124,0,2125,0,2126,0,2130,0,2131,0,2132,0,2133,0,2134,0,2135,0,2137,0,2141,0,2144,0,2145,0,2146,0,2147,0,2148,0,2149,0,2152,0,2164,0,2166,0,2167,0,2169,0,2171,0,2172,0,2174,0,2190,0,2192,0,2193,0,2194,0,2223,0,2224,0,2261,0,2267,0,2268,0,2271,0,2273,0,2274,0,2275,0,2276,0,2279,0,2281,0,2282,0,2283,0,2284,0,2285,0,2287,0,2297,0,2304,0,2307,0,2309,0,2310,0,2313,0,2316,0,2317,0,2318,0,2322,0,2349,0,2350,0,2352,0,2354,0,2370,0,2371,0,2373,0,2376,0,2378,0,2380,0,2381,0,2382,0,2389,0,2399,0,2400,0,2401,0,2402,0,2403,0,2408,0,2409,0,2410,0,2411,0,2412,0,2413,0,2414,0,2415,0,2417,0,2418,0,2419,0,2421,0,2422,0,2423,0,2425,0,2426,0,2429,0,2431,0,2432,0,2433,0,2436,0,2439,0,2440,0,2451,0,2452,0,2454,0,2456,0,2457,0,2458,0,2462,0,2464,0,2465,0,2466,0,2467,0,2468,0,2469,0,2470,0,2472,0,2473,0,2477,0,2478,0,2479,0,2480,0,2481,0,2482,0,2483,0,2486,0,2487,0,2488,0,2489,0,2491,0,2493,0,2495,0,2700,0,2703,0,2706,0,2707,0,2709,0,2710,0,2711,0,2712,0,2714,0,2715,0,2716,0,2717,0,2718,0,2722,0,2723,0,2724,0,2725,0,2727,0,2732,0,2735,0,2738,0,2739,0,2740,0,2742,0,2745,0,2746,0,2748,0,2749,0,2751,0,2752,0,2753,0,2758,0,2759,0,2762,0,2763,0,2769,0,2772,0,2773,0,2774,0,2775,0,2776,0,2777,0,2781,0,2782,0,2783,0,2786,0,2789,0,2790,0,46,0,48,0,50,0,53,0,54,0,57,0,58,0,61,0,64,0,65,0,68,0,1568,0,1569,0,1570,0,1571,0,1574,0,1575,0,1576,0,1577,0,2323,0,2325,0,2328,0,2330,0,2332,0,2333,0,2334,0,2336,0,2337,0,2340,0,2342,0,2343,0,2357,0,2362,0,2363,0,2364,0,2365,0,2366,0]},{"source":"dart:core/annotations.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fannotations.dart","uri":"dart:core/annotations.dart","_kind":"library"},"hits":[154,0,165,0,70,0,72,0,268,0,81,0]},{"source":"dart:core/uri.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Furi.dart","uri":"dart:core/uri.dart","_kind":"library"},"hits":[4634,0,4637,0,1432,1,1440,0,1453,0,1454,0,1455,0,1456,0,1462,0,1463,0,1464,0,1465,0,1467,0,1468,0,1470,0,1473,0,1477,0,1479,0,1480,0,1483,0,1484,0,1486,0,1491,1,1501,2,1502,2,1503,2,1505,1,1506,2,1507,2,1508,1,1509,1,1510,1,1514,1,1515,1,1516,1,1520,1,1525,1,1517,0,1518,0,1522,0,1530,0,1532,0,1536,0,1538,0,1541,0,1542,0,1543,0,1544,0,1545,0,1548,0,1550,0,1551,0,1552,0,1553,0,1555,0,1558,0,1559,0,1560,0,1564,0,1565,0,1566,0,1570,0,1572,0,1574,0,1575,0,1576,0,1577,0,1578,0,1593,0,1594,0,1595,0,1596,0,1597,0,1598,0,1599,0,1601,0,1602,0,1613,0,1614,0,1617,0,1623,0,1627,0,1629,0,1631,0,1632,0,1637,0,1638,0,1640,0,1641,0,1643,0,1644,0,1647,0,1648,0,1649,0,1650,0,1651,0,1657,0,1658,0,1659,0,1661,0,1665,0,1667,0,1672,0,1677,0,1678,0,1680,0,1681,0,1685,1,1686,1,1687,1,1693,0,1695,0,1706,0,1709,0,1710,0,1712,0,1714,0,1720,0,1721,0,1722,0,1726,0,1727,0,1729,0,1730,0,1734,1,1736,1,1737,3,1738,1,1740,1,1742,1,1745,0,1749,0,1750,0,1751,0,1752,0,1754,0,1755,0,1756,0,1757,0,1758,0,1763,0,1766,0,1767,0,1768,0,1769,0,1773,0,1774,0,1775,0,1777,0,1778,0,1781,0,1782,0,1784,0,1786,0,1787,0,1788,0,1789,0,1790,0,1791,0,1793,0,1797,0,1798,0,1799,0,1801,0,1802,0,1806,0,1807,0,1809,0,1810,0,1811,0,1813,0,1817,0,1832,0,1833,0,1835,0,1837,0,1839,0,1841,0,1844,0,1846,0,1849,0,1853,0,1854,0,1855,0,1856,0,1862,0,1865,0,1866,0,1867,0,1868,0,1873,0,1875,0,1879,0,1881,0,1884,0,1888,0,1889,0,1890,0,1891,0,1894,0,1895,0,1898,0,1899,0,1900,0,1902,0,1904,0,1905,0,1906,0,1910,0,1911,0,1912,0,1913,0,1915,0,1918,0,1919,0,1920,0,1921,0,1922,0,1923,0,1925,0,1926,0,1928,0,1931,0,1932,0,1933,0,1934,0,1937,1,1939,0,1954,1,1957,0,1959,0,1960,0,1961,0,1963,0,1965,0,1969,0,1970,0,1971,0,1972,0,1976,0,1979,0,1980,0,1990,0,1997,0,1998,0,1999,0,2001,0,2003,0,2006,0,2007,0,2008,0,2009,0,2012,0,2013,0,2017,0,2018,0,2021,0,2022,0,2024,0,2025,0,2026,0,2031,0,2032,0,2033,0,2036,0,2037,0,2038,0,2039,0,2043,0,2044,0,2045,0,2046,0,2047,0,2048,0,2052,0,2053,0,2054,0,2055,0,2056,0,2058,0,2066,1,2067,1,2068,1,2069,1,2073,2,2074,1,2075,1,2078,2,2082,1,2084,1,2070,0,2076,0,2083,0,2090,1,2091,1,2092,1,2093,0,2094,0,2098,1,2100,0,2103,1,2105,1,2116,3,2117,1,2119,1,2121,1,2124,1,2109,0,2113,0,2122,0,2133,1,2134,1,2137,1,2135,0,2140,1,2144,0,2146,0,2150,0,2163,0,2173,0,2176,1,2178,0,2194,0,2196,0,2199,0,2200,0,2201,0,2202,0,2203,0,2206,0,2207,0,2208,0,2209,0,2211,0,2213,0,2215,0,2222,0,2225,0,2227,0,2228,0,2229,0,2230,0,2235,0,2238,0,2243,0,2245,0,2246,0,2247,0,2248,0,2249,0,2250,0,2254,0,2263,0,2265,0,2266,0,2280,0,2287,0,2288,0,2289,0,2290,0,2294,0,2295,0,2298,0,2302,0,2308,0,2309,0,2312,0,2314,0,2315,0,2316,0,2319,0,2323,0,2325,0,2326,0,2327,0,2328,0,2335,0,2336,0,2338,0,2341,1,2342,7,2345,0,2346,0,2347,0,2353,0,2355,1,2360,1,2366,1,2368,2,2384,1,2385,4,2361,0,2362,0,2369,0,2370,0,2373,0,2376,0,2377,0,2378,0,2382,0,2393,1,2394,1,2395,1,2396,1,2404,1,2405,1,2407,0,2409,0,2411,0,2412,0,2413,0,2414,0,2415,0,2419,0,2422,0,2425,0,2426,0,2440,0,2442,0,2443,0,2447,0,2449,0,2451,0,2452,0,2453,0,2456,0,2458,0,2461,0,2464,0,2467,0,2468,0,2469,0,2473,0,2474,0,2475,0,2476,0,2477,0,2478,0,2480,0,2481,0,2489,0,2490,0,2493,1,2501,2,2513,1,2514,1,2522,1,2523,1,2524,1,2525,2,2533,1,2537,1,2552,3,2553,1,2554,1,2565,1,2569,1,2570,1,2502,0,2503,0,2504,0,2505,0,2506,0,2508,0,2509,0,2510,0,2515,0,2516,0,2518,0,2519,0,2520,0,2526,0,2527,0,2528,0,2530,0,2534,0,2538,0,2539,0,2541,0,2545,0,2549,0,2560,0,2561,0,2574,3,2576,2,2578,0,2580,0,2582,0,2584,3,2586,0,2588,0,2589,0,2590,0,2592,0,2593,0,2594,0,2596,0,2597,0,2598,0,2600,0,2601,0,2604,0,2605,0,2606,0,2607,0,2609,0,2610,0,2613,0,2614,0,2617,0,2618,0,2621,0,2622,0,2623,0,2628,0,2629,0,2630,0,2631,0,2632,0,2633,0,2636,0,2638,0,2639,0,2640,0,2641,0,2642,0,2643,0,2646,0,2648,0,2649,0,2650,0,2651,0,2652,0,2653,0,2654,0,2655,0,2658,0,2659,0,2660,0,2663,0,2664,0,2667,1,2668,2,2672,3,2673,1,2669,0,2670,0,2674,0,2675,0,2687,0,2689,1,2690,2,2693,1,2695,1,2696,5,2697,1,2700,1,2701,1,2703,2,2704,1,2705,1,2706,1,2709,0,2711,0,2713,0,2714,0,2715,0,2716,0,2717,0,2718,0,2719,0,2720,0,2721,0,2722,0,2727,0,2728,0,2731,0,2733,0,2734,0,2753,0,2754,0,2755,0,2756,0,2757,0,2758,0,2759,0,2762,0,2764,0,2775,0,2777,0,2778,0,2779,0,2780,0,2783,0,2784,0,2785,0,2787,0,2806,0,2814,0,2815,0,2816,0,2817,0,2818,0,2825,0,2826,0,2828,0,2831,0,2832,0,2833,0,2834,0,2835,0,2837,0,2838,0,2839,0,2841,0,2842,0,2843,0,2844,0,2846,0,2850,0,2853,1,2854,1,2855,2,2858,0,2859,0,2860,0,4164,1,4174,0,4175,3,4176,0,4177,0,4178,4,4179,5,4181,0,4182,0,4183,0,4184,0,4187,0,4188,0,4190,4,4191,0,4193,0,4195,0,4196,0,4197,0,4198,0,4201,1,4202,2,4203,0,4204,0,4205,0,4206,0,4207,0,4208,0,4209,0,4210,0,4211,0,4213,0,4215,0,4218,0,4219,0,4220,0,4221,0,4223,0,4224,0,4225,0,4226,0,4227,0,4228,0,4232,5,4233,0,4234,0,4236,0,4237,0,4239,0,4241,0,4242,0,4243,0,4245,0,4246,0,4247,0,4249,0,4250,0,4251,0,4253,0,4254,0,4257,0,4258,0,4261,0,4262,0,4263,0,4264,0,4265,0,4266,0,4267,0,4268,0,4269,0,4270,0,4271,0,4274,0,4275,0,4278,0,4279,0,4280,0,4283,0,4284,0,4285,0,4286,0,4287,0,4288,0,4290,0,4293,0,4294,0,4295,0,4296,0,4299,0,4301,0,4302,0,4303,0,4304,0,4305,0,4306,0,4307,0,4308,0,4309,0,4310,0,4311,0,4314,0,4326,0,4327,0,4329,0,4331,0,4333,0,4334,0,4335,0,4340,0,4342,0,4345,0,4349,0,4350,0,4351,0,4352,0,4358,0,4361,0,4362,0,4363,0,4364,0,4369,0,4370,0,4371,0,4372,0,4376,0,4377,0,4378,0,4381,0,4385,0,4386,0,4389,0,4390,0,4391,0,4393,0,4401,0,4402,0,4403,0,4404,0,4406,0,4407,0,4408,0,4409,0,4410,0,4411,0,4414,0,4415,0,4416,0,4417,0,4419,0,4420,0,4421,0,4422,0,4423,0,4424,0,4425,0,4428,0,4431,0,4432,0,4433,0,4434,0,4435,0,4436,0,4438,0,4439,0,4440,0,4441,0,4442,0,4443,0,4444,0,4446,0,4447,0,4448,0,4449,0,4450,0,4452,0,4453,0,4454,0,4455,0,4456,0,4457,0,4458,0,4460,0,4462,0,4463,0,4464,0,4465,0,4466,0,4468,0,4469,0,4470,0,4471,0,4472,0,4473,0,4474,0,4476,0,4479,0,4480,0,4481,0,4483,0,4484,0,4485,0,4486,0,4488,0,4489,0,4490,0,4491,0,4492,0,4493,0,4494,0,4507,0,4508,0,4509,0,4510,0,4511,0,4512,0,4513,0,4521,0,4522,0,4523,0,4536,0,4537,0,4538,0,4539,0,4541,0,4542,0,4546,0,4558,0,4561,0,4562,0,4563,0,4565,0,4567,0,4568,0,4569,0,4570,0,4571,0,4572,0,4573,0,4576,0,4577,0,4578,0,4579,0,4581,0,4582,0,4583,0,4586,0,4589,0,4590,0,4593,0,4594,0,4596,0,4600,0,4603,0,4608,0,4610,0,4612,0,4616,0,4617,0,4618,0,4619,0,4620,0,4621,0,4622,0,4623,0,4624,0,4627,0,3176,0,3187,0,3192,0,3193,0,3196,0,3199,0,3203,0,3206,0,3207,0,3209,0,3210,0,3211,0,3213,0,3214,0,3216,0,3225,0,3229,0,3230,0,3231,0,3232,0,3234,0,3235,0,3237,0,3238,0,3239,0,3240,0,3241,0,3242,0,3245,0,3255,0,3256,0,3257,0,3259,0,3260,0,3263,0,3264,0,3267,0,3268,0,3271,0,3282,0,3284,0,3287,0,3288,0,3290,0,3291,0,3292,0,3295,0,3296,0,3297,0,3298,0,3299,0,3303,0,3305,0,3306,0,3308,0,3335,0,3337,0,3338,0,3339,0,3340,0,3375,0,3376,0,3377,0,3378,0,3380,0,3382,0,3385,0,3388,0,3397,0,3398,0,3399,0,3401,0,3402,0,3403,0,3404,0,3405,0,3406,0,3409,0,3410,0,3411,0,3412,0,3435,0,3436,0,3437,0,3438,0,3439,0,3453,0,3455,0,3456,0,3458,0,3460,0,3461,0,3462,0,3463,0,3464,0,3465,0,3474,0,3481,0,3492,0,3493,0,3494,0,3495,0,3496,0,3502,0,3503,0,3504,0,3505,0,3506,0,3507,0,3511,0,3512,0,3513,0,3517,0,3518,0,3519,0,3520,0,3522,0,3523,0,3524,0,3525,0,3526,0,3530,0,3551,0,3553,0,3554,0,3556,0,3559,0,3560,0,3561,0,3562,0,3563,0,3565,0,3582,0,3583,0,3584,0,3585,0,3586,0,3587,0,3588,0,3589,0,3590,0,3595,0,3604,0,3608,0,3609,0,3610,0,3611,0,3612,0,3616,0,3619,0,3622,0,3624,0,3626,0,3627,0,3629,0,3630,0,3631,0,3632,0,3633,0,3637,0,3638,0,3641,0,3642,0,3643,0,3644,0,3645,0,3650,0,3651,0,3653,0,3658,0,3661,0,3664,0,3672,0,3677,0,3678,0,3679,0,3680,0,3681,0,3682,0,3684,0,3685,0,3686,0,3689,0,3690,0,3691,0,3692,0,3693,0,3699,0,3700,0,299,0,304,0,309,0,328,0,332,0,336,0,470,0,734,1,788,1,791,2,792,1,793,1,797,1,806,1,812,1,813,2,814,2,815,2,816,1,817,1,818,1,819,1,820,1,823,1,826,1,827,1,842,2,843,1,844,1,845,1,846,1,854,1,855,2,862,1,871,2,877,2,882,1,886,1,889,2,890,2,901,2,969,2,999,3,1008,1,795,0,796,0,798,0,824,0,829,0,830,0,832,0,887,0,888,0,903,0,905,0,910,0,914,0,915,0,919,0,920,0,922,0,923,0,925,0,926,0,927,0,928,0,929,0,931,0,932,0,933,0,934,0,935,0,936,0,937,0,938,0,940,0,943,0,946,0,947,0,948,0,949,0,950,0,951,0,952,0,953,0,954,0,956,0,957,0,958,0,959,0,960,0,961,0,962,0,963,0,965,0,972,0,973,0,974,0,975,0,976,0,977,0,978,0,979,0,980,0,982,0,983,0,984,0,985,0,986,0,987,0,988,0,989,0,991,0,1000,0,1001,0,1002,0,1003,0,1004,0,1005,0,1006,0,1012,0,1024,0,1027,0,1028,0,1053,0,1054,0,1090,0,1092,0,1108,0,1109,0,1110,0,1121,0,1123,0,1124,0,1136,0,1137,0,1148,0,1149,0,1165,0,1167,0,1190,0,1191,0,1194,0,1199,0,1202,0,1203,0,1204,0,1205,0,1207,0,1210,0,1211,0,1213,0,1214,0,1215,0,1217,0,1218,0,1222,0,1223,0,1226,0,1227,0,1228,0,1230,0,1252,0,1253,0,1278,0,1279,0,1285,0,1286,0,1287,0,1288,0,1290,0,1291,0,1292,0,1296,0,1299,0,1302,0,1305,0,1307,0,1308,0,1312,0,1313,0,1314,0,1316,0,1320,0,1322,0,1323,0,1324,0,1328,0,1329,0,1331,0,1332,0,1334,0,1335,0,1336,0,1337,0,1338,0,1339,0,1340,0,1341,0,1342,0,1345,0,1346,0,1347,0,3889,1,3941,3,3975,2,3976,1,3977,1,3978,2,3979,1,3980,2,3981,2,3983,2,3984,1,3985,1,3986,2,3987,2,3988,2,3989,2,3991,2,3992,1,3993,2,3994,2,3995,1,3996,2,3997,2,3999,2,4000,1,4001,2,4002,1,4003,2,4004,2,4006,2,4007,2,4008,2,4009,2,4010,2,4011,2,4013,2,4014,1,4015,2,4016,1,4017,2,4018,2,4020,2,4021,1,4022,2,4023,2,4024,2,4025,2,4026,2,4027,2,4028,2,4030,2,4031,1,4032,2,4033,2,4034,2,4035,2,4036,2,4037,2,4039,2,4040,1,4041,2,4042,2,4043,2,4044,2,4046,2,4047,1,4048,2,4049,2,4050,2,4051,2,4053,1,4054,1,4056,2,4057,1,4058,1,4059,2,4060,2,4061,2,4063,2,4064,1,4065,1,4066,2,4067,2,4068,2,4070,2,4071,1,4072,1,4073,2,4074,2,4076,2,4077,1,4078,1,4079,2,4080,2,4081,2,4083,2,4084,1,4085,1,4086,2,4087,2,4088,2,4090,2,4091,1,4092,2,4093,2,4094,2,4096,2,4097,1,4098,1,4099,2,4100,2,4102,2,4103,1,4104,1,4105,2,4107,2,4108,1,4109,1,4113,2,4114,1,4116,2,4117,1,4118,1,4119,1,4133,1,4134,1,4136,2,4137,1,4139,2,4141,1,4142,1,4143,1,4144,2,4648,1,4650,4,4651,3,4652,4,4653,4,4654,4,4659,2,2153,0,2154,0,2156,0,2157,0,2158,0,2159,0,2164,0,2165,0,2168,0,2169,0,3945,1,3946,2,3952,1,3953,3,3954,1,3955,2,3965,1,3966,4,3967,2,1471,0,1696,0,1698,0,1700,0,2739,0,2742,0,2743,0,2744,0,2747,0,2748,0,2750,0,3309,0,3310,0,3312,0,3313,0,3314,0,3316,0,3317,0,3319,0,3320,0,3321,0,3322,0,1168,0,1169,0,1170,0,1171,0,1173,0,1174,0,1175,0,1176,0,1177,0,1195,0,1196,0,1262,0,1263,0,1267,0,1268,0,1269,0,1271,0,1272,0,1273,0]},{"source":"dart:core/runtime/libinvocation_mirror_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibinvocation_mirror_patch.dart","uri":"dart:core/runtime/libinvocation_mirror_patch.dart","_kind":"library"},"hits":[53,0,55,0,56,0,57,0,171,0,175,0,60,0,61,0,62,0,63,0,64,0,65,0,66,0,67,0,68,0,70,0,71,0,72,0,76,0,77,0,78,0,80,0,83,0,84,0,85,0,88,0,89,0,90,0,91,0,96,0,98,0,102,0,105,0,106,0,109,0,110,0,111,0,114,0,115,0,116,0,118,0,121,0,122,0,123,0,124,0,125,0,126,0,127,0,129,0,130,0,131,0,132,0,133,0,134,0,135,0,136,0,138,0,140,0,143,0,144,0,145,0,147,0,150,0,151,0,152,0,154,0,157,0,158,0,159,0,161,0,164,0,165,0,166,0,168,0,179,0,182,0,191,0,197,0]},{"source":"dart:core/runtime/liberrors_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Fliberrors_patch.dart","uri":"dart:core/runtime/liberrors_patch.dart","_kind":"library"},"hits":[255,0,262,0,263,0,267,0,270,0,273,0,190,0,192,0,229,0,194,0,195,0,201,0,203,0,207,0,215,0,216,0,240,0,242,0,243,0,244,0,245,0,246,0,276,0,434,0,435,0,437,0,438,0,440,0,442,0,443,0,446,0,448,0,449,0,450,0,451,0,453,0,456,0,457,0,467,0,471,0,478,0,481,0,484,0,486,0,488,0,492,0,495,0,496,0,498,0,500,0,501,0,507,0,513,0,515,0,517,0,520,0,522,0,523,0,526,0,528,0,529,0,530,0,533,0,535,0,541,0,542,0,544,0,547,0,548,0,549,0,550,0,551,0,552,0,553,0,555,0,559,0,560,0,561,0,562,0,564,0,566,0,569,0,280,0,282,0,284,0,287,0,288,0,290,0,291,0,292,0,296,0,297,0,298,0,299,0,300,0,301,0,302,0,304,0,306,0,308,0,309,0,312,0,313,0,314,0,317,0,320,0,322,0,333,0,337,0,344,0,347,0,350,0,352,0,354,0,357,0,360,0,361,0,363,0,365,0,366,0,371,0,377,0,379,0,381,0,384,0,386,0,387,0,390,0,392,0,393,0,394,0,397,0,399,0,405,0,406,0,408,0,411,0,412,0,413,0,415,0,417,0,418,0,419,0,420,0,421,0,423,0,427,0,430,0,80,0,83,0,86,0,93,0,94,0,98,0,26,0,32,0,33,0,36,0,39,0,43,0,44,0,46,0,47,0,52,0,53,0,54,0,55,0,58,0,59,0,60,0,61,0,64,0,66,0,68,0,69,0,613,0,575,0,576,0,158,0,161,0,165,0,166,0,167,0,144,0,145,0,137,0,138,0,15,0,16,0,10,0,11,0,20,0,119,0,123,0,126,0,127,0,102,0,107,0,151,0,152,0,605,0,607,0,579,0,581,0,582,0,588,0,590,0,591,0,458,0,459,0,461,0,462,0,463,0,464,0,323,0,324,0,326,0,327,0,328,0,329,0]},{"source":"dart:core/runtime/liburi_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Fliburi_patch.dart","uri":"dart:core/runtime/liburi_patch.dart","_kind":"library"},"hits":[28,0,31,2,34,1,43,3,44,1,45,1,46,6,51,2,55,0,56,0,57,0,61,0,62,0,63,0,64,0,65,0,66,0,67,0,68,0,72,0,73,0,74,0,77,0,20,0,9,0,10,0]},{"source":"dart:core/duration.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fduration.dart","uri":"dart:core/duration.dart","_kind":"library"},"hits":[127,0,134,0,135,0,136,0,137,0,138,0,143,0,149,0,150,0,157,0,158,0,168,0,169,0,178,0,181,0,182,0,189,0,195,0,201,0,207,0,212,0,219,0,226,0,233,0,240,0,245,0,250,0,251,0,252,0,255,0,268,0,279,0,294,0,295,0,297,0,298,0,300,0,301,0,310,0,319,0,328,0,280,0,281,0,282,0,283,0,284,0,285,0,286,0,289,0,290,0,291,0]},{"source":"dart:core/runtime/libgrowable_array.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibgrowable_array.dart","uri":"dart:core/runtime/libgrowable_array.dart","_kind":"library"},"hits":[8,0,9,0,10,0,12,0,13,0,16,0,20,0,21,0,22,0,23,0,26,0,27,0,28,0,29,0,30,0,32,0,36,0,37,0,38,0,39,0,46,0,47,0,48,0,51,0,52,0,54,0,58,0,59,0,60,0,63,0,64,0,65,0,67,0,68,0,73,0,74,0,75,0,76,0,79,0,80,0,81,0,82,0,83,0,84,0,85,0,87,0,88,0,92,1,93,1,94,1,95,1,96,0,101,1,102,1,103,1,106,0,108,0,110,0,112,1,113,1,115,1,116,1,117,1,126,0,128,0,130,0,131,0,134,0,137,0,139,0,141,0,143,1,144,1,147,0,149,1,150,1,151,2,154,2,155,1,152,0,158,0,159,0,160,0,161,0,162,0,163,0,164,0,165,0,167,0,168,0,169,0,171,0,172,0,173,0,177,0,179,0,181,0,182,0,187,0,188,0,190,0,191,0,192,0,193,0,194,0,195,0,198,0,202,0,203,0,204,0,205,0,209,0,210,0,211,0,214,1,215,5,216,0,219,0,220,0,221,0,222,0,229,1,230,1,232,1,236,2,240,0,242,1,243,1,250,2,255,1,251,0,252,0,258,0,259,0,261,0,262,0,263,0,266,0,271,0,272,0,273,0,274,0,275,0,279,0,280,0,281,0,282,0,283,0,286,0,287,0,289,0,290,0,291,0,301,0,302,0,303,0,304,0,306,0,310,0,311,0,312,0,315,0,317,0,318,0,319,0,320,0,321,0,322,0,323,0,324,0,326,0,328,0,330,0,333,0,336,0,337,0,338,0,339,0,340,0,341,0,343,0,346,1,347,1,350,1,351,2,354,2,356,0,357,0,360,0,362,0,363,0,366,0,367,0,368,0,369,0,370,0,371,0,374,0,375,0,378,0,381,0,382,0]},{"source":"dart:core/exceptions.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fexceptions.dart","uri":"dart:core/exceptions.dart","_kind":"library"},"hits":[21,0,28,0,30,0,31,0,32,0,177,1,178,0,77,0,94,0,96,0,97,0,99,0,100,0,102,0,106,0,111,0,112,0,113,0,115,0,120,0,121,0,122,0,123,0,124,0,126,0,128,0,129,0,130,0,134,0,135,0,137,0,139,0,140,0,141,0,142,0,147,0,152,0,155,0,156,0,157,0,159,0,160,0,164,0,165,0,169,0,170,0,171,0]},{"source":"dart:core/runtime/libfunction.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibfunction.dart","uri":"dart:core/runtime/libfunction.dart","_kind":"library"},"hits":[8,0,10,0,11,0,12,0,14,0,17,0,19,0,21,0]},{"source":"dart:core/runtime/libexpando_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibexpando_patch.dart","uri":"dart:core/runtime/libexpando_patch.dart","_kind":"library"},"hits":[10,0,12,0,95,0,99,0,100,0,101,0,102,0,103,0,105,0,109,0,110,0,111,0,112,0,113,0,115,0,119,0,120,0,122,0,123,0,127,0,128,0,130,0,136,0,138,0,139,0,140,0,141,0,146,0,147,0,19,0,20,0,22,0,23,0,24,0,27,0,28,0,29,0,31,0,33,0,34,0,41,0,42,0,44,0,45,0,47,0,50,0,53,0,56,0,59,0,61,0,63,0,64,0,68,0,69,0,78,0,80,0,84,0,85,0,86,0,91,0,92,0]},{"source":"dart:core/expando.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fexpando.dart","uri":"dart:core/expando.dart","_kind":"library"},"hits":[43,0]},{"source":"dart:core/comparable.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fcomparable.dart","uri":"dart:core/comparable.dart","_kind":"library"},"hits":[93,0]},{"source":"dart:core/runtime/libintegers.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibintegers.dart","uri":"dart:core/runtime/libintegers.dart","_kind":"library"},"hits":[12,0,13,2,14,0,16,0,17,0,20,0,23,0,24,0,27,0,28,0,31,0,34,1,35,1,38,0,39,0,40,0,42,0,43,0,46,0,47,0,48,0,49,0,50,0,51,0,52,0,53,0,54,0,55,0,56,0,57,0,58,0,61,0,62,0,64,0,65,0,68,0,69,0,72,0,73,0,76,0,77,0,80,0,83,0,84,0,85,0,90,0,91,0,92,0,95,0,96,0,99,0,100,0,101,0,102,0,103,0,104,0,106,0,107,0,110,0,116,0,117,0,120,0,122,0,135,0,137,0,141,0,142,0,145,0,148,0,150,0,154,0,157,0,159,0,166,0,170,0,174,0,178,0,182,0,183,0,186,0,187,0,190,0,191,0,194,0,195,0,198,0,199,0,200,0,202,0,203,0,207,0,208,0,209,0,213,0,214,0,216,0,218,0,219,0,223,0,228,1,227,0,231,0,232,0,235,0,236,0,239,0,240,0,245,0,246,0,247,0,249,0,250,0,252,0,253,0,254,0,255,0,259,0,261,0,263,0,264,0,265,0,266,0,267,0,269,0,270,0,271,0,276,0,278,0,280,0,281,0,284,0,286,0,290,0,294,0,295,0,296,0,297,0,299,0,300,0,301,0,308,0,309,0,313,0,314,0,315,0,316,0,317,0,319,0,320,0,321,0,327,0,328,0,329,0,331,0,332,0,334,0,335,0,336,0,338,0,339,0,342,0,343,0,344,0,346,0,347,0,355,0,358,0,359,0,360,0,361,0,363,0,369,0,374,0,375,0,377,0,378,0,379,0,381,0,382,0,383,0,385,0,387,0,388,0,390,0,391,0,392,0,394,0,395,0,396,0,398,0,400,0,401,0,402,0,403,0,405,0,406,0,407,0,409,0,410,0,411,0,412,0,414,0,415,0,416,0,417,0,418,0,419,0,425,0,426,0,427,0,429,0,430,0,432,0,433,0,434,0,435,0,437,0,441,0,442,0,443,0,445,0,446,0,447,0,448,0,449,0,450,0,653,0,654,0,656,0,657,0,658,0,659,0,661,0,455,0,456,0,458,1,459,0,460,0,461,0,463,2,465,0,534,0,537,0,538,0,539,0,540,0,541,0,544,0,545,0,546,0,548,0,549,0,550,0,553,0,554,0,555,0,560,0,561,0,562,0,566,0,567,0,568,0,569,0,570,0,571,0,572,0,573,0,575,0,578,0,579,0,580,0,587,0,590,0,591,0,592,0,593,0,594,0,597,0,598,0,599,0,601,0,602,0,603,0,609,0,614,0,615,0,616,0,617,0,619,0,620,0,621,0,622,0,623,0,624,0,627,0,628,0,629,0,632,0,633,0,634,0,635,0,636,0,637,0,638,0,639,0,640,0,643,0,644,0,645,0]},{"source":"dart:core/stacktrace.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fstacktrace.dart","uri":"dart:core/stacktrace.dart","_kind":"library"},"hits":[58,0,59,0,17,0]},{"source":"dart:core/iterable.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fiterable.dart","uri":"dart:core/iterable.dart","_kind":"library"},"hits":[85,1,102,0,103,0,104,0,123,0,124,0,166,0,174,0,175,0,176,0,178,0,195,0,211,0,224,0,246,0,247,0,265,0,266,0,267,0,276,0,277,0,302,0,303,0,304,0,305,0,307,0,308,0,309,0,333,0,335,0,345,0,346,0,347,0,360,0,361,0,362,0,363,0,364,0,366,0,367,0,369,0,370,0,371,0,372,0,375,0,384,0,385,0,386,0,397,0,398,0,410,0,419,0,422,0,423,0,424,0,434,0,441,0,454,0,455,0,468,0,469,0,488,0,489,0,504,0,505,0,515,0,516,0,517,0,518,0,520,0,533,0,534,0,535,0,536,0,540,0,541,0,550,0,551,0,552,0,553,0,554,0,567,0,568,0,569,0,571,0,572,0,589,0,592,0,593,0,599,0,600,0,613,0,616,0,617,0,619,0,626,0,627,0,641,0,642,0,643,0,645,0,646,0,647,0,649,0,668,0,683,0,686,0,688,0,689,0,690,0,694,0]},{"source":"dart:core/runtime/libstring_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibstring_patch.dart","uri":"dart:core/runtime/libstring_patch.dart","_kind":"library"},"hits":[1227,0,1228,0,1232,0,1235,0,1236,0,1239,0,1241,0,1242,0,1281,0,1283,0,1284,0,1285,0,1287,0,1288,0,1289,0,1291,0,1294,0,1295,0,1296,0,1297,0,1312,0,1314,0,1315,0,1317,0,1318,0,1319,0,1320,0,1322,0,1264,0,1265,0,1269,0,1270,0,1273,0,1275,0,1276,0,924,0,925,0,929,0,931,0,933,0,934,0,937,0,938,0,941,0,944,0,947,1,951,2,953,2,954,2,957,0,961,0,962,0,964,0,966,0,967,0,969,0,970,0,971,0,972,0,973,0,979,1,981,1,982,1,986,1,987,2,1000,1,983,0,984,0,988,0,989,0,992,0,993,0,1003,0,1004,0,1005,0,1006,0,1007,0,1009,0,1010,0,1011,0,1012,0,1015,0,1016,0,1023,0,1026,0,1027,0,1028,0,1029,0,1030,0,1031,0,1033,0,1034,0,1035,0,1041,0,1042,0,1043,0,1044,0,1045,0,1047,0,1048,0,1049,0,1050,0,1051,0,1052,0,1054,0,1055,0,1056,0,1057,0,1060,0,1061,0,1062,0,1066,0,1067,0,1072,0,1073,0,1074,0,1075,0,1076,0,1078,0,1079,0,1080,0,1081,0,1082,0,1083,0,1085,0,1086,0,1088,0,1089,0,1090,0,1091,0,1094,0,1095,0,1096,0,1149,0,1150,0,1151,0,1152,0,1154,0,1155,0,1156,0,1158,0,1159,0,1166,0,1167,0,1168,0,1170,0,1174,0,1175,0,1176,0,1180,0,1185,0,1186,0,1187,0,1189,0,1190,0,1199,0,1201,0,1206,0,1211,1,1218,2,1219,2,1220,1,15,0,17,0,18,0,19,0,20,0,21,0,25,0,26,0,27,0,28,0,30,0,31,0,32,0,34,0,35,0,36,0,37,0,38,0,39,0,40,0,41,0,46,0,50,0,94,0,95,0,98,0,100,1,102,1,103,1,104,0,117,0,119,0,120,0,122,0,124,0,125,0,126,0,127,0,128,0,129,0,130,0,131,0,134,0,135,0,136,0,137,0,139,0,141,0,142,0,144,0,145,0,147,0,148,0,153,0,156,0,158,0,159,0,160,0,161,0,166,0,169,0,170,0,171,0,173,0,174,0,178,0,179,0,180,0,181,0,182,0,188,0,189,0,190,0,191,0,192,0,194,0,196,0,197,0,199,0,200,0,201,0,202,0,203,0,205,0,206,0,207,0,210,0,211,0,212,0,214,0,216,0,218,0,221,0,223,0,224,0,225,0,230,0,233,0,237,0,239,0,240,0,243,2,245,0,247,1,251,0,255,0,256,0,257,0,258,0,267,0,268,0,269,0,270,0,271,0,272,0,273,0,274,0,277,0,281,0,282,0,286,0,287,0,288,0,289,0,292,0,293,0,300,0,301,0,304,1,305,3,308,1,309,1,306,0,311,0,314,1,315,3,318,1,320,3,322,2,323,1,316,0,329,0,332,0,337,1,339,1,343,1,345,3,346,1,347,1,348,1,340,0,341,0,354,0,357,0,362,1,363,1,365,3,368,3,371,1,374,1,366,0,369,0,372,0,377,1,383,1,386,3,389,0,390,0,392,0,395,0,399,0,400,0,401,0,402,0,404,0,421,0,422,0,423,0,425,0,426,0,427,0,428,0,429,0,430,0,431,0,432,0,433,0,434,0,437,0,438,0,440,0,441,0,448,0,449,0,450,0,451,0,458,0,459,0,460,0,461,0,465,0,466,0,471,0,474,0,475,0,477,0,478,0,482,0,486,0,491,0,494,0,495,0,496,0,497,0,498,0,502,0,506,0,510,0,513,0,514,0,515,0,516,0,517,0,518,0,520,0,523,0,524,0,525,0,526,0,527,0,528,0,530,0,531,0,534,0,535,0,536,0,537,0,538,0,539,0,541,0,544,0,545,0,546,0,547,0,549,0,551,0,554,0,556,0,557,0,559,0,560,0,562,0,563,0,565,0,566,0,567,0,568,0,569,0,570,0,571,0,574,1,575,1,576,1,577,1,578,1,579,1,580,3,581,1,582,1,584,1,585,1,586,1,589,0,590,0,591,0,592,0,593,0,597,0,598,0,599,0,600,0,601,0,603,0,604,0,610,0,611,0,612,0,613,0,615,0,617,0,619,0,620,0,621,0,624,0,625,0,626,0,627,0,628,0,631,0,632,0,634,0,635,0,637,0,639,0,646,0,648,0,650,0,651,0,652,0,655,0,656,0,657,0,658,0,659,0,661,0,666,0,668,0,669,0,674,0,675,0,695,0,699,0,700,0,701,0,702,0,706,0,707,0,708,0,709,0,710,0,712,0,713,0,715,0,716,0,718,0,719,0,720,0,722,0,726,0,728,0,729,0,730,0,731,0,733,0,734,0,735,0,736,0,737,0,740,0,741,0,743,0,746,0,747,0,749,0,750,0,751,0,753,0,754,0,756,0,757,0,759,0,760,0,764,0,765,0,767,0,768,0,769,0,772,0,774,0,775,0,779,0,781,0,782,0,785,0,787,0,788,0,789,0,790,0,792,0,793,0,797,1,798,1,799,0,800,0,801,0,811,0,812,0,815,0,816,0,817,0,818,0,819,0,820,0,821,0,822,0,823,0,826,0,827,0,828,0,829,0,830,0,831,0,834,0,838,0,841,0,842,0,843,0,845,0,848,0,849,0,850,0,852,0,853,0,854,0,858,0,861,0,862,0,863,0,864,0,865,0,869,0,870,0,871,0,873,0,875,0,881,0,882,0,885,0,886,0,887,0,890,0,891,0,892,0,895,0,901,0,903,0,905,0,907,0,910,1,911,2,914,1,912,0,919,0,1247,0,1248,0,1252,0,1253,0,1256,0,1258,0,1259,0,1332,0,1334,0,1335,0,1336,0,1339,0,1340,0,1341,0,1342,0,1345,0,1346,0,1348,0,1349,0,1353,0]},{"source":"dart:core/runtime/libdouble.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibdouble.dart","uri":"dart:core/runtime/libdouble.dart","_kind":"library"},"hits":[8,0,10,0,11,0,13,0,14,0,17,0,19,0,20,0,23,0,25,0,26,0,29,0,31,0,32,0,35,0,37,0,38,0,41,0,43,0,44,0,47,0,49,0,50,0,53,0,55,0,57,0,58,0,61,0,62,0,63,0,64,0,67,0,68,0,71,0,72,0,73,0,76,0,77,0,81,1,80,0,85,1,84,0,89,1,88,0,93,1,92,0,97,1,96,0,101,1,100,0,104,0,107,0,108,0,109,0,110,0,112,0,114,0,115,0,118,0,119,0,120,0,124,0,125,0,126,0,127,0,129,0,130,0,131,0,132,0,134,0,135,0,136,0,138,0,139,0,142,0,143,0,145,0,146,0,147,0,151,0,153,0,164,0,166,0,168,0,170,0,171,0,178,0,180,0,181,0,182,0,186,0,189,0,190,0,194,0,195,0,202,0,207,0,208,0,211,0,214,0,216,0,225,0,226,0,229,0,230,0,234,0,235,0,236,0,242,0,245,0,248,0,255,0,256,0,259,0,260,0,263,0,264,0,265,0,267,0,270,0,274,0,276,0,278,0,280,0,281,0,282,0,283,0,284,0,288,0,293,0,294,0,307,0,311,0,315,0,316,0]},{"source":"dart:core/map.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fmap.dart","uri":"dart:core/map.dart","_kind":"library"},"hits":[394,0,396,0,168,0,169,0,177,0,178,0]},{"source":"dart:core/runtime/libarray_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibarray_patch.dart","uri":"dart:core/runtime/libarray_patch.dart","_kind":"library"},"hits":[55,1,56,1,57,1,59,0,60,0,10,0,13,0,16,0,18,0,19,0,26,0,27,0,28,0,29,0,30,0,33,0,34,0,39,0,40,0,41,0,44,0,48,0,49,0,50,0]},{"source":"dart:core/list.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Flist.dart","uri":"dart:core/list.dart","_kind":"library"},"hits":[139,0,140,0,153,1,157,2,161,2,162,2,159,0,192,0,210,0,213,0,214,0,215,0,216,0,217,0,219,0,220,0,221,0,224,0,225,0,243,0,244,0,246,0,247,0,248,0,249,0,251,0,252,0]},{"source":"dart:core/object.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fobject.dart","uri":"dart:core/object.dart","_kind":"library"},"hits":[29,1]},{"source":"dart:core/runtime/libobject_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibobject_patch.dart","uri":"dart:core/runtime/libobject_patch.dart","_kind":"library"},"hits":[21,0,22,0,23,0,25,0,27,0,28,0,29,0,36,0,41,0,52,0,56,0,60,0,61,1,62,0,66,0,14,0,35,0,39,0,44,0,46,0,50,0,7,0,8,0]},{"source":"dart:core/runtime/libcore_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibcore_patch.dart","uri":"dart:core/runtime/libcore_patch.dart","_kind":"library"},"hits":[136,0,133,0,134,0,138,0,139,0,143,0,144,0,147,0,151,0,152,0,153,0,156,0,159,0,160,0,161,0,172,0,114,0,116,0,119,0,120,0,100,0,101,0]},{"source":"dart:core/set.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fset.dart","uri":"dart:core/set.dart","_kind":"library"},"hits":[113,0,114,0]},{"source":"dart:core/runtime/libdouble_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibdouble_patch.dart","uri":"dart:core/runtime/libdouble_patch.dart","_kind":"library"},"hits":[11,0,14,0,30,0,31,0,33,0,34,0,35,0,37,0,38,0,39,0,43,0,44,0,45,0,46,0,52,0,53,0,54,0,55,0,58,0,59,0,60,0,61,0,62,0,65,0,66,0,68,0,70,0,71,0,72,0,74,0,76,0,83,0,85,0,86,0,87,0,88,0,90,0,91,0,94,0,95,0,96,0,97,0,98,0,100,0,102,0,106,0,108,0,110,0,111,0,117,0]},{"source":"dart:core/runtime/liblib_prefix.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Fliblib_prefix.dart","uri":"dart:core/runtime/liblib_prefix.dart","_kind":"library"},"hits":[9,0,10,0,11,0,12,0,15,0,16,0,17,0,18,0,22,0,23,0,24,0,25,0,26,0,27,0,40,0,49,0,54,0,55,0,60,0,61,0,62,0,63,0,64,0,65,0,70,0,71,0,72,0,73,0,75,0,28,0,34,0,35,0,36,0,37,0]},{"source":"dart:core/runtime/libarray.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibarray.dart","uri":"dart:core/runtime/libarray.dart","_kind":"library"},"hits":[8,0,10,0,12,1,13,1,16,0,18,0,20,0,21,0,22,0,23,0,24,0,28,0,32,0,36,0,37,0,38,0,40,0,41,0,43,0,44,0,46,0,47,0,49,0,50,0,51,0,53,0,54,0,55,0,56,0,58,0,59,0,60,0,65,0,66,0,67,0,68,0,69,0,70,0,76,0,77,0,78,0,79,0,83,0,84,0,87,0,88,0,89,0,92,0,93,0,94,0,97,0,98,0,99,0,100,0,103,0,104,0,105,0,106,0,108,0,113,0,202,0,204,0,209,0,211,0,212,0,213,0,216,0,217,0,125,0,126,0,130,0,133,0,135,0,137,0,138,0,139,0,140,0,141,0,142,0,143,0,145,0,146,0,152,0,153,0,154,0,155,0,159,0,160,0,163,0,164,0,165,0,168,0,169,0,170,0,173,0,174,0,175,0,176,0,179,0,180,0,181,0,182,0,183,0,184,0,187,0,188,0,191,0]},{"source":"dart:core/string.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fstring.dart","uri":"dart:core/string.dart","_kind":"library"},"hits":[664,0,679,0,683,0,684,0,688,0,689,0,690,0,691,0,692,0,693,0,702,0,713,0,714,0,715,0,716,0,729,0,730,0,731,0,732,0,733,0,739,0,746,0,756,0,757,0,758,0,759,0,762,0,763,0,764,0,765,0,768,0,769,0,770,0,771,0,772,0,773,0,774,0,778,0,779,0,783,0,784,0,785,0,786,0,789,0,790,0,791,0,792,0,793,0,794,0,795,0,799,0,800,0,614,0,616,0,618,0,619,0,620,0,622,0,623,0,624,0,625,0,626,0,627,0,635,0,638,0,641,0,642,0]},{"source":"dart:core/num.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fnum.dart","uri":"dart:core/num.dart","_kind":"library"},"hits":[470,0,471,0,473,0,474,0,483,0,484,0,486,0,490,0,491,0]},{"source":"dart:core/runtime/libintegers_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibintegers_patch.dart","uri":"dart:core/runtime/libintegers_patch.dart","_kind":"library"},"hits":[21,0,25,0,27,0,28,0,29,0,30,0,34,0,35,0,39,0,40,0,41,0,44,0,46,0,64,0,65,0,66,0,67,0,69,0,71,0,73,0,74,0,75,0,76,0,77,0,79,0,84,0,85,0,86,0,87,0,88,0,89,0,90,0,91,0,93,0,95,0,102,0,104,0,123,0,125,0,126,0,128,0,130,0,133,0,135,0,136,0,137,0,138,0,139,0,140,0,148,0,150,0,151,0,152,0,154,0,157,0,160,0,161,0,162,0,163,0,165,0,166,0,168,0,170,0,171,0,172,0,176,0,178,0,179,0,180,0,181,0,182,0,186,0,187,0,188,0,192,0,194,0,195,0,200,0,202,0,203,0,204,0,205,0,206,0,209,0,210,0,211,0,212,0,213,0,214,0,216,0,290,0,291,0,292,0,293,0,294,0,295,0,11,0,50,0,51,0,52,0,53,0,55,0,57,0,58,0,61,0,110,0,111,0,112,0,113,0,115,0,117,0,118,0,120,0]},{"source":"dart:core/runtime/libstring_buffer_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibstring_buffer_patch.dart","uri":"dart:core/runtime/libstring_buffer_patch.dart","_kind":"library"},"hits":[55,1,56,1,131,0,132,0,133,0,134,0,135,0,144,1,145,2,146,0,147,0,148,0,149,0,156,1,157,1,158,2,159,2,161,1,163,3,165,2,166,4,167,1,168,0,177,0,178,0,179,0,180,0,181,0,182,0,184,0,185,0,187,0,188,0,194,0,60,0,63,1,64,1,65,1,66,1,67,1,71,0,72,0,73,0,74,0,76,0,77,0,78,0,80,0,81,0,83,0,84,0,85,0,86,0,87,0,92,0,93,0,94,0,95,0,97,0,98,0,100,0,101,0,102,0,103,0,109,0,110,0,111,0,116,0,117,0,118,0,123,1,124,1,125,2,127,4]},{"source":"dart:core/string_buffer.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fstring_buffer.dart","uri":"dart:core/string_buffer.dart","_kind":"library"},"hits":[25,0,31,0]},{"source":"dart:core/runtime/libweak_property.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibweak_property.dart","uri":"dart:core/runtime/libweak_property.dart","_kind":"library"},"hits":[8,0,10,0,11,0,12,0,14,0,16,0,17,0,18,0]},{"source":"dart:core/runtime/libmap_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibmap_patch.dart","uri":"dart:core/runtime/libmap_patch.dart","_kind":"library"},"hits":[14,0,15,0,16,0,17,0,18,0,29,0,24,0,25,0]},{"source":"dart:core/invocation.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Finvocation.dart","uri":"dart:core/invocation.dart","_kind":"library"},"hits":[15,0,23,0,26,0,38,0,41,0,71,0,108,0,120,0,122,0,123,0,124,0,126,0,128,0,133,0,135,0,138,0,140,0,142,0,143,0,144,0,145,0,148,0,150,0,151,0,152,0,153,0,159,0,161,0]},{"source":"dart:core/stopwatch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fstopwatch.dart","uri":"dart:core/stopwatch.dart","_kind":"library"},"hits":[31,0,32,0,38,0,49,0,50,0,53,0,54,0,65,0,66,0,74,0,75,0,89,0,90,0,96,0,97,0,103,0,104,0,110,0,111,0,117,0]},{"source":"dart:core/runtime/libstopwatch_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibstopwatch_patch.dart","uri":"dart:core/runtime/libstopwatch_patch.dart","_kind":"library"},"hits":[21,0,10,0,12,0,18,0]},{"source":"dart:core/date_time.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fdate_time.dart","uri":"dart:core/date_time.dart","_kind":"library"},"hits":[224,0,246,0,265,0,424,0,425,0,426,0,427,0,428,0,429,0,431,0,432,0,319,0,320,0,321,0,346,0,347,0,348,0,349,0,350,0,351,0,353,0,355,0,357,0,359,0,362,0,364,0,365,0,366,0,367,0,368,0,371,0,374,0,376,0,378,0,382,0,385,0,386,0,451,0,452,0,453,0,476,0,477,0,500,0,501,0,524,0,525,0,536,0,538,0,551,0,552,0,553,0,569,0,570,0,571,0,574,0,575,0,576,0,577,0,578,0,579,0,580,0,583,0,585,0,586,0,587,0,588,0,591,0,592,0,593,0,594,0,597,0,598,0,599,0,613,0,614,0,615,0,616,0,617,0,618,0,619,0,620,0,621,0,622,0,623,0,625,0,650,0,652,0,653,0,654,0,655,0,656,0,657,0,658,0,659,0,660,0,661,0,663,0,323,0,325,0,330,0,332,0,337,0,338,0,339,0,340,0]},{"source":"dart:core/runtime/libdate_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibdate_patch.dart","uri":"dart:core/runtime/libdate_patch.dart","_kind":"library"},"hits":[36,0,39,0,43,0,48,0,51,0,53,0,54,0,58,0,60,0,12,0,14,0,17,0,20,0,82,0,97,0,99,0,100,0,101,0,102,0,103,0,104,0,105,0,106,0,107,0,108,0,109,0,110,0,111,0,112,0,113,0,115,0,116,0,118,0,120,0,121,0,124,0,126,0,128,0,131,0,135,0,136,0,139,0,140,0,145,0,146,0,149,0,150,0,151,0,152,0,153,0,154,0,155,0,156,0,157,0,158,0,162,0,163,0,164,0,166,0,235,0,236,0,237,0,239,0,240,0,243,0,244,0,249,0,250,0,251,0,252,0,253,0,256,0,258,0,338,0,340,0,351,0,363,0,367,0,376,0,381,0,382,0,384,0,386,0,387,0,402,0,405,0,408,0,409,0,410,0,411,0,412,0,413,0,414,0,419,0,420,0,421,0,424,0,425,0,426,0,170,0,171,0,172,0,176,0,177,0,178,0,182,0,183,0,263,0,266,0,268,0,269,0,270,0,271,0,272,0,273,0,279,0,280,0,281,0,282,0,283,0,284,0,285,0,286,0,294,0,295,0,310,0,318,0,320,0,326,0,331,0,332,0,187,0,188,0,191,0,63,0,64,0,65,0,69,0,70,0,71,0,72,0,218,0,215,0,209,0,206,0,203,0,200,0,197,0,194,0,212,0]},{"source":"dart:core/runtime/libfunction_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibfunction_patch.dart","uri":"dart:core/runtime/libfunction_patch.dart","_kind":"library"},"hits":[10,0,13,0,15,0,16,0,17,0,18,0,19,0,20,0,21,0,22,0,25,0,26,0,31,0,27,0,28,0]},{"source":"dart:core/runtime/libnull_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibnull_patch.dart","uri":"dart:core/runtime/libnull_patch.dart","_kind":"library"},"hits":[14,0,12,0]},{"source":"dart:core/null.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fnull.dart","uri":"dart:core/null.dart","_kind":"library"},"hits":[15,0,16,0,22,0]},{"source":"dart:core/runtime/libidentical_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fruntime%2Flibidentical_patch.dart","uri":"dart:core/runtime/libidentical_patch.dart","_kind":"library"},"hits":[8,0,11,0]},{"source":"dart:core/print.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acore%2Fprint.dart","uri":"dart:core/print.dart","_kind":"library"},"hits":[8,1,9,1,11,1,13,0]},{"source":"dart:isolate/runtime/libisolate_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aisolate%2Fruntime%2Flibisolate_patch.dart","uri":"dart:isolate/runtime/libisolate_patch.dart","_kind":"library"},"hits":[193,1,194,1,197,0,198,0,201,0,202,0,206,0,207,0,210,0,34,0,130,0,132,1,134,3,137,1,138,1,141,0,142,0,143,0,146,0,147,0,151,0,152,0,155,1,156,2,161,1,165,1,166,1,170,0,172,1,173,3,178,1,180,1,182,1,183,1,185,1,70,0,72,0,73,0,74,0,77,0,78,0,81,0,83,0,87,0,88,0,89,0,23,0,26,0,27,0,62,1,63,1,64,1,324,0,459,0,460,0,461,0,480,0,496,0,508,0,522,0,612,0,613,0,614,0,615,0,616,0,619,0,622,0,624,0,630,0,295,0,298,0,301,0,303,0,307,0,310,0,312,0,316,0,319,0,321,0,525,0,526,0,527,0,528,0,529,0,530,0,531,0,535,0,536,0,537,0,538,0,539,0,540,0,541,0,545,0,546,0,547,0,548,0,549,0,550,0,551,0,555,0,556,0,557,0,558,0,559,0,560,0,564,0,565,0,566,0,567,0,568,0,569,0,570,0,574,0,575,0,576,0,577,0,578,0,579,0,580,0,584,0,585,0,586,0,587,0,588,0,589,0,590,0,591,0,595,0,596,0,597,0,598,0,599,0,600,0,604,0,605,0,606,0,607,0,608,0,609,0,38,0,40,0,41,0,44,0,45,0,48,0,49,0,103,0,109,1,113,0,117,0,125,1,222,1,223,1,237,1,269,1,270,2,286,2,247,0,255,0,256,0,257,0,262,0,271,1,274,1,276,1,279,1,275,0,277,0,282,0,462,0,463,0,464,0,465,0,466,0,467,0,468,0,469,0,471,0,472,0,475,0,330,0,340,0,341,0,344,0,349,0,353,0,355,0,356,0,359,0,361,0,364,0,366,0,371,0,383,0,389,0,394,0,400,0,406,0,412,0,413,0,414,0,426,0,427,0,432,0,433,0,434,0,436,0,437,0,438,0,450,0,453,0]},{"source":"dart:isolate/runtime/libtimer_impl.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aisolate%2Fruntime%2Flibtimer_impl.dart","uri":"dart:isolate/runtime/libtimer_impl.dart","_kind":"library"},"hits":[159,0,161,0,153,0,155,0,163,0,166,0,173,0,174,0,177,0,180,0,184,0,185,0,188,0,189,0,192,0,194,0,195,0,196,0,197,0,200,0,202,0,207,0,208,0,211,0,212,0,213,0,215,0,219,0,222,0,223,0,224,0,226,0,232,0,233,0,238,0,242,0,244,0,245,0,246,0,253,0,255,0,257,0,262,0,263,0,268,0,269,0,270,0,274,0,275,0,276,0,280,0,289,0,293,0,294,0,297,0,299,0,305,0,307,0,308,0,312,0,313,0,322,0,323,0,324,0,329,0,331,0,332,0,333,0,339,0,342,0,347,0,357,0,359,0,360,0,365,0,366,0,367,0,369,0,370,0,371,0,373,0,374,0,375,0,376,0,377,0,380,0,382,0,384,0,385,0,386,0,389,0,391,0,398,0,399,0,400,0,402,0,406,0,408,0,409,0,414,0,416,0,419,0,423,0,425,0,427,0,432,0,434,0,440,0,443,0,444,0,448,0,449,0,456,0,459,0,461,0,19,0,21,0,23,0,25,0,27,0,28,0,29,0,31,0,32,0,33,0,36,0,37,0,38,0,42,0,43,0,44,0,45,0,46,0,49,0,51,0,52,0,53,0,54,0,56,0,59,0,60,0,63,0,64,0,65,0,66,0,69,0,70,0,71,0,72,0,73,0,80,0,82,0,83,0,85,0,86,0,88,0,89,0,95,0,99,0,100,0,101,0,102,0,103,0,104,0,107,0,108,0,109,0,110,0,112,0,113,0,114,0,465,1]},{"source":"dart:isolate","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aisolate","uri":"dart:isolate","_kind":"library"},"hits":[142,0,356,0,357,0,358,0,556,0,566,0,577,0,29,0,30,0,728,0,730,0,731,0,559,0,560,0,561,0,562,0,563,0,568,0,569,0,570,0,572,0,573,0,574,0]},{"source":"dart:mirrors/runtime/libmirrors_impl.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Amirrors%2Fruntime%2Flibmirrors_impl.dart","uri":"dart:mirrors/runtime/libmirrors_impl.dart","_kind":"library"},"hits":[1124,0,1132,0,1133,0,1134,0,1135,0,1136,0,1138,0,1140,0,1141,0,1142,0,1144,0,1146,0,1151,0,1152,0,1153,0,1155,0,1156,0,1161,0,1164,0,1353,0,1375,0,1376,0,1379,0,1380,0,1382,0,1385,0,1387,0,1388,0,1391,0,1392,0,1393,0,1394,0,1398,0,1399,0,1400,0,1401,0,1403,0,1406,0,1408,0,11,0,12,0,1171,0,1173,0,1175,0,211,0,213,0,215,0,216,0,217,0,218,0,219,0,220,0,223,0,224,0,230,0,233,0,234,0,237,0,238,0,239,0,242,0,243,0,245,0,246,0,247,0,249,0,250,0,252,0,253,0,254,0,255,0,256,0,936,0,938,0,940,0,943,0,944,0,945,0,946,0,948,0,952,0,953,0,954,0,955,0,957,0,960,0,961,0,962,0,963,0,966,0,969,0,971,0,972,0,975,0,980,0,981,0,982,0,983,0,984,0,986,0,987,0,988,0,991,0,995,0,996,0,997,0,998,0,1000,0,1001,0,1002,0,1005,0,1008,0,1010,0,1011,0,1012,0,1013,0,1014,0,1017,0,1018,0,1019,0,1020,0,1021,0,1022,0,1025,0,1028,0,339,0,342,0,343,0,344,0,346,0,349,0,351,0,354,0,356,0,149,0,152,0,153,0,154,0,155,0,156,0,157,0,158,0,159,0,160,0,161,0,163,0,164,0,166,0,167,0,169,0,170,0,171,0,172,0,173,0,176,0,177,0,178,0,827,0,829,0,832,0,833,0,834,0,836,0,839,0,841,0,842,0,845,0,848,0,849,0,852,0,853,0,854,0,857,0,103,0,104,0,112,0,113,0,1462,0,1463,0,1467,0,1468,0,1469,0,1470,0,1473,0,1475,0,1477,0,1484,0,1485,0,1487,0,1488,0,1489,0,1490,0,1496,0,1498,0,1500,0,1502,0,1503,0,1504,0,1505,0,1511,0,1512,0,1513,0,1516,0,761,0,765,0,769,0,770,0,771,0,773,0,777,0,778,0,779,0,781,0,785,0,786,0,787,0,788,0,790,0,794,0,795,0,796,0,797,0,798,0,800,0,803,0,804,0,805,0,806,0,807,0,808,0,810,0,812,0,815,0,818,0,1416,0,1418,0,1419,0,1421,0,1423,0,1424,0,1426,0,1427,0,1428,0,1429,0,1432,0,1433,0,1435,0,1436,0,1438,0,1440,0,1441,0,1444,0,1447,0,1449,0,1451,0,1452,0,1455,0,1456,0,265,0,268,0,269,0,272,0,274,0,278,0,280,0,282,0,284,0,285,0,286,0,289,0,292,0,295,0,296,0,299,0,300,0,301,0,305,0,307,0,308,0,309,0,310,0,311,0,312,0,313,0,316,0,317,0,323,0,326,0,329,0,331,0,334,0,862,0,863,0,866,0,867,0,868,0,869,0,871,0,874,0,875,0,878,0,879,0,880,0,882,0,885,0,886,0,887,0,890,0,892,0,893,0,895,0,896,0,898,0,900,0,901,0,902,0,903,0,906,0,908,0,909,0,910,0,911,0,912,0,915,0,916,0,917,0,918,0,919,0,920,0,923,0,926,0,123,0,125,0,127,0,138,0,384,0,395,0,400,0,401,0,402,0,403,0,406,0,409,0,411,0,412,0,414,0,418,0,419,0,420,0,422,0,425,0,426,0,427,0,428,0,430,0,433,0,435,0,437,0,438,0,442,0,443,0,444,0,445,0,446,0,451,0,452,0,454,0,457,0,458,0,462,0,463,0,464,0,465,0,466,0,467,0,468,0,470,0,471,0,472,0,474,0,475,0,477,0,480,0,481,0,483,0,484,0,485,0,487,0,488,0,489,0,493,0,494,0,495,0,496,0,497,0,498,0,501,0,504,0,506,0,510,0,514,0,515,0,516,0,517,0,532,0,533,0,535,0,539,0,540,0,541,0,542,0,543,0,545,0,563,0,564,0,566,0,570,0,571,0,573,0,575,0,576,0,577,0,578,0,579,0,582,0,583,0,584,0,585,0,586,0,589,0,590,0,593,0,594,0,598,0,599,0,600,0,605,0,606,0,607,0,608,0,610,0,612,0,613,0,615,0,616,0,617,0,619,0,620,0,622,0,626,0,627,0,628,0,629,0,630,0,632,0,633,0,636,0,639,0,641,0,642,0,645,0,649,0,651,0,655,0,656,0,657,0,658,0,659,0,660,0,663,0,664,0,670,0,671,0,674,0,677,0,678,0,681,0,682,0,683,0,684,0,685,0,688,0,690,0,691,0,692,0,693,0,694,0,697,0,698,0,699,0,700,0,701,0,702,0,705,0,706,0,707,0,710,0,711,0,712,0,717,0,719,0,721,0,724,0,727,0,730,0,732,0,735,0,738,0,741,0,744,0,746,0,748,0,751,0,754,0,75,0,76,0,77,0,78,0,79,0,81,0,84,0,87,0,90,0,91,0,92,0,94,0,97,0,99,0,1041,0,1042,0,1043,0,1047,0,1049,0,1051,0,1052,0,1054,0,1057,0,1058,0,1060,0,1061,0,1062,0,1063,0,1066,0,1067,0,1070,0,1071,0,1074,0,1077,0,1078,0,1081,0,1082,0,1083,0,1086,0,1088,0,1091,0,1092,0,1093,0,1094,0,1095,0,1097,0,1100,0,1103,0,1106,0,1108,0,1111,0,1184,0,1186,0,1199,0,1200,0,1201,0,1202,0,1203,0,1204,0,1205,0,1206,0,1207,0,1208,0,1209,0,1216,0,1219,0,1222,0,1223,0,1225,0,1228,0,1229,0,1231,0,1232,0,1235,0,1236,0,1237,0,1238,0,1240,0,1241,0,1244,0,1248,0,1249,0,1250,0,1251,0,1253,0,1256,0,1259,0,1260,0,1261,0,1262,0,1264,0,1265,0,1266,0,1268,0,1269,0,1270,0,1271,0,1273,0,1277,0,1280,0,1282,0,1283,0,1284,0,1285,0,1287,0,1291,0,1293,0,1296,0,1299,0,1302,0,185,0,187,0,188,0,189,0,191,0,192,0,193,0,194,0,195,0,196,0,197,0,198,0,199,0,200,0,201,0,1312,0,1314,0,1316,0,1318,0,1319,0,1320,0,1321,0,1322,0,1323,0,1324,0,1325,0,1327,0,1332,0,1333,0,1334,0,1336,0,1339,0,1340,0,1342,0,15,0,17,0,19,0,22,0,24,0,27,0,29,0,30,0,34,0,35,0,36,0,37,0,40,0,41,0,44,0,45,0,46,0,48,0,49,0,50,0,54,0,57,0,59,0,60,0,61,0,64,0,66,0,68,0,1032,0,1033,0,1034,0,1157,0,225,0,226,0,318,0,319,0,129,0,130,0,133,0,518,0,519,0,521,0,522,0,523,0,524,0,525,0,526,0,527,0,546,0,547,0,548,0,549,0,550,0,552,0,553,0,554,0,556,0,557,0,558,0,665,0,666,0]},{"source":"dart:mirrors/runtime/libmirror_reference.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Amirrors%2Fruntime%2Flibmirror_reference.dart","uri":"dart:mirrors/runtime/libmirror_reference.dart","_kind":"library"},"hits":[8,0,9,0,12,0]},{"source":"dart:mirrors/runtime/libmirrors_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Amirrors%2Fruntime%2Flibmirrors_patch.dart","uri":"dart:mirrors/runtime/libmirrors_patch.dart","_kind":"library"},"hits":[91,0,60,0,62,0,63,0,64,0,66,0,67,0,68,0,69,0,71,0,75,0,76,0,80,0,81,0,82,0,83,0,86,0,88,0,26,0,27,0,37,0,38,0,48,0,49,0,53,0,54,0]},{"source":"dart:mirrors","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Amirrors","uri":"dart:mirrors","_kind":"library"},"hits":[1465,0,1214,0]},{"source":"dart:collection/maps.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fmaps.dart","uri":"dart:collection/maps.dart","_kind":"library"},"hits":[121,0,122,0,123,0,124,0,128,0,129,0,130,0,134,0,135,0,136,0,141,0,142,0,143,0,145,0,148,0,149,0,150,0,153,0,155,0,158,0,159,0,160,0,164,0,165,0,168,0,169,0,170,0,171,0,172,0,177,0,178,0,179,0,183,0,184,0,185,0,186,0,188,0,189,0,193,0,194,0,195,0,196,0,197,0,198,0,255,0,257,0,259,0,260,0,261,0,264,0,268,0,276,0,277,0,281,0,282,0,286,0,287,0,291,0,292,0,296,0,297,0,232,0,234,0,235,0,236,0,237,0,238,0,239,0,241,0,311,0,313,0,314,0,315,0,316,0,319,0,320,0,323,0,324,0,327,0,328,0,329,0,330,0,331,0,334,0,335,0,336,0,337,0,338,0,339,0,340,0,342,0,344,0,345,0,348,0,349,0,351,0,352,0,354,0,355,0,358,0,359,0,372,0,374,0,375,0,24,0,26,0,30,0,32,0,33,0,35,0,44,0,47,0,50,0,53,0,61,0,66,0,67,0,77,0,78,0,79,0,81,0,82,0,85,0,86,0,87,0,91,0,37,0,40,0,41,0,42,0]},{"source":"dart:collection/runtime/libcompact_hash.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fruntime%2Flibcompact_hash.dart","uri":"dart:collection/runtime/libcompact_hash.dart","_kind":"library"},"hits":[155,0,156,0,157,0,159,0,160,0,163,0,166,0,170,0,171,0,172,0,177,0,180,0,181,0,182,0,183,0,184,0,186,0,187,0,188,0,190,0,197,0,198,0,200,0,201,0,202,0,203,0,204,0,208,0,209,0,210,0,211,0,214,0,216,0,217,0,218,0,224,0,225,0,226,0,227,0,229,0,230,0,231,0,232,0,236,0,237,0,238,0,239,0,240,0,244,0,245,0,247,0,250,0,251,0,252,0,253,0,254,0,255,0,256,0,257,0,259,0,260,0,264,0,265,0,266,0,267,0,268,0,269,0,270,0,271,0,272,0,275,0,276,0,277,0,278,0,279,0,281,0,282,0,287,0,288,0,289,0,290,0,291,0,292,0,293,0,294,0,295,0,296,0,297,0,298,0,299,0,300,0,301,0,302,0,303,0,304,0,305,0,310,0,311,0,317,0,318,0,319,0,320,0,321,0,322,0,323,0,324,0,325,0,326,0,327,0,328,0,329,0,330,0,331,0,335,0,336,0,338,0,341,0,343,0,344,0,345,0,348,0,349,0,351,0,358,0,359,0,360,0,361,0,362,0,363,0,367,0,368,0,369,0,370,0,140,0,141,0,142,0,143,0,144,0,145,0,616,0,618,0,620,0,457,0,461,0,463,0,464,0,466,0,467,0,468,0,469,0,473,0,476,0,477,0,478,0,479,0,483,0,486,0,487,0,488,0,490,0,494,0,495,0,496,0,500,0,501,0,502,0,503,0,504,0,505,0,507,0,508,0,509,0,510,0,516,0,517,0,518,0,519,0,520,0,521,0,522,0,524,0,525,0,526,0,527,0,531,0,532,0,536,0,537,0,539,0,540,0,541,0,543,0,546,0,547,0,553,0,554,0,555,0,556,0,557,0,558,0,559,0,560,0,561,0,562,0,563,0,564,0,565,0,568,0,569,0,571,0,574,0,575,0,576,0,579,0,581,0,582,0,583,0,584,0,585,0,586,0,587,0,588,0,589,0,590,0,591,0,592,0,593,0,594,0,595,0,599,0,600,0,605,0,606,0,611,0,84,0,85,0,86,0,87,0,88,0,91,0,92,0,94,0,98,0,99,0,101,0,104,0,107,0,109,0,110,0,115,0,116,0,117,0,123,0,124,0,43,0,128,0,129,0,48,0,49,0,51,0,52,0,54,0,55,0,57,0,58,0,60,0,61,0,398,0,399,0,391,0,392,0,394,0,395,0,396,0,432,0,434,0,436,0,437,0,438,0,441,0,442,0,443,0,444,0,447,0,635,0,636,0,628,0,629,0,631,0,632,0,633,0,638,0,639,0,640,0,641,0,380,0,412,0,415,0,416,0,418,0,419,0,420,0,11,0,12,0,13,0,14,0]},{"source":"dart:collection/set.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fset.dart","uri":"dart:collection/set.dart","_kind":"library"},"hits":[318,0,319,0,46,0,48,0,50,0,51,0,52,0,57,0,58,0,60,0,61,0,64,0,65,0,68,0,69,0,72,0,75,0,76,0,77,0,79,0,82,0,83,0,84,0,85,0,87,0,90,0,91,0,92,0,93,0,95,0,98,0,99,0,100,0,105,0,106,0,109,0,110,0,111,0,112,0,117,0,118,0,119,0,120,0,125,0,126,0,128,0,132,0,133,0,135,0,136,0,137,0,138,0,139,0,143,0,148,0,150,0,151,0,153,0,154,0,157,0,158,0,159,0,160,0,162,0,163,0,164,0,169,0,171,0,175,0,176,0,177,0,182,0,183,0,184,0,185,0,186,0,188,0,189,0,191,0,192,0,193,0,194,0,197,0,200,0,201,0,202,0,207,0,208,0,211,0,212,0,215,0,216,0,219,0,220,0,223,0,224,0,225,0,226,0,228,0,231,0,232,0,233,0,234,0,238,0,239,0,243,0,244,0,245,0,247,0,248,0,251,0,254,0,255,0,261,0,262,0,265,0,268,0,269,0,271,0,278,0,279,0,282,0,283,0,284,0,286,0,287,0,288,0,290,0]},{"source":"dart:collection/queue.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fqueue.dart","uri":"dart:collection/queue.dart","_kind":"library"},"hits":[148,0,149,0,150,0,151,0,152,0,155,0,156,0,157,0,158,0,159,0,171,0,174,0,175,0,179,0,180,0,183,0,184,0,185,0,189,0,192,0,277,0,278,0,279,0,282,0,287,0,288,0,292,0,293,0,943,0,945,0,946,0,947,0,949,0,951,0,952,0,953,0,954,0,957,0,958,0,306,0,307,0,325,0,326,0,327,0,328,0,339,0,340,0,342,0,343,0,345,0,346,0,347,0,350,0,351,0,352,0,355,0,356,0,357,0,360,0,361,0,362,0,363,0,367,0,368,0,369,0,370,0,374,0,375,0,376,0,377,0,381,0,382,0,383,0,384,0,385,0,387,0,390,0,391,0,394,0,399,0,400,0,401,0,402,0,403,0,405,0,407,0,409,0,410,0,416,0,417,0,420,0,421,0,424,0,425,0,426,0,429,0,430,0,431,0,434,0,437,0,438,0,439,0,441,0,454,0,455,0,468,0,469,0,472,0,473,0,476,0,477,0,478,0,479,0,500,0,501,0,502,0,504,0,509,0,510,0,511,0,512,0,513,0,519,0,520,0,523,0,205,0,209,0,210,0,213,0,214,0,219,0,221,0,222,0,223,0,226,0,227,0,228,0,576,0,579,0,581,0,582,0,585,0,603,0,604,0,605,0,606,0,608,0,609,0,611,0,615,0,616,0,618,0,619,0,620,0,632,0,633,0,637,0,638,0,640,0,641,0,642,0,643,0,644,0,648,0,650,0,652,0,653,0,654,0,657,0,658,0,659,0,662,0,663,0,664,0,665,0,668,0,669,0,670,0,673,0,676,0,678,0,680,0,686,0,687,0,690,0,691,0,693,0,694,0,695,0,696,0,698,0,699,0,702,0,703,0,704,0,705,0,707,0,708,0,709,0,710,0,713,0,715,0,719,0,720,0,721,0,722,0,723,0,724,0,731,0,732,0,733,0,734,0,735,0,736,0,737,0,739,0,740,0,742,0,753,0,754,0,763,0,764,0,767,0,768,0,769,0,770,0,772,0,773,0,777,0,781,0,782,0,785,0,786,0,787,0,788,0,789,0,792,0,793,0,794,0,795,0,796,0,797,0,801,0,802,0,803,0,804,0,805,0,806,0,817,0,826,0,828,0,830,0,831,0,837,0,838,0,839,0,844,0,845,0,846,0,847,0,848,0,861,0,862,0,863,0,864,0,865,0,868,0,869,0,870,0,873,0,874,0,875,0,877,0,879,0,880,0,881,0,884,0,892,0,893,0,894,0,895,0,896,0,897,0,898,0,899,0,902,0,904,0,905,0,906,0,909,0,910,0,911,0,912,0,917,0,922,0,923,0,924,0,925,0,926,0,927,0,531,0,533,0,535,0,536,0,537,0,538,0,539,0,542,0,543,0,544,0,546,0,547,0,551,0,239,0,242,0,243,0,244,0,247,0,248,0,249,0,252,0,253,0,254,0,255,0,258,0,259,0,260,0,263,0,65,0,66,0]},{"source":"dart:collection/runtime/libcollection_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fruntime%2Flibcollection_patch.dart","uri":"dart:collection/runtime/libcollection_patch.dart","_kind":"library"},"hits":[464,0,465,0,466,0,467,0,468,0,904,0,911,0,917,0,931,0,935,0,444,0,445,0,446,0,447,0,813,0,815,0,816,0,817,0,345,0,346,0,347,0,348,0,349,0,351,0,352,0,357,0,358,0,359,0,360,0,361,0,363,0,364,0,366,0,371,0,372,0,373,0,374,0,375,0,376,0,378,0,379,0,382,0,384,0,387,0,388,0,389,0,390,0,391,0,392,0,394,0,395,0,397,0,399,0,400,0,401,0,402,0,404,0,409,0,410,0,411,0,412,0,413,0,416,0,417,0,418,0,419,0,420,0,421,0,422,0,430,0,481,0,483,0,484,0,485,0,487,0,489,0,491,0,494,0,496,0,497,0,498,0,499,0,501,0,502,0,506,0,22,1,29,1,35,0,49,0,53,0,438,1,758,0,759,0,761,0,762,0,829,0,831,0,832,0,833,0,835,0,836,0,837,0,840,0,841,0,842,0,843,0,844,0,845,0,846,0,850,0,854,0,68,0,69,0,70,0,72,0,73,0,75,0,76,0,77,0,78,0,79,0,81,0,82,0,87,0,88,0,89,0,90,0,91,0,93,0,94,0,100,1,101,1,102,1,103,3,104,1,106,4,107,1,109,0,114,1,115,1,116,1,117,1,118,2,119,1,121,4,122,1,127,1,125,0,130,0,131,0,132,0,133,0,134,0,135,0,137,0,138,0,140,0,142,0,143,0,144,0,145,0,147,0,152,0,153,0,158,0,159,0,160,0,161,0,162,0,163,0,165,0,166,0,167,0,169,0,174,1,175,1,176,1,177,3,178,1,181,1,182,4,183,1,184,2,185,1,186,3,187,1,195,0,196,0,197,0,198,0,199,0,203,1,206,3,208,0,212,1,214,2,215,1,216,2,217,1,220,4,221,4,224,0,225,0,226,0,227,0,228,0,229,0,230,0,232,0,233,0,234,0,235,0,236,0,240,0,243,0,250,0,251,0,253,0,254,0,255,0,256,0,257,0,258,0,260,0,261,0,266,0,267,0,268,0,269,0,270,0,271,0,273,0,274,0,276,0,281,0,282,0,283,0,284,0,285,0,286,0,288,0,289,0,292,0,294,0,297,0,298,0,299,0,300,0,301,0,302,0,304,0,305,0,307,0,309,0,310,0,311,0,312,0,314,0,319,0,320,0,321,0,322,0,323,0,324,0,327,0,328,0,329,0,330,0,331,0,332,0,333,0,341,0,517,0,518,0,524,0,531,0,537,0,551,0,555,0,769,0,770,0,772,0,773,0,774,0,777,0,778,0,779,0,782,0,783,0,784,0,787,0,788,0,789,0,794,0,795,0,796,0,797,0,802,0,803,0,805,0,806,0,566,0,567,0,569,0,573,0,575,0,577,0,579,0,581,0,582,0,583,0,585,0,586,0,591,0,592,0,593,0,595,0,596,0,597,0,602,0,603,0,604,0,606,0,609,0,612,0,613,0,614,0,616,0,617,0,619,0,622,0,627,0,628,0,629,0,630,0,632,0,633,0,635,0,639,0,641,0,642,0,643,0,647,0,648,0,649,0,652,0,653,0,655,0,657,0,659,0,660,0,661,0,665,0,670,0,672,0,673,0,674,0,678,0,679,0,680,0,681,0,684,0,685,0,686,0,687,0,689,0,690,0,692,0,694,0,696,0,697,0,698,0,702,0,708,0,709,0,712,0,713,0,716,0,717,0,718,0,719,0,720,0,724,0,725,0,726,0,727,0,728,0,731,0,732,0,735,0,736,0,737,0,738,0,739,0,740,0,741,0,743,0,744,0,745,0,746,0,750,0,753,0,754,0,512,0,513,0,867,0,874,0,880,0,894,0,898,0,451,0,452,0,453,0,454,0,455,0,460,0,469,0,154,0,456,0]},{"source":"dart:collection/splay_tree.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fsplay_tree.dart","uri":"dart:collection/splay_tree.dart","_kind":"library"},"hits":[86,0,87,0,93,0,94,0,95,0,98,0,99,0,100,0,101,0,102,0,104,0,105,0,106,0,108,0,111,0,113,0,114,0,115,0,116,0,117,0,119,0,120,0,121,0,123,0,126,0,128,0,134,0,135,0,136,0,137,0,138,0,140,0,141,0,142,0,150,0,152,0,153,0,154,0,155,0,166,0,168,0,169,0,170,0,171,0,177,0,178,0,179,0,180,0,181,0,182,0,184,0,185,0,187,0,189,0,192,0,194,0,204,0,205,0,206,0,207,0,208,0,212,0,213,0,214,0,215,0,217,0,218,0,219,0,221,0,224,0,225,0,226,0,227,0,230,0,231,0,232,0,233,0,236,0,237,0,238,0,239,0,244,0,28,0,554,0,556,0,557,0,558,0,561,0,563,0,564,0,565,0,566,0,567,0,569,0,571,0,575,0,576,0,577,0,580,0,582,0,583,0,595,0,597,0,599,0,601,0,602,0,607,0,608,0,609,0,616,0,617,0,620,0,621,0,623,0,624,0,649,0,650,0,651,0,652,0,725,0,726,0,744,0,746,0,747,0,749,0,761,0,763,0,765,0,766,0,768,0,769,0,773,0,775,0,776,0,777,0,779,0,780,0,781,0,784,0,785,0,786,0,789,0,790,0,791,0,792,0,796,0,797,0,800,0,801,0,802,0,803,0,807,0,808,0,809,0,812,0,813,0,814,0,815,0,816,0,821,0,822,0,823,0,827,0,829,0,830,0,831,0,832,0,834,0,837,0,838,0,842,0,843,0,844,0,845,0,849,0,850,0,851,0,852,0,853,0,856,0,857,0,858,0,859,0,864,0,865,0,866,0,867,0,872,0,873,0,876,0,877,0,878,0,879,0,885,0,887,0,888,0,889,0,892,0,893,0,896,0,898,0,18,0,633,0,634,0,635,0,636,0,638,0,640,0,641,0,642,0,292,0,293,0,361,0,302,0,304,0,305,0,314,0,316,0,331,0,336,0,337,0,352,0,354,0,355,0,359,0,363,0,364,0,365,0,366,0,367,0,368,0,374,0,375,0,376,0,377,0,381,0,382,0,385,0,386,0,387,0,390,0,393,0,394,0,395,0,396,0,397,0,399,0,400,0,401,0,402,0,403,0,405,0,406,0,410,0,414,0,415,0,420,0,421,0,424,0,426,0,427,0,428,0,429,0,430,0,434,0,435,0,438,0,439,0,442,0,443,0,446,0,448,0,461,0,464,0,466,0,471,0,472,0,473,0,479,0,480,0,481,0,488,0,489,0,490,0,491,0,492,0,493,0,495,0,496,0,498,0,505,0,506,0,507,0,508,0,509,0,510,0,512,0,513,0,515,0,661,0,662,0,664,0,656,0,657,0,670,0,671,0,674,0,247,0,249,0,253,0,727,0,294,0,306,0,416,0,449,0,451,0,452,0,453,0,455,0,456,0]},{"source":"dart:collection/linked_hash_set.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Flinked_hash_set.dart","uri":"dart:collection/linked_hash_set.dart","_kind":"library"},"hits":[106,0,107,0,108,0,109,0,120,0,121,0]},{"source":"dart:collection/list.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Flist.dart","uri":"dart:collection/list.dart","_kind":"library"},"hits":[58,0,60,0,62,0,63,0,65,0,66,0,67,0,68,0,69,0,70,0,75,3,77,0,79,0,80,0,81,0,84,0,85,0,86,0,89,0,90,0,91,0,94,0,95,0,96,0,99,0,100,0,101,0,102,0,105,0,106,0,107,0,108,0,109,0,110,0,116,0,117,0,118,0,119,0,120,0,121,0,127,0,128,0,129,0,130,0,131,0,132,0,138,0,139,0,140,0,141,0,142,0,143,0,144,0,147,0,148,0,151,0,152,0,153,0,154,0,155,0,156,0,157,0,160,0,161,0,164,0,165,0,168,0,169,0,170,0,172,0,177,0,178,0,182,0,183,0,186,0,187,0,188,0,189,0,192,0,197,0,198,0,200,2,202,0,203,0,205,0,206,0,207,0,208,0,209,0,210,0,211,0,212,0,218,0,220,0,221,0,222,0,223,0,224,0,230,0,232,0,233,0,236,0,238,0,239,0,242,0,245,0,247,0,249,0,250,0,255,0,256,0,257,0,258,0,264,0,265,0,268,0,269,0,270,0,272,0,273,0,274,0,278,0,279,0,280,0,281,0,290,0,291,0,295,0,296,0,297,0,299,0,302,0,303,0,306,0,307,0,310,0,311,0,312,0,313,0,314,0,315,0,316,0,318,0,319,0,322,0,323,0,324,0,328,0,329,0,332,0,333,0,334,0,335,0,337,0,338,0,342,0,343,0,346,0,349,0,352,0,353,0,354,0,355,0,356,0,357,0,358,0,359,0,360,0,364,0,365,0,368,0,369,0,370,0,371,0,375,0,376,0,378,0,379,0,380,0,381,0,382,0,387,0,388,0,389,0,392,0,393,0,394,0,395,0,399,0,400,0,401,0,402,0,406,0,407,0,408,0,409,0,410,0,415,0,419,0,422,0,423,0,425,0,427,0,428,0,431,0,432,0,437,0,438,0,439,0,440,0,442,0,443,0,444,0,445,0,446,0,447,0,448,0,451,0,452,0,453,0,454,0,455,0,456,0,460,0,461,0,462,0,463,0,468,0,469,0,470,0,471,0,476,0,477,0,478,0,479,0,484,0,485,0,486,0,487,0,492,0,493,0,494,0,495,0,501,0,502,0,503,0,504,0,507,0,508,0,509,0,513,0,514,0,515,0,516,0,518,0,522,0,523,0,526,0,527,0,529,0,530,0,533,0,534,0,535,0,537,0,538,0,543,0,545,0,31,0,32,0]},{"source":"dart:collection/hash_set.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fhash_set.dart","uri":"dart:collection/hash_set.dart","_kind":"library"},"hits":[17,0,18,0,19,0,20,0,21,0,26,0,27,0,28,0,29,0,35,0,133,0,134,0,135,0,136,0,149,0,150,0]},{"source":"dart:collection/hash_map.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fhash_map.dart","uri":"dart:collection/hash_map.dart","_kind":"library"},"hits":[107,0,108,0,109,0,118,0,133,0,135,0,136,0,151,0,152,0,153,0,8,0,10,0,110,0]},{"source":"dart:collection/collections.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fcollections.dart","uri":"dart:collection/collections.dart","_kind":"library"},"hits":[22,0,24,0,25,0,27,0]},{"source":"dart:collection/linked_list.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Flinked_list.dart","uri":"dart:collection/linked_list.dart","_kind":"library"},"hits":[38,0,43,0,44,0,45,0,51,0,52,0,58,0,59,0,70,0,71,0,72,0,76,0,78,0,83,0,84,0,85,0,87,0,90,0,91,0,92,0,94,0,95,0,98,0,99,0,100,0,102,0,105,0,106,0,107,0,109,0,112,0,113,0,114,0,116,0,117,0,119,0,127,0,128,0,129,0,131,0,133,0,134,0,135,0,137,0,138,0,141,0,147,0,148,0,149,0,151,0,153,0,154,0,156,0,157,0,158,0,161,0,163,0,164,0,165,0,166,0,167,0,168,0,170,0,173,0,174,0,175,0,176,0,177,0,178,0,179,0,180,0,181,0,182,0,241,0,248,0,249,0,258,0,259,0,260,0,269,0,270,0,271,0,280,0,281,0,290,0,291,0,194,0,196,0,197,0,200,0,202,0,203,0,204,0,206,0,207,0,210,0,211,0,212,0]},{"source":"dart:collection/iterable.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fiterable.dart","uri":"dart:collection/iterable.dart","_kind":"library"},"hits":[226,0,236,0,238,0,239,0,243,0,245,0,246,0,248,0,251,0,253,0,254,0,255,0,256,0,271,0,273,0,274,0,276,0,277,0,279,0,282,0,284,0,285,0,18,0,19,0,21,0,26,0,27,0,29,0,30,0,32,0,36,0,37,0,39,0,42,0,43,0,44,0,49,0,50,0,53,0,54,0,55,0,56,0,58,0,59,0,60,0,65,0,67,0,71,0,72,0,73,0,78,0,79,0,80,0,81,0,82,0,84,0,85,0,87,0,88,0,89,0,90,0,93,0,96,0,97,0,98,0,103,0,104,0,106,0,108,0,111,0,112,0,113,0,118,0,120,0,122,0,123,0,126,0,127,0,130,0,131,0,134,0,135,0,138,0,139,0,140,0,141,0,143,0,146,0,147,0,148,0,149,0,153,0,154,0,158,0,159,0,160,0,161,0,162,0,166,0,167,0,168,0,170,0,171,0,174,0,177,0,178,0,184,0,185,0,188,0,191,0,192,0,194,0,201,0,202,0,205,0,206,0,207,0,209,0,210,0,211,0,213,0,216,0,293,0,294,0,295,0,303,0,328,0,331,0,332,0,333,0,334,0,335,0,336,0,346,0,347,0,348,0,349,0,351,0,352,0,353,0,354,0,355,0,358,0,359,0,360,0,362,0,363,0,366,0,368,0,369,0,370,0,378,0,379,0,380,0,381,0,383,0,387,0,388,0,389,0,396,0,398,0,404,0,405,0,408,0,412,0,414,0,415,0]},{"source":"dart:collection/iterator.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Fiterator.dart","uri":"dart:collection/iterator.dart","_kind":"library"},"hits":[21,0,23,0,24,0,25,0,28,0,31,0,33,0,34,0,38,0,39,0,40,0,42,0]},{"source":"dart:collection/linked_hash_map.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acollection%2Flinked_hash_map.dart","uri":"dart:collection/linked_hash_map.dart","_kind":"library"},"hits":[94,0,95,0,96,0,105,0,106,0,121,0,123,0,124,0,139,0,140,0,141,0,97,0]},{"source":"dart:developer/runtime/libprofiler.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Adeveloper%2Fruntime%2Flibprofiler.dart","uri":"dart:developer/runtime/libprofiler.dart","_kind":"library"},"hits":[18,0,19,0,20,0,10,0,11,0,14,0,25,0,27,0,24,0]},{"source":"dart:developer/service.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Adeveloper%2Fservice.dart","uri":"dart:developer/service.dart","_kind":"library"},"hits":[81,0,82,0,83,0,85,0,24,0,26,0,27,0,28,0,29,0,31,0,42,0,44,0,45,0,46,0,48,0,50,0,52,0,53,0,59,0,61,0,62,0,65,0,66,0,67,0,69,0,71,0,73,0,74,0]},{"source":"dart:developer/timeline.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Adeveloper%2Ftimeline.dart","uri":"dart:developer/timeline.dart","_kind":"library"},"hits":[51,0,58,0,59,0,67,0,74,0,275,0,278,0,279,0,280,0,284,0,285,0,286,0,187,0,191,0,192,0,193,0,199,0,203,0,204,0,206,0,207,0,208,0,210,0,211,0,215,0,219,0,220,0,223,0,224,0,226,0,227,0,231,0,235,0,236,0,239,0,240,0,245,0,246,0,247,0,251,0,310,0,314,0,316,0,317,0,318,0,319,0,320,0,324,0,325,0,102,0,106,0,107,0,109,0,111,0,114,0,115,0,116,0,118,0,119,0,121,0,125,0,129,0,130,0,133,0,139,0,143,0,147,0,148,0,150,0,155,0,156,0,158,0,159,0,164,0,166,0,168,0,170,0,176,0,329,0,330,0,334,0]},{"source":"dart:developer/profiler.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Adeveloper%2Fprofiler.dart","uri":"dart:developer/profiler.dart","_kind":"library"},"hits":[95,0,98,0,99,0,100,0,103,0,104,0,106,0,107,0,108,0,109,0,39,0,40,0,41,0,119,0,120,0,121,0,123,0,124,0,126,0,130,0,131,0,132,0,134,0,138,0,139,0,143,0,147,0,148,0,149,0,150,0,152,0,156,0,65,0,67,0,68,0,70,0,71,0,73,0,74,0,76,0,55,0,56,0,57,0,58,0,59,0,60,0,62,0,79,0,80,0,82,0,83,0,84,0,85,0,86,0,87,0]},{"source":"dart:developer/extension.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Adeveloper%2Fextension.dart","uri":"dart:developer/extension.dart","_kind":"library"},"hits":[20,0,24,0,25,0,35,0,39,0,40,0,41,0,42,0,74,0,75,0,76,0,82,0,83,0,84,0,86,0,89,0,92,0,96,0,99,0,100,0,101,0,105,0,106,0,107,0,108,0,136,0,137,0,138,0,140,0,141,0,143,0,144,0,146,0,147,0,150,0,155,0,156,0,157,0,159,0,160,0,162,0,163,0]},{"source":"dart:developer/runtime/libdeveloper.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Adeveloper%2Fruntime%2Flibdeveloper.dart","uri":"dart:developer/runtime/libdeveloper.dart","_kind":"library"},"hits":[55,0,71,0,79,0,80,0,81,0,85,0,87,0,88,0,90,0,93,0,94,0,97,0,100,0,105,0,114,0,121,0,126,0,129,0,133,0,134,0,135,0,137,0,139,0,142,0,144,0,146,0,147,0,148,0,150,0,152,0,21,0,24,0,27,0,35,0,36,0,39,0,41,0,42,0,45,0,47,0,49,0,59,0,63,0,67,0,162,0,165,0,156,0,159,0,169,0,102,0,103,0,108,0,109,0,113,0]},{"source":"dart:developer/runtime/libtimeline.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Adeveloper%2Fruntime%2Flibtimeline.dart","uri":"dart:developer/runtime/libtimeline.dart","_kind":"library"},"hits":[8,0,17,0,11,0,14,0,20,0,24,0,28,0,32,0]},{"source":"dart:typed_data/runtime/libtyped_data_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Atyped_data%2Fruntime%2Flibtyped_data_patch.dart","uri":"dart:typed_data/runtime/libtyped_data_patch.dart","_kind":"library"},"hits":[837,0,838,0,840,0,841,0,843,0,844,0,845,0,846,0,849,0,850,0,851,0,854,0,855,0,856,0,857,0,862,0,863,0,864,0,865,0,870,0,871,0,872,0,873,0,874,0,875,0,878,0,879,0,880,0,881,0,886,0,887,0,888,0,889,0,890,0,891,0,892,0,893,0,894,0,898,0,901,0,902,0,905,0,906,0,909,0,910,0,911,0,914,0,916,0,919,0,920,0,921,0,922,0,924,0,925,0,926,0,927,0,928,0,929,0,930,0,933,0,937,0,938,0,939,0,940,0,942,0,943,0,951,0,955,0,958,0,959,0,961,0,964,0,965,0,967,0,969,0,970,0,972,0,973,0,975,0,976,0,978,0,980,0,982,0,983,0,984,0,987,0,989,0,990,0,993,0,994,0,997,0,998,0,999,0,1000,0,1004,0,1005,0,1006,0,1008,0,1009,0,1010,0,1015,0,1016,0,1017,0,1018,0,1023,0,1024,0,1026,0,1027,0,1029,0,1030,0,1031,0,1032,0,1037,0,1038,0,1039,0,1040,0,1045,0,1046,0,1047,0,1048,0,1049,0,1051,0,1052,0,1055,0,1057,0,1058,0,1059,0,1060,0,1064,0,1065,0,1068,0,1071,0,1072,0,1073,0,1074,0,1076,0,1083,0,1084,0,1087,0,1088,0,1091,0,1092,0,1095,0,1096,0,1099,0,1100,0,1103,0,1104,0,1107,0,1111,0,1114,0,1115,0,1117,0,1120,0,1121,0,1126,0,1127,0,1128,0,1129,0,1132,0,1133,0,1138,0,1139,0,1142,0,1143,0,1146,0,1147,0,1150,0,1151,0,1154,0,1155,0,1156,0,1159,0,1160,0,1161,0,1164,0,1165,0,1166,0,1167,0,1170,0,1171,0,1172,0,1173,0,1174,0,1178,0,1179,0,1180,0,1183,0,1184,0,1185,0,1186,0,2224,0,2225,0,2226,0,2228,0,2231,0,2232,0,2233,0,2235,0,2239,0,2244,0,2245,0,1564,0,1565,0,1567,0,1568,0,1570,0,1571,0,1572,0,1573,0,1576,0,1577,0,1578,0,1581,0,1582,0,1583,0,1584,0,1589,0,1590,0,1591,0,1592,0,1597,0,1598,0,1599,0,1600,0,1601,0,1602,0,1605,0,1606,0,1607,0,1608,0,1613,0,1614,0,1615,0,1616,0,1617,0,1618,0,1619,0,1620,0,1621,0,1625,0,1628,0,1629,0,1632,0,1633,0,1636,0,1637,0,1638,0,1641,0,1643,0,1646,0,1647,0,1648,0,1649,0,1651,0,1652,0,1653,0,1654,0,1655,0,1656,0,1657,0,1660,0,1664,0,1665,0,1666,0,1667,0,1669,0,1670,0,1678,0,1682,0,1685,0,1686,0,1688,0,1691,0,1692,0,1694,0,1696,0,1697,0,1699,0,1700,0,1702,0,1703,0,1705,0,1707,0,1709,0,1710,0,1711,0,1714,0,1716,0,1717,0,1720,0,1721,0,1724,0,1725,0,1726,0,1727,0,1731,0,1732,0,1733,0,1735,0,1736,0,1737,0,1742,0,1743,0,1744,0,1745,0,1750,0,1751,0,1753,0,1754,0,1756,0,1757,0,1758,0,1759,0,1764,0,1765,0,1766,0,1767,0,1772,0,1773,0,1774,0,1775,0,1776,0,1778,0,1779,0,1782,0,1784,0,1785,0,1786,0,1787,0,1791,0,1792,0,1795,0,1798,0,1799,0,1800,0,1801,0,1803,0,1810,0,1811,0,1814,0,1815,0,1818,0,1819,0,1822,0,1823,0,1826,0,1827,0,1830,0,1831,0,1834,0,1838,0,1841,0,1842,0,1844,0,1847,0,1848,0,1853,0,1854,0,1855,0,1856,0,1859,0,1860,0,1865,0,1866,0,1869,0,1870,0,1873,0,1874,0,1877,0,1878,0,1881,0,1882,0,1883,0,1886,0,1887,0,1888,0,1891,0,1892,0,1893,0,1894,0,1897,0,1898,0,1899,0,1900,0,1901,0,1905,0,1906,0,1907,0,1910,0,1911,0,1912,0,1913,0,1201,0,1202,0,1204,0,1205,0,1207,0,1208,0,1209,0,1210,0,1213,0,1214,0,1215,0,1218,0,1219,0,1220,0,1221,0,1226,0,1227,0,1228,0,1229,0,1234,0,1235,0,1236,0,1237,0,1238,0,1239,0,1242,0,1243,0,1244,0,1245,0,1250,0,1251,0,1252,0,1253,0,1254,0,1255,0,1256,0,1257,0,1258,0,1262,0,1265,0,1266,0,1269,0,1270,0,1273,0,1274,0,1275,0,1278,0,1280,0,1283,0,1284,0,1285,0,1286,0,1288,0,1289,0,1290,0,1291,0,1292,0,1293,0,1294,0,1297,0,1301,0,1302,0,1303,0,1304,0,1306,0,1307,0,1315,0,1319,0,1322,0,1323,0,1325,0,1328,0,1329,0,1331,0,1333,0,1334,0,1336,0,1338,0,1339,0,1341,0,1343,0,1345,0,1346,0,1347,0,1350,0,1352,0,1353,0,1356,0,1357,0,1360,0,1361,0,1362,0,1363,0,1367,0,1368,0,1369,0,1371,0,1372,0,1373,0,1378,0,1379,0,1380,0,1381,0,1386,0,1387,0,1389,0,1390,0,1392,0,1393,0,1394,0,1395,0,1400,0,1401,0,1402,0,1403,0,1408,0,1409,0,1410,0,1411,0,1412,0,1414,0,1415,0,1418,0,1420,0,1421,0,1422,0,1423,0,1427,0,1428,0,1431,0,1434,0,1435,0,1436,0,1437,0,1439,0,1446,0,1447,0,1450,0,1451,0,1454,0,1455,0,1458,0,1459,0,1462,0,1463,0,1466,0,1467,0,1470,0,1474,0,1477,0,1478,0,1480,0,1483,0,1484,0,1489,0,1490,0,1491,0,1492,0,1495,0,1496,0,1501,0,1502,0,1505,0,1506,0,1509,0,1510,0,1513,0,1514,0,1517,0,1518,0,1519,0,1522,0,1523,0,1524,0,1527,0,1528,0,1529,0,1530,0,1533,0,1534,0,1535,0,1536,0,1537,0,1541,0,1542,0,1543,0,1546,0,1547,0,1548,0,1549,0,120,0,121,0,123,0,124,0,126,0,127,0,128,0,129,0,132,0,133,0,134,0,137,0,138,0,139,0,140,0,145,0,146,0,147,0,148,0,153,0,154,0,155,0,156,0,157,0,158,0,161,0,162,0,163,0,164,0,169,0,170,0,171,0,172,0,173,0,174,0,175,0,176,0,177,0,181,0,183,0,184,0,187,0,188,0,191,0,192,0,193,0,196,0,198,0,201,0,202,0,203,0,204,0,206,0,207,0,208,0,209,0,210,0,211,0,212,0,215,0,219,0,220,0,221,0,222,0,224,0,225,0,233,0,237,0,240,0,241,0,243,0,246,0,248,0,250,0,251,0,253,0,255,0,256,0,258,0,260,0,262,0,263,0,264,0,267,0,269,0,270,0,273,0,274,0,277,0,278,0,279,0,280,0,284,0,285,0,286,0,288,0,289,0,290,0,295,0,296,0,297,0,298,0,303,0,305,0,306,0,308,0,309,0,310,0,311,0,316,0,317,0,318,0,319,0,324,0,325,0,326,0,327,0,328,0,330,0,331,0,334,0,336,0,337,0,338,0,339,0,343,0,344,0,347,0,350,0,351,0,352,0,353,0,355,0,362,0,363,0,366,0,367,0,370,0,371,0,374,0,375,0,378,0,379,0,382,0,383,0,386,0,387,0,390,0,391,0,393,0,396,0,397,0,402,0,403,0,404,0,405,0,408,0,409,0,414,0,415,0,418,0,419,0,422,0,423,0,426,0,427,0,430,0,431,0,432,0,435,0,436,0,437,0,440,0,441,0,442,0,443,0,446,0,447,0,448,0,449,0,450,0,454,0,455,0,456,0,459,1,460,2,461,2,462,1,3675,0,3679,0,3681,0,3683,0,3684,0,3685,0,3689,0,3690,0,3691,0,3693,0,3694,0,3697,0,3698,0,3699,0,3701,0,3702,0,3705,0,3706,0,3707,0,3708,0,3709,0,3710,0,3712,0,3718,0,3724,0,3725,0,2569,0,2570,0,2571,0,2573,0,2576,0,2577,0,2578,0,2580,0,2584,0,2589,0,2590,0,2593,0,2594,0,2597,0,2598,0,3038,0,3039,0,3040,0,3042,0,3045,0,3046,0,3047,0,3049,0,3053,0,3058,0,3059,0,3062,0,3063,0,3066,0,3067,0,2556,0,2559,0,2560,0,2561,0,2605,0,2608,0,2609,0,2610,0,2310,0,2313,0,2314,0,2315,0,2750,0,2753,0,2754,0,2755,0,3461,0,3462,0,3468,0,3469,0,3472,0,3473,0,3319,0,3320,0,3321,0,3322,0,3323,0,3324,0,3325,0,3326,0,3327,0,3328,0,3329,0,3330,0,3331,0,3332,0,3334,0,3335,0,3336,0,3337,0,3338,0,3340,0,3341,0,3343,0,3344,0,3345,0,3346,0,3347,0,3348,0,3349,0,3350,0,3351,0,3152,0,3153,0,3154,0,3156,0,3159,0,3160,0,3161,0,3163,0,3167,0,3172,0,3173,0,3176,0,3177,0,3180,0,3181,0,2379,0,2380,0,2381,0,2383,0,2386,0,2387,0,2388,0,2390,0,2394,0,2399,0,2400,0,2403,0,2404,0,2407,0,2408,0,2762,0,2763,0,2764,0,2766,0,2769,0,2770,0,2771,0,2773,0,2777,0,2782,0,2783,0,2786,0,2787,0,2790,0,2791,0,477,0,478,0,480,0,481,0,483,0,484,0,485,0,486,0,489,0,490,0,491,0,494,0,495,0,496,0,497,0,502,0,503,0,504,0,505,0,510,0,511,0,512,0,513,0,514,0,515,0,518,0,519,0,520,0,521,0,526,0,527,0,528,0,529,0,530,0,531,0,532,0,533,0,534,0,538,0,541,0,542,0,545,0,546,0,549,0,550,0,551,0,554,0,556,0,559,0,560,0,561,0,562,0,564,0,565,0,566,0,567,0,568,0,569,0,570,0,573,0,577,0,578,0,579,0,580,0,582,0,583,0,591,0,595,0,598,0,599,0,601,0,604,0,605,0,607,0,609,0,610,0,612,0,614,0,615,0,617,0,619,0,621,0,622,0,623,0,626,0,628,0,629,0,632,0,633,0,636,0,637,0,638,0,639,0,643,0,644,0,645,0,647,0,648,0,649,0,654,0,655,0,656,0,657,0,662,0,663,0,665,0,666,0,668,0,669,0,670,0,671,0,676,0,677,0,678,0,679,0,684,0,685,0,686,0,687,0,688,0,690,0,691,0,694,0,696,0,697,0,698,0,699,0,703,0,704,0,707,0,710,0,711,0,712,0,713,0,715,0,722,0,723,0,726,0,727,0,730,0,731,0,734,0,735,0,738,0,739,0,742,0,743,0,746,0,747,0,750,0,751,0,753,0,756,0,757,0,762,0,763,0,764,0,765,0,768,0,769,0,774,0,775,0,778,0,779,0,782,0,783,0,786,0,787,0,790,0,791,0,792,0,795,0,796,0,797,0,800,0,801,0,802,0,803,0,806,0,807,0,808,0,809,0,810,0,814,0,815,0,816,0,819,0,820,0,821,0,822,0,2144,0,2145,0,2146,0,2148,0,2151,0,2152,0,2153,0,2155,0,2159,0,2164,0,2165,0,3868,0,3872,0,3874,0,3876,0,3877,0,3878,0,3882,0,3883,0,3884,0,3886,0,3887,0,3890,0,3891,0,3892,0,3894,0,3895,0,3899,0,3904,0,3905,0,2426,0,2427,0,2428,0,2430,0,2433,0,2434,0,2435,0,2437,0,2441,0,2446,0,2447,0,2450,0,2451,0,2454,0,2455,0,3618,0,3622,0,3624,0,3626,0,3627,0,3628,0,3632,0,3633,0,3634,0,3636,0,3637,0,3640,0,3641,0,3642,0,3644,0,3645,0,3648,0,3649,0,3650,0,3651,0,3652,0,3653,0,3655,0,3661,0,3666,0,3667,0,2133,0,2136,0,2137,0,2138,0,2654,0,2657,0,2658,0,2659,0,3573,0,3578,0,3580,0,3582,0,3583,0,3587,0,3588,0,3589,0,3591,0,3592,0,3595,0,3596,0,3597,0,3599,0,3600,0,3604,0,3609,0,3610,0,3913,0,3917,0,3919,0,3921,0,3922,0,3923,0,3927,0,3928,0,3929,0,3931,0,3932,0,3935,0,3936,0,3937,0,3939,0,3940,0,3944,0,3949,0,3950,0,2473,0,2474,0,2475,0,2477,0,2480,0,2481,0,2482,0,2484,0,2488,0,2493,0,2494,0,2497,0,2498,0,2501,0,2502,0,3733,0,3737,0,3739,0,3741,0,3742,0,3743,0,3747,0,3748,0,3749,0,3751,0,3752,0,3755,0,3756,0,3757,0,3759,0,3760,0,3764,0,3769,0,3770,0,2925,0,2926,0,2927,0,2929,0,2932,0,2933,0,2934,0,2936,0,2940,0,2945,0,2946,0,2949,0,2950,0,2953,0,2954,0,3301,0,3305,0,3308,0,3311,0,3315,0,4135,0,4139,0,4143,0,4144,0,4147,0,4148,0,4151,0,4152,0,4155,0,4161,0,4162,0,4163,0,4165,0,4168,0,4169,0,4170,0,4172,0,4175,0,4176,0,4177,0,4179,0,4182,0,4183,0,4184,0,4186,0,4189,0,4190,0,4191,0,4193,0,4194,0,4197,0,4200,0,4201,0,4202,0,4204,0,4205,0,4208,0,4209,0,4210,0,4212,0,4213,0,4216,0,4219,0,4220,0,4221,0,4223,0,4224,0,4227,0,4228,0,4229,0,4231,0,4232,0,4235,0,4238,0,4239,0,4240,0,4242,0,4243,0,4246,0,4247,0,4248,0,4250,0,4251,0,4254,0,4257,0,4258,0,4259,0,4261,0,4262,0,4265,0,4266,0,4267,0,4269,0,4270,0,4273,0,4276,0,4277,0,4278,0,4280,0,4281,0,4284,0,4285,0,4286,0,4288,0,4289,0,4292,0,4295,0,4296,0,4297,0,4299,0,4300,0,4303,0,4304,0,4305,0,4307,0,4308,0,4310,0,4311,0,4314,0,4315,0,4316,0,4318,0,4319,0,4322,0,4323,0,4326,0,4327,0,4328,0,4330,0,4331,0,4333,0,4334,0,4337,0,4338,0,4339,0,4341,0,4342,0,4345,0,4346,0,4349,0,4350,0,4351,0,4354,0,4357,0,4359,0,4360,0,4363,0,2520,0,2521,0,2522,0,2524,0,2527,0,2528,0,2529,0,2531,0,2535,0,2540,0,2541,0,2544,0,2545,0,2548,0,2549,0,2666,0,2667,0,2668,0,2670,0,2673,0,2674,0,2675,0,2677,0,2681,0,2686,0,2687,0,2690,0,2691,0,2694,0,2695,0,4048,0,4052,0,4054,0,4056,0,4057,0,4058,0,4062,0,4063,0,4064,0,4066,0,4067,0,4070,0,4071,0,4072,0,4074,0,4075,0,4079,0,4084,0,4085,0,2887,0,2888,0,2889,0,2891,0,2894,0,2895,0,2896,0,2898,0,2902,0,2907,0,2908,0,2911,0,2912,0,2915,0,2916,0,3529,0,3533,0,3535,0,3537,0,3538,0,3542,0,3543,0,3544,0,3546,0,3547,0,3550,0,3551,0,3552,0,3554,0,3555,0,3559,0,3564,0,3565,0,2252,0,2255,0,2256,0,2257,0,3823,0,3827,0,3829,0,3831,0,3832,0,3833,0,3837,0,3838,0,3839,0,3841,0,3842,0,3845,0,3846,0,3847,0,3849,0,3850,0,3854,0,3859,0,3860,0,3000,0,3001,0,3002,0,3004,0,3007,0,3008,0,3009,0,3011,0,3015,0,3020,0,3021,0,3024,0,3025,0,3028,0,3029,0,3357,0,3360,0,3364,0,3265,0,3266,0,3267,0,3269,0,3272,0,3273,0,3274,0,3276,0,3280,0,3285,0,3286,0,3289,0,3290,0,3293,0,3294,0,4003,0,4007,0,4009,0,4011,0,4012,0,4013,0,4017,0,4018,0,4019,0,4021,0,4022,0,4025,0,4026,0,4027,0,4029,0,4030,0,4034,0,4039,0,4040,0,3413,0,3414,0,3415,0,3416,0,3417,0,3418,0,3419,0,3420,0,3422,0,3423,0,3424,0,3425,0,3426,0,3427,0,3428,0,3429,0,3400,0,3403,0,3406,0,3409,0,4093,0,4097,0,4099,0,4101,0,4102,0,4103,0,4107,0,4108,0,4109,0,4111,0,4112,0,4115,0,4116,0,4117,0,4119,0,4120,0,4124,0,4129,0,4130,0,2183,0,2184,0,2185,0,2187,0,2190,0,2191,0,2192,0,2194,0,2198,0,2203,0,2204,0,2172,0,2175,0,2176,0,2177,0,3778,0,3782,0,3784,0,3786,0,3787,0,3788,0,3792,0,3793,0,3794,0,3796,0,3797,0,3800,0,3801,0,3802,0,3804,0,3805,0,3809,0,3814,0,3815,0,2962,0,2963,0,2964,0,2966,0,2969,0,2970,0,2971,0,2973,0,2977,0,2982,0,2983,0,2986,0,2987,0,2990,0,2991,0,62,0,63,0,64,0,65,0,68,0,69,0,72,0,76,0,77,0,80,0,81,0,84,0,85,0,88,0,89,0,92,0,93,0,97,0,106,0,2702,0,2705,0,2706,0,2707,0,2799,0,2800,0,2801,0,2803,0,2806,0,2807,0,2808,0,2810,0,2814,0,2819,0,2820,0,2618,0,2619,0,2620,0,2622,0,2625,0,2626,0,2627,0,2629,0,2633,0,2638,0,2639,0,2642,0,2643,0,2646,0,2647,0,2368,0,2371,0,2372,0,2373,0,2263,0,2264,0,2265,0,2267,0,2270,0,2271,0,2272,0,2274,0,2277,0,2278,0,2279,0,2280,0,2281,0,2282,0,2284,0,2289,0,2294,0,2295,0,2298,0,2299,0,2302,0,2303,0,3485,0,3489,0,3491,0,3493,0,3494,0,3498,0,3499,0,3500,0,3502,0,3503,0,3506,0,3507,0,3508,0,3510,0,3511,0,3515,0,3520,0,3521,0,1921,0,1923,0,1926,0,1927,0,1928,0,1929,0,1931,0,1933,0,1935,0,1938,0,1940,0,1942,0,1945,0,1947,0,1949,0,1952,0,1954,0,1956,0,1959,0,1962,0,1964,0,1967,0,1970,0,1972,0,1975,0,1978,0,1980,0,1983,0,1986,0,1988,0,1991,0,1994,0,1996,0,1999,0,2002,0,2004,0,2007,0,2010,0,2012,0,2015,0,2018,0,2020,0,2023,0,2026,0,2028,0,2031,0,2034,0,2036,0,2039,0,2042,0,2044,0,2462,0,2465,0,2466,0,2467,0,2857,0,2858,0,2859,0,2861,0,2864,0,2865,0,2866,0,2868,0,2872,0,2877,0,2878,0,3190,0,3191,0,3192,0,3194,0,3197,0,3198,0,3199,0,3201,0,3205,0,3210,0,3211,0,3214,0,3215,0,3218,0,3219,0,3438,0,3440,0,3445,0,3446,0,3447,0,3448,0,3449,0,3452,0,3453,0,3457,0,3114,0,3115,0,3116,0,3118,0,3121,0,3122,0,3123,0,3125,0,3129,0,3134,0,3135,0,3138,0,3139,0,3142,0,3143,0,45,0,46,0,39,0,40,0,41,0,3228,0,3229,0,3230,0,3232,0,3235,0,3236,0,3237,0,3239,0,3243,0,3248,0,3249,0,3252,0,3253,0,3256,0,3257,0,2714,0,2715,0,2716,0,2718,0,2721,0,2722,0,2723,0,2725,0,2729,0,2734,0,2735,0,2738,0,2739,0,2742,0,2743,0,2509,0,2512,0,2513,0,2514,0,3958,0,3962,0,3964,0,3966,0,3967,0,3968,0,3972,0,3973,0,3974,0,3976,0,3977,0,3980,0,3981,0,3982,0,3984,0,3985,0,3989,0,3994,0,3995,0,2211,0,2214,0,2215,0,2216,0,3076,0,3077,0,3078,0,3080,0,3083,0,3084,0,3085,0,3087,0,3091,0,3096,0,3097,0,3100,0,3101,0,3104,0,3105,0,2321,0,2322,0,2323,0,2325,0,2328,0,2329,0,2330,0,2332,0,2335,0,2336,0,2337,0,2338,0,2339,0,2340,0,2342,0,2347,0,2352,0,2353,0,2356,0,2357,0,2360,0,2361,0,3369,0,3370,0,3371,0,3372,0,3373,0,3374,0,3375,0,3376,0,3377,0,3378,0,3379,0,3380,0,3381,0,3382,0,3383,0,3384,0,3385,0,3386,0,3387,0,3388,0,3389,0,3390,0,3391,0,3392,0,3393,0,2415,0,2418,0,2419,0,2420,0,2052,0,2056,0,2057,0,2060,0,2064,0,2068,0,2069,0,2071,0,2072,0,2074,0,2075,0,2077,0,2078,0,2080,0,2081,0,2083,0,2084,0,2086,0,2087,0,2089,0,2090,0,2092,0,2093,0,2096,0,2097,0,2100,0,2101,0,2104,0,2105,0,2108,0,2109,0,2116,0,2119,0,2120,0,2121,0,2122,0,2123,0,2124,0,2125,0,2828,0,2829,0,2830,0,2832,0,2835,0,2836,0,2837,0,2839,0,2843,0,2848,0,2849,0,4371,0,4372,0,4375,0,4376,0,4377,0,4381,0,4382,0,4391,0,4392,0,4393,0,4397,0,4398,0,4401,0,4402,0,4405,0,4406,0,4407,0,4411,0,4412,0,4415,0,4416,0,4419,0,4420,0,4423,0,4424,0,4427,0,4428,0,4429,0,4431,0,4432,0,4434,0,4435,0,4439,0,4440,0,4441,0,4446,0]},{"source":"dart:typed_data/unmodifiable_typed_data.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Atyped_data%2Funmodifiable_typed_data.dart","uri":"dart:typed_data/unmodifiable_typed_data.dart","_kind":"library"},"hits":[150,0,152,0,154,0,156,0,158,0,160,0,162,0,182,0,75,0,77,0,79,0,81,0,83,0,85,0,86,0,88,0,89,0,91,0,92,0,94,0,95,0,97,0,98,0,100,0,101,0,103,0,104,0,106,0,107,0,109,0,110,0,112,0,113,0,115,0,116,0,118,0,119,0,121,0,122,0,124,0,125,0,127,0,128,0,130,0,131,0,133,0,135,0,137,0,139,0,141,0,142,0,172,0,222,0,262,0,282,0,292,0,13,0,15,0,17,0,18,0,20,0,21,0,23,0,24,0,25,0,27,0,28,0,30,0,31,0,33,0,34,0,36,0,37,0,39,0,40,0,42,0,43,0,45,0,46,0,47,0,49,0,50,0,51,0,53,0,54,0,55,0,57,0,58,0,59,0,61,0,62,0,63,0,65,0,66,0,272,0,252,0,202,0,242,0,212,0,302,0,192,0,232,0]},{"source":"dart:typed_data","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Atyped_data","uri":"dart:typed_data","_kind":"library"},"hits":[1273,0,1275,0,1321,0,1323,0,1000,0,1002,0,1486,0,1488,0,434,1,781,0,783,0,1368,0,1370,0,945,0,947,0,419,0,425,0,831,0,833,0,1423,0,1425,0,1054,0,1056,0,1163,0,1165,0,486,0,488,0,1218,0,1220,0,891,0,893,0,1109,0,1111,0]},{"source":"dart:async/stream_pipe.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fstream_pipe.dart","uri":"dart:async/stream_pipe.dart","_kind":"library"},"hits":[370,0,374,0,377,0,379,0,381,0,385,0,387,0,347,0,351,0,352,0,353,0,356,0,357,0,358,0,361,0,362,0,363,0,423,0,427,0,429,0,433,0,435,0,437,0,442,0,444,0,446,0,450,0,451,0,242,0,246,0,248,0,249,0,254,0,220,0,224,0,227,0,229,0,232,0,463,0,467,0,469,0,470,0,473,0,475,0,476,0,478,0,479,0,484,0,485,0,487,0,490,0,494,0,495,0,119,0,122,0,123,0,130,0,131,0,132,0,135,0,136,0,137,0,142,0,143,0,144,0,147,0,148,0,149,0,152,0,153,0,154,0,155,0,156,0,163,0,164,0,167,0,168,0,171,0,172,0,395,0,400,0,403,0,405,0,406,0,409,0,411,0,412,0,413,0,416,0,269,0,274,0,276,0,278,0,280,0,286,0,289,0,291,0,296,0,304,0,309,0,312,0,314,0,315,0,316,0,318,0,319,0,322,0,324,0,325,0,326,0,327,0,328,0,329,0,332,0,79,0,81,0,83,0,86,0,89,0,91,0,97,0,98,0,101,0,102,0,105,0,106,0,194,0,198,0,201,0,203,0,207,0,8,0,11,0,13,0,15,0,17,0,18,0,19,0,26,0,28,0,29,0,30,0,32,0,36,0,38,0,40,0,41,0,43,0,49,0,58,0,59,0,60,0,61,0,63,0,182,0,183,0,185,0,186,0,188,0,51,0,52,0]},{"source":"dart:async/stream_impl.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fstream_impl.dart","uri":"dart:async/stream_impl.dart","_kind":"library"},"hits":[784,0,790,0,791,0,793,0,794,0,796,0,797,0,800,0,802,0,804,0,807,0,809,0,810,0,811,0,814,0,817,0,818,0,819,0,820,0,821,0,824,0,825,0,826,0,831,0,832,0,833,0,834,0,839,0,840,0,842,0,843,0,844,0,845,0,848,0,849,0,850,0,853,0,854,0,855,0,858,0,859,0,860,0,109,0,112,0,113,0,114,0,123,0,126,0,127,0,128,0,129,0,135,0,139,0,142,0,144,0,145,0,146,0,147,0,148,0,150,0,155,0,157,0,160,0,161,0,162,0,163,0,165,0,166,0,167,0,168,0,171,0,172,0,173,0,174,0,175,0,176,0,178,0,181,0,182,0,188,0,192,0,193,0,194,0,196,0,199,0,200,0,203,0,206,0,222,0,223,0,224,0,225,0,226,0,227,0,228,0,229,0,230,0,231,0,232,0,234,0,236,0,237,0,238,0,239,0,241,0,242,0,252,0,254,0,259,0,261,0,262,0,263,0,265,0,269,0,270,0,271,0,272,0,274,0,278,0,280,0,281,0,282,0,283,0,285,0,293,0,297,0,301,0,314,0,315,0,316,0,317,0,319,0,320,0,321,0,322,0,323,0,330,0,334,0,335,0,336,0,337,0,338,0,341,0,345,0,363,0,364,0,365,0,366,0,367,0,368,0,370,0,373,0,375,0,379,0,393,0,394,0,395,0,396,0,397,0,399,0,410,0,412,0,413,0,414,0,415,0,416,0,429,0,431,0,432,0,433,0,434,0,440,0,441,0,444,0,445,0,446,0,448,0,450,0,452,0,455,0,456,0,593,0,594,0,595,0,582,0,583,0,584,0,1050,0,1051,0,1052,0,1054,0,601,1,602,0,603,0,606,0,608,0,609,0,683,0,685,0,686,0,687,0,689,0,693,0,695,0,696,0,697,0,698,0,700,0,703,0,704,0,705,0,501,0,503,0,505,0,506,0,507,0,509,0,468,0,472,0,473,0,479,0,481,0,486,0,965,0,967,0,968,0,969,0,974,0,975,0,976,0,977,0,978,0,979,0,980,0,983,0,985,0,993,0,995,0,998,0,999,0,1000,0,1001,0,1004,0,1007,0,1008,0,1009,0,1010,0,1012,0,1013,0,1015,0,1017,0,1019,0,1022,0,1024,0,1025,0,1026,0,1027,0,1028,0,1031,0,1033,0,1034,0,1035,0,1036,0,1039,0,1041,0,1042,0,1043,0,1044,0,639,0,640,0,648,0,649,0,651,0,653,0,656,0,662,0,665,0,666,0,723,0,724,0,727,0,728,0,729,0,731,0,732,0,733,0,734,0,737,0,738,0,739,0,740,0,743,0,744,0,745,0,748,0,749,0,750,0,751,0,752,0,757,0,759,0,760,0,761,0,767,0,768,0,769,0,770,0,771,0,870,0,872,0,873,0,877,0,878,0,882,0,883,0,887,0,888,0,891,0,892,0,895,0,896,0,897,0,900,0,901,0,904,0,905,0,519,0,521,0,523,0,524,0,525,0,534,0,536,0,537,0,541,0,543,0,544,0,548,0,549,0,550,0,561,0,564,0,565,0,569,0,204,0,207,0,208,0,209,0,213,0,347,0,350,0,351,0,353,0,354,0,355,0,358,0,360,0,384,0,387,0,388,0,389,0,390,0,657,0,658,0,659,0,660,0,762,0,210,0]},{"source":"dart:async/future.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Ffuture.dart","uri":"dart:async/future.dart","_kind":"library"},"hits":[42,0,43,0,170,0,171,0,172,0,196,0,197,0,198,0,220,0,222,0,223,0,225,0,227,0,229,0,232,0,233,0,235,0,236,0,238,0,258,0,259,0,274,0,275,0,276,0,277,0,279,0,280,0,283,0,306,0,307,0,308,0,346,0,348,0,385,0,387,0,409,0,411,0,412,0,414,0,418,0,425,0,448,0,449,0,456,0,457,0,459,0,480,0,481,0,482,0,491,0,515,0,516,0,522,0,541,0,710,0,712,0,714,0,715,0,786,0,838,0,902,0,903,0,905,0,906,0,908,0,912,0,913,0,915,0,916,0,918,0,922,0,174,0,176,0,200,0,202,0,310,0,312,0,357,0,358,0,361,0,364,0,371,0,372,0,377,0,378,0,388,0,390,0,391,0,392,0,397,0,401,0,402,0,450,0,451,0,453,0,454,0,483,0,484,0,485,0,526,0,530,0,533,0,534,0,539,0,365,0,398,0]},{"source":"dart:async/stream_controller.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fstream_controller.dart","uri":"dart:async/stream_controller.dart","_kind":"library"},"hits":[813,0,815,0,817,0,823,0,825,0,827,0,829,0,777,0,778,0,781,0,782,0,785,0,786,0,935,0,938,0,939,0,836,0,840,0,841,0,844,0,845,0,848,0,849,0,856,0,857,0,858,0,861,0,862,0,865,0,867,0,869,0,465,0,468,0,473,0,480,0,483,0,486,0,487,0,489,0,491,0,492,0,494,0,497,0,506,0,508,0,509,0,511,0,512,0,516,0,518,0,519,0,520,0,522,0,523,0,524,0,530,0,532,0,533,0,534,0,536,0,544,0,545,0,546,0,549,0,553,0,554,0,555,0,557,0,558,0,559,0,560,0,561,0,571,0,573,0,574,0,575,0,577,0,583,0,584,0,585,0,591,0,592,0,593,0,594,0,596,0,597,0,599,0,616,0,617,0,618,0,620,0,621,0,622,0,625,0,626,0,627,0,628,0,629,0,630,0,637,0,638,0,639,0,640,0,641,0,645,0,646,0,647,0,648,0,649,0,653,0,656,0,657,0,658,0,659,0,664,0,666,0,667,0,669,0,672,0,673,0,674,0,675,0,676,0,677,0,679,0,681,0,682,0,689,0,699,0,700,0,701,0,703,0,704,0,705,0,707,0,712,0,717,0,721,0,732,0,734,0,740,0,741,0,742,0,743,0,745,0,748,0,749,0,750,0,751,0,753,0,78,0,85,0,86,0,140,0,143,0,144,0,882,0,884,0,885,0,887,0,888,0,889,0,892,0,897,0,898,0,901,0,902,0,913,0,914,0,916,0,919,0,924,0,925,0,762,0,763,0,766,0,767,0,770,0,771,0,379,0,380,0,381,0,801,0,804,0,806,0,683,0,725,0,726,0,727,0,893,0,894,0,920,0]},{"source":"dart:async/stream_transformers.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fstream_transformers.dart","uri":"dart:async/stream_transformers.dart","_kind":"library"},"hits":[12,0,14,0,15,0,18,0,19,0,22,0,23,0,271,0,280,0,281,0,42,0,46,0,47,0,48,0,49,0,53,0,64,0,65,0,66,0,68,0,78,0,79,0,80,0,82,0,92,0,93,0,94,0,96,0,101,0,102,0,105,0,106,0,109,0,110,0,111,0,112,0,113,0,118,0,120,0,122,0,126,0,128,0,131,0,133,0,138,0,140,0,141,0,143,0,321,0,323,0,326,0,327,0,328,0,329,0,304,0,306,0,307,0,160,0,162,0,163,0,214,0,216,0,217,0,221,0,223,0,226,0,227,0,228,0,231,0,232,0,233,0,235,0,236,0,238,0,242,0,243,0,244,0,246,0,247,0,249,0,253,0,254,0,255,0,256,0,257,0,258,0,260,0,179,0,177,0,181,0,185,0,186,0,275,0,276,0]},{"source":"dart:async/zone.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fzone.dart","uri":"dart:async/zone.dart","_kind":"library"},"hits":[802,0,823,0,825,0,1242,0,1244,0,1246,0,1248,0,1250,0,1252,0,1254,0,1256,0,1258,0,1261,0,1263,0,1266,0,1268,0,1270,0,1275,0,1280,0,1286,0,1288,0,1297,0,1301,0,1303,0,1304,0,1307,0,1309,0,1313,0,1315,0,1316,0,1319,0,1321,0,1325,0,1327,0,1328,0,1331,0,1333,0,1337,0,1341,0,1345,0,1350,0,1354,0,1358,0,1363,0,1367,0,1368,0,1371,0,1372,0,1375,0,1376,0,1377,0,1380,0,1381,0,1382,0,1385,0,1386,0,1387,0,1390,0,1392,0,1394,0,1398,0,1400,0,1401,0,1404,0,1405,0,1408,0,1409,0,1412,0,1413,0,865,0,869,0,870,0,871,0,872,0,873,0,874,0,875,0,876,0,877,0,878,0,879,0,880,0,881,0,882,0,883,0,884,0,885,0,886,0,887,0,888,0,889,0,890,0,891,0,892,0,893,0,894,0,895,0,896,0,897,0,898,0,899,0,900,0,901,0,902,0,903,0,904,0,905,0,906,0,907,0,908,0,909,0,910,0,911,0,912,0,859,0,860,0,861,0,862,0,921,0,923,0,925,0,927,0,931,0,933,0,935,0,939,0,941,0,943,0,947,0,948,0,952,0,953,0,957,0,959,0,963,0,964,0,968,0,969,0,973,0,975,0,979,0,980,0,981,0,983,0,988,0,990,0,1000,0,1001,0,1003,0,1004,0,1005,0,1006,0,1009,0,1010,0,1012,0,1013,0,1014,0,1015,0,1018,0,1019,0,1021,0,1022,0,1023,0,1026,0,1027,0,1029,0,1030,0,1031,0,1034,0,1035,0,1037,0,1038,0,1039,0,1042,0,1043,0,1045,0,1046,0,1047,0,1050,0,1051,0,1053,0,1054,0,1055,0,1058,0,1060,0,1062,0,1063,0,1064,0,1067,0,1068,0,1070,0,1072,0,1073,0,1074,0,1077,0,1078,0,1080,0,1081,0,1082,0,1085,0,1086,0,1088,0,1089,0,1090,0,1093,0,1094,0,1096,0,1097,0,1098,0,1101,0,1102,0,1104,0,1105,0,1106,0,97,0,111,0,112,0,113,0,114,0,115,0,116,0,118,0,120,0,121,0,122,0,123,0,124,0,125,0,126,0,52,1,44,0,46,0,270,0,294,0,659,0,662,0,672,0,152,0,699,0,701,0,702,0,703,0,704,0,705,0,706,0,709,0,710,0,711,0,712,0,713,0,716,0,717,0,718,0,719,0,720,0,723,0,724,0,725,0,726,0,727,0,730,0,731,0,732,0,733,0,734,0,737,0,738,0,739,0,740,0,741,0,744,0,746,0,747,0,748,0,749,0,752,0,753,0,754,0,756,0,757,0,758,0,761,0,762,0,763,0,764,0,765,0,768,0,769,0,770,0,771,0,772,0,775,0,776,0,777,0,778,0,779,0,782,0,783,0,784,0,785,0,786,0,789,0,790,0,791,0,792,0,793,0,794,0,691,0,692,0,693,0,1110,0,1112,0,1121,0,1122,0,1124,0,1126,0,1128,0,1132,0,1134,0,1136,0,1138,0,1140,0,1144,0,1146,0,1148,0,1150,0,1152,0,1156,0,1161,0,1166,0,1171,0,1175,0,1178,0,1180,0,1182,0,1187,0,1190,0,1193,0,1195,0,1198,0,1202,0,1204,0,1207,0,1208,0,1211,0,1212,0,1215,0,1224,0,1225,0,1230,0,1231,0,1233,0,1236,0,1238,0,1462,0,1465,0,1469,0,1471,0,1474,0,1496,0,1498,0,1502,0,1505,0,1508,0,1515,0,1516,0,1517,0,1518,0,1338,0,1342,0,1347,0,1351,0,1355,0,1360,0,949,0,954,0,960,0,965,0,970,0,976,0,1113,0,1115,0,1477,0,1481,0,1484,0,1488,0,1490,0]},{"source":"dart:async/deferred_load.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fdeferred_load.dart","uri":"dart:async/deferred_load.dart","_kind":"library"},"hits":[18,0,33,0,34,0]},{"source":"dart:async/runtime/libdeferred_load_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fruntime%2Flibdeferred_load_patch.dart","uri":"dart:async/runtime/libdeferred_load_patch.dart","_kind":"library"},"hits":[12,0,15,0,16,0]},{"source":"dart:async/broadcast_stream_controller.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fbroadcast_stream_controller.dart","uri":"dart:async/broadcast_stream_controller.dart","_kind":"library"},"hits":[359,0,364,0,366,0,367,0,368,0,371,0,374,0,375,0,376,0,377,0,378,0,379,0,380,0,381,0,382,0,386,0,391,0,392,0,393,0,398,0,399,0,400,0,406,0,99,0,102,0,103,0,107,0,108,0,112,0,113,0,117,0,118,0,124,0,126,0,128,0,136,0,139,0,146,0,148,0,152,0,154,0,156,0,158,0,159,0,160,0,165,0,168,0,170,0,172,0,173,0,174,0,175,0,177,0,179,0,183,0,186,0,187,0,190,0,192,0,196,0,198,0,201,0,206,0,208,0,210,0,212,0,214,0,215,0,217,0,222,0,225,0,226,0,227,0,229,0,232,0,233,0,239,0,240,0,244,0,245,0,246,0,249,0,252,0,253,0,254,0,257,0,258,0,259,0,260,0,262,0,263,0,265,0,268,0,269,0,271,0,273,0,274,0,275,0,276,0,280,0,282,0,283,0,284,0,285,0,286,0,290,0,291,0,294,0,295,0,298,0,300,0,301,0,302,0,303,0,307,0,309,0,310,0,313,0,316,0,323,0,324,0,326,0,327,0,328,0,329,0,330,0,331,0,332,0,334,0,337,0,340,0,342,0,343,0,347,0,349,0,351,0,353,0,412,0,417,0,418,0,420,0,421,0,425,0,426,0,428,0,429,0,433,0,434,0,435,0,437,0,438,0,443,0,8,0,11,0,463,0,466,0,468,0,469,0,470,0,472,0,475,0,476,0,477,0,480,0,481,0,482,0,486,0,487,0,488,0,491,0,492,0,493,0,494,0,498,0,499,0,500,0,501,0,502,0,504,0,509,0,510,0,511,0,512,0,514,0,26,0,29,0,32,0,34,0,35,0,38,0,40,0,42,0,45,0,46,0,50,0,54,0,387,0,394,0,401,0]},{"source":"dart:async/stream.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fstream.dart","uri":"dart:async/stream.dart","_kind":"library"},"hits":[77,0,85,0,101,0,105,0,106,0,113,0,131,0,132,0,152,0,153,0,154,0,157,0,158,0,172,0,173,0,186,0,192,0,215,0,241,0,284,0,286,0,297,0,298,0,303,0,326,0,329,0,383,0,384,0,405,0,406,0,419,0,448,0,449,0,456,0,467,0,488,0,512,0,513,0,520,0,531,0,565,0,566,0,588,0,589,0,608,0,609,0,629,0,630,0,652,0,653,0,657,0,668,0,704,0,705,0,708,0,714,0,736,0,737,0,738,0,741,0,770,0,771,0,773,0,781,0,798,0,799,0,801,0,807,0,828,0,829,0,831,0,839,0,860,0,861,0,863,0,871,0,891,0,892,0,894,0,898,0,920,0,921,0,923,0,927,0,941,0,952,0,953,0,954,0,955,0,959,0,976,0,977,0,978,0,979,0,983,0,1002,0,1003,0,1026,0,1027,0,1052,0,1053,0,1071,0,1072,0,1093,0,1094,0,1118,0,1119,0,1140,0,1141,0,1143,0,1147,0,1169,0,1170,0,1173,0,1178,0,1204,0,1205,0,1209,0,1223,0,1264,0,1265,0,1267,0,1275,0,1302,0,1303,0,1307,0,1316,0,1342,0,1343,0,1347,0,1364,0,1400,0,1401,0,1402,0,1405,0,1413,0,1449,0,1512,0,1513,0,1514,0,1522,0,2027,1,2029,0,2030,0,2105,0,2107,0,2108,0,2111,0,2112,0,2115,0,2116,0,1982,0,1984,0,2044,0,2048,0,1711,0,1715,0,1717,0,1720,0,1722,0,1724,0,107,0,108,0,109,0,110,0,111,0,137,0,138,0,139,0,140,0,143,0,144,0,145,0,146,0,174,0,194,0,195,0,199,0,201,0,205,0,208,0,210,0,217,0,218,0,219,0,221,0,222,0,224,0,226,0,228,0,229,0,230,0,236,0,237,0,239,0,423,0,424,0,428,0,429,0,445,0,451,0,452,0,458,0,459,0,461,0,462,0,464,0,491,0,495,0,508,0,509,0,515,0,516,0,522,0,523,0,525,0,526,0,528,0,658,0,660,0,662,0,669,0,675,0,677,0,680,0,709,0,710,0,712,0,715,0,716,0,743,0,747,0,749,0,751,0,752,0,753,0,754,0,774,0,775,0,779,0,782,0,783,0,802,0,804,0,805,0,808,0,809,0,832,0,833,0,837,0,840,0,841,0,864,0,865,0,869,0,872,0,873,0,895,0,896,0,899,0,900,0,924,0,925,0,928,0,929,0,956,0,957,0,960,0,961,0,980,0,981,0,984,0,985,0,1144,0,1145,0,1148,0,1150,0,1152,0,1174,0,1179,0,1181,0,1185,0,1187,0,1210,0,1214,0,1216,0,1224,0,1226,0,1230,0,1232,0,1268,0,1269,0,1273,0,1276,0,1278,0,1282,0,1284,0,1308,0,1309,0,1314,0,1317,0,1319,0,1323,0,1327,0,1329,0,1348,0,1349,0,1362,0,1365,0,1367,0,1372,0,1375,0,1377,0,1406,0,1407,0,1408,0,1411,0,1414,0,1415,0,1416,0,1457,0,1458,0,1459,0,1460,0,1463,0,1464,0,1468,0,1469,0,1472,0,1473,0,1474,0,1477,0,1482,0,1492,0,1493,0,1501,0,1502,0,1505,0,1506,0,1507,0,1516,0,1517,0,1518,0,1519,0,1520,0,211,0,232,0,233,0,432,0,434,0,437,0,438,0,440,0,441,0,443,0,498,0,500,0,504,0,505,0,777,0,835,0,867,0,1271,0,1353,0,1355,0,1484,0,1485,0,1486,0,1494,0,1495,0,1496,0,1497,0]},{"source":"dart:async/future_impl.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Ffuture_impl.dart","uri":"dart:async/future_impl.dart","_kind":"library"},"hits":[19,0,20,0,21,0,22,0,24,0,25,0,27,0,34,0,49,0,50,0,51,0,54,0,55,0,38,0,39,0,40,0,43,0,44,0,81,0,87,0,92,0,97,0,99,0,100,0,101,0,102,0,104,0,106,0,109,0,110,0,112,0,115,0,117,0,123,0,125,0,128,0,129,0,132,0,133,0,134,0,137,0,139,0,142,0,143,0,144,0,147,0,151,0,153,0,209,0,211,0,212,0,215,0,216,0,220,0,221,0,224,0,225,0,226,0,227,0,228,0,229,0,231,0,233,0,234,0,237,0,238,0,240,0,245,0,248,0,252,0,254,0,255,0,259,0,260,0,261,0,262,0,263,0,265,0,269,0,270,0,271,0,272,0,274,0,278,0,280,0,282,0,285,0,287,0,290,0,292,0,295,0,297,0,301,0,303,0,304,0,307,0,309,0,310,0,313,0,314,0,320,0,323,0,324,0,327,0,329,0,330,0,331,0,333,0,337,0,338,0,339,0,342,0,346,0,352,0,354,0,355,0,356,0,359,0,360,0,362,0,365,0,369,0,370,0,371,0,374,0,377,0,378,0,384,0,388,0,389,0,390,0,393,0,397,0,398,0,409,0,414,0,416,0,437,0,445,0,447,0,448,0,450,0,451,0,452,0,453,0,455,0,456,0,457,0,461,0,463,0,464,0,465,0,467,0,470,0,471,0,472,0,476,0,480,0,481,0,482,0,485,0,488,0,489,0,490,0,493,0,506,0,507,0,510,0,511,0,516,0,517,0,518,0,520,0,521,0,525,0,530,0,533,0,536,0,537,0,546,0,549,0,552,0,553,0,554,0,561,0,563,0,564,0,565,0,568,0,582,0,583,0,584,0,586,0,587,0,588,0,593,0,595,0,663,0,664,0,666,0,667,0,670,0,671,0,676,0,680,0,684,0,685,0,686,0,687,0,688,0,692,0,695,0,700,0,701,0,703,0,706,0,713,0,714,0,715,0,718,0,723,0,724,0,725,0,733,0,759,0,760,0,762,0,764,0,765,0,767,0,347,0,379,0,421,0,422,0,428,0,430,0,438,0,512,0,522,0,538,0,600,0,608,0,610,0,611,0,613,0,618,0,619,0,620,0,621,0,631,0,636,0,638,0,640,0,645,0,647,0,648,0,649,0,650,0,654,0,655,0,657,0,719,0,720,0,727,0,729,0,734,0,735,0,736,0,738,0,739,0,740,0,741,0]},{"source":"dart:async/runtime/libasync_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fruntime%2Flibasync_patch.dart","uri":"dart:async/runtime/libasync_patch.dart","_kind":"library"},"hits":[24,0,26,0,27,0,28,0,29,0,30,0,32,0,38,0,39,0,40,0,42,0,48,0,49,0,50,0,53,0,54,0,236,0,237,0,238,0,239,0,240,0,146,0,147,0,148,0,149,0,154,0,155,0,156,0,157,0,160,0,161,0,164,0,165,0,178,0,179,0,180,0,182,0,185,0,186,0,187,0,196,0,197,0,199,0,200,0,202,0,203,0,211,0,212,0,215,0,219,0,220,0,227,0,228,0,231,0,233,0,243,0,245,0,246,0,249,0,250,0,251,0,255,0,256,0,259,0,260,0,264,0,265,0,268,0,18,0,60,0,76,0,77,0,82,0,85,0,86,0,94,0,96,0,97,0,98,0,99,0,109,0,110,0,115,0,116,0,120,0,123,0,124,0,128,0,132,0,291,0,292,0,297,0,300,0,303,0,306,0,273,0,33,0,43,0,204,0,205,0,206,0,84,0]},{"source":"dart:async/runtime/libschedule_microtask_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fruntime%2Flibschedule_microtask_patch.dart","uri":"dart:async/runtime/libschedule_microtask_patch.dart","_kind":"library"},"hits":[10,0,12,0,14,0,24,1,28,0,29,0]},{"source":"dart:async/schedule_microtask.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fschedule_microtask.dart","uri":"dart:async/schedule_microtask.dart","_kind":"library"},"hits":[12,0,35,0,39,0,41,0,45,0,50,0,55,0,66,0,67,0,71,0,74,0,87,0,89,0,93,0,95,0,98,0,99,0,101,0,132,0,133,0,137,0,140,0,141,0,142,0,143,0,144,0,147,0]},{"source":"dart:async/timer.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Ftimer.dart","uri":"dart:async/timer.dart","_kind":"library"},"hits":[45,0,46,0,49,0,51,0,52,0,71,0,72,0,75,0,77,0,78,0,86,0,87,0]},{"source":"dart:async/runtime/libtimer_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fruntime%2Flibtimer_patch.dart","uri":"dart:async/runtime/libtimer_patch.dart","_kind":"library"},"hits":[10,0,16,0,18,0,19,0,20,0,26,0,33,0,35,0,36,0,37,0,21,0]},{"source":"dart:async/async_error.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aasync%2Fasync_error.dart","uri":"dart:async/async_error.dart","_kind":"library"},"hits":[7,0,9,0,10,0,13,0]},{"source":"dart:convert/string_conversion.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fstring_conversion.dart","uri":"dart:convert/string_conversion.dart","_kind":"library"},"hits":[241,0,243,0,244,0,245,0,246,0,247,0,250,0,251,0,188,0,189,0,192,0,193,0,196,0,197,0,292,0,293,0,295,0,296,0,297,0,300,0,301,0,304,0,306,0,307,0,321,0,322,0,324,0,326,0,329,0,330,0,331,0,332,0,333,0,334,0,336,0,340,0,341,0,344,0,345,0,346,0,347,0,348,0,349,0,352,0,92,0,94,0,95,0,98,0,99,0,102,0,103,0,106,0,107,0,110,0,111,0,265,0,267,0,268,0,271,0,272,0,273,0,275,0,277,0,280,0,281,0,127,0,128,0,130,0,131,0,132,0,135,0,136,0,137,0,140,0,141,0,142,0,145,0,146,0,147,0,150,0,151,0,152,0,153,0,154,0,156,0,157,0,159,0,160,0,161,0,162,0,167,0,168,0,169,0,170,0,21,0,206,0,208,0,209,0,210,0,211,0,212,0,215,0,217,0,220,0,221,0,224,0,225,0,228,0,229,0]},{"source":"dart:convert/base64.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fbase64.dart","uri":"dart:convert/base64.dart","_kind":"library"},"hits":[79,1,80,0,82,0,84,0,95,0,111,0,112,0,118,0,122,0,124,0,127,0,128,0,129,0,130,0,133,0,141,0,142,0,143,0,144,0,145,0,146,0,148,0,150,0,153,0,155,0,157,0,158,0,159,0,160,0,165,0,168,0,169,0,173,0,174,0,177,0,178,0,180,0,183,0,184,0,185,0,188,0,191,0,192,0,193,0,197,0,198,0,200,0,203,0,205,0,211,0,213,0,214,0,220,0,221,0,224,0,225,0,504,1,516,0,517,0,518,0,519,0,520,0,521,0,525,0,526,0,247,1,248,1,250,0,251,0,252,0,253,0,254,0,257,0,258,0,259,0,261,0,894,0,896,0,897,0,898,0,899,0,902,0,903,0,904,0,907,0,908,0,909,0,910,0,911,0,913,0,914,0,429,0,431,0,432,0,433,0,436,0,601,0,603,0,609,0,611,0,617,0,619,0,625,0,628,0,634,0,636,0,639,0,648,0,652,0,653,0,656,0,657,0,658,0,663,0,664,0,665,0,667,0,668,0,671,0,681,0,689,0,690,0,696,0,697,0,698,0,699,0,700,0,701,0,702,0,703,0,705,0,706,0,707,0,711,0,712,0,713,0,714,0,715,0,718,0,719,0,721,0,722,0,725,0,732,0,733,0,734,0,735,0,737,0,739,0,740,0,744,0,745,0,746,0,748,0,756,0,759,0,760,0,762,0,765,0,766,0,767,0,769,0,788,0,794,0,795,0,796,0,797,0,798,0,802,0,803,0,804,0,805,0,807,0,808,0,809,0,810,0,812,0,813,0,842,0,844,0,845,0,848,0,849,0,850,0,851,0,852,0,853,0,856,0,857,0,858,0,859,0,860,0,867,0,868,0,870,0,871,0,872,0,873,0,874,0,877,0,878,0,879,0,880,0,882,0,883,0,885,0,481,0,482,0,484,0,485,0,487,0,462,0,463,0,465,0,466,0,468,0,469,0,472,0,297,0,301,0,303,0,307,0,310,0,315,0,330,0,334,0,336,0,337,0,338,0,339,0,340,0,341,0,342,0,344,0,345,0,346,0,347,0,353,0,355,0,357,0,363,0,364,0,365,0,366,0,367,0,368,0,369,0,370,0,371,0,372,0,377,0,378,0,379,0,382,0,387,0,388,0,389,0,390,0,392,0,393,0,402,0,405,0,406,0,407,0,408,0,409,0,412,0,413,0,414,0,415,0,441,0,442,0,445,0,446,0,449,0,450,0,451,0,452,0,46,0,53,0,60,0]},{"source":"dart:convert/byte_conversion.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fbyte_conversion.dart","uri":"dart:convert/byte_conversion.dart","_kind":"library"},"hits":[63,0,65,0,66,0,69,0,70,0,87,0,90,0,91,0,92,0,94,0,95,0,96,0,97,0,98,0,100,0,101,0,104,0,106,0,107,0,108,0,109,0,110,0,111,0,112,0,116,0,117,0,48,0,49,0,50,0,19,0]},{"source":"dart:convert/converter.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fconverter.dart","uri":"dart:convert/converter.dart","_kind":"library"},"hits":[79,0,81,0,83,0,84,0,14,1,25,0,26,0,39,0,40,0,49,0,50,0,51,0,54,0,55,0,67,0,56,0]},{"source":"dart:convert/latin1.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Flatin1.dart","uri":"dart:convert/latin1.dart","_kind":"library"},"hits":[178,0,180,0,181,0,182,0,183,0,184,0,185,0,187,0,188,0,191,0,192,0,195,0,96,0,105,0,107,0,110,0,113,0,114,0,78,0,120,0,122,0,123,0,124,0,127,0,128,0,131,0,136,0,137,0,140,0,141,0,142,0,143,0,147,0,149,0,152,0,154,0,155,0,157,0,160,0,163,0,165,0,166,0,167,0,168,0,42,1,44,0,46,0,58,0,59,0,61,0,63,0,67,0,69,0]},{"source":"dart:convert/json.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fjson.dart","uri":"dart:convert/json.dart","_kind":"library"},"hits":[488,0,492,0,493,0,496,0,497,0,498,0,500,0,501,0,502,0,503,0,506,0,507,0,508,0,509,0,456,0,465,0,466,0,467,0,469,0,470,0,471,0,472,0,475,0,524,0,542,0,552,0,952,0,954,0,968,0,972,0,975,0,977,0,978,0,985,0,986,0,987,0,989,0,990,0,993,0,995,0,996,0,1000,0,1003,0,1004,0,1006,0,1010,0,1011,0,1014,0,1018,0,1019,0,1020,0,1021,0,1023,0,1025,0,1026,0,1028,0,1029,0,1030,0,1034,0,1039,0,1040,0,1041,0,1044,0,1047,0,1048,0,1049,0,1050,0,1053,0,1054,0,1055,0,1056,0,1059,0,1062,0,1064,0,1065,0,1066,0,1067,0,1070,0,1072,0,1073,0,1074,0,1075,0,1077,0,370,0,372,0,376,0,378,0,381,0,382,0,384,0,386,0,390,0,391,0,403,0,404,0,405,0,407,0,408,0,410,0,411,0,412,0,413,0,414,0,428,0,430,0,433,0,435,0,436,0,440,0,441,0,930,0,933,0,934,0,53,0,54,0,809,0,810,0,811,0,813,0,814,0,815,0,816,0,817,0,818,0,819,0,820,0,822,0,823,0,824,0,825,0,829,0,830,0,831,0,834,0,837,0,845,0,846,0,848,0,849,0,851,0,852,0,853,0,854,0,855,0,857,0,858,0,859,0,860,0,29,0,32,0,33,0,35,0,40,0,1087,0,1091,0,1092,0,1093,0,1094,0,1095,0,1096,0,1097,0,1098,0,1102,0,1103,0,1104,0,1105,0,1106,0,1107,0,1109,0,1110,0,590,0,605,0,610,0,612,0,613,0,614,0,615,0,616,0,617,0,618,0,619,0,621,0,622,0,624,0,625,0,627,0,628,0,630,0,631,0,633,0,634,0,637,0,638,0,639,0,640,0,641,0,644,0,645,0,646,0,647,0,648,0,651,0,652,0,653,0,654,0,664,0,665,0,666,0,667,0,670,0,679,0,682,0,691,0,695,0,696,0,698,0,699,0,700,0,701,0,703,0,705,0,706,0,716,0,717,0,718,0,719,0,722,0,725,0,728,0,730,0,731,0,732,0,733,0,735,0,736,0,737,0,738,0,740,0,741,0,743,0,744,0,752,0,753,0,754,0,755,0,756,0,757,0,758,0,761,0,765,0,766,0,767,0,770,0,773,0,781,0,783,0,784,0,786,0,787,0,788,0,790,0,233,0,255,0,286,0,287,0,298,0,299,0,300,0,301,0,302,0,303,0,304,0,305,0,308,0,312,0,314,0,315,0,320,0,322,0,871,0,884,0,885,0,886,0,887,0,895,0,899,0,902,0,904,0,907,0,909,0,910,0,913,0,914,0,917,0,918,0,921,0,922,0,142,1,154,0,167,0,168,0,169,0,170,0,184,0,185,0,186,0,187,0,190,0,191,0,192,0,195,0,196,0,197,0,85,0,86,0,100,0,101,0,560,0,394,0,395,0,396,0,397,0,398,0,400,0,838,0,841,0,842,0,774,0,777,0,778,0]},{"source":"dart:convert/codec.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fcodec.dart","uri":"dart:convert/codec.dart","_kind":"library"},"hits":[100,0,97,0,98,0,18,1,25,0,32,0,75,0,76,0,84,0,106,0,108,0,109,0,111,0]},{"source":"dart:convert/runtime/libconvert_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fruntime%2Flibconvert_patch.dart","uri":"dart:convert/runtime/libconvert_patch.dart","_kind":"library"},"hits":[1428,0,1429,0,1444,0,1445,0,1447,0,1450,0,1452,0,1454,0,1457,0,1458,0,1459,0,1460,0,1461,0,1464,0,1465,0,1468,0,1469,0,1470,0,1471,0,1472,0,1475,0,1476,0,1477,0,1740,0,1743,0,1744,0,1747,0,1749,0,1751,0,1752,0,1754,0,1755,0,1756,0,1760,0,1761,0,1764,0,1765,0,1766,0,1769,0,1770,0,1771,0,1774,0,1775,0,1776,0,1777,0,1780,0,1781,0,1782,0,1785,0,1786,0,1787,0,460,0,468,0,469,0,475,0,476,0,492,0,493,0,494,0,495,0,496,0,500,0,501,0,502,0,503,0,504,0,507,0,510,0,511,0,521,0,522,0,606,0,608,0,618,0,620,0,629,0,632,0,633,0,634,0,635,0,642,0,643,0,644,0,646,0,647,0,648,0,650,0,651,0,652,0,653,0,654,0,655,0,669,0,672,0,673,0,674,0,677,0,678,0,679,0,680,0,681,0,682,0,687,0,688,0,689,0,690,0,692,0,695,0,697,0,700,0,701,0,702,0,704,0,707,0,711,0,712,0,713,0,714,0,716,0,717,0,720,0,721,0,722,0,725,0,729,0,730,0,731,0,732,0,734,0,735,0,737,0,738,0,739,0,740,0,744,0,746,0,747,0,748,0,749,0,751,0,756,0,757,0,766,0,767,0,768,0,770,0,771,0,773,0,774,0,777,0,778,0,779,0,780,0,781,0,782,0,783,0,784,0,786,0,787,0,793,0,794,0,795,0,796,0,798,0,801,0,802,0,803,0,804,0,806,0,807,0,808,0,812,0,814,0,815,0,816,0,817,0,818,0,819,0,820,0,826,0,827,0,828,0,829,0,831,0,841,0,842,0,843,0,844,0,845,0,847,0,848,0,849,0,851,0,852,0,853,0,854,0,855,0,857,0,858,0,859,0,860,0,862,0,863,0,864,0,865,0,867,0,869,0,870,0,871,0,872,0,874,0,876,0,877,0,878,0,879,0,881,0,882,0,883,0,884,0,886,0,887,0,888,0,889,0,891,0,892,0,893,0,895,0,897,0,898,0,899,0,901,0,902,0,903,0,905,0,907,0,910,0,911,0,912,0,913,0,914,0,915,0,917,0,919,0,920,0,922,0,923,0,924,0,925,0,926,0,927,0,929,0,931,0,932,0,935,0,936,0,937,0,938,0,942,0,950,0,952,0,953,0,955,0,956,0,957,0,958,0,960,0,961,0,969,0,971,0,972,0,974,0,975,0,976,0,977,0,978,0,980,0,981,0,989,0,991,0,992,0,994,0,995,0,996,0,997,0,999,0,1000,0,1003,0,1005,0,1008,0,1009,0,1010,0,1011,0,1013,0,1023,0,1027,0,1029,0,1030,0,1031,0,1033,0,1036,0,1037,0,1038,0,1039,0,1040,0,1042,0,1043,0,1046,0,1047,0,1050,0,1051,0,1052,0,1062,0,1063,0,1064,0,1076,0,1077,0,1078,0,1079,0,1080,0,1081,0,1094,0,1095,0,1098,0,1099,0,1100,0,1102,0,1104,0,1105,0,1106,0,1107,0,1109,0,1110,0,1111,0,1112,0,1114,0,1117,0,1121,0,1122,0,1124,0,1125,0,1126,0,1141,0,1142,0,1143,0,1145,0,1148,0,1151,0,1154,0,1157,0,1160,0,1161,0,1162,0,1164,0,1165,0,1167,0,1168,0,1169,0,1170,0,1171,0,1172,0,1173,0,1175,0,1176,0,1177,0,1179,0,1185,0,1186,0,1188,0,1189,0,1195,0,1196,0,1197,0,1198,0,1199,0,1200,0,1201,0,1202,0,1206,0,1207,0,1208,0,1209,0,1210,0,1211,0,1212,0,1213,0,1217,0,1218,0,1219,0,1220,0,1221,0,1225,0,1226,0,1227,0,1230,0,1231,0,1233,0,1234,0,1235,0,1236,0,1238,0,1243,0,1248,0,1258,0,1260,0,1261,0,1262,0,1264,0,1265,0,1266,0,1267,0,1270,0,1273,0,1274,0,1275,0,1276,0,1277,0,1279,0,1282,0,1283,0,1284,0,1285,0,1286,0,1287,0,1289,0,1291,0,1293,0,1294,0,1295,0,1296,0,1297,0,1299,0,1300,0,1301,0,1302,0,1303,0,1304,0,1305,0,1307,0,1309,0,1313,0,1314,0,1315,0,1318,0,1319,0,1320,0,1321,0,1322,0,1324,0,1325,0,1326,0,1329,0,1330,0,1331,0,1332,0,1333,0,1334,0,1335,0,1338,0,1344,0,1346,0,1347,0,1348,0,1349,0,1352,0,1353,0,1356,0,1357,0,1365,0,1369,0,1372,0,1374,0,59,0,61,0,62,0,63,0,64,0,65,0,66,0,67,0,70,0,71,0,37,0,38,0,39,0,40,0,44,0,49,0,1524,0,1542,0,1544,0,1546,0,1547,0,1548,0,1549,0,1552,0,1553,0,1554,0,1555,0,1558,0,1564,0,1565,0,1566,0,1567,0,1569,0,1572,0,1573,0,1574,0,1576,0,1579,0,1583,0,1586,0,1587,0,1588,0,1589,0,1591,0,1594,0,1595,0,1597,0,1598,0,1599,0,1600,0,1601,0,1604,0,1605,0,1606,0,1607,0,1608,0,1611,0,1614,0,1616,0,1618,0,1619,0,1620,0,1622,0,1624,0,1625,0,1626,0,1629,0,1630,0,1632,0,1633,0,1635,0,1637,0,1638,0,1641,0,1643,0,1644,0,1645,0,1646,0,1647,0,1648,0,1650,0,1654,0,1655,0,1656,0,1657,0,1658,0,1659,0,1660,0,1661,0,1662,0,1664,0,1665,0,1668,0,1669,0,1670,0,1671,0,1672,0,1674,0,1677,0,1680,0,1681,0,1682,0,1685,0,1686,0,1687,0,1690,0,1691,0,1693,0,1694,0,1695,0,1697,0,1698,0,1701,0,1702,0,1704,0,1707,0,1708,0,1709,0,1710,0,1711,0,1713,0,1714,0,1715,0,1716,0,1717,0,1718,0,1719,0,1720,0,1721,0,1723,0,1724,0,1725,0,1728,0,1801,0,1802,0,1804,0,1807,0,1809,0,1811,0,1814,0,1815,0,1816,0,1819,0,1820,0,1823,0,1824,0,1825,0,1826,0,1829,0,1830,0,1831,0,1832,0,1833,0,198,0,200,0,201,0,202,0,203,0,206,0,207,0,208,0,211,0,212,0,83,0,84,0,85,0,86,0,87,0,88,0,89,0,90,0,91,0,92,0,93,0,230,0,231,0,233,0,241,0,242,0,243,0,244,0,249,0,250,0,251,0,252,0,253,0,254,0,257,0,258,0,265,0,266,0,126,0,127,0,128,0,132,0,133,0,134,0,135,0,138,0,139,0,142,0,143,0,146,0,147,0,150,0,151,0,154,0,155,0,156,0,159,0,160,0,161,0,164,0,165,0,166,0,167,0,170,0,171,0,174,0,175,0,176,0,179,0,180,0,181,0,182,0,185,0,186,0,190,0,192,0,1385,0,1387,0,1389,0,1390,0,1393,0,1394,0,1397,0,1398,0,1399,0,1402,0,1403,0,1404,0,1407,0,1408,0,1409,0,1410,0,1413,0,1414,0,1415,0,1416,0,1420,0,1421,0,1791,0,19,0,22,0,24,0,26,0,27,0,28,0,29,0,30,0,31,0]},{"source":"dart:convert/line_splitter.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fline_splitter.dart","uri":"dart:convert/line_splitter.dart","_kind":"library"},"hits":[169,0,171,0,173,0,174,0,22,0,52,0,53,0,54,0,57,0,59,0,60,0,61,0,62,0,63,0,67,0,68,0,70,0,71,0,76,0,77,0,78,0,80,0,83,0,84,0,107,0,109,0,110,0,113,0,114,0,117,0,119,0,121,0,122,0,123,0,124,0,125,0,127,0,129,0,130,0,133,0,134,0,135,0,136,0,138,0,141,0,144,0,146,0,147,0,148,0,149,0,150,0,154,0,155,0,157,0,158,0,160,0,30,0,31,0,34,0,36,0,37,0,38,0,39,0,40,0,44,0,45,0,47,0,48,0,85,0]},{"source":"dart:convert/ascii.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fascii.dart","uri":"dart:convert/ascii.dart","_kind":"library"},"hits":[289,0,291,0,292,0,295,0,296,0,297,0,298,0,301,0,304,0,305,0,306,0,307,0,308,0,309,0,311,0,313,0,125,0,136,0,138,0,139,0,142,0,143,0,144,0,145,0,146,0,147,0,148,0,151,0,153,0,259,0,261,0,262,0,265,0,266,0,269,0,270,0,271,0,272,0,273,0,275,0,276,0,279,0,280,0,282,0,229,0,238,0,240,0,243,0,249,0,250,0,252,0,180,0,189,0,190,0,191,0,194,0,195,0,196,0,197,0,198,0,200,0,203,0,206,0,207,0,208,0,209,0,210,0,211,0,213,0,222,0,225,0,80,0,88,0,89,0,90,0,92,0,93,0,94,0,95,0,96,0,97,0,99,0,110,0,111,0,112,0,114,0,118,0,43,1,45,0,47,0,59,0,60,0,62,0,64,0,68,0,70,0]},{"source":"dart:convert/encoding.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fencoding.dart","uri":"dart:convert/encoding.dart","_kind":"library"},"hits":[11,1,16,0,18,0,19,0,20,0,74,0,76,0,77,0]},{"source":"dart:convert/chunked_conversion.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fchunked_conversion.dart","uri":"dart:convert/chunked_conversion.dart","_kind":"library"},"hits":[21,0,51,0,53,0,54,0,57,0,58,0,81,0,83,0,85,0,86,0,89,0,90,0,93,0,94,0]},{"source":"dart:convert/utf.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Futf.dart","uri":"dart:convert/utf.dart","_kind":"library"},"hits":[324,0,337,0,340,0,345,0,346,0,348,0,349,0,350,0,351,0,352,0,361,0,363,0,366,0,368,0,372,0,148,0,150,0,151,0,156,0,169,0,170,0,171,0,176,0,177,0,178,0,179,0,187,0,188,0,189,0,203,0,204,0,207,0,210,0,211,0,213,0,214,0,215,0,216,0,217,0,220,0,221,0,222,0,225,0,226,0,227,0,228,0,231,0,232,0,233,0,234,0,86,0,95,0,96,0,97,0,99,0,100,0,103,0,104,0,106,0,110,0,114,0,117,0,126,0,127,0,128,0,130,0,134,0,249,0,251,0,252,0,254,0,257,0,260,0,261,0,263,0,267,0,269,0,270,0,274,0,277,0,278,0,281,0,282,0,283,0,284,0,288,0,293,0,295,0,297,0,298,0,299,0,300,0,51,1,54,0,70,0,71,0,72,0,75,0,76,0,77,0,415,0,417,0,427,0,428,0,440,0,441,0,442,0,443,0,446,0,447,0,448,0,449,0,453,0,454,0,455,0,456,0,457,0,458,0,459,0,481,0,483,0,486,0,487,0,489,0,490,0,491,0,495,0,496,0,499,0,500,0,501,0,503,0,504,0,507,0,508,0,509,0,511,0,516,0,517,0,518,0,520,0,522,0,526,0,527,0,529,0,532,0,533,0,534,0,535,0,536,0,537,0,538,0,540,0,546,0,548,0,549,0,550,0,552,0,554,0,557,0,558,0,562,0,563,0,568,0,569,0,573,0,574,0,575,0,577,0,581,0,582,0,587,0,588,0,589,0,590,0,393,0,394,0,395,0,396,0,397,0,398,0,399,0,461,0,464,0,465,0,466,0,468,0,471,0,474,0]},{"source":"dart:convert/html_escape.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aconvert%2Fhtml_escape.dart","uri":"dart:convert/html_escape.dart","_kind":"library"},"hits":[176,0,178,0,179,0,189,0,191,0,192,0,195,0,198,0,199,0,201,0,202,0,204,0,205,0,207,0,208,0,210,0,211,0,215,0,216,0,217,0,218,0,222,0,223,0,226,0,227,0,228,0,230,0,122,1,132,0,140,0,238,0,240,0,241,0,243,0,245,0,246,0,250,0,251,0]},{"source":"dart:_internal/list.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Flist.dart","uri":"dart:_internal/list.dart","_kind":"library"},"hits":[89,0,90,0,94,0,95,0,99,0,100,0,103,0,104,0,108,0,109,0,113,0,114,0,118,0,119,0,123,0,124,0,128,0,129,0,133,0,134,0,138,0,139,0,143,0,144,0,148,0,149,0,153,0,154,0,158,0,159,0,163,0,164,0,168,0,169,0,173,0,174,0,178,0,179,0,183,0,184,0,188,0,189,0,14,0,15,0,20,0,21,0,25,0,26,0,30,0,31,0,35,0,36,0,40,0,41,0,45,0,46,0,50,0,51,0,55,0,56,0,60,0,61,0,65,0,66,0,70,0,71,0,75,0,76,0,289,0,290,0,293,0,294,0,297,0,298,0,252,0,254,0,256,0,266,0,267,0,270,0,271,0,274,0,275,0,278,0,279,0,226,0,228,0,229,0,231,0,232,0,234,0,235,0,236,0,237,0,239,0,240,0,241,0,242,0,243,0,244,0,214,0,216,0,217,0,218,0]},{"source":"dart:_internal/iterable.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Fiterable.dart","uri":"dart:_internal/iterable.dart","_kind":"library"},"hits":[591,0,584,0,585,0,586,0,588,0,593,0,594,0,597,0,598,0,362,0,355,0,356,0,357,0,359,0,364,0,367,0,368,0,371,0,372,0,373,0,374,0,872,0,873,0,664,0,666,0,667,0,668,0,669,0,670,0,673,0,676,0,379,0,854,0,855,0,857,0,858,0,859,0,860,0,861,0,862,0,867,0,388,0,390,0,391,0,392,0,395,0,399,0,327,0,329,0,332,0,334,0,335,0,336,0,337,0,339,0,340,0,343,0,344,0,32,1,34,0,36,0,37,0,38,0,39,0,40,0,41,0,46,0,48,0,49,0,50,0,53,0,54,0,55,0,58,0,59,0,60,0,61,0,64,0,65,0,66,0,67,0,68,0,69,0,75,0,76,0,77,0,78,0,79,0,80,0,86,0,87,0,88,0,89,0,90,0,91,0,97,0,98,0,99,0,100,0,101,0,102,0,103,0,106,0,107,0,110,0,111,0,112,0,113,0,114,0,115,0,116,0,119,0,120,0,123,0,124,0,127,0,128,0,129,0,131,0,136,0,137,0,141,0,142,0,145,1,146,1,147,1,148,1,149,2,150,2,153,1,154,2,155,1,156,2,157,2,161,1,151,0,158,0,163,0,164,0,165,0,166,0,167,0,170,0,174,0,176,0,178,0,179,0,180,0,181,0,182,0,183,0,184,0,185,0,191,0,193,0,194,0,195,0,196,0,197,0,203,0,205,0,207,0,209,0,211,0,214,0,216,0,218,0,219,0,224,0,225,0,226,0,227,0,239,0,240,0,241,0,242,0,243,0,244,0,249,0,250,0,251,0,252,0,255,0,256,0,257,0,258,0,261,0,262,0,263,0,264,0,265,0,267,0,270,0,271,0,272,0,273,0,275,0,278,0,279,0,280,0,281,0,282,0,284,0,287,0,288,0,289,0,290,0,292,0,293,0,294,0,298,0,299,0,300,0,301,0,302,0,303,0,305,0,306,0,307,0,308,0,423,0,425,0,428,0,469,0,471,0,473,0,474,0,475,0,476,0,477,0,480,0,481,0,486,0,528,0,532,0,533,0,534,0,535,0,537,0,541,0,542,0,543,0,551,0,553,0,554,0,683,0,685,0,687,0,689,0,691,0,693,0,694,0,697,0,698,0,701,0,702,0,705,0,706,0,709,0,711,0,713,0,715,0,716,0,717,0,720,0,721,0,722,0,725,0,726,0,727,0,730,0,732,0,734,0,736,0,737,0,740,0,744,0,745,0,749,0,751,0,752,0,756,0,758,0,760,0,435,0,437,0,438,0,439,0,446,0,563,0,565,0,566,0,567,0,568,0,574,0,575,0,576,0,411,1,413,3,414,4,455,0,457,0,505,0,495,0,496,0,497,0,499,0,500,0,502,0,507,0,508,0,652,0,654,0,655,0,635,0,639,0,640,0,641,0,642,0,645,0,894,0,896,0,898,0,608,0,604,0,605,0,611,0,612,0,613,0,617,0,618,0,619,0,514,0,517,0,518,0,519,0,765,1,766,0,767,0,12,1,878,0,879,0,880,0,881,0,886,0,773,0,775,0,777,0,778,0,780,0,783,0,785,0,786,0,787,0,790,0,791,0,793,0,794,0,795,0,796,0,799,0,800,0,801,0,802,0,803,0,806,0,815,0,819,0,820,0,821,0,822,0,823,0,826,0,827,0,828,0,829,0,830,0,833,0,834,0,835,0,836,0,839,0,840,0,841,0,844,0,845,0,846,0,623,0,624,0,625,0,627,0]},{"source":"dart:_internal/async_cast.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Fasync_cast.dart","uri":"dart:_internal/async_cast.dart","_kind":"library"},"hits":[11,0,12,0,14,0,16,0,17,0,18,0,19,0,22,0,110,0,112,0,116,0,117,0,119,0,120,0,100,0,102,0,103,0,104,0,105,0,38,0,39,0,42,0,44,0,45,0,47,0,50,0,51,0,53,0,54,0,55,0,56,0,58,0,62,0,63,0,66,0,67,0,70,0,72,0,73,0,74,0,75,0,77,0,81,0,84,0,85,0,88,0,89,0,92,0,94,0]},{"source":"dart:_internal/runtime/libsymbol_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Fruntime%2Flibsymbol_patch.dart","uri":"dart:_internal/runtime/libsymbol_patch.dart","_kind":"library"},"hits":[10,1,56,0,58,0,13,0,16,0,17,0,26,0,29,0,32,0,38,0,39,0,40,0,42,0,46,0,50,0,52,0]},{"source":"dart:_internal/symbol.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Fsymbol.dart","uri":"dart:_internal/symbol.dart","_kind":"library"},"hits":[107,0,110,0,112,0,119,0,121,0,122,0,123,0,127,0,129,0,137,0,138,0]},{"source":"dart:_internal/cast.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Fcast.dart","uri":"dart:_internal/cast.dart","_kind":"library"},"hits":[99,0,101,0,102,0,105,0,106,0,109,0,110,0,113,0,114,0,117,0,118,0,122,0,123,0,126,0,127,0,130,0,131,0,134,0,135,0,138,0,140,0,142,0,144,0,145,0,148,0,149,0,152,0,153,0,155,0,156,0,159,0,160,0,163,0,164,0,167,0,168,0,257,0,258,0,261,0,262,0,265,0,266,0,269,0,270,0,273,0,274,0,275,0,278,0,279,0,282,0,283,0,286,0,287,0,187,0,189,0,191,0,192,0,194,0,195,0,198,0,200,0,201,0,204,0,205,0,208,0,209,0,212,0,213,0,216,0,218,0,219,0,220,0,223,0,224,0,225,0,228,0,229,0,230,0,231,0,232,0,237,0,239,0,240,0,243,0,244,0,245,0,249,0,251,0,12,0,35,0,36,0,37,0,39,0,40,0,42,0,43,0,44,0,45,0,47,0,51,0,52,0,53,0,55,0,82,0,174,0,176,0,60,0,61,0,62,0,294,0,296,0,298,0,300,0,302,0,304,0,305,0,308,0,309,0,311,0,312,0,315,0,317,0,318,0,321,0,322,0,327,0,329,0,331,0,333,0,335,0,337,0,338,0,339,0,342,0,343,0,346,0,347,0,351,0,352,0,353,0,357,0,358,0,68,0,70,0,71,0,72,0,74,0,77,0,365,0,366,0,119,0,323,0,348,0]},{"source":"dart:_internal/linked_list.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Flinked_list.dart","uri":"dart:_internal/linked_list.dart","_kind":"library"},"hits":[109,0,110,0,113,0,116,0,117,0,119,0,120,0,123,0,124,0,89,0,90,0,91,0,13,0,18,0,20,0,22,0,24,0,26,0,27,0,28,0,29,0,35,0,36,0,38,0,40,0,42,0,43,0,44,0,45,0,55,0,56,0,57,0,58,0,60,0,62,0,64,0,66,0,68,0,70,0,71,0,74,0]},{"source":"dart:_internal/runtime/libinternal_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Fruntime%2Flibinternal_patch.dart","uri":"dart:_internal/runtime/libinternal_patch.dart","_kind":"library"},"hits":[81,0,82,0,83,0,84,0,85,0,86,0,89,0,90,0,54,1,59,0,61,0,69,0,71,0,72,0,75,0,76,0,100,0,104,0,105,0,106,0,107,0,28,0,20,0,24,0]},{"source":"dart:_internal","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal","uri":"dart:_internal","_kind":"library"},"hits":[85,0,67,0,69,0,70,0,72,0,93,0,98,0,99,0,100,0,101,0,108,0,110,0,111,0,112,0]},{"source":"dart:_internal/runtime/libclass_id_fasta.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Fruntime%2Flibclass_id_fasta.dart","uri":"dart:_internal/runtime/libclass_id_fasta.dart","_kind":"library"},"hits":[8,0]},{"source":"dart:_internal/sort.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Fsort.dart","uri":"dart:_internal/sort.dart","_kind":"library"},"hits":[32,0,33,0,45,0,46,0,49,0,55,0,57,0,58,0,60,0,64,0,66,0,67,0,69,0,70,0,71,0,73,0,77,0,82,0,83,0,84,0,85,0,86,0,87,0,89,0,90,0,91,0,92,0,93,0,96,0,101,0,106,0,111,0,116,0,121,0,126,0,131,0,136,0,147,0,148,0,149,0,151,0,152,0,154,0,155,0,157,0,174,0,175,0,176,0,177,0,178,0,179,0,180,0,181,0,183,0,195,0,196,0,197,0,201,0,203,0,204,0,205,0,209,0,210,0,236,0,237,0,238,0,239,0,240,0,241,0,242,0,244,0,246,0,247,0,249,0,250,0,251,0,252,0,258,0,259,0,261,0,262,0,263,0,266,0,267,0,282,0,283,0,284,0,285,0,293,0,294,0,306,0,307,0,308,0,310,0,311,0,330,0,331,0,332,0,333,0,334,0,335,0,336,0,338,0,340,0,341,0,343,0,344,0,345,0,346,0,352,0,353,0,355,0,356,0,357,0,360,0,361,0,375,0,382,0]},{"source":"dart:_internal/patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Fpatch.dart","uri":"dart:_internal/patch.dart","_kind":"library"},"hits":[8,0]},{"source":"dart:_internal/runtime/libprint_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_internal%2Fruntime%2Flibprint_patch.dart","uri":"dart:_internal/runtime/libprint_patch.dart","_kind":"library"},"hits":[16,0,17,0,12,1,13,2]},{"source":"dart:math/rectangle.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Amath%2Frectangle.dart","uri":"dart:math/rectangle.dart","_kind":"library"},"hits":[149,0,150,0,151,0,166,1,167,1,168,1,169,1,165,0,170,0,21,0,33,0,35,0,37,0,38,0,41,0,42,0,43,0,44,0,45,0,46,0,49,0,50,0,62,1,63,1,66,1,67,1,61,0,65,0,69,0,70,0,79,0,80,0,81,0,82,0,83,0,90,1,91,1,93,1,94,1,89,0,96,0,102,0,103,0,104,0,105,0,106,0,112,0,113,0,114,0,115,0,116,0,119,0,120,0,121,0,122,0,123,0,209,0,210,0,211,0,226,1,227,1,228,1,229,1,225,0,230,0,233,0,244,0,245,0,246,0,249,0,260,0,261,0,262,0,271,0,273,0]},{"source":"dart:math/runtime/libmath_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Amath%2Fruntime%2Flibmath_patch.dart","uri":"dart:math/runtime/libmath_patch.dart","_kind":"library"},"hits":[185,0,200,0,202,0,204,0,205,0,208,0,210,0,211,0,217,0,218,0,219,0,220,0,224,0,225,0,228,0,229,0,243,0,245,0,247,0,249,0,250,0,163,0,164,0,166,0,167,0,168,0,169,0,170,0,174,0,175,0,255,0,257,0,261,0,263,0,264,0,266,0,267,0,273,0,274,0,275,0,279,0,280,0,283,0,284,0,86,0,87,0,91,0,92,0,93,0,95,0,97,0,100,0,101,0,104,0,106,0,109,0,111,0,114,0,115,0,116,0,118,0,120,0,121,0,148,0,149,0,150,0,151,0,152,0,153,0,154,0,155,0,156,0,157,0,17,0,21,0,22,0,24,0,25,0,26,0,29,0,30,0,34,0,39,0,46,0,50,0,51,0,53,0,54,0,55,0,58,0,59,0,63,0,68,0,72,0,128,0,79,0,80,0,81,0,83,0,130,0,132,0,134,0,136,0,138,0,140,0,142,0,144,0,146,0]},{"source":"dart:math/point.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Amath%2Fpoint.dart","uri":"dart:math/point.dart","_kind":"library"},"hits":[13,0,17,0,26,0,27,0,28,0,31,0,38,0,39,0,47,0,48,0,60,0,61,0,68,0,73,0,74,0,75,0,76,0,85,0,86,0,87,0,88,0]},{"source":"dart:math/jenkins_smi_hash.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Amath%2Fjenkins_smi_hash.dart","uri":"dart:math/jenkins_smi_hash.dart","_kind":"library"},"hits":[25,0,26,0,27,0,28,0,31,0,32,0,33,0,34,0,37,0,39,0,40,0]},{"source":"dart:_vmservice/running_isolates.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_vmservice%2Frunning_isolates.dart","uri":"dart:_vmservice/running_isolates.dart","_kind":"library"},"hits":[75,0,99,0,100,0,101,0,102,0,104,0,107,0,108,0,111,0,115,0,118,0,121,0,123,0,124,0,125,0,126,0,127,0,128,0,129,0,131,0,133,0,136,0,137,0,138,0,140,0,141,0,142,0,143,0,150,0,151,0,152,0,153,0,154,0,163,0,165,0,168,0,169,0,171,0,172,0,173,0,174,0,183,0,184,0,185,0,186,0,187,0,188,0,190,0,193,0,194,0,197,0,198,0,201,0,205,0,206,0,207,0,210,0,11,0,13,0,14,0,15,0,17,0,18,0,21,0,22,0,23,0,25,0,29,0,30,0,32,0,33,0,34,0,35,0,37,0,38,0,39,0,42,0,44,0,45,0,46,0,49,0,53,0,58,0,59,0,62,0,63,0,65,0,70,0,77,0,78,0,79,0,81,0,82,0,83,0,88,0,90,0,92,0,145,0,147,0,156,0,157,0,159,0,175,0,176,0,178,0]},{"source":"dart:_vmservice/running_isolate.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_vmservice%2Frunning_isolate.dart","uri":"dart:_vmservice/running_isolate.dart","_kind":"library"},"hits":[12,0,14,0,17,0,19,0,23,0]},{"source":"dart:_vmservice/message.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_vmservice%2Fmessage.dart","uri":"dart:_vmservice/message.dart","_kind":"library"},"hits":[7,1,51,0,54,0,55,0,56,0,57,0,62,0,65,0,67,0,68,0,73,0,76,0,78,0,82,0,85,0,87,0,100,0,106,0,109,0,110,0,113,0,116,0,117,0,118,0,11,0,14,0,29,0,30,0,31,0,32,0,33,0,35,0,36,0,38,0,39,0,41,0,42,0,44,0,45,0,47,0,90,0,94,0,97,0,121,0,122,0,125,0,129,0,130,0,131,0,132,0,133,0,134,0,135,0,136,0,139,0,140,0,141,0,143,0,144,0,148,0,157,0,161,0,162,0,163,0,168,0,169,0,170,0,174,0,175,0,176,0,177,0,178,0,179,0,180,0,181,0,182,0,183,0,184,0,185,0,189,0,192,0,200,0,202,0,203,0,204,0,205,0,206,0,207,0,208,0,209,0,216,0,217,0,218,0,222,0,223,0,224,0,225,0,226,0,229,0,230,0,231,0,232,0,233,0,234,0,235,0,237,0,239,0,241,0,244,0,247,0,248,0,251,0,252,0,255,0,256,0,260,0,263,0,265,0,171,0,172,0,219,0,220,0]},{"source":"dart:_vmservice/message_router.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_vmservice%2Fmessage_router.dart","uri":"dart:_vmservice/message_router.dart","_kind":"library"},"hits":[28,0,42,0,43,0,49,0,50,0,51,0,52,0,53,0,54,0,56,0,57,0,58,0,61,0,66,0,67,0,68,0,69,0,70,0,71,0,72,0,12,1]},{"source":"dart:_vmservice/asset.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_vmservice%2Fasset.dart","uri":"dart:_vmservice/asset.dart","_kind":"library"},"hits":[11,0,13,0,14,0,15,0,17,0,19,0,21,0,23,0,25,0,27,0,29,0,31,0,33,0,40,0,41,0,45,0,46,0,47,0,48,0,49,0,54,0,57,0,60,0,63,0,65,0]},{"source":"dart:_vmservice","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_vmservice","uri":"dart:_vmservice","_kind":"library"},"hits":[348,0,349,0,212,0,215,0,218,0,219,0,220,0,221,0,222,0,223,0,226,0,227,0,229,0,232,0,234,0,237,0,239,0,245,0,246,0,250,0,251,0,252,0,253,0,258,0,260,0,261,0,263,0,264,0,265,0,267,0,318,0,319,0,320,0,322,0,325,0,328,0,331,0,335,0,338,0,341,0,345,0,352,0,354,0,355,0,360,0,361,0,362,0,369,0,370,0,371,0,372,0,430,0,431,0,432,0,433,0,434,0,653,0,654,0,655,0,656,0,657,0,31,0,33,0,34,0,35,0,36,0,38,0,95,0,96,0,98,0,99,0,101,0,105,0,109,0,112,0,113,0,114,0,117,0,118,0,119,0,120,0,123,0,124,0,128,0,129,0,131,0,134,0,137,0,138,0,662,0,664,0,667,0,668,0,669,0,673,0,676,0,679,0,683,0,686,0,689,0,692,0,273,0,275,0,277,0,280,0,281,0,283,0,285,0,288,0,289,0,294,0,297,0,301,0,302,0,306,0,307,0,308,0,310,0,312,0,315,0,383,0,384,0,385,0,387,0,388,0,390,0,391,0,392,0,393,0,399,0,400,0,401,0,402,0,403,0,410,0,411,0,414,0,415,0,416,0,418,0,419,0,421,0,422,0,423,0,424,0,427,0,436,0,437,0,438,0,439,0,441,0,442,0,443,0,445,0,446,0,447,0,449,0,450,0,452,0,457,0,458,0,460,0,463,0,466,0,468,0,469,0,470,0,473,0,475,0,478,0,480,0,486,0,488,0,492,0,493,0,494,0,495,0,497,0,498,0,499,0,500,0,501,0,508,0,509,0,510,0,513,0,514,0,517,0,518,0,520,0,522,0,523,0,525,0,527,0,529,0,530,0,532,0,533,0,535,0,536,0,538,0,539,0,540,0,542,0,545,0,547,0,549,0,553,0,556,0,557,0,558,0,561,0,563,0,565,0,569,0,572,0,575,0,577,0,578,0,581,0,583,0,584,0,585,0,588,0,589,0,590,0,593,0,594,0,598,0,599,0,601,0,603,0,604,0,609,0,612,0,613,0,616,0,618,0,619,0,622,0,623,0,625,0,626,0,628,0,629,0,631,0,632,0,634,0,635,0,637,0,638,0,640,0,641,0,643,0,644,0,646,0,648,0,649,0,503,0,505,0,548,0,550,0]},{"source":"dart:_vmservice/named_lookup.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_vmservice%2Fnamed_lookup.dart","uri":"dart:_vmservice/named_lookup.dart","_kind":"library"},"hits":[47,0,50,0,52,0,53,0,55,0,57,0,58,0,63,0,64,0,65,0,13,0,14,0,16,0,17,0,18,0,19,0,22,0,23,0,24,0,25,0,28,0,29,0,31,0]},{"source":"dart:_vmservice/devfs.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_vmservice%2Fdevfs.dart","uri":"dart:_vmservice/devfs.dart","_kind":"library"},"hits":[71,0,85,0,90,0,91,0,92,0,94,0,95,0,98,0,99,0,23,0,28,0,29,0,30,0,32,0,37,0,38,0,42,0,45,0,48,0,49,0,53,0,54,0,61,0,62,0,64,0,65,0,7,0,8,0,12,0,13,0,14,0,17,0,18,0,19,0,102,0,103,0,104,0,105,0,106,0,107,0,108,0,109,0,110,0,111,0,112,0,113,0,114,0,115,0,116,0,117,0,119,0,120,0,124,0,127,0,130,0,133,0,135,0,136,0,138,0,140,0,145,0,147,0,148,0,150,0,152,0,155,0,157,0,160,0,161,0,164,0,165,0,166,0,167,0,168,0,171,0,174,0,176,0,178,0,180,0,181,0,183,0,185,0,187,0,188,0,189,0,190,0,193,0,196,0,198,0,200,0,202,0,203,0,205,0,207,0,209,0,210,0,213,0,216,0,218,0,220,0,222,0,223,0,225,0,227,0,230,0,232,0,233,0,234,0,236,0,237,0,239,0,242,0,245,0,247,0,249,0,250,0,252,0,254,0,258,0,259,0,260,0,262,0,263,0,267,0,270,0,272,0,274,0,276,0,277,0,279,0,281,0,284,0,286,0,287,0,288,0,290,0,291,0,293,0,296,0,299,0,301,0,303,0,304,0,306,0,308,0,311,0,313,0,315,0,316,0,318,0,320,0,321,0,324,0,327,0,329,0,331,0,333,0,334,0,336,0,338,0,340,0,342,0,344,0,345,0,347,0,348,0,349,0,350,0,351,0,352,0,353,0,354,0,355,0,358,0,360,0,361,0,364,0,366,0,367,0,368,0,369,0,371,0,372,0,375,0,378,0,380,0,382,0,384,0,385,0,387,0,389,0,391,0,393,0,394,0,396,0,397,0]},{"source":"dart:_vmservice/client.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_vmservice%2Fclient.dart","uri":"dart:_vmservice/client.dart","_kind":"library"},"hits":[28,0,29,0,36,0,37,0,41,0,44,0,47,0,48,0,52,0,56,0,62,0,63,0]},{"source":"dart:_builtin","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_builtin","uri":"dart:_builtin","_kind":"library"},"hits":[25,0,27,1,28,2,31,1,53,0,56,0,58,0,59,0,60,0,65,0,66,0,85,0,86,0,89,1,98,1,103,0,105,0,107,0,113,0,115,0,119,0,123,0,125,0,131,0,132,0,133,0,140,1,142,1,147,1,145,0,149,0,155,0,157,0,160,0,162,0,163,0,164,0,165,0,166,0,168,0,169,0,170,0,176,0,179,0,185,0,187,0,194,0,199,0,200,0,201,0,204,0,206,0,209,0,216,1,223,1,225,1,226,2,229,1,239,1,218,0,237,0,244,0,246,0,251,0,253,0,254,0,257,0,260,0,262,0,266,0,268,0,272,0,273,0,275,0,279,0,280,0,281,0,282,0,283,0,284,0,285,0,286,0,287,0,288,0,293,0,295,0,300,0,302,0,304,0,306,0,309,0,311,0,315,1,337,0,339,0,342,0,345,0,347,0,350,0,62,0,63,0,325,0,327,0,328,0,330,0,332,0,353,0,355,0,357,0,359,0,364,0,365,0,366,0,368,0,373,0]},{"source":"dart:io/stdio.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fstdio.dart","uri":"dart:io/stdio.dart","_kind":"library"},"hits":[206,0,211,0,219,0,227,0,251,0,261,0,262,0,263,0,265,0,34,0,51,0,55,0,57,0,58,0,59,0,63,0,64,0,67,0,68,0,69,0,75,0,76,0,77,0,80,0,81,0,87,0,88,0,89,0,91,0,92,0,94,0,95,0,98,0,99,0,102,0,105,0,176,0,178,0,179,0,376,1,377,0,294,0,296,0,297,0,299,0,307,0,308,0,310,0,313,0,314,0,315,0,322,0,324,0,325,0,326,0,329,0,330,0,333,0,334,0,337,0,338,0,341,0,342,0,345,0,346,0,349,0,350,0,353,0,354,0,355,0,356,0,16,0,18,0,20,0,273,0,275,0,276,0,284,0,286,0,287,0,391,0,398,0,400,0,406,0,408,0,414,0,416,0,423,0,424,0,425,0,426,0,427,0,428,0,429,0,431,0,433,0,437,0,440,0,441,0,444,0,446,0,448,0,452,0,454,0,301,0,303,0,304,0]},{"source":"dart:io/runtime/binstdio_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinstdio_patch.dart","uri":"dart:io/runtime/binstdio_patch.dart","_kind":"library"},"hits":[143,0,144,0,145,0,146,0,151,0,162,0,129,0,131,0,139,0,141,0,154,0,155,0,156,0,157,0,118,0,119,0,120,0,121,0,122,0,123,0,68,0,69,0,70,0,71,0,77,0,79,0,82,0,83,0,84,0,89,0,90,0,91,0,92,0,98,0,100,0,103,0,104,0,105,0,110,0,111,0,112,0,113,0,59,0,60,0,61,0,62,0,44,0,45,0,46,0,47,0,25,0,26,0,27,0,28,0,29,0,30,0,31,0,33,0,34,0,10,0,11,0,12,0,13,0,14,0,15,0,16,0,17,0,19,0,20,0,39,0,40,0,53,0,165,0,166,0]},{"source":"dart:io/socket.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fsocket.dart","uri":"dart:io/socket.dart","_kind":"library"},"hits":[368,0,575,0,577,0,579,0,582,0,418,0,419,0,425,0,395,0,67,0,75,0,83,0,91,0,642,0,25,1,27,0,28,0,29,0,30,0,36,0,37,0,38,0,40,0,42,0,45,0,49,0,768,0,769,1,775,0,776,0,777,0,778,0,779,0,780,0,781,0,783,0,784,0,786,0,787,0,789,0,790,0,792,0]},{"source":"dart:io/string_transformer.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fstring_transformer.dart","uri":"dart:io/string_transformer.dart","_kind":"library"},"hits":[74,0,76,0,77,0,80,0,81,0,83,0,85,0,88,0,89,0,90,0,92,0,93,0,120,0,122,0,123,0,126,0,127,0,23,1,25,0,27,0,28,0,30,0,31,0,38,0,39,0,48,1,50,0,51,0,53,0,61,0,62,0,98,1,100,0,101,0,107,0,108,0]},{"source":"dart:io/runtime/binsecure_socket_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinsecure_socket_patch.dart","uri":"dart:io/runtime/binsecure_socket_patch.dart","_kind":"library"},"hits":[126,0,127,0,131,0,132,0,136,0,17,0,10,0,11,0,141,0,142,0,144,0,148,0,152,0,153,0,154,0,157,0,160,0,161,0,162,0,165,0,168,0,169,0,170,0,173,0,176,0,177,0,178,0,181,0,184,0,186,0,187,0,190,0,192,0,203,0,206,0,207,0,208,0,209,0,211,0,215,0,216,0,217,0,218,0,220,0,224,0,225,0,226,0,227,0,229,0,232,0,233,0,234,0,235,0,239,0,240,0,243,0,244,0,29,0,27,0,31,0,35,0,41,0,42,0,43,0,45,0,48,0,49,0,50,0,52,0,73,0,74,0,75,0,76,0,77,0,81,0,89,0,90,0,91,0,94,0,96,0,98,0,100,0,102,0,104,0,107,0,109,0,111,0,114,0,118,0,23,0]},{"source":"dart:io/security_context.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fsecurity_context.dart","uri":"dart:io/security_context.dart","_kind":"library"},"hits":[206,0,207,0,208,0,210,0,214,0,215,0,216,0,217,0,219,0,220,0,224,0,225,0,230,0,232,0,233,0,236,0,240,0,241,0,242,0,243,0,247,0,248,0,254,0,271,0,272,0,273,0,276,0,277,0,281,0,256,0,257,0,258,0,260,0,261,0,262,0,265,0,268,0]},{"source":"dart:io/runtime/binfile_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinfile_patch.dart","uri":"dart:io/runtime/binfile_patch.dart","_kind":"library"},"hits":[393,0,396,0,397,0,398,0,399,0,400,0,401,0,408,0,411,0,412,0,413,0,365,0,368,0,369,0,370,0,371,0,372,0,380,0,383,0,384,0,385,0,59,0,60,0,127,0,128,0,129,0,130,0,132,0,133,0,136,0,138,0,141,0,142,0,144,0,146,0,153,0,155,0,156,0,157,0,160,0,161,0,163,0,164,0,165,0,168,0,169,0,171,0,172,0,173,0,174,0,175,0,177,0,179,0,180,0,181,0,187,0,189,0,191,0,193,0,195,0,196,0,199,0,200,0,201,0,202,0,307,0,308,0,310,0,312,0,314,0,316,0,111,0,113,0,114,0,116,0,117,0,119,0,120,0,121,0,123,0,305,0,92,0,10,0,12,0,14,0,17,0,20,0,23,0,26,0,29,0,32,0,35,0,44,0,47,0,38,0,41,0,50,0,53,0,324,0,327,0,330,0,341,0,342,0,345,0,346,0,347,0,348,0,350,0,353,0,354,0,355,0,356,0,357,0,65,0,67,0,68,0,70,0,72,0,73,0,74,0,75,0,76,0,77,0,78,0,79,0,80,0,81,0,82,0,83,0,84,0,417,0,418,0,402,0,403,0,405,0,374,0,375,0,377,0,203,0,204,0,205,0,206,0,240,0,242,0,243,0,244,0,248,0,249,0,250,0,251,0,253,0,254,0,256,0,257,0,259,0,260,0,261,0,262,0,263,0,264,0,265,0,266,0,267,0,268,0,270,0,273,0,276,0,277,0,279,0,280,0,282,0,285,0,288,0,289,0,290,0,291,0,294,0,295,0,299,0,331,0,332,0,333,0,335,0,207,0,208,0,209,0,210,0,211,0,216,0,217,0,219,0,221,0,224,0,225,0,226,0,229,0,230,0,231,0,233,0]},{"source":"dart:io/common.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fcommon.dart","uri":"dart:io/common.dart","_kind":"library"},"hits":[45,0,91,0,69,0,72,0,73,0,74,0,75,0,76,0,77,0,78,0,80,0,81,0,83,0,19,0,20,0,25,0,27,0,28,0,29,0,30,0,31,0,32,0,33,0,34,0,35,0,37,0,98,0,100,0,101,0,103,0,104,0,106,0,107,0,108,0,109,0,111,0,112,0,114,0]},{"source":"dart:io/runtime/binsocket_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinsocket_patch.dart","uri":"dart:io/runtime/binsocket_patch.dart","_kind":"library"},"hits":[1415,0,1418,0,1419,0,1373,0,1375,0,1431,0,1433,0,1434,0,1435,0,1436,0,1437,0,1456,0,1459,0,1460,0,1461,0,1464,0,1465,0,1468,0,1469,0,1470,0,1471,0,1472,0,1474,0,1476,0,1477,0,1478,0,1479,0,1484,0,1485,0,1487,0,1489,0,1491,0,1495,0,1496,0,1497,0,1498,0,1499,0,1500,0,83,0,68,0,69,0,73,0,77,0,576,0,577,0,579,0,581,0,582,0,585,0,587,0,588,0,589,0,590,0,593,0,596,0,351,0,353,0,354,0,366,0,367,0,368,0,377,0,381,0,382,0,403,0,405,0,406,0,407,0,408,0,411,0,419,0,554,0,555,0,598,0,599,0,600,0,601,0,602,0,603,0,605,0,606,0,607,0,614,1,609,0,610,0,611,0,613,0,615,0,616,0,617,0,618,0,622,0,625,0,626,0,631,0,632,0,637,0,638,0,639,0,640,0,641,0,649,0,652,0,653,0,658,0,659,0,664,0,665,0,668,0,669,0,671,0,673,0,674,0,675,0,676,0,678,0,679,0,681,0,682,0,684,0,686,0,687,0,689,0,695,0,696,0,699,0,702,0,703,0,708,0,710,0,711,0,713,0,714,0,715,0,716,0,718,0,723,0,724,0,729,0,731,0,733,0,734,0,735,0,736,0,737,0,738,0,739,0,740,0,743,0,745,0,750,0,751,0,752,0,753,0,754,0,755,0,758,0,759,0,760,0,761,0,762,0,765,0,767,0,768,0,769,0,770,0,771,0,772,0,773,0,776,0,777,0,778,0,779,0,801,0,804,0,805,0,806,0,819,0,820,0,822,0,827,0,831,0,832,0,833,0,834,0,835,0,836,0,837,0,841,0,842,0,843,0,847,0,848,0,849,0,851,0,852,0,857,0,858,0,864,0,865,0,867,0,868,0,869,0,870,0,874,0,875,0,876,0,878,0,881,0,885,0,886,0,887,0,891,0,892,0,895,0,897,0,898,0,903,0,904,0,905,0,906,0,907,0,908,0,911,0,912,0,913,0,914,0,915,0,916,0,917,0,918,0,919,0,920,0,921,0,925,0,926,0,927,0,928,0,930,0,933,0,934,0,936,0,937,0,939,0,940,0,942,0,943,0,946,0,951,0,952,0,953,0,954,0,956,0,958,0,962,0,963,0,964,0,965,0,967,0,969,0,973,0,974,0,976,0,977,0,980,0,982,0,983,0,986,0,988,0,995,0,997,0,998,0,1001,0,1005,0,1006,0,1011,0,1013,0,1014,0,1016,0,1018,0,1019,0,1020,0,1021,0,1022,0,1023,0,1027,0,1030,0,1034,0,1035,0,1037,0,1038,0,1041,0,1044,0,1045,0,1046,0,1047,0,1051,0,1052,0,1053,0,1054,0,1057,0,1061,0,1063,0,1064,0,1065,0,1069,0,1074,0,1081,0,1082,0,1083,0,1084,0,1085,0,1086,0,1089,0,1090,0,1091,0,1092,0,1093,0,1094,0,1097,0,1098,0,1099,0,1100,0,1101,0,1103,0,1105,0,1106,0,1108,0,1109,0,1111,0,1113,0,1114,0,1115,0,1116,0,1117,0,1118,0,1119,0,1121,0,1123,0,10,0,12,0,1236,0,1237,0,1238,0,1240,0,1241,0,1242,0,1243,0,1244,0,1257,0,1229,0,1232,0,1233,0,1263,0,1264,0,1265,0,1266,0,1267,0,1270,0,1271,0,1272,0,1273,0,1274,0,1276,0,1277,0,1278,0,1283,0,1285,0,1289,0,1291,0,1292,0,1293,0,1294,0,1295,0,1296,0,1299,0,1303,0,1307,0,1308,0,1310,0,1312,0,1314,0,1316,0,1318,0,1320,0,1322,0,1323,0,1324,0,1325,0,1326,0,1330,0,1331,0,1332,0,1333,0,1334,0,1338,0,1339,0,1341,0,1342,0,1345,0,1346,0,1349,0,1350,0,1351,0,1353,0,1357,0,1358,0,1359,0,1361,0,1365,0,1366,0,230,0,232,0,233,0,28,0,29,0,33,0,34,0,38,0,39,0,43,0,44,0,48,0,49,0,53,0,55,0,59,0,61,0,158,0,111,0,115,0,117,0,119,0,120,0,121,0,122,0,124,0,125,0,126,0,128,0,132,0,133,0,134,0,136,0,138,0,140,0,144,0,145,0,146,0,148,0,150,0,152,0,156,0,160,0,161,0,162,0,164,0,166,0,168,0,171,0,173,0,174,0,175,0,176,0,177,0,178,0,179,0,180,0,181,0,182,0,183,0,184,0,185,0,186,0,187,0,190,0,195,0,196,0,197,0,200,0,201,0,202,0,204,0,205,0,210,0,212,0,213,0,218,0,219,0,222,0,1735,0,1737,0,1389,0,1382,0,1385,0,1386,0,1391,0,1393,0,1400,0,1402,0,1404,0,1405,0,1407,0,1408,0,1142,0,1133,0,1135,0,1136,0,1138,0,1139,0,1144,0,1146,0,1147,0,1149,0,1150,0,1152,0,1153,0,1154,0,1155,0,1156,0,1163,0,1173,0,1177,0,1179,0,1181,0,1182,0,1191,0,1192,0,1195,0,1196,0,1199,0,1200,0,1201,0,1203,0,1207,0,1208,0,1209,0,1211,0,1215,0,1216,0,19,0,21,0,1514,0,1515,0,1517,0,1518,0,1519,0,1520,0,1521,0,1522,0,1525,0,1528,0,1531,0,1532,0,1535,0,1536,0,1543,0,1545,0,1547,0,1551,0,1553,0,1554,0,1557,0,1559,0,1561,0,1563,0,1565,0,1567,0,1568,0,1571,0,1572,0,1575,0,1577,0,1579,0,1581,0,1583,0,1584,0,1585,0,1586,0,1587,0,1590,0,1591,0,1592,0,1595,0,1596,0,1598,0,1601,0,1602,0,1604,0,1607,0,1608,0,1610,0,1613,0,1614,0,1616,0,1619,0,1620,0,1621,0,1622,0,1633,0,1634,0,1635,0,1636,0,1640,0,1641,0,1642,0,1643,0,1644,0,1647,0,1648,0,1649,0,1651,0,1652,0,1655,0,1656,0,1657,0,1662,0,1663,0,1664,0,1668,0,1670,0,1671,0,1672,0,1674,0,1675,0,1677,0,1678,0,1679,0,1684,0,1685,0,1686,0,1687,0,1689,0,1692,0,1693,0,1694,0,1695,0,1696,0,1698,0,1701,0,1702,0,1704,0,1705,0,1708,0,1709,0,1710,0,1714,0,1715,0,1716,0,1718,0,1719,0,1720,0,1725,0,1728,0,1748,0,1749,0,1750,0,1752,0,1753,0,1754,0,1755,0,1756,0,1769,0,1775,0,1776,0,1778,0,1779,0,1782,0,1784,0,1788,0,1790,0,1791,0,1793,0,1794,0,1797,0,1798,0,1801,0,1802,0,1805,0,1806,0,1807,0,1808,0,1809,0,1813,0,1814,0,1815,0,1816,0,1817,0,1821,0,1822,0,1823,0,1824,0,1826,0,1827,0,1828,0,1830,0,1831,0,1834,0,1835,0,1836,0,1838,0,1840,0,1842,0,1843,0,1846,0,1847,0,1850,0,1851,0,1852,0,1854,0,1858,0,1859,0,1860,0,1862,0,86,0,87,0,88,0,1867,0,1869,0,1440,0,1441,0,1443,0,1445,0,1446,0,1447,0,1449,0,1450,0,1451,0,1452,0,1453,0,355,0,356,0,358,0,361,0,369,0,370,0,372,0,383,0,384,0,386,0,398,0,412,0,413,0,420,0,421,0,423,0,517,0,518,0,522,0,523,0,526,0,527,0,528,0,530,0,534,0,536,0,538,0,540,0,541,0,542,0,543,0,544,0,545,0,548,0,549,0,550,0,558,0,560,0,562,0,564,0,566,0,567,0,568,0,571,0,572,0,780,0,781,0,782,0,783,0,784,0,785,0,786,0,787,0,789,0,790,0,794,0,796,0,797,0,798,0,807,0,808,0,809,0,810,0,811,0,812,0,813,0,815,0,1245,0,1246,0,1249,0,1250,0,1252,0,1253,0,1254,0,1255,0,1258,0,1259,0,1157,0,1158,0,1160,0,1161,0,1164,0,1165,0,1166,0,1167,0,1168,0,1169,0,1170,0,1183,0,1184,0,1185,0,1624,0,1625,0,1626,0,1757,0,1758,0,1761,0,1762,0,1764,0,1765,0,1766,0,1767,0,1770,0,1771,0,359,0,360,0,387,0,388,0,389,0,390,0,391,0,392,0,393,0,394,0,395,0,414,0,415,0,425,0,426,0,437,0,439,0,441,0,442,0,445,0,447,0,451,0,452,0,453,0,456,0,459,0,460,0,462,0,465,0,466,0,467,0,469,0,472,0,476,0,478,0,479,0,484,0,485,0,486,0,488,0,491,0,513,0,427,0,428,0,429,0,430,0,431,0,432,0,433,0,492,0,494,0,496,0,497,0,498,0,499,0,505,0,506,0,507,0,510,0,511,0,500,0,501,0,502,0,503,0]},{"source":"dart:io/link.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Flink.dart","uri":"dart:io/link.dart","_kind":"library"},"hits":[161,0,162,0,163,0,166,0,167,0,170,0,171,0,172,0,175,0,177,0,179,0,181,0,183,0,185,0,186,0,187,0,190,0,192,0,194,0,203,0,205,0,207,0,208,0,210,0,211,0,215,0,216,0,217,0,218,0,219,0,220,0,221,0,223,0,224,0,229,0,234,0,235,0,238,0,243,0,246,0,248,0,249,0,250,0,252,0,253,0,261,0,263,0,265,0,266,0,269,0,270,0,271,0,280,0,281,0,282,0,283,0,286,0,287,0,288,0,297,0,298,0,299,0,303,0,304,0,305,0,309,0,310,0,313,0,315,0,316,0,317,0,318,0,319,0,320,0,321,0,323,0,15,0,16,0,18,0,20,0,23,0,25,0,37,0,193,0,195,0,196,0,197,0,254,0,255,0,272,0,273,0,274,0,276,0,289,0,290,0,291,0]},{"source":"dart:io/runtime/binfilter_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinfilter_patch.dart","uri":"dart:io/runtime/binfilter_patch.dart","_kind":"library"},"hits":[23,0,25,0,27,0,15,0,16,0,18,0,8,0,10,0,34,0,42,0,45,0,47,0]},{"source":"dart:io/file_system_entity.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Ffile_system_entity.dart","uri":"dart:io/file_system_entity.dart","_kind":"library"},"hits":[954,0,957,0,103,0,106,0,123,0,124,0,126,0,128,0,131,0,133,0,134,0,136,0,137,0,138,0,139,0,140,0,141,0,142,0,143,0,144,0,155,0,156,0,158,0,160,0,163,0,165,0,166,0,168,0,185,0,186,0,187,0,188,0,189,0,190,0,191,0,202,0,203,0,205,0,206,0,207,0,208,0,210,0,211,0,212,0,213,0,981,0,984,0,997,0,1000,0,1001,0,1002,0,1003,0,1004,0,1005,0,40,1,42,0,43,0,947,0,970,0,973,0,974,0,269,0,355,0,356,0,357,0,395,0,396,0,397,0,414,0,427,0,447,0,448,0,466,0,499,0,502,0,503,0,505,0,507,0,513,0,514,0,515,0,538,0,539,0,541,0,543,0,556,0,557,0,558,0,560,0,575,0,576,0,577,0,578,0,579,0,580,0,582,0,586,0,587,0,588,0,590,0,591,0,592,0,593,0,594,0,596,0,597,0,598,0,602,0,603,0,604,0,620,0,621,0,623,0,625,0,633,0,634,0,636,0,638,0,643,0,644,0,646,0,650,0,651,0,652,0,659,0,664,0,665,0,667,0,683,0,685,0,701,0,702,0,708,0,710,0,711,0,716,0,717,0,722,0,723,0,724,0,730,0,732,0,733,0,739,0,740,0,746,0,747,0,769,0,771,0,772,0,774,0,775,0,776,0,779,0,784,0,785,0,786,0,787,0,788,0,797,0,799,0,801,0,802,0,803,0,806,0,808,0,810,0,812,0,813,0,816,0,818,0,819,0,828,0,830,0,832,0,834,0,835,0,838,0,839,0,840,0,841,0,847,0,849,0,850,0,851,0,852,0,853,0,856,0,857,0,864,0,866,0,867,0,868,0,869,0,870,0,873,0,874,0,169,0,170,0,174,0,175,0,176,0,177,0,178,0,179,0,180,0,181,0,358,0,359,0,360,0,516,0,517,0,518,0,820,0,821,0,822,0,824,0]},{"source":"dart:io/runtime/binfile_system_entity_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinfile_system_entity_patch.dart","uri":"dart:io/runtime/binfile_system_entity_patch.dart","_kind":"library"},"hits":[10,0,16,0,19,0,22,0]},{"source":"dart:io/data_transformer.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fdata_transformer.dart","uri":"dart:io/data_transformer.dart","_kind":"library"},"hits":[548,0,549,0,552,0,553,0,555,0,557,0,561,0,451,0,455,0,462,0,463,0,464,0,465,0,466,0,467,0,475,0,476,0,477,0,479,0,593,0,595,0,596,0,599,0,600,0,601,0,602,0,604,0,606,0,607,0,608,0,610,0,611,0,614,0,618,0,621,0,622,0,625,0,628,0,629,0,632,0,635,0,636,0,382,0,390,0,391,0,392,0,393,0,400,0,401,0,402,0,403,0,404,0,405,0,413,0,414,0,415,0,417,0,418,0,581,0,584,0,565,0,576,0,275,0,283,0,284,0,285,0,286,0,289,0,301,0,303,0,304,0,305,0,306,0,307,0,308,0,313,0,314,0,491,0,500,0,508,0,513,0,158,0,166,0,167,0,168,0,169,0,172,0,184,0,186,0,187,0,188,0,189,0,190,0,191,0,196,0,197,0,640,0,641,0,642,0,643,0,648,0,649,0,650,0,654,0,655,0,656,0,661,0,669,0,670,0]},{"source":"dart:io/bytes_builder.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fbytes_builder.dart","uri":"dart:io/bytes_builder.dart","_kind":"library"},"hits":[90,0,91,0,92,0,93,0,95,0,96,0,97,0,98,0,99,0,100,0,103,0,104,0,106,0,107,0,110,0,113,0,114,0,117,0,120,0,121,0,124,0,127,0,128,0,131,0,133,0,134,0,135,0,138,0,139,0,140,0,141,0,145,0,146,0,147,0,148,0,151,0,153,0,155,0,157,0,158,0,159,0,162,0,164,0,165,0,166,0,167,0,168,0,169,0,170,0,178,0,180,0,183,0,185,0,186,0,189,0,190,0,191,0,194,0,195,0,196,0,197,0,198,0,201,0,203,0,204,0,205,0,207,0,211,0,212,0,213,0,215,0,216,0,217,0,222,0,224,0,226,0,228,0,229,0,230,0,22,0,24,0,26,0]},{"source":"dart:io/file.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Ffile.dart","uri":"dart:io/file.dart","_kind":"library"},"hits":[250,0,251,0,253,0,255,0,263,0,269,0,271,0,44,0,97,0,978,0,980,0,981,0,982,0,983,0,984,0,985,0,986,0,988,0,989,0,991,0,992,0,993,0,994,0,996,0,997,0,999,0]},{"source":"dart:io/secure_socket.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fsecure_socket.dart","uri":"dart:io/secure_socket.dart","_kind":"library"},"hits":[1078,0,1079,0,1082,0,1084,0,1085,0,1086,0,1092,0,1094,0,1095,0,1096,0,1102,0,1104,0,1106,0,1108,0,1110,0,1111,0,1112,0,1113,0,1120,1,1127,1,1116,0,1118,0,1122,0,1123,0,1126,0,1128,0,1129,0,1130,0,1140,1,1147,1,1135,0,1136,0,1137,0,1142,0,1143,0,1144,0,1145,0,1146,0,1152,0,1154,0,1156,0,1158,0,1159,0,1160,0,1161,0,1162,0,1163,0,1164,0,1169,0,1172,0,1173,0,1174,0,1175,0,1176,0,1247,0,1222,0,1225,0,1227,0,1228,0,1229,0,1230,0,1231,0,1232,0,1233,0,1235,0,1236,0,1238,0,460,0,472,0,473,0,475,0,477,0,478,0,479,0,480,0,481,0,484,0,485,0,486,0,487,0,488,0,490,0,491,0,492,0,495,0,496,0,498,0,499,0,500,0,504,0,505,0,506,0,508,0,509,0,510,0,511,0,515,0,516,0,517,0,518,0,519,0,520,0,521,0,523,0,525,0,389,0,390,0,426,0,437,0,439,0,440,0,442,0,444,0,456,0,457,0,529,0,531,0,532,0,536,0,543,0,544,0,546,0,547,0,549,0,550,0,552,0,553,0,555,0,556,0,558,0,559,0,563,0,565,0,567,0,569,0,570,0,573,0,574,0,576,0,579,0,580,0,581,0,584,0,585,0,588,0,589,0,590,0,591,0,592,0,594,0,596,0,597,0,598,0,599,0,600,0,602,0,603,0,605,0,606,0,609,0,610,0,611,0,612,0,613,0,614,0,615,0,616,0,617,0,621,0,622,0,623,0,624,0,625,0,626,0,627,0,632,0,634,0,635,0,637,0,641,0,643,0,644,0,645,0,648,0,649,0,650,0,651,0,653,0,654,0,656,0,659,0,660,0,665,0,666,0,667,0,668,0,670,0,671,0,672,0,674,0,675,0,678,0,680,0,683,0,684,0,685,0,687,0,691,0,693,0,695,0,696,0,697,0,698,0,699,0,700,0,703,0,704,0,705,0,708,0,710,0,711,0,712,0,713,0,714,0,715,0,718,0,722,0,723,0,724,0,727,0,728,0,729,0,732,0,733,0,734,0,738,0,739,0,741,0,745,0,747,0,749,0,752,0,753,0,754,0,755,0,756,0,757,0,758,0,759,0,760,0,763,0,765,0,766,0,767,0,768,0,769,0,772,0,777,0,779,0,780,0,781,0,782,0,783,0,785,0,789,0,793,0,794,0,797,0,799,0,800,0,801,0,804,0,805,0,806,0,807,0,809,0,811,0,813,0,818,0,819,0,820,0,822,0,823,0,824,0,825,0,829,0,830,0,831,0,833,0,838,0,839,0,844,0,845,0,846,0,849,0,850,0,853,0,854,0,855,0,856,0,904,0,908,0,909,0,910,0,911,0,914,0,915,0,916,0,917,0,920,0,921,0,927,0,928,0,929,0,930,0,931,0,933,0,937,0,938,0,939,0,940,0,942,0,947,0,948,0,949,0,950,0,951,0,952,0,953,0,954,0,958,0,959,0,960,0,961,0,962,0,963,0,964,0,965,0,966,0,971,0,972,0,973,0,974,0,975,0,976,0,977,0,978,0,982,0,983,0,984,0,985,0,986,0,987,0,988,0,989,0,990,0,994,0,995,0,44,0,50,0,55,0,86,0,90,0,91,0,97,0,121,0,127,0,128,0,135,0,203,0,208,0,210,0,246,0,252,0,253,0,254,0,255,0,287,0,294,0,295,0,296,0,1257,0,370,0,857,0,858,0,859,0,860,0,861,0,864,0,865,0,867,0,868,0,872,0,873,0,874,0,875,0,876,0,880,0,882,0,885,0,886,0,887,0,888,0,890,0,891,0,893,0,894,0,896,0,897,0,899,0,900,0,903,0,996,0,999,0,1000,0,1004,0,1005,0,1011,0,1014,0,1015,0,1018,0,1022,0,1023,0,1025,0,1026,0,1027,0,1028,0,1029,0,1030,0,1032,0,1034,0,1035,0,1036,0,1037,0,1038,0,1039,0,1041,0,1043,0,1044,0,1045,0,1046,0,1047,0,1048,0,1050,0,1052,0,1053,0,1054,0,1055,0,1056,0,1057,0,1059,0,92,0,93,0,129,0,130,0,211,0,1008,0,1009,0]},{"source":"dart:io/process.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fprocess.dart","uri":"dart:io/process.dart","_kind":"library"},"hits":[521,0,653,0,655,0,657,0,658,0,660,0,172,0,162,0,168,0,169,0,685,0,687,0,688,0,689,0,690,0,625,1,627,0,646,0,45,0,46,0,47,0,50,0,53,0,68,0,69,0,70,0,72,0,85,0,93,0,94,0,95,0,96,0,99,0,102,0,108,0]},{"source":"dart:io/io_resource_info.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fio_resource_info.dart","uri":"dart:io/io_resource_info.dart","_kind":"library"},"hits":[96,0,97,0,100,0,102,0,105,0,107,0,110,0,111,0,114,0,116,0,117,0,118,0,121,0,122,0,125,0,127,0,129,0,130,0,131,0,134,0,135,0,18,0,16,0,26,0,28,0,29,0,30,0,33,0,212,0,213,0,216,0,217,0,218,0,222,0,223,0,224,0,226,0,229,0,230,0,233,0,234,0,235,0,236,0,237,0,238,0,239,0,241,0,242,0,245,0,246,0,249,0,250,0,252,0,256,0,259,0,261,0,262,0,263,0,266,0,268,0,269,0,270,0,273,0,275,0,278,0,280,0,147,0,148,0,150,0,153,0,155,0,156,0,159,0,160,0,161,0,162,0,163,0,164,0,165,0,167,0,170,0,172,0,175,0,177,0,180,0,181,0,183,0,186,0,187,0,188,0,191,0,193,0,194,0,195,0,196,0,197,0,198,0,66,0,47,0,48,0,49,0,50,0,56,0,57,0,60,0,61,0,62,0,63,0,75,0,76,0,77,0,78,0,79,0,80,0,81,0,82,0,83,0,84,0]},{"source":"dart:io/directory.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fdirectory.dart","uri":"dart:io/directory.dart","_kind":"library"},"hits":[129,0,130,0,132,0,134,0,137,0,139,0,147,0,153,0,154,0,156,0,158,0,188,0,189,0,191,0,194,0,229,0,230,0,232,0,234,0]},{"source":"dart:io/overrides.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Foverrides.dart","uri":"dart:io/overrides.dart","_kind":"library"},"hits":[37,0,38,0,46,0,54,0,90,0,120,0,121,0,130,0,132,0,133,0,144,0,150,0,156,0,157,0,164,0,172,0,180,0,181,0,188,0,189,0,199,0,200,0,208,0,209,0,216,0,217,0,224,0,225,0,234,0,235,0,242,0,250,0,258,0,260,0,298,0,331,0,332,0,333,0,334,0,338,0,339,0,340,0,341,0,345,0,346,0,347,0,348,0,349,0,351,0,355,0,356,0,357,0,358,0,363,0,364,0,365,0,366,0,371,0,372,0,373,0,374,0,378,0,379,0,380,0,381,0,386,0,387,0,388,0,389,0,393,0,394,0,395,0,396,0,400,0,401,0,402,0,403,0,407,0,408,0,409,0,410,0,415,0,416,0,417,0,418,0,422,0,423,0,424,0,425,0,430,0,431,0,432,0,433,0,438,0,440,0,441,0,444,0,445,0,448,0]},{"source":"dart:io/secure_server_socket.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fsecure_server_socket.dart","uri":"dart:io/secure_server_socket.dart","_kind":"library"},"hits":[134,0,140,0,142,0,143,0,144,0,145,0,198,0,207,0,208,0,216,0,218,0,225,0,230,0,236,0,237,0,238,0,241,0,244,0,251,0,252,0,255,0,256,0,257,0,258,0,264,0,271,0,272,0,273,0,275,0,279,0,280,0,281,0,282,0,284,0,288,0,289,0,16,0,69,0,78,0,85,0,88,0,90,0,100,0,105,0,111,0,113,0,114,0,259,0,260,0,262,0,265,0,266,0]},{"source":"dart:io/runtime/binsync_socket_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinsync_socket_patch.dart","uri":"dart:io/runtime/binsync_socket_patch.dart","_kind":"library"},"hits":[65,0,67,0,71,0,72,0,75,0,79,0,80,0,84,0,116,0,119,0,120,0,122,0,123,0,124,0,126,0,129,0,130,0,133,0,136,0,137,0,140,0,141,0,144,0,145,0,148,0,149,0,152,0,153,0,156,0,159,0,160,0,161,0,162,0,163,0,169,0,171,0,172,0,175,0,179,0,181,0,182,0,186,0,187,0,188,0,189,0,194,0,195,0,196,0,197,0,200,0,201,0,202,0,203,0,206,0,208,0,209,0,212,0,213,0,214,0,216,0,220,0,221,0,222,0,223,0,226,0,227,0,229,0,232,0,233,0,238,0,239,0,242,0,243,0,248,0,249,0,252,0,253,0,257,0,258,0,260,0,261,0,263,0,264,0,267,0,271,0,272,0,275,0,276,0,278,0,280,0,283,0,284,0,287,0,288,0,290,0,292,0,295,0,296,0,297,0,298,0,300,0,301,0,302,0,303,0,306,0,309,0,310,0,315,0,316,0,317,0,318,0,319,0,322,0,323,0,327,0,328,0,334,0,336,0,338,0,339,0,340,0,341,0,342,0,343,0,345,0,346,0,347,0,18,0,20,0,21,0,22,0,23,0,26,0,27,0,28,0,29,0,31,0,33,0,35,0,36,0,38,0,40,0,42,0,43,0,10,0,11,0,85,0,86,0,91,0,92,0,93,0,94,0,95,0,98,0,100,0,104,0,107,0,109,0,111,0]},{"source":"dart:io/runtime/binprocess_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinprocess_patch.dart","uri":"dart:io/runtime/binprocess_patch.dart","_kind":"library"},"hits":[16,0,22,0,28,0,36,0,40,0,47,0,59,0,66,0,78,0,79,0,80,0,82,0,146,0,162,0,163,0,164,0,166,0,137,0,139,0,141,0,143,0,145,0,148,0,149,0,150,0,151,0,152,0,153,0,154,0,155,0,156,0,157,0,159,0,190,0,191,0,182,0,183,0,184,0,173,0,174,0,175,0,10,0,207,0,217,0,219,0,225,0,226,0,229,0,230,0,232,0,234,0,235,0,237,0,238,0,239,0,240,0,241,0,242,0,244,0,245,0,246,0,250,0,251,0,252,0,255,0,258,0,259,0,261,0,269,0,279,0,280,0,282,0,284,0,286,0,288,0,290,0,292,0,293,0,295,0,296,0,299,0,300,0,301,0,302,0,303,0,304,0,306,0,307,0,308,0,311,0,312,0,313,0,316,0,317,0,323,0,325,0,326,0,327,0,328,0,329,0,330,0,333,0,334,0,335,0,336,0,337,0,338,0,339,0,341,0,346,0,348,0,349,0,350,0,356,0,357,0,359,0,360,0,362,0,363,0,364,0,365,0,367,0,368,0,369,0,371,0,372,0,373,0,375,0,376,0,380,0,381,0,382,0,383,0,384,0,385,0,387,0,388,0,394,0,395,0,396,0,397,0,398,0,401,0,402,0,403,0,404,0,408,0,466,0,469,0,470,0,471,0,472,0,473,0,474,0,475,0,476,0,477,0,478,0,479,0,480,0,481,0,482,0,485,0,486,0,489,0,491,0,492,0,499,0,501,0,502,0,503,0,504,0,505,0,508,0,521,0,524,0,525,0,528,0,529,0,532,0,533,0,536,0,538,0,539,0,540,0,543,0,544,0,547,0,94,0,95,0,96,0,99,0,101,0,102,0,103,0,104,0,105,0,108,0,109,0,110,0,120,0,121,0,122,0,123,0,127,0,128,0,132,0,568,0,579,0,584,0,614,0,623,0,631,0,262,0,263,0,264,0,266,0,273,0,274,0,409,0,410,0,411,0,412,0,413,0,414,0,415,0,416,0,417,0,418,0,419,0,420,0,423,0,424,0,428,0,429,0,433,0,436,0,437,0,464,0,494,0,496,0,111,0,112,0,113,0,114,0,585,0,588,0,605,0,606,0,608,0,455,0,456,0,457,0,458,0,459,0,591,0,594,0,595,0,597,0,601,0,609,0,438,0,439,0,440,0,442,0,445,0,446,0,447,0,449,0,450,0,452,0,598,0,599,0]},{"source":"dart:io/runtime/binio_service_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinio_service_patch.dart","uri":"dart:io/runtime/binio_service_patch.dart","_kind":"library"},"hits":[15,0,17,0,18,0,19,0,20,0,21,0,23,0,24,0,26,0,31,0,32,0,36,0,37,0,38,0,39,0,43,0,75,0,77,0,78,0,79,0,90,0,92,0,96,0,97,0,98,0,55,0,58,0,59,0,60,0,61,0,62,0,63,0,65,0,67,0,68,0,69,0,72,0,81,0,82,0,83,0,84,0]},{"source":"dart:io/runtime/binnamespace_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinnamespace_patch.dart","uri":"dart:io/runtime/binnamespace_patch.dart","_kind":"library"},"hits":[41,0,44,0,36,0,37,0,6,0,8,0,10,0,12,0,17,0,18,0,21,0,25,0,30,0]},{"source":"dart:io/runtime/binplatform_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbinplatform_patch.dart","uri":"dart:io/runtime/binplatform_patch.dart","_kind":"library"},"hits":[43,1,44,1,10,0,12,0,14,0,16,0,18,0,20,0,22,0,24,0,26,0,35,0,37,0,29,0,32,0,40,0,45,0,46,0,47,0,48,0,49,0,50,0,51,0,53,0]},{"source":"dart:io/platform_impl.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fplatform_impl.dart","uri":"dart:io/platform_impl.dart","_kind":"library"},"hits":[45,0,46,0,47,0,57,0,58,0,59,0,60,0,63,0,65,0,66,0,74,0,75,0,76,0,82,0,84,0,86,0,87,0,88,0,90,0,91,0,92,0,101,0,102,0,103,0,104,0,107,0,113,0,120,0,128,0,129,0,130,0,131,0,132,0,133,0,136,0,137,0,140,0,141,0,144,0,146,0,147,0,150,0,151,0,154,0,155,0,156,0,157,0,158,0,160,0,162,0,163,0,165,0,166,0,168,0,169,0,172,0,173,0,176,0]},{"source":"dart:io/file_impl.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Ffile_impl.dart","uri":"dart:io/file_impl.dart","_kind":"library"},"hits":[30,0,31,0,34,0,36,0,38,0,39,0,43,0,44,0,46,0,47,0,54,0,55,0,56,0,58,0,65,0,66,0,79,1,69,0,71,0,72,0,73,0,76,0,78,0,80,0,81,0,82,0,83,0,84,0,85,0,90,0,107,0,116,0,117,0,118,0,119,0,120,0,148,0,149,0,150,0,151,0,154,0,156,0,681,0,682,0,683,0,684,0,687,0,688,0,689,0,693,0,699,0,701,0,707,0,708,0,709,0,718,0,719,0,720,0,721,0,722,0,724,0,725,0,728,0,729,0,738,0,739,0,740,0,741,0,742,0,744,0,748,0,749,0,750,0,752,0,762,0,763,0,764,0,765,0,767,0,768,0,769,0,771,0,775,0,776,0,777,0,778,0,779,0,781,0,782,0,783,0,785,0,786,0,798,0,799,0,800,0,801,0,802,0,803,0,805,0,806,0,809,0,810,0,811,0,813,0,817,0,818,0,819,0,821,0,830,0,831,0,832,0,833,0,835,0,836,0,837,0,839,0,843,0,845,0,846,0,847,0,848,0,850,0,851,0,852,0,856,0,858,0,861,0,862,0,863,0,864,0,865,0,866,0,875,0,876,0,877,0,878,0,879,0,880,0,882,0,883,0,887,0,888,0,889,0,890,0,891,0,893,0,896,0,898,0,899,0,901,0,902,0,905,0,906,0,907,0,909,0,910,0,913,0,914,0,922,0,923,0,924,0,925,0,926,0,931,0,932,0,933,0,941,0,942,0,943,0,944,0,945,0,949,0,950,0,958,0,959,0,960,0,961,0,962,0,966,0,967,0,975,0,976,0,977,0,978,0,979,0,984,0,985,0,993,0,994,0,995,0,996,0,997,0,1007,0,1009,0,1011,0,1012,0,1014,0,1015,0,1017,0,1018,0,1019,0,1027,0,1028,0,1029,0,1031,0,1032,0,1034,0,1035,0,1043,0,1045,0,1046,0,1047,0,1049,0,1050,0,1052,0,1053,0,1054,0,1055,0,1059,0,1060,0,1061,0,1062,0,1064,0,1065,0,1067,0,1068,0,1069,0,1080,0,1082,0,1083,0,1084,0,1086,0,1088,0,1093,0,1095,0,1096,0,1097,0,1102,0,1103,0,1104,0,1105,0,1107,0,1108,0,209,0,210,0,211,0,213,0,214,0,217,0,219,0,221,0,222,0,225,0,232,0,234,0,235,0,236,0,239,0,240,0,241,0,251,0,252,0,253,0,257,0,259,0,261,0,263,0,265,0,280,0,282,0,284,0,285,0,288,0,290,0,292,0,293,0,305,0,307,0,309,0,310,0,313,0,314,0,315,0,330,0,331,0,332,0,333,0,336,0,337,0,338,0,350,0,351,0,352,0,353,0,356,0,357,0,358,0,359,0,360,0,361,0,362,0,363,0,365,0,366,0,374,0,375,0,376,0,387,0,388,0,389,0,393,0,394,0,395,0,406,0,407,0,408,0,409,0,412,0,413,0,414,0,415,0,416,0,427,0,428,0,429,0,430,0,431,0,432,0,436,0,437,0,438,0,449,0,450,0,451,0,452,0,455,0,456,0,457,0,458,0,459,0,471,0,472,0,473,0,474,0,475,0,476,0,482,0,483,0,484,0,485,0,486,0,487,0,488,0,490,0,491,0,492,0,497,0,498,0,499,0,500,0,502,0,505,0,506,0,509,0,510,0,511,0,512,0,513,0,514,0,516,0,517,0,520,0,539,0,550,0,551,0,554,0,555,0,557,0,559,0,560,0,561,0,562,0,564,0,568,0,572,0,574,0,576,0,577,0,581,0,585,0,586,0,595,0,596,0,598,0,599,0,601,0,602,0,604,0,606,0,614,0,616,0,618,0,619,0,621,0,625,0,630,0,632,0,636,0,640,0,643,0,645,0,646,0,647,0,166,0,167,0,170,0,171,0,174,0,175,0,176,0,196,0,197,0,200,0,201,0,48,0,49,0,50,0,60,0,61,0,62,0,91,0,92,0,93,0,96,0,97,0,98,0,100,0,101,0,103,0,104,0,105,0,108,0,109,0,110,0,111,0,124,0,125,0,126,0,127,0,130,0,131,0,132,0,138,0,142,0,143,0,144,0,145,0,710,0,711,0,713,0,714,0,730,0,731,0,733,0,753,0,754,0,756,0,757,0,787,0,788,0,790,0,791,0,792,0,793,0,822,0,823,0,825,0,867,0,868,0,870,0,915,0,916,0,934,0,935,0,951,0,952,0,968,0,969,0,986,0,987,0,1020,0,1021,0,1036,0,1037,0,1098,0,242,0,243,0,264,0,266,0,267,0,294,0,295,0,316,0,317,0,318,0,320,0,339,0,340,0,341,0,343,0,367,0,368,0,370,0,377,0,378,0,379,0,396,0,397,0,398,0,400,0,417,0,418,0,439,0,440,0,441,0,443,0,460,0,461,0,462,0,521,0,522,0,523,0,535,0,536,0,540,0,546,0,588,0,590,0,607,0,610,0,184,0,133,0,134,0,135,0,524,0,525,0,532,0,541,0,543,0,545,0,608,0,178,0,179,0,180,0,181,0,185,0,188,0,189,0,191,0,193,0,194,0,526,0,527,0,528,0,530,0]},{"source":"dart:io/runtime/bindirectory_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbindirectory_patch.dart","uri":"dart:io/runtime/bindirectory_patch.dart","_kind":"library"},"hits":[49,0,51,0,52,0,54,0,56,0,10,0,12,0,15,0,18,0,20,0,23,0,26,0,29,0,32,0,43,0,44,0,61,0,62,0,63,0,64,0,67,0,70,1]},{"source":"dart:io/directory_impl.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fdirectory_impl.dart","uri":"dart:io/directory_impl.dart","_kind":"library"},"hits":[11,0,12,0,13,0,15,0,16,0,19,0,21,0,23,0,24,0,27,0,46,0,47,0,48,0,49,0,52,0,55,0,57,0,60,0,61,0,65,0,66,0,67,0,69,0,73,0,76,0,77,0,78,0,79,0,84,0,85,0,88,0,89,0,90,0,98,0,99,0,100,0,101,0,103,0,106,0,108,0,110,0,121,0,122,0,131,0,133,0,134,0,135,0,138,0,139,0,140,0,144,0,145,0,147,0,149,0,150,0,156,0,157,0,159,0,161,0,162,0,171,0,173,0,174,0,180,0,181,0,183,0,185,0,186,0,187,0,188,0,191,0,194,0,195,0,196,0,204,0,205,0,206,0,207,0,211,0,212,0,213,0,221,0,222,0,223,0,225,0,226,0,227,0,229,0,232,0,234,0,237,0,238,0,241,0,244,0,246,0,247,0,249,0,250,0,251,0,256,0,262,0,264,0,265,0,267,0,269,0,270,0,271,0,272,0,273,0,274,0,275,0,277,0,311,0,312,0,313,0,321,0,322,0,325,0,327,0,328,0,329,0,343,0,344,0,345,0,349,0,350,0,352,0,353,0,356,0,359,0,360,0,361,0,364,0,367,0,371,0,373,0,404,0,405,0,406,0,407,0,410,0,411,0,414,0,417,0,419,0,421,0,423,0,424,0,428,0,429,0,430,0,431,0,432,0,433,0,434,0,435,0,436,0,438,0,439,0,440,0,442,0,443,0,445,0,91,0,92,0,94,0,112,0,113,0,117,0,123,0,124,0,163,0,164,0,167,0,197,0,198,0,214,0,215,0,217,0,330,0,331,0,332,0,333,0,334,0,335,0,337,0,338,0,374,0,375,0,376,0,378,0,380,0,381,0,382,0,384,0,385,0,387,0,388,0,390,0,391,0,393,0,394,0,399,0,114,0]},{"source":"dart:io/service_object.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fservice_object.dart","uri":"dart:io/service_object.dart","_kind":"library"},"hits":[12,0,13,0,14,0,19,0,25,0,26,0,27,0]},{"source":"dart:io/io_sink.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fio_sink.dart","uri":"dart:io/io_sink.dart","_kind":"library"},"hits":[288,0,290,0,292,0,293,0,294,0,296,0,299,0,300,0,301,0,302,0,305,0,306,0,307,0,308,0,310,0,311,0,313,0,314,0,315,0,316,0,321,0,322,0,323,0,326,0,327,0,27,0,28,0,147,0,149,0,152,0,155,0,158,0,159,0,160,0,163,0,164,0,165,0,168,0,171,0,172,0,173,0,176,0,179,0,180,0,181,0,183,0,185,0,186,0,187,0,188,0,189,0,193,0,198,0,199,0,200,0,202,0,205,0,206,0,207,0,208,0,213,0,214,0,215,0,217,0,218,0,219,0,220,0,222,0,225,0,228,0,229,0,232,0,234,0,235,0,236,0,240,0,241,0,242,0,243,0,247,0,248,0,249,0,251,0,252,0,254,0,255,0,256,0,257,0,280,0,194,0,209,0,258,0,260,0,261,0,262,0,265,0,267,0,268,0,270,0,271,0,272,0,276,0]},{"source":"dart:io/runtime/bincommon_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbincommon_patch.dart","uri":"dart:io/runtime/bincommon_patch.dart","_kind":"library"},"hits":[55,0,58,1]},{"source":"dart:io/runtime/bineventhandler_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fruntime%2Fbineventhandler_patch.dart","uri":"dart:io/runtime/bineventhandler_patch.dart","_kind":"library"},"hits":[13,0,10,0]},{"source":"dart:io/platform.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Aio%2Fplatform.dart","uri":"dart:io/platform.dart","_kind":"library"},"hits":[79,0,85,0,90,0,95,0,100,0,105,0,158,0,169,0,178,0,195,0,204,0,211,0,221,0,231,0]},{"source":"dart:_http/overrides.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_http%2Foverrides.dart","uri":"dart:_http/overrides.dart","_kind":"library"},"hits":[35,0,36,0,44,0,49,0,56,0,57,0,58,0,67,0,69,0,70,0,79,0,80,0,87,0,88,0,99,0,102,0,103,0,104,0,105,0,109,0,110,0,111,0,113,0,114,0,116,0]},{"source":"dart:_http","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_http","uri":"dart:_http","_kind":"library"},"hits":[1010,0,1012,0,1026,0,1027,0,2244,0,2246,0,2248,0,2179,0,2180,0,1579,0,1580,0,1582,0,1584,0,1843,0,1845,0,1847,0,1849,0,898,0,899,0,906,0,910,0,362,0,364,0,406,0,412,0,420,0,421,0,2168,0,2169,0,1105,0,1111,0,1112,0,2229,0,2231,0,2232,0,2233,0,2234,0,2236,0]},{"source":"dart:_http/http_headers.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_http%2Fhttp_headers.dart","uri":"dart:_http/http_headers.dart","_kind":"library"},"hits":[781,0,786,0,787,0,788,0,790,0,791,0,800,0,801,0,805,0,807,0,808,0,809,0,810,0,811,0,812,0,813,0,815,0,816,0,817,0,822,0,824,0,826,0,828,0,22,0,25,0,28,0,29,0,30,0,31,0,32,0,33,0,35,0,36,0,37,0,41,0,43,0,44,0,45,0,47,0,48,0,50,0,53,0,54,0,55,0,58,0,60,0,61,0,62,0,65,0,69,0,70,0,71,0,72,0,73,0,74,0,76,0,79,0,80,0,81,0,82,0,83,0,85,0,86,0,87,0,89,0,91,0,92,0,96,0,97,0,98,0,99,0,102,0,103,0,106,0,107,0,108,0,111,0,113,0,114,0,115,0,117,0,118,0,120,0,121,0,125,0,128,0,129,0,131,0,134,0,137,0,139,0,140,0,141,0,142,0,143,0,144,0,148,0,149,0,150,0,151,0,152,0,154,0,155,0,156,0,161,0,163,0,164,0,165,0,166,0,169,0,171,0,172,0,174,0,176,0,179,0,181,0,184,0,186,0,187,0,188,0,189,0,192,0,194,0,195,0,196,0,197,0,200,0,201,0,204,0,205,0,212,0,213,0,215,0,216,0,219,0,220,0,223,0,224,0,231,0,232,0,234,0,235,0,238,0,239,0,242,0,243,0,250,0,251,0,253,0,254,0,257,0,258,0,260,0,266,0,267,0,268,0,271,0,272,0,273,0,274,0,275,0,276,0,277,0,278,0,282,0,286,0,287,0,288,0,289,0,292,0,293,0,297,0,298,0,299,0,303,0,304,0,305,0,309,0,310,0,311,0,315,0,316,0,317,0,321,0,322,0,323,0,326,0,327,0,331,0,334,0,335,0,336,0,337,0,338,0,340,0,344,0,345,0,346,0,348,0,352,0,353,0,354,0,355,0,356,0,358,0,362,0,363,0,364,0,365,0,366,0,368,0,372,0,373,0,374,0,375,0,376,0,378,0,382,0,383,0,384,0,385,0,386,0,387,0,389,0,390,0,392,0,394,0,395,0,398,0,399,0,400,0,404,0,406,0,410,0,411,0,412,0,413,0,414,0,415,0,417,0,420,0,421,0,424,0,425,0,427,0,428,0,430,0,431,0,432,0,433,0,435,0,439,0,441,0,442,0,443,0,446,0,447,0,450,0,451,0,452,0,455,0,456,0,457,0,463,0,464,0,467,0,468,0,469,0,470,0,471,0,472,0,473,0,474,0,475,0,476,0,478,0,479,0,481,0,482,0,483,0,484,0,485,0,488,0,490,0,491,0,495,0,496,0,497,0,512,0,515,0,517,0,580,0,582,0,587,0,588,0,589,0,590,0,591,0,594,0,597,0,598,0,599,0,600,0,601,0,602,0,841,0,843,0,844,0,847,0,849,0,853,0,927,0,928,0,929,0,931,0,932,0,933,0,934,0,935,0,936,0,939,0,940,0,941,0,942,0,943,0,945,0,946,0,948,0,949,0,951,0,952,0,954,0,955,0,956,0,959,0,979,0,980,0,981,0,982,0,983,0,984,0,985,0,988,0,989,0,990,0,991,0,992,0,993,0,994,0,995,0,996,0,614,0,616,0,620,0,625,0,626,0,630,0,632,0,633,0,634,0,638,0,639,0,640,0,641,0,643,0,646,0,647,0,648,0,649,0,650,0,654,0,657,0,768,0,769,0,770,0,771,0,772,0,773,0,792,0,793,0,794,0,796,0,498,0,499,0,500,0,501,0,503,0,505,0,508,0,510,0,518,0,555,0,556,0,557,0,558,0,559,0,560,0,561,0,564,0,565,0,567,0,571,0,572,0,573,0,574,0,856,0,858,0,860,0,861,0,862,0,864,0,867,0,869,0,870,0,871,0,873,0,876,0,877,0,878,0,879,0,881,0,884,0,903,0,904,0,906,0,907,0,908,0,910,0,911,0,912,0,913,0,914,0,915,0,916,0,917,0,918,0,919,0,920,0,921,0,923,0,651,0,661,0,663,0,664,0,665,0,666,0,670,0,672,0,673,0,674,0,675,0,676,0,677,0,679,0,682,0,683,0,684,0,686,0,689,0,690,0,693,0,694,0,695,0,739,0,740,0,741,0,742,0,743,0,744,0,745,0,748,0,749,0,750,0,751,0,754,0,755,0,757,0,759,0,760,0,761,0,763,0,764,0,521,0,523,0,524,0,525,0,526,0,530,0,532,0,533,0,534,0,536,0,539,0,541,0,542,0,543,0,545,0,548,0,549,0,550,0,551,0,885,0,887,0,888,0,889,0,891,0,894,0,896,0,897,0,898,0,900,0,697,0,699,0,700,0,701,0,702,0,703,0,704,0,705,0,707,0,710,0,711,0,713,0,714,0,715,0,716,0,717,0,718,0,720,0,721,0,723,0,724,0,725,0,728,0,729,0,731,0,734,0,735,0]},{"source":"dart:_http/http_impl.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_http%2Fhttp_impl.dart","uri":"dart:_http/http_impl.dart","_kind":"library"},"hits":[2528,0,2529,0,2530,0,2531,0,2532,0,2535,0,2536,0,2537,0,2538,0,2539,0,2500,0,2503,0,2504,0,2509,0,2518,0,2523,0,2542,0,2543,0,2544,0,2545,0,2546,0,2547,0,2551,0,2553,0,2554,0,2555,0,2556,0,2558,0,2559,0,2560,0,2572,0,2574,0,2585,0,2586,0,2590,0,2591,0,2593,0,2594,0,2596,0,2598,0,2600,0,2601,0,2605,0,2606,0,2608,0,2612,0,2613,0,2614,0,2615,0,2616,0,2617,0,2618,0,2619,0,2623,0,2624,0,2625,0,2628,0,2629,0,2630,0,2633,0,2634,0,2637,0,2638,0,2639,0,2641,0,2645,0,2647,0,2648,0,2651,0,2652,0,2653,0,2656,0,2657,0,2658,0,2661,0,2663,0,2664,0,2666,0,2669,0,2670,0,2671,0,2672,0,2680,0,2687,0,2688,0,2690,0,2691,0,2692,0,2693,0,2694,0,2695,0,2701,0,2703,0,2704,0,2710,0,2711,0,2712,0,2713,0,2714,0,2740,0,2742,0,2744,0,2745,0,2795,0,3027,0,3029,0,3031,0,3039,0,3040,0,3043,0,3044,0,3047,0,3048,0,2841,0,2843,0,2845,0,2849,0,2851,0,2852,0,2855,0,2856,0,2859,0,2860,0,2863,0,2864,0,2867,0,2868,0,2871,0,2872,0,2875,0,2876,0,2878,0,2879,0,2882,0,2883,0,2886,0,2888,0,2890,0,2892,0,2894,0,2896,0,2898,0,2900,0,2901,0,2904,0,2905,0,1582,0,1584,0,1585,0,1589,0,1633,0,1634,0,1635,0,1638,0,1640,0,1643,0,1646,0,1648,0,1649,0,1650,0,1651,0,1652,0,1653,0,1654,0,1655,0,1657,0,1661,0,1662,0,1663,0,1664,0,1666,0,1669,0,1672,0,1673,0,1676,0,1678,0,1683,0,1684,0,1755,0,1756,0,1760,0,1761,0,1762,0,1763,0,1766,0,1767,0,1768,0,1769,0,1770,0,1773,0,1776,0,1777,0,1781,0,1782,0,1784,0,1795,0,1802,0,1804,0,1805,0,1808,0,1809,0,1810,0,1811,0,1815,0,1817,0,2824,0,2827,0,2829,0,2830,0,2831,0,2998,0,3001,0,3002,0,3003,0,3006,0,3009,0,3012,0,1828,0,12,0,13,0,14,0,19,0,25,0,26,0,27,0,3131,0,2970,0,2973,0,2974,0,2975,0,2977,0,2978,0,2979,0,2980,0,2983,0,2986,0,2989,0,2990,0,532,0,534,0,535,0,536,0,537,0,540,0,541,0,542,0,545,0,548,0,549,0,550,0,553,0,556,0,557,0,558,0,560,0,561,0,569,0,570,0,571,0,572,0,575,0,576,0,577,0,579,0,582,0,583,0,584,0,585,0,590,0,591,0,592,0,594,0,595,0,596,0,597,0,599,0,602,0,605,0,606,0,609,0,611,0,612,0,613,0,617,0,618,0,619,0,620,0,624,0,625,0,626,0,628,0,629,0,631,0,632,0,633,0,634,0,657,0,1843,0,1846,0,1848,0,1850,0,1852,0,1854,0,1855,0,1856,0,1857,0,1861,0,1862,0,1863,0,1867,0,1868,0,1871,0,1873,0,1874,0,1875,0,1876,0,1879,0,1881,0,1882,0,1883,0,1886,0,1888,0,1889,0,1891,0,1892,0,1895,0,1896,0,1901,0,1903,0,1904,0,1905,0,1906,0,1908,0,1909,0,1910,0,1911,0,1914,0,1916,0,1923,0,1924,0,1925,0,1926,0,1927,0,1928,0,2943,0,2944,0,2951,0,2952,0,2953,0,2954,0,2955,0,2956,0,2957,0,2958,0,2962,0,2807,0,2809,1,2816,0,3057,0,3059,0,3061,0,3062,0,3063,0,3064,0,3065,0,3066,0,3067,0,3073,0,3074,0,3075,0,3076,0,3077,0,3078,0,3079,0,3081,0,3082,0,3083,0,3084,0,3085,0,3086,0,3087,0,3088,0,3089,0,3092,0,3093,0,3094,0,3096,0,3098,0,3099,0,3100,0,3101,0,3102,0,3103,0,3104,0,3105,0,3107,0,3108,0,3109,0,3111,0,3112,0,3115,0,3116,0,3117,0,3120,0,3122,0,3123,0,1167,0,1169,0,1170,0,1173,0,1174,0,1175,0,1177,0,1181,0,2350,0,2351,0,2352,0,2353,0,2354,0,2405,0,2406,0,2409,0,2411,0,2412,0,2413,0,2414,0,2415,0,2416,0,2419,0,2420,0,2422,0,2424,0,2426,0,2432,0,2434,0,2435,0,2436,0,2437,0,2439,0,2440,0,2442,0,2443,0,2444,0,2445,0,2446,0,2447,0,2454,0,2456,0,2458,0,2459,0,2465,0,2466,0,2467,0,2469,0,2470,0,2472,0,2473,0,2475,0,2476,0,2479,0,180,0,182,0,183,0,184,0,187,0,188,0,189,0,190,0,2916,1,2918,0,2919,0,2920,0,2924,0,2925,0,2926,0,155,0,153,0,157,0,159,0,160,0,162,0,167,0,169,0,170,0,171,0,172,0,40,0,41,0,42,0,43,0,45,0,46,0,47,0,48,0,49,0,50,0,53,0,54,0,56,0,57,0,60,0,63,0,64,0,67,0,70,0,71,0,74,0,77,0,78,0,81,0,83,0,84,0,85,0,88,0,89,0,90,0,91,0,95,0,96,0,97,0,98,0,101,0,103,0,105,0,107,0,108,0,109,0,112,0,114,0,115,0,116,0,117,0,118,0,119,0,120,0,1979,0,1971,0,1981,0,1982,0,1983,0,1984,0,1986,0,1987,0,1992,0,1994,0,1997,0,2001,0,2002,0,2003,0,2004,0,2005,0,2008,0,2013,0,2014,0,2015,0,2017,0,2019,0,2022,0,2023,0,2025,0,2026,0,2028,0,2030,0,2031,0,2033,0,2035,0,2036,0,2038,0,2040,0,2041,0,2043,0,2045,0,2046,0,2048,0,2050,0,2051,0,2053,0,2055,0,2056,0,2057,0,2058,0,2064,0,2065,0,2068,0,2069,0,2072,0,2074,0,2077,0,2079,0,2082,0,2084,0,2086,0,2089,0,2091,0,2092,0,2093,0,2094,0,2095,0,2096,0,2100,0,2101,0,2102,0,2109,0,2113,0,2115,0,2118,0,2119,0,2134,0,2138,0,2139,0,2158,0,2159,0,2160,0,2164,0,2165,0,2166,0,2168,0,2169,0,2170,0,2172,0,2176,0,2177,0,2178,0,2182,0,2183,0,2184,0,2188,0,2189,0,2190,0,2196,0,2198,0,2215,0,2219,0,2222,0,2236,0,2239,0,2240,0,2241,0,2242,0,2248,0,2249,0,2250,0,2251,0,2255,0,2256,0,2257,0,2258,0,2262,0,2302,0,2306,0,2307,0,2308,0,2312,0,2313,0,2314,0,2315,0,2318,0,2319,0,2320,0,2321,0,1240,0,1244,0,1245,0,1246,0,1249,0,1251,0,1252,0,1253,0,1254,0,1256,0,1257,0,1260,0,1261,0,1263,0,1267,0,1268,0,1273,0,1275,0,1276,0,1277,0,1278,0,1279,0,1280,0,1284,0,1287,0,1291,0,1292,0,1293,0,1294,0,1296,0,1297,0,1298,0,1300,0,1302,0,1308,0,1339,0,1340,0,1341,0,1344,0,1345,0,1349,0,1352,0,1367,0,1369,0,1372,0,1373,0,1374,0,1375,0,1379,0,1380,0,1381,0,1382,0,1384,0,1385,0,1386,0,1387,0,1391,0,1392,0,1393,0,1395,0,1396,0,1397,0,1398,0,1399,0,1441,0,1443,0,1445,0,1448,0,1450,0,1452,0,1453,0,1456,0,1457,0,1458,0,1459,0,1461,0,1462,0,1472,0,1473,0,1474,0,1476,0,1477,0,1478,0,1481,0,1482,0,1483,0,1484,0,1486,0,1487,0,1489,0,1490,0,1491,0,1495,0,1496,0,1497,0,1500,0,1501,0,1502,0,1504,0,1507,0,1508,0,1509,0,1510,0,1512,0,1513,0,1515,0,1516,0,1520,0,1539,0,1540,0,1543,0,1546,0,1547,0,1548,0,1550,0,1551,0,1552,0,1553,0,1556,0,1557,0,1558,0,1560,0,1561,0,1028,0,1033,0,1034,0,1036,0,1040,0,1041,0,1042,0,1043,0,1045,0,1048,0,1049,0,1050,0,1053,0,1054,0,1055,0,1056,0,1059,0,1060,0,1061,0,1062,0,1065,0,1067,0,1068,0,1070,0,1071,0,1074,0,1075,0,1078,0,1083,0,1084,0,1085,0,1086,0,1088,0,1090,0,1091,0,1094,0,1095,0,1099,0,1110,0,1111,0,1113,0,1117,0,1119,0,1120,0,1122,0,1128,0,1129,0,1132,0,1133,0,1135,0,1136,0,1138,0,1139,0,1140,0,1143,0,1144,0,1145,0,1146,0,1147,0,1149,0,1152,0,1155,0,1156,0,1157,0,1158,0,1159,0,295,0,299,0,287,0,302,0,303,0,305,0,306,0,307,0,308,0,311,0,312,0,313,0,314,0,316,0,320,0,323,0,324,0,325,0,326,0,327,0,328,0,329,0,330,0,335,0,339,0,342,0,346,0,348,0,350,0,352,0,353,0,354,0,355,0,356,0,360,0,361,0,362,0,370,0,372,0,376,0,377,0,379,0,380,0,381,0,382,0,384,0,388,0,389,0,390,0,393,0,395,0,397,0,398,0,400,0,403,0,405,0,406,0,408,0,411,0,459,0,462,0,464,0,465,0,468,0,473,0,475,0,479,0,480,0,481,0,482,0,485,0,487,0,488,0,489,0,490,0,493,0,494,0,495,0,497,0,499,0,508,0,511,0,665,0,667,0,669,0,670,0,671,0,673,0,676,0,677,0,678,0,679,0,682,0,683,0,684,0,685,0,687,0,688,0,690,0,691,0,692,0,693,0,698,0,699,0,700,0,703,0,704,0,204,0,207,0,208,0,209,0,210,0,213,0,215,0,216,0,217,0,218,0,219,0,220,0,221,0,228,0,230,0,234,0,236,0,237,0,238,0,240,0,241,0,242,0,245,0,247,0,249,0,251,0,254,0,256,0,259,0,261,0,262,0,263,0,265,0,267,0,270,0,273,0,276,0,278,0,279,0,280,0,790,0,793,0,796,0,798,0,799,0,800,0,803,0,804,0,805,0,806,0,809,0,810,0,811,0,812,0,815,0,816,0,817,0,818,0,819,0,822,0,823,0,824,0,825,0,828,0,832,0,835,0,836,0,843,0,845,0,847,0,848,0,849,0,851,0,852,0,857,0,858,0,861,0,862,0,864,0,866,0,867,0,868,0,869,0,870,0,871,0,873,0,874,0,876,0,879,0,880,0,881,0,882,0,883,0,884,0,889,0,890,0,891,0,892,0,896,0,897,0,902,0,905,0,906,0,907,0,908,0,909,0,912,0,913,0,914,0,918,0,920,0,922,0,924,0,926,0,928,0,930,0,932,0,934,0,936,0,938,0,940,0,942,0,944,0,946,0,948,0,950,0,952,0,954,0,956,0,958,0,960,0,962,0,964,0,966,0,968,0,970,0,972,0,974,0,976,0,978,0,980,0,982,0,984,0,986,0,988,0,990,0,992,0,994,0,996,0,999,0,720,0,723,0,724,0,730,0,731,0,734,0,735,0,736,0,739,0,740,0,741,0,744,0,745,0,746,0,747,0,750,0,751,0,752,0,755,0,756,0,760,0,763,0,764,0,765,0,768,0,769,0,770,0,771,0,773,0,778,0,3134,0,3135,0,3137,0,3138,0,3139,0,2505,0,2524,0,2561,0,2562,0,2563,0,2565,0,2575,0,2577,0,2578,0,2579,0,2582,0,2583,0,2673,0,2674,0,2677,0,2681,0,2746,0,2747,0,2748,0,2752,0,2754,0,2755,0,2756,0,2757,0,2758,0,2759,0,2760,0,2761,0,2763,0,2764,0,2767,0,2768,0,2769,0,2770,0,2772,0,2773,0,2774,0,2776,0,2779,0,2780,0,2781,0,2785,0,2786,0,2787,0,2789,0,1592,0,1594,0,1595,0,1597,0,1604,0,1605,0,1607,0,1613,0,1614,0,1616,0,1617,0,1618,0,1619,0,1620,0,1622,0,1623,0,1624,0,1626,0,1627,0,1629,0,1686,0,1689,0,1737,0,1740,0,1747,0,1749,0,1750,0,1757,0,1785,0,1787,0,1789,0,1790,0,1791,0,1792,0,1793,0,1794,0,1796,0,1797,0,1798,0,1818,0,1819,0,563,0,564,0,586,0,635,0,637,0,638,0,639,0,642,0,644,0,645,0,647,0,648,0,649,0,653,0,1912,0,1918,0,1920,0,1929,0,1930,0,1932,0,1933,0,1934,0,1936,0,1937,0,1944,0,1945,0,1947,0,1948,0,1949,0,2355,0,2357,0,2362,0,2363,0,2364,0,2365,0,2366,0,2367,0,2369,0,2370,0,2371,0,2372,0,2394,0,2395,0,2396,0,2397,0,2398,0,2399,0,2401,0,2427,0,2428,0,161,0,2127,0,2128,0,2130,0,2142,0,2144,0,2146,0,2147,0,2148,0,2152,0,2153,0,2191,0,2200,0,2201,0,2202,0,2203,0,2204,0,2205,0,2206,0,2208,0,2216,0,2223,0,2224,0,2226,0,2264,0,2266,0,2267,0,2268,0,2269,0,2270,0,2271,0,2272,0,2279,0,2281,0,2282,0,2283,0,2284,0,2285,0,2287,0,2288,0,2289,0,2292,0,2293,0,2294,0,2296,0,2298,0,1309,0,1311,0,1312,0,1313,0,1314,0,1315,0,1316,0,1317,0,1318,0,1321,0,1322,0,1324,0,1325,0,1326,0,1327,0,1329,0,1330,0,1331,0,1336,0,1353,0,1354,0,1356,0,1357,0,1358,0,1359,0,1360,0,1403,0,1406,0,1407,0,1408,0,1409,0,1410,0,1411,0,1413,0,1414,0,1415,0,1417,0,1420,0,1421,0,1424,0,1428,0,1464,0,1465,0,1466,0,1467,0,1079,0,1080,0,1101,0,1102,0,1103,0,1104,0,1105,0,317,0,363,0,364,0,365,0,366,0,412,0,414,0,422,0,424,0,425,0,428,0,430,0,431,0,434,0,436,0,438,0,442,0,444,0,445,0,447,0,448,0,449,0,451,0,452,0,454,0,455,0,513,0,514,0,853,0,898,0,1606,0,1608,0,1609,0,1610,0,1690,0,1691,0,1713,0,1714,0,1715,0,1717,0,1718,0,1719,0,1724,0,1725,0,1726,0,1728,0,1729,0,1730,0,1733,0,1738,0,1742,0,1743,0,565,0,1939,0,1940,0,1941,0,2358,0,2373,0,2374,0,2375,0,2376,0,2377,0,2378,0,2379,0,2380,0,2381,0,2382,0,2385,0,2389,0,2391,0,2392,0,2120,0,2121,0,2122,0,1429,0,1430,0,1431,0,1432,0,1433,0,1434,0,415,0,416,0,417,0,418,0,1692,0,1693,0,1694,0,1697,0,1699,0,1700,0,1701,0,1703,0,1704,0,1706,0]},{"source":"dart:_http/websocket_impl.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_http%2Fwebsocket_impl.dart","uri":"dart:_http/websocket_impl.dart","_kind":"library"},"hits":[397,0,844,0,846,0,847,0,848,0,852,0,853,0,854,0,856,0,860,0,861,0,862,0,864,0,868,0,869,0,870,0,871,0,872,0,876,0,877,0,878,0,880,0,881,0,882,0,883,0,884,0,885,0,902,0,903,0,905,0,907,0,909,0,913,0,914,0,915,0,916,0,918,0,919,0,920,0,922,0,923,0,924,0,925,0,927,0,930,0,931,0,936,0,937,0,940,0,941,0,942,0,943,0,946,0,947,0,948,0,949,0,1112,0,1115,0,1116,0,1117,0,1118,0,1120,0,1121,0,1157,0,1158,0,1160,0,1165,0,1166,0,1168,0,982,0,985,0,986,0,987,0,990,0,992,0,993,0,994,0,996,0,998,0,999,0,1000,0,1001,0,1002,0,1003,0,1004,0,1005,0,1006,0,1033,0,1077,0,1079,0,1085,0,1087,0,1089,0,1091,0,1102,0,1103,0,1104,0,1171,0,1173,0,1177,0,1179,0,1180,0,1181,0,1182,0,1184,0,1186,0,1196,0,1198,0,1199,0,1200,0,1202,0,1203,0,1206,0,1207,0,1208,0,1210,0,1213,0,1214,0,1217,0,1218,0,1220,0,1221,0,1222,0,1224,0,1225,0,1226,0,1228,0,1234,0,1235,0,1237,0,1239,0,1249,0,1252,0,1254,0,1255,0,1258,0,1259,0,1260,0,1261,0,1262,0,1264,0,1265,0,1266,0,1269,0,1270,0,1272,0,1273,0,1274,0,1275,0,1276,0,1284,0,1286,0,1287,0,1296,0,1298,0,1299,0,1300,0,1301,0,1302,0,1303,0,1304,0,52,0,53,0,41,0,573,0,580,0,581,0,582,0,583,0,588,0,589,0,590,0,591,0,596,0,597,0,599,0,600,0,601,0,603,0,604,0,607,0,608,0,611,0,612,0,613,0,616,0,619,0,620,0,621,0,624,0,625,0,626,0,627,0,628,0,631,0,636,0,639,0,640,0,643,0,644,0,645,0,648,0,649,0,102,0,104,0,106,0,115,0,116,0,119,0,120,0,213,1,126,0,127,0,129,0,130,0,131,0,133,0,134,0,136,0,137,0,138,0,139,0,140,0,142,0,144,0,147,0,149,0,150,0,151,0,153,0,157,0,158,0,159,0,160,0,165,0,166,0,168,0,170,0,171,0,173,0,175,0,177,0,178,0,179,0,180,0,181,0,182,0,184,0,185,0,186,0,187,0,188,0,189,0,190,0,191,0,194,0,198,0,199,0,200,0,201,0,205,0,206,0,207,0,208,0,214,0,216,0,217,0,220,0,221,0,222,0,223,0,225,0,226,0,227,0,229,0,233,0,238,0,242,0,245,0,247,0,248,0,249,0,250,0,252,0,253,0,254,0,255,0,258,0,259,0,261,0,263,0,264,0,265,0,267,0,268,0,269,0,273,0,274,0,275,0,279,0,280,0,281,0,282,0,284,0,286,0,287,0,289,0,290,0,294,0,295,0,296,0,299,0,302,0,303,0,304,0,305,0,306,0,307,0,309,0,310,0,312,0,313,0,316,0,318,0,321,0,325,0,326,0,327,0,328,0,329,0,332,0,333,0,334,0,336,0,337,0,340,0,342,0,345,0,346,0,347,0,348,0,349,0,350,0,351,0,352,0,354,0,355,0,356,0,358,0,359,0,362,0,363,0,366,0,367,0,370,0,371,0,374,0,377,0,378,0,379,0,380,0,383,0,384,0,385,0,386,0,387,0,388,0,389,0,390,0,391,0,402,0,415,0,417,0,418,0,426,0,429,0,430,0,433,0,434,0,435,0,436,0,437,0,438,0,440,0,442,0,446,0,448,0,449,0,452,0,453,0,454,0,455,0,481,0,486,0,487,0,488,0,494,0,499,0,501,0,505,0,507,0,511,0,512,0,513,0,515,0,517,0,518,0,520,0,521,0,522,0,525,0,526,0,535,0,536,0,539,0,543,0,547,0,548,0,551,0,552,0,555,0,664,0,665,0,668,0,669,0,679,0,680,0,681,0,684,0,685,0,691,0,693,0,694,0,697,0,699,0,701,0,704,0,705,0,710,0,713,0,714,0,717,0,718,0,719,0,722,0,723,0,724,0,726,0,729,0,730,0,733,0,734,0,737,0,738,0,739,0,740,0,745,0,748,0,751,0,752,0,753,0,754,0,756,0,760,0,761,0,762,0,764,0,767,0,768,0,770,0,771,0,775,0,776,0,779,0,780,0,781,0,782,0,787,0,790,0,791,0,793,0,794,0,795,0,796,0,797,0,799,0,804,0,805,0,808,0,809,0,811,0,813,0,814,0,815,0,819,0,820,0,827,0,829,0,886,0,887,0,888,0,889,0,890,0,891,0,892,0,893,0,896,0,897,0,921,0,932,0,933,0,1122,0,1123,0,1124,0,1126,0,1128,0,1130,0,1131,0,1132,0,1133,0,1135,0,1138,0,1139,0,1140,0,1141,0,1142,0,1143,0,1144,0,1145,0,1146,0,1148,0,1150,0,1153,0,1154,0,1155,0,1161,0,1162,0,1163,0,1007,0,1010,0,1011,0,1014,0,1017,0,1018,0,1019,0,1020,0,1021,0,1022,0,1024,0,1027,0,1028,0,1029,0,1032,0,1042,0,1043,0,1044,0,1045,0,1046,0,1048,0,1050,0,1052,0,1054,0,1055,0,1056,0,1057,0,1058,0,1059,0,1061,0,1062,0,1063,0,1066,0,1069,0,1071,0,1093,0,1094,0,1099,0,1187,0,1188,0,1189,0,1241,0,1242,0,1243,0,1244,0,1245,0,107,0,108,0,110,0,419,0,420,0,421,0,422,0,423,0,458,0,461,0,462,0,463,0,464,0,465,0,466,0,467,0,468,0,470,0,473,0,475,0,476,0,489,0,490,0,496,0,497,0,544,0,670,0,671,0,672,0,674,0,741,0,1034,0,1036,0,1039,0,1072,0,1191,0,477,0,1037,0]},{"source":"dart:_http/crypto.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_http%2Fcrypto.dart","uri":"dart:_http/crypto.dart","_kind":"library"},"hits":[45,0,46,0,47,0,48,0,53,0,54,0,55,0,56,0,58,0,61,0,63,0,64,0,69,0,70,0,72,0,75,0,77,0,81,0,82,0,83,0,84,0,85,0,86,0,87,0,88,0,90,0,91,0,92,0,99,0,100,0,101,0,102,0,103,0,104,0,105,0,106,0,107,0,108,0,109,0,110,0,111,0,114,0,117,0,119,0,120,0,121,0,127,0,128,0,129,0,130,0,131,0,132,0,137,0,138,0,139,0,144,0,145,0,146,0,147,0,149,0,150,0,152,0,155,0,156,0,157,0,158,0,159,0,162,0,163,0,164,0,165,0,320,0,321,0,322,0,323,0,324,0,328,0,329,0,355,0,358,0,359,0,360,0,361,0,366,0,367,0,368,0,370,0,371,0,372,0,373,0,374,0,375,0,377,0,378,0,384,0,385,0,389,0,390,0,391,0,392,0,191,0,193,0,194,0,195,0,199,0,200,0,201,0,204,0,205,0,206,0,210,0,211,0,212,0,214,0,215,0,216,0,218,0,222,0,223,0,233,0,234,0,237,0,238,0,239,0,240,0,244,0,245,0,246,0,247,0,253,0,256,0,257,0,258,0,259,0,260,0,261,0,262,0,263,0,264,0,265,0,266,0,271,0,272,0,273,0,274,0,275,0,276,0,282,0,283,0,284,0,285,0,287,0,288,0,289,0,291,0,297,0,298,0,299,0,300,0,301,0,302,0,303,0,304,0,306,0,308,0,309,0,310,0,312,0,313,0,399,0,400,0,402,0,403,0,404,0,405,0,406,0,410,0,411,0,416,0,419,0,420,0,421,0,422,0,423,0,425,0,426,0,427,0,429,0,430,0,432,0,433,0,434,0,435,0,436,0,437,0,438,0,440,0,445,0,447,0,450,0,451,0,452,0,453,0,454,0]},{"source":"dart:_http/websocket.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_http%2Fwebsocket.dart","uri":"dart:_http/websocket.dart","_kind":"library"},"hits":[365,0,356,0,361,0,386,0,391,0,394,0,462,0,467,0,468,0,104,1,116,0,117,0,121,0,122,0,125,0,126,0,128,0,129,0,131,0,132,0,133,0,136,0,137,0,143,0,148,0,151,0,154,0,156,0,157,0,172,0,173,0,174,0,178,0,180,0,183,0,184,0,187,0,190,0,191,0,194,0,195,0,196,0,198,0,199,0,475,0,477,0,248,0,251,0,271,0,274,0,281,0,282,0,130,0]},{"source":"dart:_http/http_parser.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_http%2Fhttp_parser.dart","uri":"dart:_http/http_parser.dart","_kind":"library"},"hits":[119,0,122,0,124,0,125,0,127,0,128,0,129,0,130,0,133,0,134,0,135,0,138,0,139,0,142,0,143,0,146,0,147,0,148,0,150,0,152,0,157,0,158,0,159,0,161,0,162,0,166,0,167,0,168,0,169,0,170,0,189,0,191,0,193,0,194,0,195,0,196,0,197,0,198,0,199,0,201,0,202,0,203,0,206,0,278,0,279,0,297,0,270,0,271,0,274,0,275,0,300,0,302,0,306,0,312,0,313,0,316,0,318,0,320,0,321,0,328,0,329,0,331,0,334,0,339,0,340,0,341,0,342,0,344,0,345,0,346,0,348,0,349,0,350,0,351,0,352,0,354,0,355,0,357,0,358,0,359,0,360,0,361,0,362,0,363,0,364,0,367,0,368,0,369,0,370,0,371,0,372,0,374,0,375,0,376,0,377,0,378,0,379,0,383,0,385,0,386,0,399,0,401,0,402,0,403,0,405,0,406,0,408,0,409,0,410,0,411,0,413,0,414,0,415,0,418,0,419,0,420,0,421,0,423,0,424,0,427,0,428,0,430,0,431,0,432,0,434,0,438,0,439,0,440,0,442,0,443,0,444,0,447,0,448,0,449,0,451,0,454,0,455,0,457,0,458,0,460,0,461,0,462,0,463,0,465,0,470,0,471,0,473,0,474,0,475,0,476,0,478,0,479,0,480,0,481,0,482,0,484,0,485,0,486,0,487,0,488,0,490,0,492,0,496,0,497,0,498,0,500,0,501,0,502,0,503,0,505,0,509,0,510,0,511,0,512,0,514,0,515,0,517,0,518,0,520,0,524,0,525,0,526,0,527,0,528,0,529,0,531,0,532,0,533,0,534,0,536,0,537,0,538,0,540,0,543,0,544,0,546,0,547,0,548,0,553,0,554,0,555,0,556,0,559,0,560,0,561,0,562,0,565,0,567,0,568,0,569,0,571,0,576,0,577,0,578,0,580,0,581,0,583,0,587,0,588,0,589,0,590,0,591,0,594,0,595,0,596,0,597,0,600,0,603,0,604,0,605,0,606,0,607,0,608,0,609,0,612,0,613,0,617,0,618,0,619,0,621,0,622,0,624,0,628,0,629,0,630,0,631,0,632,0,633,0,635,0,636,0,640,0,641,0,642,0,643,0,644,0,646,0,650,0,651,0,652,0,655,0,656,0,657,0,659,0,660,0,661,0,662,0,663,0,665,0,666,0,667,0,669,0,670,0,671,0,672,0,673,0,676,0,678,0,681,0,683,0,684,0,686,0,687,0,688,0,689,0,690,0,693,0,694,0,699,0,700,0,701,0,708,0,709,0,710,0,713,0,714,0,715,0,718,0,719,0,720,0,721,0,722,0,724,0,725,0,729,0,730,0,731,0,735,0,736,0,737,0,738,0,740,0,744,0,745,0,746,0,749,0,750,0,751,0,752,0,755,0,757,0,758,0,759,0,760,0,765,0,766,0,767,0,768,0,769,0,771,0,772,0,773,0,774,0,775,0,777,0,782,0,794,0,795,0,798,0,799,0,800,0,805,0,806,0,808,0,809,0,810,0,813,0,815,0,816,0,818,0,819,0,820,0,821,0,822,0,823,0,825,0,826,0,830,0,831,0,832,0,835,0,839,0,840,0,844,0,845,0,848,0,850,0,854,0,855,0,857,0,860,0,861,0,863,0,866,0,867,0,868,0,870,0,876,0,877,0,878,0,879,0,881,0,882,0,885,0,887,0,888,0,891,0,892,0,893,0,894,0,895,0,899,0,900,0,901,0,902,0,903,0,904,0,905,0,906,0,908,0,909,0,911,0,912,0,913,0,914,0,915,0,917,0,918,0,920,0,923,0,924,0,925,0,928,0,929,0,932,0,933,0,934,0,935,0,938,0,939,0,942,0,943,0,944,0,945,0,946,0,947,0,949,0,951,0,955,0,961,0,965,0,966,0,967,0,968,0,973,0,974,0,975,0,979,0,980,0,981,0,982,0,983,0,984,0,985,0,987,0,991,0,996,0,1024,0,1025,0,1026,0,1027,0,1030,0,1032,0,1033,0,1034,0,1035,0,1036,0,1037,0,1038,0,1040,0,1041,0,1044,0,1045,0,1046,0,1047,0,1050,0,1051,0,1056,0,1057,0,1058,0,1059,0,1060,0,171,0,172,0,173,0,174,0,177,0,178,0,179,0,281,0,282,0,284,0,285,0,286,0,288,0,289,0,290,0,292,0,293,0,294,0,998,0,999,0,1001,0,1002,0,1004,0,1005,0,1007,0,1008,0,1010,0,1011,0,1013,0,1014,0,1016,0,1017,0,1018,0,1019,0,1021,0,1022,0]},{"source":"dart:_http/http_session.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_http%2Fhttp_session.dart","uri":"dart:_http/http_session.dart","_kind":"library"},"hits":[109,0,111,0,113,0,114,0,117,0,119,0,120,0,123,0,124,0,126,0,127,0,131,0,132,0,133,0,134,0,137,0,138,0,141,0,142,0,143,0,146,0,147,0,149,0,150,0,155,0,156,0,157,0,161,0,162,0,163,0,165,0,166,0,168,0,170,0,171,0,172,0,174,0,175,0,177,0,180,0,181,0,183,0,184,0,185,0,186,0,190,0,192,0,193,0,194,0,195,0,199,0,200,0,201,0,202,0,25,0,27,0,28,0,29,0,30,0,35,0,36,0,37,0,40,0,42,0,44,0,45,0,49,0,50,0,51,0,52,0,53,0,56,0,57,0,58,0,59,0,60,0,63,0,64,0,67,0,69,0,70,0,73,0,74,0,76,0,77,0,80,0,81,0,82,0,84,0,85,0,88,0,89,0,90,0,91,0,92,0,94,0]},{"source":"dart:_http/http_date.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3A_http%2Fhttp_date.dart","uri":"dart:_http/http_date.dart","_kind":"library"},"hits":[40,0,57,0,58,0,59,0,60,0,61,0,62,0,63,0,64,0,65,0,66,0,67,0,68,0,69,0,70,0,71,0,72,0,73,0,74,0,91,0,233,0,240,0,241,0,242,0,243,0,244,0,245,0,246,0,247,0,249,0,250,0,251,0,252,0,253,0,254,0,255,0,256,0,258,0,259,0,263,0,326,0,327,0,328,0,330,0,331,0,332,0,340,0,341,0,343,0,344,0,345,0,347,0,349,0,352,0,353,0,354,0,363,0,366,0,367,0,368,0,369,0,370,0,372,0,373,0,375,0,377,0,378,0,379,0,380,0,381,0,382,0,383,0,384,0,386,0,158,0,159,0,160,0,162,0,163,0,164,0,166,0,169,0,172,0,173,0,174,0,175,0,176,0,177,0,178,0,179,0,184,0,185,0,186,0,187,0,191,0,192,0,197,0,200,0,201,0,202,0,203,0,204,0,205,0,206,0,207,0,210,0,212,0,213,0,215,0,217,0,218,0,220,0,222,0,223,0,227,0,228,0,229,0,281,0,282,0,285,0,287,0,288,0,289,0,290,0,291,0,292,0,293,0,297,0,298,0,299,0,300,0,301,0,302,0,303,0,304,0,305,0,309,0,310,0,311,0,315,0,316,0,317,0,320,0,322,0,323,0]},{"source":"dart:cli/wait_for.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acli%2Fwait_for.dart","uri":"dart:cli/wait_for.dart","_kind":"library"},"hits":[61,1,57,0,59,0,49,1,113,0,118,0,128,0,130,0,134,0,135,0,137,0,139,0,142,0,144,0,147,0,121,0]},{"source":"dart:cli/runtime/bincli_patch.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/dart%3Acli%2Fruntime%2Fbincli_patch.dart","uri":"dart:cli/runtime/bincli_patch.dart","_kind":"library"},"hits":[8,0]},{"source":"file:///Users/cbracken/main.dart","script":{"type":"@Script","fixedId":true,"id":"libraries/1/scripts/file%3A%2F%2F%2FUsers%2Fcbracken%2Fmain.dart","uri":"file:///Users/cbracken/main.dart","_kind":"library"},"hits":[1,1,2,1]}]}
\ No newline at end of file
diff --git a/coverage/lib/src/collect.dart b/coverage/lib/src/collect.dart
index 13134db..1b23138 100644
--- a/coverage/lib/src/collect.dart
+++ b/coverage/lib/src/collect.dart
@@ -81,8 +81,8 @@
VMServiceClient service, VMSourceReport report) async {
var scriptRefs = report.ranges.map((r) => r.script).toSet();
var scripts = <Uri, VMScript>{};
- for (var script in await Future
- .wait<VMScript>(scriptRefs.map((ref) => ref.load()).toList())) {
+ for (var script in await Future.wait<VMScript>(
+ scriptRefs.map((ref) => ref.load()).toList())) {
scripts[script.uri] = script;
}
diff --git a/coverage/lib/src/hitmap.dart b/coverage/lib/src/hitmap.dart
index f11d74a..da7c5ee 100644
--- a/coverage/lib/src/hitmap.dart
+++ b/coverage/lib/src/hitmap.dart
@@ -8,7 +8,9 @@
/// Creates a single hitmap from a raw json object. Throws away all entries that
/// are not resolvable.
-Map<String, Map<int, int>> createHitmap(List<Map> jsonResult) {
+///
+/// `jsonResult` is expected to be a List<Map<String, dynamic>>.
+Map<String, Map<int, int>> createHitmap(List jsonResult) {
// Map of source file to map of line to hit count for that line.
var globalHitMap = <String, Map<int, int>>{};
@@ -73,7 +75,7 @@
var globalHitmap = <String, Map<int, int>>{};
for (var file in files) {
String contents = file.readAsStringSync();
- List<Map<String, dynamic>> jsonResult = json.decode(contents)['coverage'];
+ List jsonResult = json.decode(contents)['coverage'];
mergeHitmaps(createHitmap(jsonResult), globalHitmap);
}
return globalHitmap;
diff --git a/coverage/lib/src/resolver.dart b/coverage/lib/src/resolver.dart
index 4ff6175..15b8e18 100644
--- a/coverage/lib/src/resolver.dart
+++ b/coverage/lib/src/resolver.dart
@@ -80,7 +80,7 @@
String resolveSymbolicLinks(String path) {
var normalizedPath = p.normalize(path);
var type = FileSystemEntity.typeSync(normalizedPath, followLinks: true);
- if (type == FileSystemEntityType.NOT_FOUND) return null;
+ if (type == FileSystemEntityType.notFound) return null;
return new File(normalizedPath).resolveSymbolicLinksSync();
}
diff --git a/coverage/lib/src/run_and_collect.dart b/coverage/lib/src/run_and_collect.dart
index 189a123..d12d130 100644
--- a/coverage/lib/src/run_and_collect.dart
+++ b/coverage/lib/src/run_and_collect.dart
@@ -11,7 +11,7 @@
Future<Map<String, dynamic>> runAndCollect(String scriptPath,
{List<String> scriptArgs,
- bool checked: true,
+ bool checked: false,
String packageRoot,
Duration timeout}) async {
var dartArgs = [
diff --git a/coverage/lib/src/util.dart b/coverage/lib/src/util.dart
index 2e859c7..071d991 100644
--- a/coverage/lib/src/util.dart
+++ b/coverage/lib/src/util.dart
@@ -59,11 +59,11 @@
ServerSocket socket;
try {
- socket = await ServerSocket.bind(InternetAddress.LOOPBACK_IP_V4, 0);
+ socket = await ServerSocket.bind(InternetAddress.loopbackIPv4, 0);
} catch (_) {
// try again v/ V6 only. Slight possibility that V4 is disabled
- socket = await ServerSocket.bind(InternetAddress.LOOPBACK_IP_V6, 0,
- v6Only: true);
+ socket =
+ await ServerSocket.bind(InternetAddress.loopbackIPv6, 0, v6Only: true);
}
try {
diff --git a/coverage/pubspec.yaml b/coverage/pubspec.yaml
index f2d67b3..7f911ce 100644
--- a/coverage/pubspec.yaml
+++ b/coverage/pubspec.yaml
@@ -1,12 +1,12 @@
name: coverage
-version: 0.11.0
+version: 0.12.1
author: Dart Team <misc@dartlang.org>
description: Coverage data manipulation and formatting
homepage: https://github.com/dart-lang/coverage
environment:
- sdk: '>=2.0.0-dev.30 <2.0.0'
+ sdk: '>=2.0.0-dev.64.1 <2.0.0'
dependencies:
- args: '>=1.0.0 <2.0.0'
+ args: '>=1.4.0 <2.0.0'
logging: '>=0.9.0 <0.12.0'
package_config: ">=0.1.5 <2.0.0"
path: '>=0.9.0 <2.0.0'
diff --git a/glob/.travis.yml b/glob/.travis.yml
index 45a6c6e..d93de37 100644
--- a/glob/.travis.yml
+++ b/glob/.travis.yml
@@ -1,27 +1,24 @@
language: dart
-# Speed up builds by using containerization.
-sudo: false
-
dart:
- dev
- stable
# See https://docs.travis-ci.com/user/languages/dart/ for details.
dart_task:
- - test: --platform vm,dartium,firefox
+ - test: --platform vm,firefox
install_dartium: true
- dartanalyzer
-# Only building master means that we don't run two builds for each pull request.
-branches:
- only: [master]
-
matrix:
include:
- dart: stable
dart_task: dartfmt
+# Only building master means that we don't run two builds for each pull request.
+branches:
+ only: [master]
+
cache:
directories:
- $HOME/.pub-cache
diff --git a/glob/BUILD.gn b/glob/BUILD.gn
index 999452e..07b773e 100644
--- a/glob/BUILD.gn
+++ b/glob/BUILD.gn
@@ -1,4 +1,4 @@
-# This file is generated by importer.py for glob-1.1.5
+# This file is generated by importer.py for glob-1.1.6
import("//build/dart/dart_library.gni")
diff --git a/glob/CHANGELOG.md b/glob/CHANGELOG.md
index 1768bab..3c5854c 100644
--- a/glob/CHANGELOG.md
+++ b/glob/CHANGELOG.md
@@ -1,3 +1,7 @@
+## 1.1.6
+
+* Improve support for Dart 2 runtime semantics.
+
## 1.1.5
* Declare support for `async` 2.0.0.
diff --git a/glob/lib/src/ast.dart b/glob/lib/src/ast.dart
index 130342e..809d4bc 100644
--- a/glob/lib/src/ast.dart
+++ b/glob/lib/src/ast.dart
@@ -147,7 +147,7 @@
var text = literal.text;
if (context.style == p.Style.windows) text = text.replaceAll("/", "\\");
- var components = context.split(text);
+ Iterable<String> components = context.split(text);
// If the first component is absolute, that means it's a separator (on
// Windows some non-separator things are also absolute, but it's invalid
diff --git a/glob/pubspec.yaml b/glob/pubspec.yaml
index 8aceccb..6f25684 100644
--- a/glob/pubspec.yaml
+++ b/glob/pubspec.yaml
@@ -1,5 +1,5 @@
name: glob
-version: 1.1.5
+version: 1.1.6
author: "Dart Team <misc@dartlang.org>"
homepage: https://github.com/dart-lang/glob
description: Bash-style filename globbing.
diff --git a/googleapis_auth/.gitignore b/googleapis_auth/.gitignore
index aac1f4f..84b973a 100644
--- a/googleapis_auth/.gitignore
+++ b/googleapis_auth/.gitignore
@@ -1,4 +1,5 @@
.packages
.pub
+.dart_tool/
packages
pubspec.lock
diff --git a/googleapis_auth/BUILD.gn b/googleapis_auth/BUILD.gn
index 32bf420..5297c9d 100644
--- a/googleapis_auth/BUILD.gn
+++ b/googleapis_auth/BUILD.gn
@@ -1,4 +1,4 @@
-# This file is generated by importer.py for googleapis_auth-0.2.5
+# This file is generated by importer.py for googleapis_auth-0.2.5+1
import("//build/dart/dart_library.gni")
diff --git a/googleapis_auth/CHANGELOG.md b/googleapis_auth/CHANGELOG.md
index a640622..1ea34ea 100644
--- a/googleapis_auth/CHANGELOG.md
+++ b/googleapis_auth/CHANGELOG.md
@@ -1,3 +1,8 @@
+
+## 0.2.5+1
+
+* Switch all uppercase constants from `dart:convert` to lowercase.
+
## 0.2.5
* Add an optional `loginHint` parameter to browser oauth2 flow APIs which can be
diff --git a/googleapis_auth/lib/auth.dart b/googleapis_auth/lib/auth.dart
index 86dc554..cb66ff6 100644
--- a/googleapis_auth/lib/auth.dart
+++ b/googleapis_auth/lib/auth.dart
@@ -114,7 +114,7 @@
/// to impersonate if impersonating a user.
factory ServiceAccountCredentials.fromJson(json, {String impersonatedUser}) {
if (json is String) {
- json = JSON.decode(json);
+ json = jsonDecode(json);
}
if (json is! Map) {
throw new ArgumentError('json must be a Map or a String encoding a Map.');
@@ -239,7 +239,7 @@
];
var body = new Stream<List<int>>.fromIterable(
- [(ASCII.encode(formValues.join('&')))]);
+ [(ascii.encode(formValues.join('&')))]);
var request = new RequestImpl('POST', _googleTokenUri, body);
request.headers['content-type'] = 'application/x-www-form-urlencoded';
@@ -256,17 +256,17 @@
}
var object = await response.stream
- .transform(ASCII.decoder)
- .transform(JSON.decoder)
+ .transform(ascii.decoder)
+ .transform(json.decoder)
.first;
- var json = object as Map;
+ var jsonMap = object as Map;
- var idToken = json['id_token'];
- var token = json['access_token'];
- var seconds = json['expires_in'];
- var tokenType = json['token_type'];
- var error = json['error'];
+ var idToken = jsonMap['id_token'];
+ var token = jsonMap['access_token'];
+ var seconds = jsonMap['expires_in'];
+ var tokenType = jsonMap['token_type'];
+ var error = jsonMap['error'];
if (response.statusCode != 200 && error != null) {
throw new RefreshFailedException('Refreshing attempt failed. '
diff --git a/googleapis_auth/lib/src/crypto/asn1.dart b/googleapis_auth/lib/src/crypto/asn1.dart
index b22ef20..67defcd 100644
--- a/googleapis_auth/lib/src/crypto/asn1.dart
+++ b/googleapis_auth/lib/src/crypto/asn1.dart
@@ -100,7 +100,7 @@
return new ASN1Sequence(objects);
default:
invalidFormat(
- 'Unexpected tag $tag at offset ${offset-1} (end: $end).');
+ 'Unexpected tag $tag at offset ${offset - 1} (end: $end).');
}
// Unreachable.
return null;
diff --git a/googleapis_auth/lib/src/crypto/pem.dart b/googleapis_auth/lib/src/crypto/pem.dart
index e9498a5..c3b9041 100644
--- a/googleapis_auth/lib/src/crypto/pem.dart
+++ b/googleapis_auth/lib/src/crypto/pem.dart
@@ -25,8 +25,7 @@
/// Helper function for decoding the base64 in [pemString].
Uint8List _getBytesFromPEMString(String pemString) {
- var lines = LineSplitter
- .split(pemString)
+ var lines = LineSplitter.split(pemString)
.map((line) => line.trim())
.where((line) => line.isNotEmpty)
.toList();
@@ -38,7 +37,7 @@
'begin/end markers expected in a PEM file.');
}
var base64 = lines.sublist(1, lines.length - 1).join('');
- return new Uint8List.fromList(BASE64.decode(base64));
+ return new Uint8List.fromList(base64Decode(base64));
}
/// Helper to decode the ASN.1/DER bytes in [bytes] into an [RSAPrivateKey].
diff --git a/googleapis_auth/lib/src/oauth2_flows/auth_code.dart b/googleapis_auth/lib/src/oauth2_flows/auth_code.dart
index 9aa5f10..fb62eb3 100644
--- a/googleapis_auth/lib/src/oauth2_flows/auth_code.dart
+++ b/googleapis_auth/lib/src/oauth2_flows/auth_code.dart
@@ -36,7 +36,7 @@
var response = await client.post(url);
if (response.statusCode == 200) {
- Map json = JSON.decode(response.body);
+ Map json = jsonDecode(response.body);
var scope = json['scope'];
if (scope is! String) {
throw new Exception(
@@ -62,22 +62,22 @@
];
var body = new Stream<List<int>>.fromIterable(
- <List<int>>[ASCII.encode(formValues.join('&'))]);
+ <List<int>>[ascii.encode(formValues.join('&'))]);
var request = new RequestImpl('POST', uri, body);
request.headers['content-type'] = CONTENT_TYPE_URLENCODED;
var response = await client.send(request);
- Map json = await response.stream
- .transform(UTF8.decoder)
- .transform(JSON.decoder)
+ Map jsonMap = await response.stream
+ .transform(utf8.decoder)
+ .transform(json.decoder)
.first;
- var idToken = json['id_token'];
- var tokenType = json['token_type'];
- var accessToken = json['access_token'];
- var seconds = json['expires_in'];
- var refreshToken = json['refresh_token'];
- var error = json['error'];
+ var idToken = jsonMap['id_token'];
+ var tokenType = jsonMap['token_type'];
+ var accessToken = jsonMap['access_token'];
+ var seconds = jsonMap['expires_in'];
+ var refreshToken = jsonMap['refresh_token'];
+ var error = jsonMap['error'];
if (response.statusCode != 200 && error != null) {
throw new Exception('Failed to exchange authorization code. '
@@ -142,8 +142,7 @@
if (state != null) {
queryValues.add('state=${Uri.encodeQueryComponent(state)}');
}
- return Uri
- .parse('https://accounts.google.com/o/oauth2/auth'
+ return Uri.parse('https://accounts.google.com/o/oauth2/auth'
'?${queryValues.join('&')}')
.toString();
}
diff --git a/googleapis_auth/lib/src/oauth2_flows/jwt.dart b/googleapis_auth/lib/src/oauth2_flows/jwt.dart
index 2ca5eb3..0aaaf54 100644
--- a/googleapis_auth/lib/src/oauth2_flows/jwt.dart
+++ b/googleapis_auth/lib/src/oauth2_flows/jwt.dart
@@ -51,10 +51,10 @@
return claimSet;
}
- var jwtHeaderBase64 = _base64url(ASCII.encode(JSON.encode(jwtHeader())));
- var jwtClaimSetBase64 = _base64url(UTF8.encode(JSON.encode(jwtClaimSet())));
+ var jwtHeaderBase64 = _base64url(ascii.encode(jsonEncode(jwtHeader())));
+ var jwtClaimSetBase64 = _base64url(utf8.encode(jsonEncode(jwtClaimSet())));
var jwtSignatureInput = '$jwtHeaderBase64.$jwtClaimSetBase64';
- var jwtSignatureInputInBytes = ASCII.encode(jwtSignatureInput);
+ var jwtSignatureInputInBytes = ascii.encode(jwtSignatureInput);
var signature = _signer.sign(jwtSignatureInputInBytes);
var jwt = "$jwtSignatureInput.${_base64url(signature)}";
@@ -64,15 +64,15 @@
'assertion=${Uri.encodeComponent(jwt)}';
var body = new Stream<List<int>>.fromIterable(
- <List<int>>[UTF8.encode(requestParameters)]);
+ <List<int>>[utf8.encode(requestParameters)]);
var request =
new RequestImpl('POST', Uri.parse(GOOGLE_OAUTH2_TOKEN_URL), body);
request.headers['content-type'] = CONTENT_TYPE_URLENCODED;
var httpResponse = await _client.send(request);
var object = await httpResponse.stream
- .transform(UTF8.decoder)
- .transform(JSON.decoder)
+ .transform(utf8.decoder)
+ .transform(json.decoder)
.first;
Map response = object as Map;
var tokenType = response['token_type'];
@@ -93,6 +93,6 @@
}
String _base64url(List<int> bytes) {
- return BASE64URL.encode(bytes).replaceAll('=', '');
+ return base64Url.encode(bytes).replaceAll('=', '');
}
}
diff --git a/googleapis_auth/lib/src/oauth2_flows/metadata_server.dart b/googleapis_auth/lib/src/oauth2_flows/metadata_server.dart
index fa92704..934e775 100644
--- a/googleapis_auth/lib/src/oauth2_flows/metadata_server.dart
+++ b/googleapis_auth/lib/src/oauth2_flows/metadata_server.dart
@@ -75,7 +75,7 @@
Future<Map> _getToken() async {
var response = await _client.get(_tokenUrl, headers: _HEADERS);
- return JSON.decode(response.body);
+ return jsonDecode(response.body);
}
Future<String> _getScopes() async {
diff --git a/googleapis_auth/pubspec.yaml b/googleapis_auth/pubspec.yaml
index 302f4d1..e1ca012 100644
--- a/googleapis_auth/pubspec.yaml
+++ b/googleapis_auth/pubspec.yaml
@@ -1,10 +1,10 @@
name: googleapis_auth
-version: 0.2.5
+version: 0.2.5+1
author: Dart Team <misc@dartlang.org>
description: Obtain Access credentials for Google services using OAuth 2.0
homepage: https://github.com/dart-lang/googleapis_auth
environment:
- sdk: '>=2.0.0-dev.13.0 <2.0.0'
+ sdk: '>=2.0.0-dev.56.0 <2.0.0'
dependencies:
crypto: '>=0.9.2 <3.0.0'
http: '>=0.11.1 <0.12.0'
diff --git a/json_schema/.gitignore b/json_schema/.gitignore
index aff650d..2a6ceb1 100644
--- a/json_schema/.gitignore
+++ b/json_schema/.gitignore
@@ -2,6 +2,7 @@
.idea/
.packages
.project
+.dart_tool/
.pub/
*.dart.js
*.iml
diff --git a/json_schema/BUILD.gn b/json_schema/BUILD.gn
index 191076d..74e9998 100644
--- a/json_schema/BUILD.gn
+++ b/json_schema/BUILD.gn
@@ -1,4 +1,4 @@
-# This file is generated by importer.py for json_schema-1.0.8
+# This file is generated by importer.py for json_schema-1.0.9
import("//build/dart/dart_library.gni")
diff --git a/json_schema/Dockerfile b/json_schema/Dockerfile
new file mode 100644
index 0000000..5b3fe37
--- /dev/null
+++ b/json_schema/Dockerfile
@@ -0,0 +1,37 @@
+FROM drydock-prod.workiva.net/workiva/smithy-runner-generator:355624 as build
+
+# Build Environment Vars
+ARG BUILD_ID
+ARG BUILD_NUMBER
+ARG BUILD_URL
+ARG GIT_COMMIT
+ARG GIT_BRANCH
+ARG GIT_TAG
+ARG GIT_COMMIT_RANGE
+ARG GIT_HEAD_URL
+ARG GIT_MERGE_HEAD
+ARG GIT_MERGE_BRANCH
+ARG GIT_SSH_KEY
+ARG KNOWN_HOSTS_CONTENT
+
+WORKDIR /build/
+ADD . /build/
+
+RUN mkdir /root/.ssh
+RUN echo "$KNOWN_HOSTS_CONTENT" > "/root/.ssh/known_hosts"
+RUN echo "$GIT_SSH_KEY" > "/root/.ssh/id_rsa"
+RUN chmod 700 /root/.ssh/
+RUN chmod 600 /root/.ssh/id_rsa
+
+RUN echo "Starting the script sections" && \
+ eval "$(ssh-agent -s)" && \
+ ssh-add /root/.ssh/id_rsa && \
+ pub get && \
+ git config remote.origin.url "git@github.com:Workiva/semver-audit-dart.git" && \
+ git clone ssh://git@github.com/workiva/semver-audit-dart.git --branch 1.4.0 && \
+ git config remote.origin.url "git@github.com:Workiva/json_schema.git" && \
+ pub global activate --source path ./semver-audit-dart && \
+ pub global run semver_audit report --repo Workiva/json_schema && \
+ echo "Script sections completed"
+ARG BUILD_ARTIFACTS_BUILD=/build/pubspec.lock
+FROM scratch
diff --git a/json_schema/lib/src/json_schema/schema.dart b/json_schema/lib/src/json_schema/schema.dart
index 3fcf953..52f80b1 100644
--- a/json_schema/lib/src/json_schema/schema.dart
+++ b/json_schema/lib/src/json_schema/schema.dart
@@ -607,7 +607,7 @@
/// Maps any non-key top level property to its original value
Map<String, dynamic> _freeFormMap = {};
- Completer _thisCompleter = new Completer();
+ Completer<Schema> _thisCompleter = new Completer<Schema>();
List<Future<Schema>> _retrievalRequests = [];
/// Set of strings to gaurd against path cycles
diff --git a/json_schema/pubspec.yaml b/json_schema/pubspec.yaml
index d7a14c5..870cfa1 100644
--- a/json_schema/pubspec.yaml
+++ b/json_schema/pubspec.yaml
@@ -1,5 +1,5 @@
name: json_schema
-version: 1.0.8
+version: 1.0.9
authors:
- Michael Carter <michael.carter@workiva.com>
diff --git a/json_schema/smithy.yaml b/json_schema/smithy.yaml
index b9debae..9372f30 100644
--- a/json_schema/smithy.yaml
+++ b/json_schema/smithy.yaml
@@ -2,10 +2,17 @@
language: dart
# dart 1.24.2
-runner_image: drydock-prod.workiva.net/workiva/smithy-runner-generator:179735
+runner_image: drydock-prod.workiva.net/workiva/smithy-runner-generator:355624
script:
- pub get
+ # Clone Semver Audit repo at tag
+ - git config remote.origin.url "git@github.com:Workiva/semver-audit-dart.git"
+ - git clone ssh://git@github.com/workiva/semver-audit-dart.git --branch 1.4.0
+ - git config remote.origin.url "git@github.com:Workiva/json_schema.git"
+ # Run the audit
+ - pub global activate --source path ./semver-audit-dart
+ - pub global run semver_audit report --repo Workiva/json_schema
artifacts:
build:
diff --git a/node_preamble/BUILD.gn b/node_preamble/BUILD.gn
index 30d1e7f..3415b8d 100644
--- a/node_preamble/BUILD.gn
+++ b/node_preamble/BUILD.gn
@@ -1,4 +1,4 @@
-# This file is generated by importer.py for node_preamble-1.4.2
+# This file is generated by importer.py for node_preamble-1.4.3
import("//build/dart/dart_library.gni")
diff --git a/node_preamble/CHANGELOG.md b/node_preamble/CHANGELOG.md
index b66850b..e7bd571 100644
--- a/node_preamble/CHANGELOG.md
+++ b/node_preamble/CHANGELOG.md
@@ -1,3 +1,8 @@
+## 1.4.3
+
+* Add Node detector for Browserify/Webpack-type environments. (thanks to @lexaknyazev for reporting!)
+* Add examples for pub (thanks @bcko!)
+
## 1.4.2
* Keep `Uri.base` up to date when the current working directory changes.
diff --git a/node_preamble/example/example.dart b/node_preamble/example/example.dart
new file mode 100644
index 0000000..d741e27
--- /dev/null
+++ b/node_preamble/example/example.dart
@@ -0,0 +1,5 @@
+import "package:node_preamble/preamble.dart" as preamble;
+
+main() {
+ print(preamble.getPreamble());
+}
diff --git a/node_preamble/lib/preamble.dart b/node_preamble/lib/preamble.dart
index 3f50954..598242b 100644
--- a/node_preamble/lib/preamble.dart
+++ b/node_preamble/lib/preamble.dart
@@ -1,24 +1,20 @@
library node_preamble;
-final _minified = r"""var self=Object.create(global);self.location={get href(){return"file://"+(e=process.cwd(),"win32"!=process.platform?e:"/"+e.replace(/\\/g,"/"))+"/";var e}},self.scheduleImmediate=setImmediate,self.require=require,self.exports=exports,self.process=process,self.__dirname=__dirname,self.__filename=__filename,function(){var e=null;self.document={get currentScript(){return null==e&&(e={src:function(){try{throw new Error}catch(a){var e=a.stack,r=new RegExp("^ *at [^(]*\\((.*):[0-9]*:[0-9]*\\)$","mg"),l=null;do{var t=r.exec(e);null!=t&&(l=t)}while(null!=t);return l[1]}}()}),e}}}(),self.dartDeferredLibraryLoader=function(e,r,l){try{load(e),r()}catch(e){l(e)}};""";
+final _minified = r"""var self=Object.create(global);self.scheduleImmediate=self.setImmediate?function(e){global.setImmediate(e)}:function(e){setTimeout(e,0)},self.require=require,self.exports=exports,self.process=process,self.__dirname=__dirname,self.__filename=__filename,global.window||(self.location={get href(){return"file://"+(e=process.cwd(),"win32"!=process.platform?e:"/"+e.replace(/\\/g,"/"))+"/";var e}},function(){var e=null;self.document={get currentScript(){return null==e&&(e={src:function(){try{throw new Error}catch(n){var e=n.stack,r=new RegExp("^ *at [^(]*\\((.*):[0-9]*:[0-9]*\\)$","mg"),l=null;do{var t=r.exec(e);null!=t&&(l=t)}while(null!=t);return l[1]}}()}),e}}}(),self.dartDeferredLibraryLoader=function(e,r,l){try{load(e),r()}catch(e){l(e)}});""";
final _normal = r"""
+// make sure to keep this as 'var'
+// we don't want block scoping
var self = Object.create(global);
-// TODO: This isn't really a correct transformation. For example, it will fail
-// for paths that contain characters that need to be escaped in URLs. Once
-// dart-lang/sdk#27979 is fixed, it should be possible to make it better.
-self.location = {
- get href() {
- return "file://" + (function() {
- var cwd = process.cwd();
- if (process.platform != "win32") return cwd;
- return "/" + cwd.replace(/\\/g, "/");
- })() + "/";
- }
-};
+self.scheduleImmediate = self.setImmediate
+ ? function (cb) {
+ global.setImmediate(cb);
+ }
+ : function(cb) {
+ setTimeout(cb, 0);
+ };
-self.scheduleImmediate = setImmediate;
self.require = require;
self.exports = exports;
self.process = process;
@@ -26,42 +22,58 @@
self.__dirname = __dirname;
self.__filename = __filename;
-(function() {
- function computeCurrentScript() {
- try {
- throw new Error();
- } catch(e) {
- var stack = e.stack;
- var re = new RegExp("^ *at [^(]*\\((.*):[0-9]*:[0-9]*\\)$", "mg");
- var lastMatch = null;
- do {
- var match = re.exec(stack);
- if (match != null) lastMatch = match;
- } while (match != null);
- return lastMatch[1];
- }
- }
-
- var cachedCurrentScript = null;
- self.document = {
- get currentScript() {
- if (cachedCurrentScript == null) {
- cachedCurrentScript = {src: computeCurrentScript()};
- }
- return cachedCurrentScript;
+// if we're running in a browser, Dart supports most of this out of box
+// make sure we only run these in Node.js environment
+if (!global.window) {
+ // TODO: This isn't really a correct transformation. For example, it will fail
+ // for paths that contain characters that need to be escaped in URLs. Once
+ // dart-lang/sdk#27979 is fixed, it should be possible to make it better.
+ self.location = {
+ get href() {
+ return "file://" + (function() {
+ var cwd = process.cwd();
+ if (process.platform != "win32") return cwd;
+ return "/" + cwd.replace(/\\/g, "/");
+ })() + "/";
}
};
-})();
-self.dartDeferredLibraryLoader = function(uri, successCallback, errorCallback) {
- try {
- load(uri);
- successCallback();
- } catch (error) {
- errorCallback(error);
- }
-};
-""";
+ (function() {
+ function computeCurrentScript() {
+ try {
+ throw new Error();
+ } catch(e) {
+ var stack = e.stack;
+ var re = new RegExp("^ *at [^(]*\\((.*):[0-9]*:[0-9]*\\)$", "mg");
+ var lastMatch = null;
+ do {
+ var match = re.exec(stack);
+ if (match != null) lastMatch = match;
+ } while (match != null);
+ return lastMatch[1];
+ }
+ }
+
+ var cachedCurrentScript = null;
+ self.document = {
+ get currentScript() {
+ if (cachedCurrentScript == null) {
+ cachedCurrentScript = {src: computeCurrentScript()};
+ }
+ return cachedCurrentScript;
+ }
+ };
+ })();
+
+ self.dartDeferredLibraryLoader = function(uri, successCallback, errorCallback) {
+ try {
+ load(uri);
+ successCallback();
+ } catch (error) {
+ errorCallback(error);
+ }
+ };
+}""";
/// Returns the text of the preamble.
///
diff --git a/node_preamble/pubspec.yaml b/node_preamble/pubspec.yaml
index 24f7e1b..c7e66f1 100644
--- a/node_preamble/pubspec.yaml
+++ b/node_preamble/pubspec.yaml
@@ -1,7 +1,9 @@
name: node_preamble
author: Michael Bullington <mikebullingtn@gmail.com>
homepage: https://github.com/mbullington/node_preamble.dart
-version: 1.4.2
+version: 1.4.3
description: Better node.js preamble for dart2js, use it in your build system.
+environment:
+ sdk: '<2.0.0'
dev_dependencies:
http: '^0.11.0'
diff --git a/source_maps/.travis.yml b/source_maps/.travis.yml
index 25aeabec..a49fc6f 100644
--- a/source_maps/.travis.yml
+++ b/source_maps/.travis.yml
@@ -2,8 +2,6 @@
dart:
- dev
- - stable
-
dart_task:
- test: -p vm,chrome
- dartanalyzer
diff --git a/source_maps/BUILD.gn b/source_maps/BUILD.gn
index 985b88a..7a01f91 100644
--- a/source_maps/BUILD.gn
+++ b/source_maps/BUILD.gn
@@ -1,4 +1,4 @@
-# This file is generated by importer.py for source_maps-0.10.5
+# This file is generated by importer.py for source_maps-0.10.6
import("//build/dart/dart_library.gni")
diff --git a/source_maps/CHANGELOG.md b/source_maps/CHANGELOG.md
index 8ef25be..d8ee77a 100644
--- a/source_maps/CHANGELOG.md
+++ b/source_maps/CHANGELOG.md
@@ -1,3 +1,7 @@
+## 0.10.6
+
+* Require version 2.0.0 of the Dart SDK.
+
## 0.10.5
* Add a `SingleMapping.files` field which provides access to `SourceFile`s
diff --git a/source_maps/lib/builder.dart b/source_maps/lib/builder.dart
index cdba2d2..0769d50 100644
--- a/source_maps/lib/builder.dart
+++ b/source_maps/lib/builder.dart
@@ -55,7 +55,7 @@
}
/// Encodes all mappings added to this builder as a json string.
- String toJson(String fileUrl) => JSON.encode(build(fileUrl));
+ String toJson(String fileUrl) => jsonEncode(build(fileUrl));
}
/// An entry in the source map builder.
diff --git a/source_maps/lib/parser.dart b/source_maps/lib/parser.dart
index e46b783..a500b13 100644
--- a/source_maps/lib/parser.dart
+++ b/source_maps/lib/parser.dart
@@ -24,7 +24,7 @@
// TODO(tjblasi): Ignore the first line of [jsonMap] if the JSON safety string
// `)]}'` begins the string representation of the map.
Mapping parse(String jsonMap, {Map<String, Map> otherMaps, mapUrl}) =>
- parseJson(JSON.decode(jsonMap), otherMaps: otherMaps, mapUrl: mapUrl);
+ parseJson(jsonDecode(jsonMap), otherMaps: otherMaps, mapUrl: mapUrl);
/// Parses a source map or source map bundle directly from a json string.
///
@@ -32,7 +32,7 @@
/// the source map file itself. If it's passed, any URLs in the source
/// map will be interpreted as relative to this URL when generating spans.
Mapping parseExtended(String jsonMap, {Map<String, Map> otherMaps, mapUrl}) =>
- parseJsonExtended(JSON.decode(jsonMap),
+ parseJsonExtended(jsonDecode(jsonMap),
otherMaps: otherMaps, mapUrl: mapUrl);
/// Parses a source map or source map bundle.
diff --git a/source_maps/pubspec.yaml b/source_maps/pubspec.yaml
index 7bf7bcd..2c70f6b 100644
--- a/source_maps/pubspec.yaml
+++ b/source_maps/pubspec.yaml
@@ -1,11 +1,11 @@
name: source_maps
-version: 0.10.5
+version: 0.10.6
author: Dart Team <misc@dartlang.org>
description: Library to programmatically manipulate source map files.
homepage: http://github.com/dart-lang/source_maps
dependencies:
source_span: ^1.3.0
environment:
- sdk: '>=1.8.0 <2.0.0'
+ sdk: '>=2.0.0-dev.17.0 <2.0.0'
dev_dependencies:
test: '>=0.12.0 <0.13.0'
diff --git a/video_player/BUILD.gn b/video_player/BUILD.gn
index 803e4be..8fb456b 100644
--- a/video_player/BUILD.gn
+++ b/video_player/BUILD.gn
@@ -1,4 +1,4 @@
-# This file is generated by importer.py for video_player-0.6.1
+# This file is generated by importer.py for video_player-0.6.4
import("//build/dart/dart_library.gni")
diff --git a/video_player/CHANGELOG.md b/video_player/CHANGELOG.md
index 71352ac..b013600 100644
--- a/video_player/CHANGELOG.md
+++ b/video_player/CHANGELOG.md
@@ -1,3 +1,15 @@
+## 0.6.4
+
+* Android: add support for hls, dash and ss video formats.
+
+## 0.6.3
+
+* iOS: Allow audio playback in silent mode.
+
+## 0.6.2
+
+* `VideoPlayerController.seekTo()` is now frame accurate on both platforms.
+
## 0.6.1
* iOS: add missing observer removals to prevent crashes on deallocation.
diff --git a/video_player/android/build.gradle b/video_player/android/build.gradle
index b3b4d5f..c6e627d 100644
--- a/video_player/android/build.gradle
+++ b/video_player/android/build.gradle
@@ -34,5 +34,8 @@
dependencies {
implementation 'com.google.android.exoplayer:exoplayer-core:2.8.0'
+ implementation 'com.google.android.exoplayer:exoplayer-hls:2.8.0'
+ implementation 'com.google.android.exoplayer:exoplayer-dash:2.8.0'
+ implementation 'com.google.android.exoplayer:exoplayer-smoothstreaming:2.8.0'
}
}
diff --git a/video_player/android/src/main/java/io/flutter/plugins/videoplayer/VideoPlayerPlugin.java b/video_player/android/src/main/java/io/flutter/plugins/videoplayer/VideoPlayerPlugin.java
index 61fd18b..b65373a 100644
--- a/video_player/android/src/main/java/io/flutter/plugins/videoplayer/VideoPlayerPlugin.java
+++ b/video_player/android/src/main/java/io/flutter/plugins/videoplayer/VideoPlayerPlugin.java
@@ -19,14 +19,21 @@
import com.google.android.exoplayer2.Player.DefaultEventListener;
import com.google.android.exoplayer2.SimpleExoPlayer;
import com.google.android.exoplayer2.audio.AudioAttributes;
+import com.google.android.exoplayer2.extractor.DefaultExtractorsFactory;
import com.google.android.exoplayer2.source.ExtractorMediaSource;
import com.google.android.exoplayer2.source.MediaSource;
+import com.google.android.exoplayer2.source.dash.DashMediaSource;
+import com.google.android.exoplayer2.source.dash.DefaultDashChunkSource;
+import com.google.android.exoplayer2.source.hls.HlsMediaSource;
+import com.google.android.exoplayer2.source.smoothstreaming.DefaultSsChunkSource;
+import com.google.android.exoplayer2.source.smoothstreaming.SsMediaSource;
import com.google.android.exoplayer2.trackselection.DefaultTrackSelector;
import com.google.android.exoplayer2.trackselection.TrackSelector;
import com.google.android.exoplayer2.upstream.DataSource;
import com.google.android.exoplayer2.upstream.DefaultDataSourceFactory;
import com.google.android.exoplayer2.upstream.DefaultHttpDataSource;
import com.google.android.exoplayer2.upstream.DefaultHttpDataSourceFactory;
+import com.google.android.exoplayer2.util.Util;
import io.flutter.plugin.common.EventChannel;
import io.flutter.plugin.common.MethodCall;
import io.flutter.plugin.common.MethodChannel;
@@ -83,13 +90,33 @@
true);
}
- MediaSource mediaSource =
- new ExtractorMediaSource.Factory(dataSourceFactory).createMediaSource(uri);
+ MediaSource mediaSource = buildMediaSource(uri, dataSourceFactory);
exoPlayer.prepare(mediaSource);
setupVideoPlayer(eventChannel, textureEntry, result);
}
+ private MediaSource buildMediaSource(Uri uri, DataSource.Factory mediaDataSourceFactory) {
+ int type = Util.inferContentType(uri.getLastPathSegment());
+ switch (type) {
+ case C.TYPE_SS:
+ return new SsMediaSource(
+ uri, null, new DefaultSsChunkSource.Factory(mediaDataSourceFactory), null, null);
+ case C.TYPE_DASH:
+ return new DashMediaSource(
+ uri, null, new DefaultDashChunkSource.Factory(mediaDataSourceFactory), null, null);
+ case C.TYPE_HLS:
+ return new HlsMediaSource(uri, mediaDataSourceFactory, null, null);
+ case C.TYPE_OTHER:
+ return new ExtractorMediaSource(
+ uri, mediaDataSourceFactory, new DefaultExtractorsFactory(), null, null);
+ default:
+ {
+ throw new IllegalStateException("Unsupported type: " + type);
+ }
+ }
+ }
+
private void setupVideoPlayer(
EventChannel eventChannel,
TextureRegistry.SurfaceTextureEntry textureEntry,
diff --git a/video_player/example/lib/main.dart b/video_player/example/lib/main.dart
index 25fe0ae..ab1816a 100644
--- a/video_player/example/lib/main.dart
+++ b/video_player/example/lib/main.dart
@@ -251,11 +251,15 @@
children: <Widget>[
new FlatButton(
child: const Text('BUY TICKETS'),
- onPressed: () {/* ... */},
+ onPressed: () {
+ /* ... */
+ },
),
new FlatButton(
child: const Text('SELL TICKETS'),
- onPressed: () {/* ... */},
+ onPressed: () {
+ /* ... */
+ },
),
],
),
@@ -373,6 +377,23 @@
),
body: new TabBarView(
children: <Widget>[
+ new Column(
+ children: <Widget>[
+ new NetworkPlayerLifeCycle(
+ 'http://www.sample-videos.com/video/mp4/720/big_buck_bunny_720p_20mb.mp4',
+ (BuildContext context, VideoPlayerController controller) =>
+ new AspectRatioVideo(controller),
+ ),
+ new Container(
+ padding: const EdgeInsets.only(top: 20.0),
+ ),
+ new NetworkPlayerLifeCycle(
+ 'http://184.72.239.149/vod/smil:BigBuckBunny.smil/playlist.m3u8',
+ (BuildContext context, VideoPlayerController controller) =>
+ new AspectRatioVideo(controller),
+ ),
+ ],
+ ),
new NetworkPlayerLifeCycle(
'http://www.sample-videos.com/video/mp4/720/big_buck_bunny_720p_20mb.mp4',
(BuildContext context, VideoPlayerController controller) =>
diff --git a/video_player/ios/Classes/VideoPlayerPlugin.m b/video_player/ios/Classes/VideoPlayerPlugin.m
index 9e7da66..7699831 100644
--- a/video_player/ios/Classes/VideoPlayerPlugin.m
+++ b/video_player/ios/Classes/VideoPlayerPlugin.m
@@ -229,7 +229,9 @@
}
- (void)seekTo:(int)location {
- [_player seekToTime:CMTimeMake(location, 1000)];
+ [_player seekToTime:CMTimeMake(location, 1000)
+ toleranceBefore:kCMTimeZero
+ toleranceAfter:kCMTimeZero];
}
- (void)setIsLooping:(bool)isLooping {
@@ -313,6 +315,9 @@
- (void)handleMethodCall:(FlutterMethodCall*)call result:(FlutterResult)result {
if ([@"init" isEqualToString:call.method]) {
+ // Allow audio playback when the Ring/Silent switch is set to silent
+ [[AVAudioSession sharedInstance] setCategory:AVAudioSessionCategoryPlayback error:nil];
+
for (NSNumber* textureId in _players) {
[_registry unregisterTexture:[textureId unsignedIntegerValue]];
[[_players objectForKey:textureId] dispose];
diff --git a/video_player/pubspec.yaml b/video_player/pubspec.yaml
index 757608f..7de6a44 100644
--- a/video_player/pubspec.yaml
+++ b/video_player/pubspec.yaml
@@ -2,7 +2,7 @@
description: Flutter plugin for displaying inline video with other Flutter
widgets on Android and iOS.
author: Flutter Team <flutter-dev@googlegroups.com>
-version: 0.6.1
+version: 0.6.4
homepage: https://github.com/flutter/plugins/tree/master/packages/video_player
flutter:
diff --git a/watcher/.travis.yml b/watcher/.travis.yml
index c977430..2547644 100644
--- a/watcher/.travis.yml
+++ b/watcher/.travis.yml
@@ -8,7 +8,7 @@
# Only building master means that we don't run two builds for each pull request.
branches:
- only: [master, 0.9.7+x]
+ only: [master]
cache:
directories:
diff --git a/watcher/BUILD.gn b/watcher/BUILD.gn
index cbb4f92..a832abc 100644
--- a/watcher/BUILD.gn
+++ b/watcher/BUILD.gn
@@ -1,4 +1,4 @@
-# This file is generated by importer.py for watcher-0.9.7+8
+# This file is generated by importer.py for watcher-0.9.7+9
import("//build/dart/dart_library.gni")
diff --git a/watcher/CHANGELOG.md b/watcher/CHANGELOG.md
index fe30bc6..d8f2d5f 100644
--- a/watcher/CHANGELOG.md
+++ b/watcher/CHANGELOG.md
@@ -1,3 +1,7 @@
+# 0.9.7+9
+
+* Internal changes only.
+
# 0.9.7+8
* Fix Dart 2.0 type issues on Mac and Windows.
diff --git a/watcher/lib/src/constructable_file_system_event.dart b/watcher/lib/src/constructable_file_system_event.dart
index a0f153e..29b7c8d 100644
--- a/watcher/lib/src/constructable_file_system_event.dart
+++ b/watcher/lib/src/constructable_file_system_event.dart
@@ -14,7 +14,7 @@
class ConstructableFileSystemCreateEvent extends _ConstructableFileSystemEvent
implements FileSystemCreateEvent {
- final type = FileSystemEvent.CREATE;
+ final type = FileSystemEvent.create;
ConstructableFileSystemCreateEvent(String path, bool isDirectory)
: super(path, isDirectory);
@@ -24,7 +24,7 @@
class ConstructableFileSystemDeleteEvent extends _ConstructableFileSystemEvent
implements FileSystemDeleteEvent {
- final type = FileSystemEvent.DELETE;
+ final type = FileSystemEvent.delete;
ConstructableFileSystemDeleteEvent(String path, bool isDirectory)
: super(path, isDirectory);
@@ -35,7 +35,7 @@
class ConstructableFileSystemModifyEvent extends _ConstructableFileSystemEvent
implements FileSystemModifyEvent {
final bool contentChanged;
- final type = FileSystemEvent.MODIFY;
+ final type = FileSystemEvent.modify;
ConstructableFileSystemModifyEvent(
String path, bool isDirectory, this.contentChanged)
@@ -48,7 +48,7 @@
class ConstructableFileSystemMoveEvent extends _ConstructableFileSystemEvent
implements FileSystemMoveEvent {
final String destination;
- final type = FileSystemEvent.MOVE;
+ final type = FileSystemEvent.move;
ConstructableFileSystemMoveEvent(
String path, bool isDirectory, this.destination)
diff --git a/watcher/lib/src/directory_watcher/mac_os.dart b/watcher/lib/src/directory_watcher/mac_os.dart
index 5ad9984..9da413a 100644
--- a/watcher/lib/src/directory_watcher/mac_os.dart
+++ b/watcher/lib/src/directory_watcher/mac_os.dart
@@ -244,13 +244,13 @@
// If we previously thought this was a MODIFY, we now consider it to be a
// CREATE or REMOVE event. This is safe for the same reason as above.
- if (type == FileSystemEvent.MODIFY) {
+ if (type == FileSystemEvent.modify) {
type = event.type;
continue;
}
// A CREATE event contradicts a REMOVE event and vice versa.
- assert(type == FileSystemEvent.CREATE || type == FileSystemEvent.DELETE);
+ assert(type == FileSystemEvent.create || type == FileSystemEvent.delete);
if (type != event.type) return null;
}
@@ -258,23 +258,23 @@
// from FSEvents reporting an add that happened prior to the watch
// beginning. If we also received a MODIFY event, we want to report that,
// but not the CREATE.
- if (type == FileSystemEvent.CREATE &&
+ if (type == FileSystemEvent.create &&
hadModifyEvent &&
_files.contains(batch.first.path)) {
- type = FileSystemEvent.MODIFY;
+ type = FileSystemEvent.modify;
}
switch (type) {
- case FileSystemEvent.CREATE:
+ case FileSystemEvent.create:
// Issue 16003 means that a CREATE event for a directory can indicate
// that the directory was moved and then re-created.
// [_eventsBasedOnFileSystem] will handle this correctly by producing a
// DELETE event followed by a CREATE event if the directory exists.
if (isDir) return null;
return new ConstructableFileSystemCreateEvent(batch.first.path, false);
- case FileSystemEvent.DELETE:
+ case FileSystemEvent.delete:
return new ConstructableFileSystemDeleteEvent(batch.first.path, isDir);
- case FileSystemEvent.MODIFY:
+ case FileSystemEvent.modify:
return new ConstructableFileSystemModifyEvent(
batch.first.path, isDir, false);
default:
diff --git a/watcher/lib/src/directory_watcher/windows.dart b/watcher/lib/src/directory_watcher/windows.dart
index db9ca83..0e550ae 100644
--- a/watcher/lib/src/directory_watcher/windows.dart
+++ b/watcher/lib/src/directory_watcher/windows.dart
@@ -132,7 +132,7 @@
// the directory is now gone.
if (event is FileSystemMoveEvent ||
event is FileSystemDeleteEvent ||
- (FileSystemEntity.typeSync(path) == FileSystemEntityType.NOT_FOUND)) {
+ (FileSystemEntity.typeSync(path) == FileSystemEntityType.notFound)) {
for (var path in _files.paths) {
_emitEvent(ChangeType.REMOVE, path);
}
@@ -283,27 +283,27 @@
// If we previously thought this was a MODIFY, we now consider it to be a
// CREATE or REMOVE event. This is safe for the same reason as above.
- if (type == FileSystemEvent.MODIFY) {
+ if (type == FileSystemEvent.modify) {
type = event.type;
continue;
}
// A CREATE event contradicts a REMOVE event and vice versa.
- assert(type == FileSystemEvent.CREATE ||
- type == FileSystemEvent.DELETE ||
- type == FileSystemEvent.MOVE);
+ assert(type == FileSystemEvent.create ||
+ type == FileSystemEvent.delete ||
+ type == FileSystemEvent.move);
if (type != event.type) return null;
}
switch (type) {
- case FileSystemEvent.CREATE:
+ case FileSystemEvent.create:
return new ConstructableFileSystemCreateEvent(batch.first.path, isDir);
- case FileSystemEvent.DELETE:
+ case FileSystemEvent.delete:
return new ConstructableFileSystemDeleteEvent(batch.first.path, isDir);
- case FileSystemEvent.MODIFY:
+ case FileSystemEvent.modify:
return new ConstructableFileSystemModifyEvent(
batch.first.path, isDir, false);
- case FileSystemEvent.MOVE:
+ case FileSystemEvent.move:
return null;
default:
throw 'unreachable';
diff --git a/watcher/lib/src/file_watcher/native.dart b/watcher/lib/src/file_watcher/native.dart
index 8e7dd09..5f56f73 100644
--- a/watcher/lib/src/file_watcher/native.dart
+++ b/watcher/lib/src/file_watcher/native.dart
@@ -49,7 +49,7 @@
}
void _onBatch(List<FileSystemEvent> batch) {
- if (batch.any((event) => event.type == FileSystemEvent.DELETE)) {
+ if (batch.any((event) => event.type == FileSystemEvent.delete)) {
// If the file is deleted, the underlying stream will close. We handle
// emitting our own REMOVE event in [_onDone].
return;
diff --git a/watcher/pubspec.yaml b/watcher/pubspec.yaml
index 3cb9643..2f449ff 100644
--- a/watcher/pubspec.yaml
+++ b/watcher/pubspec.yaml
@@ -1,12 +1,12 @@
name: watcher
-version: 0.9.7+8
+version: 0.9.7+9
author: Dart Team <misc@dartlang.org>
homepage: https://github.com/dart-lang/watcher
description: >
A file system watcher. It monitors changes to contents of directories and
sends notifications when files have been added, removed, or modified.
environment:
- sdk: '>=2.0.0-dev.20.0 <2.0.0'
+ sdk: '>=2.0.0-dev.61.0 <2.0.0'
dependencies:
async: '>=1.10.0 <3.0.0'
path: '>=0.9.0 <2.0.0'